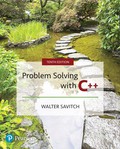
Concept explainers
Linked list:
- Linked list denotes a linear data structure.
- The elements are not stored at contiguous locations; the elements are linked using pointers.
- It stores linear data of similar types not like arrays.
- The size of linked list can be changed based on requirement.
- It is represented by a pointer to first linked list node.
- The first node denotes a head.
- If linked list is empty, value of head is NULL.
- The node in a list has two parts, data and pointer to next node.
Given code:
//Define a structure
struct Box
{
//Declare variable
string name;
//Declare variable
int number;
//Define pointer to next
Box *next;
};
//Create an instance
BoxPtr head;
//Assign value
head = new Box;
//Assign value
head->name = "Sally";
//Assign value
head->number = 18;
//Assign value
head->next = NULL;
//Display value
cout << (*head).name << endl;
//Display value
cout << head->name << endl;
//Display value
cout << (*head).number << endl;
//Display value
cout << head->number << endl;
Explanation:
- The “Box” defines a structure with “name” and “number” as members.
- It contains a pointer to next element of list.
- The “BoxPtr head” creates an instance of structure.
- A new “Box” element is created.
- The values of “name” and “number” are been set.
- The values of “name” and “number” are been displayed.
- The member variable next to the node is been set to NULL.
Complete program:
//Include libraries
#include<iostream>
#include<string>
//Use namespace
using namespace std;
//Define a structure
struct Box
{
//Declare variable
string name;
//Declare variable
int number;
//Define pointer to next
Box *next;
};
//Define instance
typedef Box* BoxPtr;
//Define main method
void main()
{
//Create an instance
BoxPtr head;
//Assign value
head = new Box;
//Assign value
head->name = "Sally";
//Assign value
head->number = 18;
//Display value
cout << (*head).name << endl;
//Display value
cout << head->name << endl;
//Display value
cout << (*head).number << endl;
//Display value
cout << head->number << endl;
//Delete
delete head;
//Display message
cout<<"List head is been destroyed"<<endl;
//Pause console window
system("pause");
}
Explanation:
- The “Box” defines a structure with “name” and “number” as members.
- It contains a pointer to next element of list.
- Define a main method
- The “BoxPtr head” creates an instance of structure.
- A new “Box” element is created.
- The values of “name” and “number” are been set.
- The values of “name” and “number” are been displayed.
- The member variable next to the node is been set to NULL.
- The linked list is been destroyed using “delete” statement.

Want to see the full answer?
Check out a sample textbook solution
Chapter 13 Solutions
Problem Solving with C++ (10th Edition)
- Tell whether or not the following statement is accurate:Only pointers and references are permissible for dynamically bound virtual functions.arrow_forwardDifferences between void and NULL pointers are as follows: Provide relevant instances to back up your claim.arrow_forwardInspect the code and in your own interpretation, describe the reason call to function ChangeIt_1(n) will not change x in main()? Inspect the code and in your own interpretation, describe the reason parameter n in ChangeIt_2(int *n) is a pointer, and statement in the function is *n = 6 ? Write a function called void swap with two parameters, which will receive the address of x and y as pointer. The function should swap the value of x and y only if x is larger than y. Print the value of x and y after the function call. Write a function called double getAverage(int *array, int SIZE), where it accept the array as pointer, and return the average of the array. Print the returned value from this function. Always use *array rather than subscript to access the array value.arrow_forward
- Indicate whether the following is true or false:Only pointers and references are permissible for use with dynamically bound virtual functions.arrow_forwardSuppose you are writing a C++ function that has three alternativeapproaches for accomplishing its requirements. Write a skeletal versionof this function so that if the first alternative raises any exception, thesecond is tried, and if the second alternative raises any exception, thethird is executed. Write the code as if the three methods were proceduresnamed alt1, alt2, and alt3.arrow_forwardWhat's the distinction between void and NULL pointers? Give appropriate examples to back up your answer.arrow_forward
- Need help in this question Consider the following skeletal C program:void fun1(void); /* prototype */void fun2(void); /* prototype */void fun3(void); /* prototype */void main() {int a, b, c;. . .}void fun1(void) {int b, c, d;. . .}void fun2(void) {int c, d, e;. . .}void fun3(void) {int d, e, f;. . .}Given the following calling sequences and assuming that dynamic scopingis used, what variables are visible during execution of the last functioncalled? Include with each visible variable the name of the function inwhich it was defined.a. main calls fun1; fun1 calls fun2; fun2 calls fun3.b. main calls fun1; fun1 calls fun3.c. main calls fun2; fun2 calls fun3; fun3 calls funarrow_forwardThe definition of a pointer variable is exactly the same as its name. What is the function of it? What does it imply exactly to have something called a "dynamic array"? What kind of connection exists between pointers and dynamic arrays?arrow_forwardThe distinction between void and NULL pointers is as follows: Make use of appropriate examples to support your response.arrow_forward
- Is it better to have virtual functions that are statically or dynamically bound?arrow_forwardWhat's the distinction between void and NULL pointers? Give appropriate examples to back up your answer. Give the distinctions in TABULAR form.arrow_forwardWhat is the method for storing local declarations in computer memory? Is there any reason to avoid using local declarations if the same objective can be achieved without them? Why use value parameters if reference parameters can be used in any function? What role do value parameters play in program data processing?arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
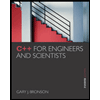