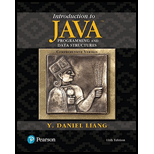
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
11th Edition
ISBN: 9780134670942
Author: Y. Daniel Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 13, Problem 13.12PE
Program Plan Intro
SumArea.Java
Program Plan:
- Include a class name named “Exercise_13_12”.
- Declare the main() method.
- Create an array of four objects two of circle type and two of rectangle type.
- Print total elements in the array.
- Create a function that returns the sum of the areas of all the geometric objects in an array.
- Close the main() method.
- Close the class.
- Include an abstract class “GeometricObject” that implements Comparable.
- Declare all the data types of the variables.
- Construct a default geometric object.
- Construct a geometric object with color and filled value.
- Assign color and fill to the object.
- Set a new color.
- Define an isFilled method returns filled and since filled is Boolean; the get method is named isFilled.
- Set a new filled and getDateCreated method.
- Override toString function to return output.
- Override function to implement the compareTo method.
- Abstract method getMax to return maximum object.
- Define Abstract method getArea.
- Define Abstract method getPerimeter.
- Close class.
- Include a class “Rectangle” that extends the class GeometricObject class.
- Declare width and height of rectangle.
- Create a Rectangle constructor.
- Assign values to height and width.
- Assign rectangle values to all attributes.
- Create a function to return width.
- Create a function to return radius.
- Create a function to set a new width.
- Create a function to return height.
- Create a function to set a new height.
- Create an override function to return area.
- Create an override function to return Perimeter.
- Overriding function to return String description of this rectangle.
- Close class.
- Include a class “Circle” that extends the class GeometricObject class.
- Declare radius variable.
- Create a Circle constructor.
- Assign values to radius.
- Create a function to set color and set fill in the Circle.
- Create a Function to return radius.
- Create a function to set a new radius.
- Create an override function to return area.
- Create an override function to return perimeter.
- Create an override string description of circle object.
- Close class.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Is there a way to increase the size of an array after its declaration?
Write the following method
What happens when you use either the ref or out keyword with an array parameter and see what happens?
Chapter 13 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Ch. 13.2 - Prob. 13.2.1CPCh. 13.2 - The getArea() and getPerimeter() methods may be...Ch. 13.2 - True or false? a.An abstract class can be used...Ch. 13.3 - Prob. 13.3.1CPCh. 13.3 - Prob. 13.3.2CPCh. 13.3 - Prob. 13.3.3CPCh. 13.3 - What is wrong in the following code? (Note the...Ch. 13.3 - What is wrong in the following code? public class...Ch. 13.4 - Can you create a Calendar object using the...Ch. 13.4 - Prob. 13.4.2CP
Ch. 13.4 - How do you create a Calendar object for the...Ch. 13.4 - For a Calendar object c, how do you get its year,...Ch. 13.5 - Prob. 13.5.1CPCh. 13.5 - Prob. 13.5.2CPCh. 13.5 - Prob. 13.5.3CPCh. 13.5 - Prob. 13.5.4CPCh. 13.6 - Prob. 13.6.1CPCh. 13.6 - Prob. 13.6.2CPCh. 13.6 - Can the following code be compiled? Why? Integer...Ch. 13.6 - Prob. 13.6.4CPCh. 13.6 - What is wrong in the following code? public class...Ch. 13.6 - Prob. 13.6.6CPCh. 13.6 - Listing 13.5 has an error. If you add list.add...Ch. 13.7 - Can a class invoke the super.clone() when...Ch. 13.7 - Prob. 13.7.2CPCh. 13.7 - Show the output of the following code:...Ch. 13.7 - Prob. 13.7.4CPCh. 13.7 - What is wrong in the following code? public class...Ch. 13.7 - Show the output of the following code: public...Ch. 13.8 - Prob. 13.8.1CPCh. 13.8 - Prob. 13.8.2CPCh. 13.8 - Prob. 13.8.3CPCh. 13.9 - Show the output of the following code: Rational r1...Ch. 13.9 - Prob. 13.9.2CPCh. 13.9 - Prob. 13.9.3CPCh. 13.9 - Simplify the code in lines 8285 in Listing 13.13...Ch. 13.9 - Prob. 13.9.5CPCh. 13.9 - The preceding question shows a bug in the toString...Ch. 13.10 - Describe class-design guidelines.Ch. 13 - (Triangle class) Design a new Triangle class that...Ch. 13 - (Shuffle ArrayList) Write the following method...Ch. 13 - (Sort ArrayList) Write the following method that...Ch. 13 - (Display calendars) Rewrite the PrintCalendar...Ch. 13 - (Enable GeometricObject comparable) Modify the...Ch. 13 - Prob. 13.6PECh. 13 - (The Colorable interface) Design an interface...Ch. 13 - (Revise the MyStack class) Rewrite the MyStack...Ch. 13 - Prob. 13.9PECh. 13 - Prob. 13.10PECh. 13 - (The Octagon class) Write a class named Octagon...Ch. 13 - Prob. 13.12PECh. 13 - Prob. 13.13PECh. 13 - (Demonstrate the benefits of encapsulation)...Ch. 13 - Prob. 13.15PECh. 13 - (Math: The Complex class) A complex number is a...Ch. 13 - (Use the Rational class) Write a program that...Ch. 13 - (Convert decimals to fractious) Write a program...Ch. 13 - (Algebra: solve quadratic equations) Rewrite...Ch. 13 - (Algebra: vertex form equations) The equation of a...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- What transpires when a parameter for an array is sent in with either the ref or out keyword, and the results are observed?arrow_forwardJara, need to use array and method carrow_forwardPlease use java only and public int method. Show the steps with the pictures also. In this assignment, you will implement a class calledArrayAndArrayList. This class includes some interesting methods for working with Arrays and ArrayLists. For example, the ArrayAndArrayList class has a “findMax” method which finds and returns the max number in a given array. For a defined array: int[] array = {1, 3, 5, 7, 9}, calling findMax(array) will return 9. There are 4 methods that need to be implemented in the A rrayAndArrayList class: ● howMany(int[] array, int element) - Counts the number of occurrences of the given element in the given array. ● findMax(int[] array) - Finds the max number in the given array. ● maxArray(int[] array) - Keeps track of every occurrence of the max number in the given array. ● swapZero(int[] array) - Puts all of the zeros in the given array, at the end of the given array. Each method has been defined for you, but without the code. See the javadoc for each…arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
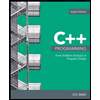
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning