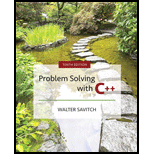
Problem Solving with C++ (10th Edition)
10th Edition
ISBN: 9780134448282
Author: Walter Savitch, Kenrick Mock
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 13.1, Problem 4STE
Program Plan Intro
Linked list:
- Linked list denotes a linear data structure.
- The elements are not stored at contiguous locations; the elements are linked using pointers.
- It stores linear data of similar types not like arrays.
- The size of linked list can be changed based on requirement.
- It is represented by a pointer to first linked list node.
- The first node denotes a head.
- If linked list is empty, value of head is NULL.
- The node in a list has two parts, data and pointer to next node.
Given code:
//Define a structure
struct ListNode
{
//Declare variable
string item;
//Declare variable
int count;
//Define next node
ListNode *link;
};
//Create instance
ListNode *head = new ListNode;
Explanation:
- The “ListNode” defines a structure with “item” and “count” as members.
- It has a pointer “link” to next element of list.
- The “ListNode *head = new ListNode” creates an instance of structure and a new element is created.
Complete program:
//Include libraries
#include<iostream>
#include<string>
//Use namespace
using namespace std;
//Define a structure
struct ListNode
{
//Declare variable
string item;
//Declare variable
int count;
//Define next node
ListNode *link;
};
//Create instance
ListNode *head = new ListNode;
//Define main method
void main()
{
//Assign value
head->item = "Wilbur's brother Orville";
//Display value
cout << head->item << endl;
//Pause console window
system("pause");
}
Explanation:
- The “ListNode” defines a structure with “item” and “count” as members.
- It contains a pointer “link” to next element of list.
- The “ListNode *head = new ListNode” creates an instance of structure and a new element is created.
- The main method assigns value to “item” to head of linked list.
- The value of head of linked list is been displayed.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
The definition of linked list is given as follows:
struct Node {
ElementType Element ;
struct Node *Next ;
} ;
typedef struct Node *PtrToNode, *List, *Position;
If L is head pointer of a linked list, then the data type of L should be ??
#ifndef H_StackType#define H_StackType#include <iostream>#include <cassert>using namespace std;template <class Type>struct nodeType{ Type info; nodeType<Type> *link;};template <class Type>class linkedStackType: public stackADT<Type>class linkedStackType{public: const linkedStackType<Type>& operator= (const linkedStackType<Type>&); bool isEmptyStack() const; bool isFullStack() const; void initializeStack(); //Function to initialize the stack to an empty state. void push(const Type& newItem); Type top() const; //Function to return the top element of the stack. //Precondition: The stack exists and is not empty. //Postcondition: If the stack is empty, the program // terminates; otherwise, the top element of // the stack is returned. void pop(); linkedStackType(); //Postcondition: stackTop = NULL; linkedStackType(const…
Question:
struct node { char *name; int marks; node *next; }; By using the above declaration for the Linked List, write a program in C++ to display the summary report regarding the pass/fail ratio of the subject Data Structure for the class BSI-3 that have only 15 students. The required Report Summary for the exam will be like wise: The No. of A grades........??? The No. of B grades........??? And so on by applying the grading criteria of COMSATS-University Islamabad. Question:
struct node { char *name; int marks; node *next; }; By using the above declaration for the Linked List, write a program in C++ to display the summary report regarding the pass/fail ratio of the subject Data Structure for the class BSI-3 that have only 15 students. The required Report Summary for the exam will be like wise: The No. of A grades........??? The No. of B grades........??? And so on by applying the grading criteria of COMSATS-University Islamabad.
Chapter 13 Solutions
Problem Solving with C++ (10th Edition)
Ch. 13.1 - Suppose your program contains the following type...Ch. 13.1 - Suppose that your program contains the type...Ch. 13.1 - Prob. 3STECh. 13.1 - Prob. 4STECh. 13.1 - Prob. 5STECh. 13.1 - Prob. 6STECh. 13.1 - Prob. 7STECh. 13.1 - Suppose your program contains type definitions and...Ch. 13.1 - Prob. 9STECh. 13.2 - Prob. 10STE
Ch. 13.2 - Prob. 11STECh. 13.2 - Prob. 12STECh. 13.2 - Prob. 13STECh. 13 - The following program creates a linked list with...Ch. 13 - Re-do Practice Program 1, but instead of a struct,...Ch. 13 - Write a void function that takes a linked list of...Ch. 13 - Write a function called mergeLists that takes two...Ch. 13 - In this project you will redo Programming Project...Ch. 13 - A harder version of Programming Project 4 would be...Ch. 13 - Prob. 6PPCh. 13 - Prob. 8PPCh. 13 - Prob. 9PPCh. 13 - Prob. 10PP
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- The data structure that allows for elements to be added and removed at both ends but not in the middle is called: 1.Double-ended Queue. 2.Priority Queue. 3.Duck. 4.Torque.arrow_forwardProvide a detailed explanation line by line. Explain how this C++ program functions Source Codes #include using namespace std; // Node struct to hold data and pointer to next node struct Node { int data; Node* next; }; // Linked List class class LinkedList { private: Node* head; // pointer to head node public: LinkedList() { head = NULL; // initialize head to NULL } // Insert a new node at the front of the list void insert(int value) { Node* newNode = new Node(); // create a new node newNode->data = value; // set the data of the new node to the input value newNode->next = head; // set the next pointer of the new node to the current head node head = newNode; // set the head pointer to the new node } // Delete a node with a specific value from the list void deleteNode(int value) { Node* current = head; // set a pointer to the current node Node* previous = NULL; // set a pointer…arrow_forwardWhat advantage does a circular linked structure with a dummy header node give the programmer?arrow_forward
- Write a linked list structure declaration to define a record of car partscontaining: part identification (integer) part name (maximum 20 characters) quantity on stock (integer) cost per unit (double) supplier's name (maximum 30 characters) pointer to the next nodearrow_forward1 T OR F Queue is a structure in which elements are added to the front and removed from the rear.arrow_forwardCreate a method that accepts a pointer to a Node structure as an argument and returns a full copy of the data structure put in. There are two pointers to other Nodes in the Node data structure.arrow_forward
- Write C code that implements a soccer team as a linked list. 1. Each node in the linkedlist should be a member of the team and should contain the following information: What position they play whether they are the captain or not Their pay 2. Write a function that adds a new members to this linkedlist at the end of the list.arrow_forwardProvide a detailed explanation line by line. Explain how this C++ program functionsSource Codes #include <iostream> using namespace std; // Node struct to hold data and pointer to next node struct Node { int data; Node* next; }; // Linked List class class LinkedList { private: Node* head; // pointer to head node public: LinkedList() { head = NULL; // initialize head to NULL } // Insert a new node at the front of the list void insert(int value) { Node* newNode = new Node(); // create a new node newNode->data = value; // set the data of the new node to the input value newNode->next = head; // set the next pointer of the new node to the current head node head = newNode; // set the head pointer to the new node } // Delete a node with a specific value from the list void deleteNode(int value) { Node* current = head; // set a pointer to the current node Node* previous =…arrow_forwardPython problem: "Write a Node class for singly linked structure. Define 3 node objects with which to put these data in them. "4", "-7", "34" " Here is the node code: """ File: node.py Node classes for one-way linked structures and two-way linked structures. """ class Node(object): def __init__(self, data, next = None): """Instantiates a Node with default next of None""" self.data = data self.next = next class TwoWayNode(Node): def __init__(self, data, previous = None, next = None): Node.__init__(self, data, next) self.previous = previous # Just an empty link node1 = None # A node containing data and an empty link node2 = Node("A", None) # A node containing data and a link to node2 node3 = Node("B", node2)arrow_forward
- Given the declarations (C++)struct ListNode{ float volume;ListNode* link;};ListNode* headPtr;ListNode* currPtr;assume that headPtr and currPtr point to a linked list of many nodes. Write the statements that remove the second node.arrow_forwardprogram Linked List: modify the following program to make a node containing data values of int, char, and string. #include <iostream> using namespace std; struct node { int data; struct Node *next; }; struct Node* head = nullptr;//or Null or 0; void insert(int new_data) { struct Node* new_node=(struct Node*) new(struct Node); new_mode->data=new_data; new_mode->next=head; head=new_node; } void display() { struct Node* ptr; ptr=head; while(ptr ! = NULL) { cout<<ptr->data<<""; ptr=ptr->next; } } int main() { insert{2}; display{}; return0; }arrow_forwardHi everyone, can somone help me with this Programming Assignment from Data Structures. Thank you.In this assignment, you will use a modified Node structure to create a “Double Ended Doubly Linked List” data structure with extended functionality. The Node structure is defined as follows (we will use integers for data elements): public class Node { public int data; public Node next; public Node previous; } The Double Ended Doubly Linked List (DEDLL) class definition is as follows: public class DEDLL { public int currentSize; public Node head; public Node tail; } This design is a modification of the standard linked list. In the standard that we discussed in class, there is only a head node that represents the first node in the list and each node only contains a reference to the next node in the list until the chain of nodes reach null. In this DEDLL, each node also has a reference to the previous node. The…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
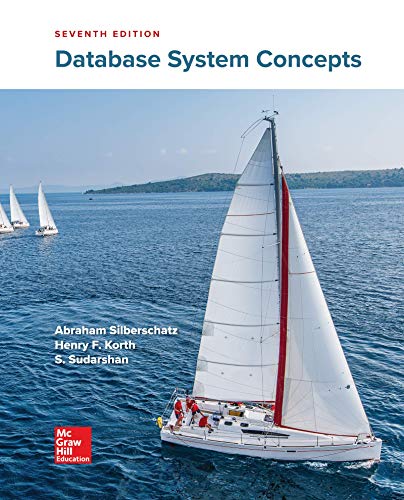
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
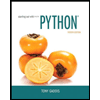
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
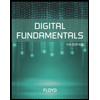
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
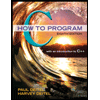
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
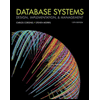
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
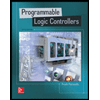
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education