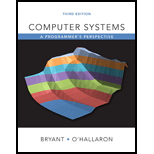
Concept explainers
Explanation of Solution
Implementation of a concurrent prethreaded version of the TINY web server:
Modified code for “sbuf.h” file:
The modified code for “sbuf.h” from section 12.5.4 in book is given below:
#ifndef SBUF_HEADER
#define SBUF_HEADER
#include "csapp.h"
typedef struct
{
int *buf; /* Buffer array */
int n; /* Maximum number of slots */
int front; /* buf[(front+1)%n] is first item */
int rear; /* buf[rear%n] is last item */
sem_t mutex; /* Protects accesses to buf */
sem_t slots; /* Counts available slots */
sem_t items; /* Counts available items */
} sbuf_t;
//Function declaration
void sbuf_init(sbuf_t *sp, int n);
void sbuf_deinit(sbuf_t *sp);
void sbuf_insert(sbuf_t *sp, int item);
int sbuf_remove(sbuf_t *sp);
//Function declaration for sbuf_empty
int sbuf_empty(sbuf_t *sp);
//Function declaration for sbuf_full
int sbuf_full(sbuf_t *sp);
#endif
Modified code for “sbuf.c” file:
The modified code for “sbuf.c” from section 12.5.4 in book is given below:
#include "csapp.h"
#include "sbuf.h"
/* Create an empty, bounded, shared FIFO buffer with n slots */
void sbuf_init(sbuf_t *sp, int n)
{
sp->buf = Calloc(n, sizeof(int));
sp->n = n; /* Buffer holds max of n items */
sp->front = sp->rear = 0; /* Empty buffer if front == rear */
Sem_init(&sp->mutex, 0, 1); /* Binary semaphore for locking */
Sem_init(&sp->slots, 0, n); /* Initially, buf has n empty slots */
Sem_init(&sp->items, 0, 0); /* Initially, buf has zero data items */
}
/* Clean up buffer sp */
void sbuf_deinit(sbuf_t *sp)
{
Free(sp->buf);
}
/* Insert item onto the rear of shared buffer sp */
void sbuf_insert(sbuf_t *sp, int item)
{
P(&sp->slots); /* Wait for available slot */
P(&sp->mutex); /* Lock the buffer */
sp->buf[(++sp->rear)%(sp->n)] = item; /* Insert the item */
V(&sp->mutex); /* Unlock the buffer */
V(&sp->items); /* Announce available item */
}
/* Remove and return the first item from buffer sp */
int sbuf_remove(sbuf_t *sp)
{
int item;
P(&sp->items); /* Wait for available item */
P(&sp->mutex); /* Lock the buffer */
item = sp->buf[(++sp->front)%(sp->n)]; /* Remove the item */
V(&sp->mutex); /* Unlock the buffer */
V(&sp->slots); /* Announce available slot */
return item;
}
//Function definition for empty buffer
int sbuf_empty(sbuf_t *sp)
{
//Declare variable
int ne;
//For lock the buffer
P(&sp->mutex);
ne = sp->front == sp->rear;
//For lock the buffer
V(&sp->mutex);
return ne;
}
//Function definition for full buffer
int sbuf_full(sbuf_t *sp)
{
//Declare variable
int fn;
//For lock the buffer
P(&sp->mutex);
fn = (sp->rear - sp->front) == sp->n;
//For lock the buffer
V(&sp->mutex);
return fn;
}
For code “tiny.c” and “tiny.h”:
Same code as section 11.6 in book.
sample.html:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<title>Home</title>
</head>
<body>
Tiny server Example
</body>
</html>
main.c:
#include <stdio.h>
#include "csapp.h"
#include "tiny.h"
#include "sbuf...

Want to see the full answer?
Check out a sample textbook solution
Chapter 12 Solutions
Computer Systems: A Programmer's Perspective (3rd Edition)
- Try pthreads.cpp. Modify it so that they run 3 threads (instead of two) and each thread runs a different function, displaying a different message. Copy-and-paste the source code and the outputs in your report. /* pthreads_demo.cpp A very simple example demonstrating the usage of pthreads. Compile: g++ -o pthreads_demo pthreads_demo.cpp -lpthread Execute: ./pthreads_demo */ #include <pthread.h> #include <stdio.h> using namespace std; //The thread void * thread_func (void *data) { char *tname = (char *) data; printf("My thread identifier is %s\n", tname); pthread_exit (0); } int main () { pthread_t id1, id2; //thread identifiers pthread_attr_t attr1, attr2; //set of thread attributes char *tnames[2] = { "Thread 1", "Thread 2" }; //names of threads //get the default attributes pthread_attr_init (&attr1); pthread_attr_init (&attr2); //create the threads pthread_create (&id1, &attr1, thread_func,…arrow_forwardHere, a solution that uses a single thread is preferable than one that uses several threads.arrow_forwardImplement a solution to the critical section problem with threads using semaphores. you must add a third counting thread which counts by 1 each time it enters its critical section to 3,000,000. Each counts to 3,000,000 for a total of 9,000,000.arrow_forward
- Is it possible, in your opinion, for a single threaded process to get deadlocked without affecting any other threads? If you could elaborate on your answer in the following sentence, that would be great.arrow_forwardWrite the code to implements a simple socket server which first accepts an incoming connection and then hands it off to a background thread for reads and writes, hence freeing the main thread to accept the other incoming connections. in Python programming languagearrow_forwardhow can I write a client program that send 10 one after the other by a thread and by user. and the server recieves the 10 messages and write a different 10 messages.arrow_forward
- Do we really need to have all VM threads use the same method space?arrow_forwardWrite a Java program to create five threads with different priorities. Send two threads of the highest priority to sleep state. Check the aliveness of the threads and mark which thread is long lasting .arrow_forwardWrite a MultiThreaded ServerSocket Program in C# that can handle multiple clients at the same time. a C# Server Socket use TcpListener Class and listen to PORT 9393. When the C# Server Socket gets a request from Client side, the Server passes the instance of the client request to a separate class handleClient. For each Client request, there is a new thread instant is created in C# Server for separate communication with Client.arrow_forward
- Write a program that has a counter as a global variable. Spawn 10 threads in theprogram, and let each thread increment the counter 1000 times in a loop. Print the finalvalue of the counter after all the threads finish—the expected value of the counter is10000. Run this program first without using locking across threads and observe theincorrect updating of the counter due to race conditions (the final value will be slightly lessthan 10000). Next, use locks when accessing the shared counter and verify that the counteris now updated correctlyarrow_forwardHow does this method cause memory leaks? How do I make it multi-thread safe? I have this JUnit test method that checks if my method is working. What it does is to. Inherit @Before and @After methods from BaseTest Since for every class, a session is created before, use it for ServiceTest.testUpdate() when we have to use session.save. ----------------------------------------------------------------------------------------------------------------------- public class BaseTest{ @Autowired protected SessionFactory sessionFactory; protected Session session; @Before public void createSession() { session = sessionFactory.openSession(); } @After public void closeSession(){ if (session != null) { session.flush(); session.close(); } } } public class ServiceTest extends BaseTest{ @Autowired private FoodService foodService; @Test public void testUpdate() { Food food= new Food("Japanese", "Ramen"); session.save(student); int id = (int) session.getIdentifier(student); session.flush();…arrow_forwardIs it even feasible to have a deadlock if there is just one process using one thread? When responding, please include more information.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
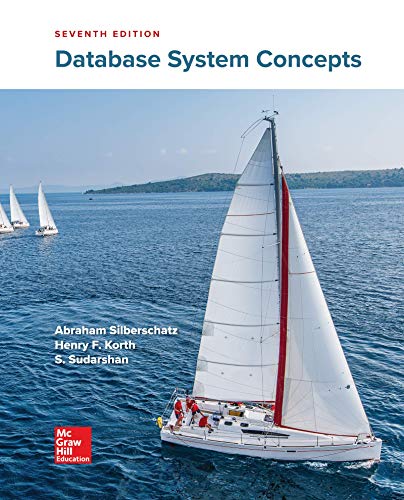
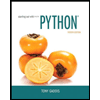
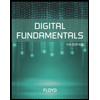
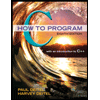
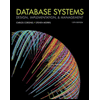
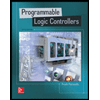