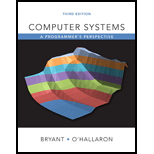
Readers-Writers problem:
- The readers-writers interactions would happen frequently in real systems.
- It has some variations; each is centered on priority of writers and readers.
- The details for “first readers-writers problem” is displayed below:
- This problem favors readers.
- It needs that no reader must be kept waiting lest a writer has already been granted approval to use object.
- There should be no reader waiting due to waiting of writer.
- The details for “second readers-writers problem” is displayed below:
- This problem favors writers.
- It requires that after a writer is set to write, it performs write as fast as possible.
- A reader arriving after writer should wait, even if writer is also waiting.
- The “w” semaphore controls access to critical sections that access shared object.
- The “mutex” semaphore would protect admittance to shared variable “readcnt”.
- It counts number of readers currently in critical section.
- A writer locks “w” mutex each time it would enter critical section and unlocks it each time it leaves.
- This guarantees that there exists at most one writer in critical section at any time point.
- The first reader who enter critical section locks “w” and last reader to leave critical section unlocks it.
- The “w” mutex is ignored by readers who enter and leave while other readers are present.
- A correct solution to either of readers-writers problem could result in starvation.
- A thread is been blocked indefinitely and is failed from making progress.

Explanation of Solution
C code for readers-writers problem:
//Include libraries
#include <stdio.h>
#include "csapp.h"
//Define constants
#define WrteLmt 100000
#define Pple 20
#define N 5
//Declare variable
static int readtms;
//Declare variable
static int writetms;
//Declare semaphore variable
sem_t mtx;
//Declare semaphore variable
sem_t rdrcnt;
//Declare reader method
void *reader(void *vargp)
{
//Loop
while (1)
{
//P operation
P(&rdrcnt);
//P operation
P(&mtx);
//Increment variable
readtms++;
//V operation
V(&mtx);
//V operation
V(&rdrcnt);
}
}
//Declare writer method
void *writer(void *vargp)
{
//Loop
while (1)
{
//P operation
P(&mtx);
//Increment value
writetms++;
//If condition satisfies
if (writetms == WrteLmt)
{
//Display
printf("read/write: %d/%d\n", readtms, writetms);
//Exit
exit(0);
}
//V operation
V(&mtx);
}
}
//Declare init method
void init(void)
{
//Declare variables
readtms = 0;
//Declare variables
writetms = 0;
//Call method
Sem_init(&mtx, 0, 1);
//Call method
Sem_init(&rdrcnt, 0, N);
}
//Define main
int main(int argc, char* argv[])
{
//Declare variable
int li;
//Declare thread variable
pthread_t lTd;
//Call method
init();
//Loop
for (li = 0; li < Pple; li++)
{
//If condition satisfies
if (li%2 == 0)
//Call method
Pthread_create(&lTd, NULL, reader, NULL);
//If condition does not satisfies
else
//Call method
Pthread_create(&lTd, NULL, writer, NULL);
}
//Call method
Pthread_exit(NULL);
//Exit
exit(0);
}
Explanation:
- The reader method decrements the reader count and semaphore initially.
- The reading operation is performed after that.
- The reader count and semaphore values are incremented after the operation.
- The writer method decrements the semaphore variable initially.
- The write operation is then performed.
- If count reaches limit of writers, then display count.
- The semaphore values are incremented after the operation.
read/write: 142746/100000
Want to see more full solutions like this?
Chapter 12 Solutions
Computer Systems: A Programmer's Perspective (3rd Edition)
- This paper deals with Extended Huffman Codes. For instance, for a source emitting two symbols A and B, the second order extension involves coding messages AA, AB, BA and BB (2^2 in number). The third order extension involves messages such as AAA, AAB, etc. (2^3 in number). The probabilities of such strings are computed by multiplying the individual probabilities. Calculate third, fourth and fifth order extensions of a source message. 1. Choose an alphabet a set of at least six (6) symbols with assigned probabilities. It can be assigned as [ A=0.3; B= 0.7; C=0.1;D=0.2;E=0.5;F=0.15]. Compute the third, fourth and fifth order extension probabilities. Using the built-in algorithm, derive the Huffman Code for each extension. Compute the following quantities: (i) Average length of the codeword; (ii) The code efficiency; (iii) The Compression Ratio. Note: I am assuming but needs to be verified that if we need are using 6 symbols A to F. 6^3=216, the 6 to the third power comes…arrow_forwardWhen trying to find a solution, you should give serious attention to employing both the selective-repeat technique and the Go-Back-N strategy if the sequence number space is wide enough to incorporate k bits of information. If the sequence number space is not big enough, you should not even bother trying to find a solution. In its whole, what is the maximum size that the sender window that we are allowed to use is authorised to be allowed to be?arrow_forwardLet n be the product of two large primes. Alice wants to send a message m to Bob, where gcd(m, n) = 1. Alice and Bob choose integers a and b that are relatively prime to Φ(n). Alice computes c ≡ ma (mod n) and sends c to Bob. Bob computes d ≡ cb (mod n) and sends d back to Alice. Since Alice knows a, she finds a1 such that aa1 ≡ 1 (mod Φ(n)). Then she computes e ≡ da1 (mod n) and sends e to Bob. Explain what Bob must do to obtain m and show that this works.arrow_forward
- Let us define a sequence parity function as a function that takes in a sequence of binary inputs and returns a sequence indicating the number of 1’s in the input so far; specifically, if at time t the 1’s in the input so far is even it returns 1, and 0 if it is odd. For example, given input sequence [0, 1, 0, 1, 1, 0], the parity sequence is [0, 0, 0, 1, 0, 0]. Implement the minimal vanilla recurrent neural network to learn the parity function. Explain your rationale using a state transition diagram and parameters of the network.arrow_forwardSuppose that Turing machine M computes the unary number-theoretic function f defined by f(n) = n2 + 6n + 3 and suppose, further, that M is started scanning the leftmost 1 in an unbroken string of six 1s on an otherwise blank tape. Then M will halt scanning the leftmost 1 in an unbroken string of how many 1s?arrow_forwardSuppose you’re consulting for a bank that’s concerned about fraud detection, and they come to youwith the following problem. They have a collection of n bank cards that they’ve confiscated, suspectingthem of being used in fraud. Each bank card is a small plastic object, containing a magnetic stripe withsome encrypted data, and it corresponds to a unique account in the bank. Each account can have manybank cards corresponding to it, and we’ll say that two bank cards are equivalent if they correspond to thesame account.It’s very difficult to read the account number off a bank card directly, but the bank has a high-tech “equivalence tester” that takes two bank cards and, after performing some computations, determines whether theyare equivalent.Their question is the following: among the collection of n cards, is there a set of more than n/2 of themthat are all equivalent to one another? Assume that the only feasible operations you can do with the cardsare to pick two of them and plug them…arrow_forward
- Discuss the trade-off between fairness and throughput of operations in the readers-writers problem. Propose a method for solving the readers-writers problem without causing starvation.arrow_forwardLet G be a pseudorandom generator with length function l(n) = n + 1. Define a new function H by the rule H(rx) = r||G(x) , where |r| = 2. Thus H just passes the first 2 bits of its input through untouched and applies G to the last n – 2 bits. Prove with a full reduction that H is a PRG. (You can ignore the behavior of H on inputs of length less than 2.arrow_forwardConstruct a npda that accept the following language, L = { anbm : n ≤≤m ≤≤3n}. Algorithm for the npda: for each a read, we put ______________ tokens to be consumed by b. When read b, change state and for each b, pop one token. Group of answer choices A)1 or 2 or 3 B)1 or 3 C)3arrow_forward
- Algorithm to An iterative solution to Towers of Hanoi.in: triplet S = s0, s1, s2 representing the current game stateout: triplet R = r0, r1, r2 representing the new game statelocal: pole indices a, b, z ∈ {0, 1, 2}; disc numbers g, h ∈ [2, n]; last(Q) = Q|Q|−1, if1 ≤ |Q|, otherwise, last(Q) = +∞arrow_forwardRestrictivenessThe problem with the preceding composition is the need for a machine to act the same way whether a LOW input is preceded by a HIGH input, a LOW input, or no input. The machine dog does not meet this criterion. If the first message to dog is stop count, dog emits a 0. If a HIGH input precedes stop count, dog may emit either a 0 or a 1. State Machine ModelAssume a state machine of the type discussed in Section 9.2. Let the machine have two levels, LOW and HIGH. Now consider such a system with the following properties: For every input ik and state σj, there is an element cm ∈ C* such that T* (cm, σj) = σn, where σn ≠ σj. There exists an equivalence relation ≡ such that: If the system is in state σi and a sequence of HIGH inputs causes a transition from σi to σj, then σi ≡ σj. If σi ≡ σj and a sequence of LOW inputs i1, ..., in causes a system in state σi to transition to state , then there is a state such that and the inputs i1, ..., in cause a system in state σj to…arrow_forwardCreate an impulse word problem and solve it.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
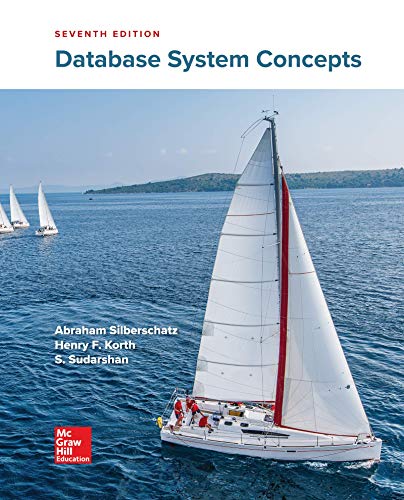
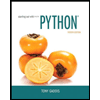
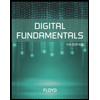
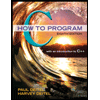
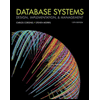
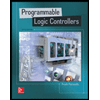