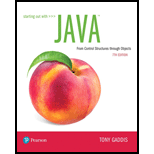
Explanation of Solution
Event handler:
- The event handler is nothing but an object that responds to the events.
- A particular method in the event handler is called if an event source is connected to the event handler. The object of the event is passed as the argument to that method.
- This process is known as event firing.
- The event handler class should implement the “EventHandler” interface.
- This interface is in the package “javafx.event”.
Step 1: Register the instance of the class with “myButton” control. It is done with the help of the statement “setOnAction (new ButtonClickHandler ())”.
Statement to register an instance of the class with “myButton” control:
//Register the event handler
myButton.setOnAction(new ButtonClickHandler ());
Example program:
The statement to register the instance of the class with “myButton” control is highlighted.
//Import required packages
import javafx.application.Application;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.geometry.Pos;
import javafx.geometry.Insets;
import javafx.stage.Stage;
import javafx.scene.Scene;
import javafx.scene.layout.VBox;
import javafx.scene.control.Label;
import javafx.scene.control.Button;
//Declare the main class
public class Example extends Application
{
//Create a label
private Label outputLabel;
//Declare the main method
public static void main(String[] args)
{
// Launch the application...

Want to see the full answer?
Check out a sample textbook solution
Chapter 12 Solutions
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
- The following JavaFx code is called as event ________ Model Button btOK = new Button("OK"); OKHandlerClass handler = new OKHandlerClass(); btOK.setOnAction(handler); Window Display Delegation Dialogarrow_forwardThis property allows one to position a visible control within a window or panel along the top, bottom, sides,so that if the window or panel shrink/grow, so too does the control. PositionDockFloatScrollchoose the followingarrow_forwardWhat's the difference between event bubbling and capturing an event?arrow_forward
- This is the process of connecting an event listener object with a GUI component.a. Registering the event listenerb. Firing the event listenerc. Decorating the event listenerd. Framing the event listenerarrow_forwardAnalyze the following JavaFX program and complete the missing code based on the comments. The Program should show window like this. public class EmployeeInterface extends Application{ @Override public void start(Stage stage) throws Exception { 7- // create the label and TextField for the Major and add them in the first row in the grid pane 8- // Put the commands in the buttom of the BorderPane 9- // Put the GridPane which contain student information in the center of BorderPanearrow_forwardIf you inadvertently create a Click() method for a control that should not generate a click event, you can successfully eliminate the method by ______________. a. deleting the method code from the Form1.cs file b. eliminating the method from the Events list in the Properties window c. adding the method to the Discard window d. making the method a comment by placing two forward slashes at the start of each linearrow_forward
- Similar to how it is the attribute of other controls, a control's attribute decides whether it is displayed on the form during runtime. Similar to this control quality, others also have an impact.arrow_forwardIs this true or false? The Text attribute of a Label control is initially set to the same value as the Label control's name, which makes sense.arrow_forwardHow do we close the window by adding a condition if escape key is entered then close the window. i am trying to do that for a splashkit program in visual studio codearrow_forward
- You created a GUI in Scene Builder and saved it to a file named Testing.fxml. The GUI has a Button component with the fx:id myButton, and a Label component with the fx:id myLabel. You have already written the main application class. Write the code for the controller class. The controller class should have an event listener for the Button component that displays the string “Testing 1, 2, 3” in the Label component.arrow_forwardWhat is the type of the object of the evt function parameter in the following JavaScript code. window.addEventListener("keypress", eventHandler, false);function eventHandler(evt) { // Appropriate code} one of the following choices DateTime object EventListener object Keyboard object MouseEvent objectarrow_forwardIn Visual Basic, when you drag a field object to an existing control in the interface, Visual Basic replaces the current control with the newly created control. True or false? a. True b. Falsearrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,New Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage Learning
- Programming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
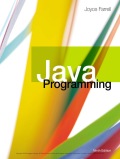
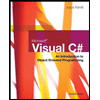
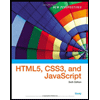
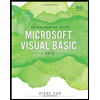