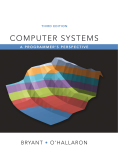
Concept explainers
A.
IP addresses:
- The IP address denotes an unsigned integer that is 32-bit.
- The IP addresses is been stored by network programs in IP address structure.
- The addresses present in IP address structure are stored in network byte order.
- An unsigned 32-bit integer is transformed from host byte order to network byte order by “htonl” function.
- An unsigned 32-bit integer is transformed from network byte order host byte order by “ntohl” function.
- The IP address is presented to humans in a form known as “dotted-decimal” notation.
- Each byte is been represented by its corresponding decimal value and is separated by a period from other bytes.
Passing
- The arguments for “GET” requests are passed in the URI.
- The character “?” separates filename from the arguments.
- The character “&” separates each argument.
- The arguments do not allow spaces in it.
Server passes arguments to child:
- The server calls “fork” to create a child process and calls “execve” to run program in child’s context once it receives a request.
- The child process sets CGI environment variable values.
- The “adder” program can reference it at run time using “getenv” function of linux.
Output is sent by child:
- The dynamic content of a CGI program is to be sent to standard output.
- A CGI program sends dynamic content to standard output.
- It uses “dup2” function for redirecting standard output to connected descriptor associated with client.
- The result written to standard output by CGI program, it goes directly to client.
A.

Explanation of Solution
Modified C code:
//Define method
void clienterror(int fd, char *cause, char *errnum, char *shortmsg, char *longmsg);
//Define method
void echo(int connfd);
//Define main method
int main(int argc, char **argv)
{
//Declare variables
int listenfd, connfd;
//Call method
Getnameinfo((SA *) &clientaddr, clientlen, hostname, MAXLINE,port, MAXLINE, 0);
//Display message
printf("Accepted connection from (%s, %s)\n", hostname, port);
//Call method
echo(connfd);
//Close
Close(connfd);
}
//Define method
void echo(int connfd)
{
//Declare variable
size_t n;
//Declare array
char buf[MAXLINE];
//Declare variable
rio_t rio;
//Call method
Rio_readinitb(&rio, connfd);
//Loop
while ((n = Rio_readlineb(&rio, buf, MAXLINE)) != 0)
{
//If condition satisfies
if (strcmp(buf, "\r\n") == 0)
//Break
break;
//Call method
Rio_writen(connfd, buf, n);
}
}
Explanation:
- The method “echo” declares the variables first.
- It handles one HTTP response/request transaction.
- The value is been read using method “readinitb” and is stored.
- The value is been written into buffer using “written” method.
- The comparison is made until new line occurs.
B.
IP addresses:
- The IP address denotes an unsigned integer that is 32-bit.
- The IP addresses is been stored by network programs in IP address structure.
- The addresses present in IP address structure are stored in network byte order.
- An unsigned 32-bit integer is converted from host byte order to network byte order by “htonl” function.
- An unsigned 32-bit integer is converted from network byte order host byte order by “ntohl” function.
- The IP address is presented to humans in a form known as “dotted-decimal” notation.
- Each byte is been represented by its corresponding decimal value and is separated by a period from other bytes.
Passing program arguments to server:
- The arguments for “GET” requests are passed in the URI.
- The character “?” separates filename from the arguments.
- The character “&” separates each argument.
- The arguments do not allow spaces in it.
Server passes arguments to child:
- The server calls “fork” to create a child process and calls “execve” to run program in child’s context once it receives a request.
- The child process sets CGI environment variable values.
- The “adder” program can reference it at run time using “getenv” function of linux.
Output is sent by child:
- The dynamic content of a CGI program is to be sent to standard output.
- A CGI program sends dynamic content to standard output.
- It uses “dup2” function for redirecting standard output to connected descriptor associated with client.
- The result written to standard output by CGI program, it goes directly to client.
B.

Explanation of Solution
Request to TINY for static content:
GET / HTTP/1.1
Host: localhost:5000
User-Agent: Mozilla/5.0 (X11; Ubuntu; Linux x86_64; rv:53.0) Gecko/20100101 Firefox/53.0
Accept: text/html,application/xhtml+xml,application/xml;q=0.9,*/*;q=0.8
Accept-Language: en-US,en;q=0.5
Accept-Encoding: gzip, deflate
Connection: keep-alive
Explanation:
- The given code denotes a request to “TINY” for static content.
- It echoes each and every request line.
- It echoes each and every request header.
- It takes a particular “Accept-Language” and “Accept-Encoding” for the content.
C.
IP addresses:
- The IP address denotes an unsigned integer that is 32-bit.
- The IP addresses is been stored by network programs in IP address structure.
- The addresses present in IP address structure are stored in network byte order.
- An unsigned 32-bit integer is converted from host byte order to network byte order by “htonl” function.
- An unsigned 32-bit integer is converted from network byte order host byte order by “ntohl” function.
- The IP address is presented to humans in a form known as “dotted-decimal” notation.
- Each byte is been represented by its corresponding decimal value and is separated by a period from other bytes.
Passing program arguments to server:
- The arguments for “GET” requests are passed in the URI.
- The character “?” separates filename from the arguments.
- The character “&” separates each argument.
- The arguments do not allow spaces in it.
Server passes arguments to child:
- The server calls “fork” to create a child process and calls “execve” to run program in child’s context once it receives a request.
- The child process sets CGI environment variable values.
- The “adder” program can reference it at run time using “getenv” function of linux.
Output is sent by child:
- The dynamic content of a CGI program is to be sent to standard output.
- A CGI program sends dynamic content to standard output.
- It uses “dup2” function for redirecting standard output to connected descriptor associated with client.
- The result written to standard output by CGI program, it goes directly to client.
C.

Explanation of Solution
Version of HTTP:
- The version of HTTP used by browser is HTTP 1.1.
- The output from “TINY” is been inspected for determining HTTP version of browser.
- The “TINY” denotes an iterative server that listens for connection requests on ports.
- The “TINY” executes infinite server loop; it accepts a connection request repeatedly.
- It performs the transaction and closes its end of connection.
- Hence, version of HTTP is “HTTP 1.1”.
D.
IP addresses:
- The IP address denotes an unsigned integer that is 32-bit.
- The IP addresses is been stored by network programs in IP address structure.
- The addresses present in IP address structure are stored in network byte order.
- An unsigned 32-bit integer is converted from host byte order to network byte order by “htonl” function.
- An unsigned 32-bit integer is converted from network byte order host byte order by “ntohl” function.
- The IP address is presented to humans in a form known as “dotted-decimal” notation.
- Each byte is been represented by its corresponding decimal value and is separated by a period from other bytes.
Passing program arguments to server:
- The arguments for “GET” requests are passed in the URI.
- The character “?” separates filename from the arguments.
- The character “&” separates each argument.
- The arguments do not allow spaces in it.
Server passes arguments to child:
- The server calls “fork” to create a child process and calls “execve” to run program in child’s context once it receives a request.
- The child process sets CGI environment variable values.
- The “adder” program can reference it at run time using “getenv” function of linux.
Output is sent by child:
- The dynamic content of a CGI program is to be sent to standard output.
- A CGI program sends dynamic content to standard output.
- It uses “dup2” function for redirecting standard output to connected descriptor associated with client.
- The result written to standard output by CGI program, it goes directly to client.
D.

Explanation of Solution
Meaning of HTTP headers:
- The details of each HTTP header is shown below:
- Accept:14.1:
- It can be used for specifying certain media types that are acceptable for response.
- Accept headers are used to indicate that request is limited specifically to a small set of desired types.
- It has same impact as in case of an in-line image request.
- Accept-Encoding: 14.3:
- The request header field is almost similar to “Accept”.
- It restricts the content-coding that are acceptable in response.
- Accept-Language: 14.4:
- The “Accept-Language” request header is similar to that of “Accept”.
- It restricts set of natural languages preferred as response to request.
- Connection: 14.10:
- The connection header field allows sender to specify options that are desired for particular connection.
- It must not be communicated by proxies over further connections.
- Host: 14.23:
- The host request header field would specify internet host and port number of resource being requested.
- The host field value denotes origin server’s naming authority or gateway given by original URL.
- It allows origin server or gateway in differentiating between ambiguous URLs.
- The root “/” server’s URL is used for multiple host names with single IP address.
- User-Agent: 14.43:
- The user-agent request header has information about user agent initiating the request.
- It is used for tracing protocol violations, automated acknowledgment of user agents for avoiding limitations of user agent.
- The requests are been added with the field.
- The field contains multiple product tokens and comments that identifies agent.
- It includes sub products that forms a significant part of user agent.
- The product tokens are listed in order of their significance for application identification.
- Accept:14.1:
Want to see more full solutions like this?
Chapter 11 Solutions
Computer Systems: A Programmer's Perspective (3rd Edition)
- Which of the following best describes the Stream Control Transmission Protocol?a.REliable, message orientedb. Unreliable, message orientedc.REliable, character-orientedd.unrEliable, character-orientedarrow_forwardPlease write the code in the nodejs and use the localhost server to show the current date and time by creating a function. Please write the code only in Node js the address: - http://localhost:8080/v Please write the code in the nodejs and use the localhost server to show the current date and time by creating a function. Please write the code only in Node js the address: - http://localhost:8080/v Please write the code in the nodejs and use the localhost server to show the current date and time by creating a function. Please write the code only in Node js the address: - http://localhost:8080/arrow_forwardPlease do not use a python code already on the internet. The following code must be written in python for this HW assignment. I will provide a skeleten for the code and you must write some lines of code to satisfy the objective of the prorgram. you need to develop a web server that handles one HTTP request on at a time. the web server should acceptand parse the HTTP request, get the requested file from the server’s file system, and create an HTTP responsemessage consisting of the requested file preceded by header lines, and then send the response directly tothe client. If the requested file is not present in the server, the server should send an HTTP “404 NotFound” message back to the client. Dont need the hole code just right the information and code it to the skeleten pictures below.arrow_forward
- Question 1 In this programming problem you'll code up Dijkstra's shortest-path algorithm. Download the following text file: dijkstraData TXT File File Link is: https://d3c33hcgiwev3.cloudfront.net/_dcf1d02570e57d23ab526b1e33ba6f12_dijkstraData.txt?Expires=1656979200&Signature=jDtzBLV8iI~UD6soIHVD2PPZwLD3tcJwuSTS3ZPepSVvyrIuTM5VHstUOqosqy8pYJk~DMDUBfZfuISMJrgpiKuK63qda1~ypGQVdv2cFIp8YHeg-tVjPI5C5rJm~4bA7JtKOu3boIwQ03FlVB17EojSDJ2tPpArMcpA-Yp6Et0_&Key-Pair-Id=APKAJLTNE6QMUY6HBC5A The file contains an adjacency list representation of an undirected weighted graph with 200 vertices labeled 1 to 200. Each row consists of the node tuples that are adjacent to that particular vertex along with the length of that edge. For example, the 6th row has 6 as the first entry indicating that this row corresponds to the vertex labeled 6. The next entry of this row "141,8200" indicates that there is an edge between vertex 6 and vertex 141 that has length 8200. The rest of the pairs of this…arrow_forwardUSING UBUNTU! Show me all code for each step! Be detailed please. * Apache running * All user html directories named pub. Therefore http://googee.nmu.edu/~fred gets mapped to /home/fred/pub. * Make a new user named WWW. The "/" gets mapped to /home/WWW/pub. Therefore http://googee.nmu.edu gets mapped to /home/WWW/pub/ * A basic index.html file in /home/WWW/pub * Setting such that there are no directory listings and no cgis runnable * The URL http://googee.nmu.edu/icons mapped to /home/WWW/Pictures/icons * Logs stored in /var/log/my_wb_logs in combined logfile format * A web analyzer (webalizer = 0.5, analog = 1.0) that shows activity stats accessable via the web. * The user 'someone' is unable to make web pages in his home directory tree, even if he's tricky. No hand written and fast answer with explanationarrow_forwardConsider the following string of ASCII characters that were captured by Wireshark when the browser sent an HTTP GET message (i.e., this is the actual content of an HTTP GET message). The characters <cr><lf> are carriage return and line-feed characters (that is, the italized character string <cr> in the text below represents the single carriage-return character that was contained at that point in the HTTP header). Answer the following questions, indicating where in the HTTP GET message below you will find the answer.arrow_forward
- The If-Modified-Since header can be used to check whether a cached page is still valid. Requests can be made for pages containing images, sound, video, and so on, as well as HTML. Do you think the effectiveness of this technique is better or worse for JPEG images as compared to HTML?arrow_forwardSuppose you are writing JavaScript code to send an asynchronous GET request to the action.pl file on the server. You have written code that defines your request. Which statement should you use next to send it to the server? a. httpReq.send("id=41088"); b. httpReq.XMLHttpRequest("get", "action.pl&id=41088"); c. httpReq.send(null); d. httpReq.open("get", "action.pl&id=41088");arrow_forwardUse the winpractice file for the following exercises. Capture your filtered traffic to a file. Submit the file (with a .txt extension) and the full command you used. For Snort, submit the entire alert.1. Use Windump to filter on all echo reply packets.2. Use Windump to filter on all packets destined for 172.20.42.32. Do not use name resolution.3. Use Snort to alert on any tcp traffic with only the SYN and FIN flags set. Label the alert packets with an appropriate message.arrow_forward
- In this assignment, you will develop a simple Web server in Python that is capable of processing only one request. Specifically, your Web server will (i) Create a connection socket when contacted by a client (browser) (ii) Receive the HTTP request from this connection(iii) Parse the request to determine the specific file being requested(iv) Get the requested file from the server’s file system(v) Create an HTTP response message consisting of the requested file preceded by header lines(vi) Send the response over the TCP connection to the requesting browser. If a browser requests a file that is not present in your server, your server should return a “404 Not Found” error message. Your job is to code the steps above, run your server, and then test your server by sending requests from browsers running on different hosts. If you run your server on a host that already has a Web server running on it, then you should use a different port than port 80 for your Web server. Make sure to test your…arrow_forwardWrite a snort rule to generate an alert for the following: search for the word “HTTP” in the first 20bytes of the payload of a packet that is originating in subnet (192.168.34.0/24) and going toyour subnet (192.168.56.0/24). The alert should read: “HTTP detected”. Could you please also include the nmap command used to scan the target?arrow_forwardMy final question on the day seems to be on properly slicing strings. Currently I have URL = input("Please enter the absolute URL: ")if URL.startswith("https"): print("Type: https")elif URL.startswith("http"): print("http") however I get stuck when trying to slice out the domain and path and the book is only showing simpler commands (startswith, endswith, find, count etc..), so I am unfamiliar with something more complex like URLParse which I saw elsewhere.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
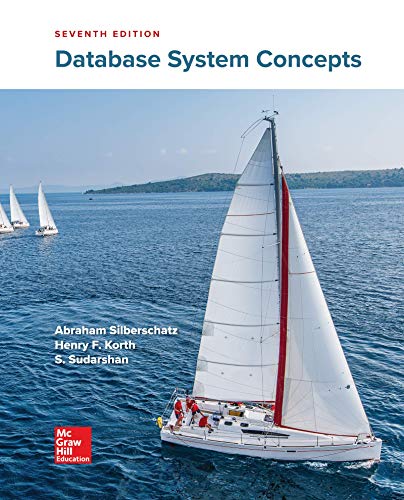
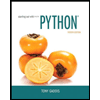
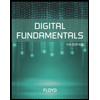
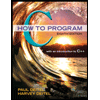
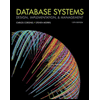
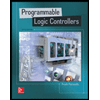