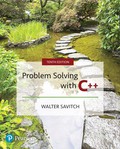
Problem Solving with C++ (10th Edition)
10th Edition
ISBN: 9780134521176
Author: SAVITCH
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 13, Problem 10PP
Program Plan Intro
Creation of program to display result of computation of Reverse polish Notation
Program Plan:
- Define a file “Stack.h” to declare operation and methods in stack.
- Define a structure “StckNde” that contains data and link to next node as members.
- Define a class “Stack” to define methods and prototypes.
- Define function prototypes for methods in program.
- Define a file “Stack.cpp” to define operations and methods in stack.
- Define a constructor “Stack()” to create an empty stack.
- Define a destructor “~Stack()” to destroy a stack.
- Define a method “empty()” to check whether stack is empty.
- Define a method “pop()” to remove top element of stack.
- Define a method “datatyeCon()” to display error if stack is empty.
- Define a method “operator=()” to define operation for “=” operator.
- Define a method “operator==()” to define operation for “==” operator.
- Define a file “Main.cpp” to call methods in stack and perform all computations.
- Declare variables required for the program.
- Get expression from user.
- Check for operators and operands.
- Define a switch case to perform different computations in expression.
- Display result of operation.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
A SpecialStackTM is a stack modified to support the following two operations: PUSHCLEAR(v) successively pops consecutive items from the top of the stack that are less than u, then pushes v onto the stack. POP() deletes the item at the top of the stack. Assume these operations are implemented using a singly-linked list. PUSHCLEAR(v) iter- ates through the linked list to pop the applicable items and then adds v to the beginning. POP() deletes the first item. (a) Describe the ordering of values on the stack. (b) Explain how a single call to PUSHCLEAR(V) could take (n) time. (c) If we assume that every operation takes linear time, we get a naive bound of O(n²) on the total runtime. But this doesn't consider the fact that these expensive operations happen infrequently so let's analyze it more closely. Show that any sequence of n operations takes O(n) time. -
Given a stack, switch_pairs function takes a stack as a parameter and thatswitches successive pairs of numbers starting at the bottom of the stack.For example, if the stack initially stores these values:bottom [3, 8, 17, 9, 1, 10] topYour function should switch the first pair (3, 8),the second pair (17, 9), ...:bottom [8, 3, 9, 17, 10, 1] topif there are an odd number of values in the stack, the value at the top of thestack is not moved: For example:bottom [3, 8, 17, 9, 1] topIt would again switch pairs of values, but the value at thetop of the stack (1)would not be movedbottom [8, 3, 9, 17, 1] topNote: There are 2 solutions:first_switch_pairs: it uses a single stack as auxiliary storagesecond_switch_pairs: it uses a single queue as auxiliary storage""".
Write a program for Stack (Array-based or linked list-based) in Python. Test the scenario below with the implementation and with the reasoning of the answer. Make comments with a short description of what is implemented. Include source codes and screen-captured outputs.
Stack:
A letter means doing a push operation and an asterisk means doing a pop operation in the below sequence.
Give the sequence of letters that are returned by the pop operations when this sequence of operations is performed on an initially empty stack.
A*BCE**F*GH***I*
Chapter 13 Solutions
Problem Solving with C++ (10th Edition)
Ch. 13.1 - Suppose your program contains the following type...Ch. 13.1 - Suppose that your program contains the type...Ch. 13.1 - Prob. 3STECh. 13.1 - Prob. 4STECh. 13.1 - Prob. 5STECh. 13.1 - Prob. 6STECh. 13.1 - Prob. 7STECh. 13.1 - Suppose your program contains type definitions and...Ch. 13.1 - Prob. 9STECh. 13.2 - Prob. 10STE
Ch. 13.2 - Prob. 11STECh. 13.2 - Prob. 12STECh. 13.2 - Prob. 13STECh. 13 - The following program creates a linked list with...Ch. 13 - Re-do Practice Program 1, but instead of a struct,...Ch. 13 - Write a void function that takes a linked list of...Ch. 13 - Write a function called mergeLists that takes two...Ch. 13 - In this project you will redo Programming Project...Ch. 13 - A harder version of Programming Project 4 would be...Ch. 13 - Prob. 6PPCh. 13 - Prob. 8PPCh. 13 - Prob. 9PPCh. 13 - Prob. 10PP
Knowledge Booster
Similar questions
- Write a Java public static general function which doesn't belong to the Stack class, isGreaterThan that determines if one stack is greater than another stack. If the summation of every other item(starting at the top) from one stack is greater than the summation of every other item(starting at the top) from another, return true, otherwise, return false. After you call this method, both stacks should stay the same as they are called before. Receives: a Stack type argument S1 and another Stack type argument S2 Postcondition: If the summation of every other item (starting at the top) from S1 is greater than the summation of every other item (starting at the top) from S2, return true, otherwise, return false. Both S1 and S2 should stay the same as they are called before. public static boolean isGreaterThan(Stack S1, Stack S2)arrow_forwardWrite a structure to represent a Node in a singly linked-list-based stack similar to the onediscussed in class. Your stack will store integer values. Your program will read a series of integers(until the user enters a non-integer) and store them in a stack, then print them in the reverse order;You will write three functions to assist with this task: push, printStack, and deleteStack.The function push takes a number and a Node and adds the number to the head (top) of thestack by creating a new Node (using malloc) and returning a pointer to this Node. This new nodeis now the top of your stack.The function printStack will need to iterate through your stack and print each one (5 spacesper number, left-aligned), with a new line following the nal number. You will need a pointer thatyou update to the next Node each time you print one. Since you add new items to the top of thestack, the newest elements will be printed rst, giving the reverse-order behavior requested.The function deleteStack…arrow_forwardCode in C One of the applications of a stack is to backtrack - that is, to retrace its steps. As an example, imagine we want to read a list of items, and each time we read a negative number we must backtrack and print the five numbers that come before the negative number and then discard the negative number. Use a stack to solve this problem. Use a stack and push them into the stack (without printing them) until a negative number is read. At this time, stop reading and pop five items from the stack and print them. If there are fewer than five items in the stack, print an error message and stop the program. After printing the five items, resume reading data and placing them in the stack. When the end of the file is detected, print the message and the items remaining in the stack. Sample Output 1 2 3 4 5 6 7 -1 7 6 5 4 3 9 6 4 3 -2 3 4 6 9 2 -3 Number of items left is less than five! Program is terminating! Project name : Backtrack Filenames: backtrack.h, backtrack.c,…arrow_forward
- There is a bag-like data structure, supporting two operations: 1 x1 x: Throw an element xx into the bag. 22: Take out an element from the bag. Given a sequence of operations with return values, you’re going to guess the data structure. It is a stack (Last-In, First-Out), a queue (First-In, First-Out), a priority-queue (Always take out larger elements first) or something else that you can hardly imagine! Input There are several test cases. Each test case begins with a line containing a single integer nn (1≤n≤10001≤n≤1000). Each of the next nn lines is either a type-1 command, or an integer 22 followed by an integer xx. This means that executing the type-2 command returned the element xx. The value of xx is always a positive integer not larger than 100100. The input is terminated by end-of-file (EOF). The size of input file does not exceed 1MB. Output For each test case, output one of the following: stackIt’s definitely a stack. queueIt’s definitely a queue. priority queueIt’s definitely…arrow_forwardConvert the following infix notation to its postfix notation. You must show the stack contents in your simulation. (5+ x!=d)==|{(3!=e*6) && (1==5/5} != 4 -51 Priority: */,% (high) t, = I= && (low)arrow_forwardSolve the code much needed. Given a stack, a function is_consecutive takes a stack as a parameter and thatreturns whether or not the stack contains a sequence of consecutive integersstarting from the bottom of the stack (returning true if it does, returningfalse if it does not). For example:bottom [3, 4, 5, 6, 7] topThen the call of is_consecutive(s) should return true.bottom [3, 4, 6, 7] topThen the call of is_consecutive(s) should return false.bottom [3, 2, 1] topThe function should return false due to reverse order. Note: There are 2 solutions:first_is_consecutive: it uses a single stack as auxiliary storagesecond_is_consecutive: it uses a single queue as auxiliary storage"""import collections def first_is_consecutive(stack): storage_stack = [] for i in range(len(stack)): first_value = stack.pop() if len(stack) == 0: # Case odd number of values in stack return True second_value = stack.pop() if first_value - second_value != 1: # Not…arrow_forward
- A string may use more than one type of delimiter to bracket information into blocks. For example, a string may use braces { }, parentheses ( ), and brackets [ ] as delimiters. A string is properly delimited if each right delimiter is matched with a preceding left delimiter of the same type in such a way that either the resulting blocks of information are disjoint, or one of them is completely nested within the other. Write a program that uses a single stack to check whether a string containing braces, parentheses, and brackets is properly delimited.Use input file string.txt as input to test your program.Each line in the input file is a string. If the string is properly delimited, display a message stating so. If it is not properly delimited, display a message stating so.For example, if the input file contains the following 3 lines of strings:if (a[i] > b[i])while (c[index] < 0) { a++; b--; c[index++];}for ({int i = 0; num[i }];would display the following output: Processing…arrow_forwardA CapnStackSparrow is an extended stack that supports four main operations: the standard Stack operations push(x) and pop() and the following non-standard operations:• max(): returns the maximum value stored on the Stack.• ksum(k): returns the sum of the top k elements on the Stack.The zip file gives an implementation SlowSparrow that implements these operations so that push(x) and pop() each run in ?(1) time, but max() and ksum(k) run in ?(?) time. For this question, you should complete the implementation of FastSparrow that implements all four operations in ?(1) (amortized) time per operation. As part of your implementation, you may use any of the classes in the Java Collections Framework and you may use any of the source code provided with the Java version of the textbook. Don't forget to also implement the size() and iterator() methods. Think carefully about your solution before you start coding. Here are two hints:1. don't use any kind of SortedSet or SortedMap, these all require…arrow_forwardWrite a program to enter a natural number n and find all sphenic numbers from 1 to n using Linked List ADT with necessary functions and Linked List principle. Note: The size of stack is a constant (size = 3) and to use Stack ADT, there should be three steps:Step 1. Find and push every prime numbers that constitute a specific sphenic number in the stack.Step 2. Pop out all numbers found in the stack and calculate the product of these 3 prime numbers.Step 3. Display the result in the stack.arrow_forward
- The PostfixEvaluator class uses the java.util.Stack class to create thestack attribute. The java.util.Stack class is one of two stack implementationsprovided by the Java Collections API. We revisit the other implementation, theDeque interface, in Chapter 13.The evaluate method performs the evaluation algorithm described earlier, supported by the isOperator and evalSingleOp methods. Note that in the evaluatemethod, only operands are pushed onto the stack. Operators are used as they areencountered and are never put on the stack. This is consistent with the evaluation algorithm we discussed. An operand is put on the stack as an Integer object, insteadof as an int primitive value, because the stack collection is designed to store objects.When an operator is encountered, the two most recent operands are poppedoff the stack. As mentioned above, the first operand popped is actually the secondoperand in the expression and that the second operand popped is the first operandin the expression.…arrow_forwardA stack is very useful in situations when data have to be stored and then retrieved in the —- order. A) same B) reverse C) ascending D) descendingarrow_forwardWrite a program for Stack (Array-based or linked list-based) in Python. Test the scenario below with the implementation and with the reasoning of the answer. Make comments with a short description of what is implemented. Include source codes and screen-captured outputs. Stack: Given an empty stack in which the values A, B, C, D, E, F are pushed on the stack in that order but can be popped at any time, give a sequence of push and pop operations which results in pop()ed order of BADECFarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
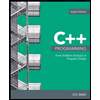
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning