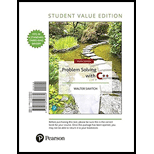
Problem Solving with C++, Student Value Edition Plus MyLab Programming with Pearson eText - Access Card Package (10th Edition)
10th Edition
ISBN: 9780134756424
Author: Walter Savitch
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 13.1, Problem 7STE
Program Plan Intro
Linked list:
- Linked list denotes a linear data structure.
- The elements are not stored at contiguous locations; the elements are linked using pointers.
- It stores linear data of similar types not like arrays.
- The size of linked list can be changed based on requirement.
- It is represented by a pointer to first linked list node.
- The first node denotes a head.
- If linked list is empty, value of head is NULL.
- The node in a list has two parts, data and pointer to next node.
Given code:
//Define a structure
struct Node
{
//Define member
double data;
//Define next node
Node *next;
};
//Define instance of Node
typedef Node* Pointer;
//Create instance
Pointer p1, p2;
Explanation:
- The “Node” defines a structure with “data” as member.
- It has a pointer “next” to next element of list.
- The “Node *Pointer” creates an instance of structure.
- The “Pointer p1, p2” creates instance variables “p1” and “p2” of type “Pointer”.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Please program in C
8.18 LAB: Simple linked list
Given an IntNode struct and the operating functions for a linked list, complete the following functions to extend the functionality of the linked list:
IntNode* IntNode_GetNth(IntNode* firstNode, int n)- Return a pointer to the nth node of the list starting at firstNode.
void IntNode_PrintList(IntNode* firstNode) - Call IntNode_PrintNodeData() to output values of the list starting at firstNode. Do not add extra space characters in between values.
int IntNode_SumList(IntNode* firstNode) - Return the sum of the values of all nodes starting at firstNode.
Note: The code for IntNode_Create() provided here differs from the code shown in the book.
The given main() performs various actions to test IntNode_GetNth(), IntNode_PrintList(), and IntNode_SumList().
main() reads 5 integers from a user:
The number of nodes to be added to a new list
The value of the first node of the list
An increment between the values of two consecutive…
Assume that, you have a Linked List class "LL" with a function named "fun1". You can assume that "value" is the value of the nodes and "Next" is the next pointer of the node. You created an instance of the LL class, named "L1", where "head" is the head node. And you inserted the following numbers: 31 -> 32 -> 43 -> 17 -> 34 -> 57 ->91. What will be the output of the linked list L1 after the "fun1" operation to it?*
31 -> 32 -> 43 -> 17 -> 34 -> 57 ->91
31 -> 32 -> 17 -> 34 -> 57 ->91
31 -> 32 -> 43 -> 17 -> 57 ->91
31 -> 32 -> 43 -> 17 -> 34 -> 57
31 -> 32 -> 43 -> 57 ->91
31 -> 32 -> 43 -> 34 -> 57 ->91
in C++
kth ElementExtend the class linkedListType by adding the following operations:a. Write a function that returns the info of the kth element of the linked list. If no such element exists, terminate the program.b. Write a function that deletes the kth element of the linked list. If no such element exists, terminate the program. Provide the definitions of these functions in the class linkedListType.
please, do not copy from any other sources, give me a fresh new code. Thank you
Chapter 13 Solutions
Problem Solving with C++, Student Value Edition Plus MyLab Programming with Pearson eText - Access Card Package (10th Edition)
Ch. 13.1 - Suppose your program contains the following type...Ch. 13.1 - Suppose that your program contains the type...Ch. 13.1 - Prob. 3STECh. 13.1 - Prob. 4STECh. 13.1 - Prob. 5STECh. 13.1 - Prob. 6STECh. 13.1 - Prob. 7STECh. 13.1 - Suppose your program contains type definitions and...Ch. 13.1 - Prob. 9STECh. 13.2 - Prob. 10STE
Ch. 13.2 - Prob. 11STECh. 13.2 - Prob. 12STECh. 13.2 - Prob. 13STECh. 13 - The following program creates a linked list with...Ch. 13 - Re-do Practice Program 1, but instead of a struct,...Ch. 13 - Write a void function that takes a linked list of...Ch. 13 - Write a function called mergeLists that takes two...Ch. 13 - In this project you will redo Programming Project...Ch. 13 - A harder version of Programming Project 4 would be...Ch. 13 - Prob. 6PPCh. 13 - Prob. 8PPCh. 13 - Prob. 9PPCh. 13 - Prob. 10PP
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Suppose a node of a linked list is defined as follows in your program: typedef struct{ int data; struct Node* link; } Node; Write the definition of a function, printOdd(), which displays all the odd numbers of a linked list. What will be the time complexity of printOdd()? Express the time complexity in terms of Big-O notation.arrow_forwardConsider the below code of circular linked list. Your work is to update the same code, by providing the functionalities with tail pointer only. #include <iostream> using namespace std; class Node{ public: int data; Node *next; }; class List{ public: Node *head; List(){ head = NULL; } void insert(int data){ Node *nn = new Node; nn->data = data; if(head==NULL){ head = nn; nn->next = head; cout<<"Node inserted successfully at head"<<endl; } else{ Node *temp = head; while(temp->next!=head) temp = temp->next; nn->next = head; temp->next=nn; cout<<"Node inserted successfully at end"<<endl; } } void insertInBetween(Node *node, int data){ Node *nn = new Node; nn->data = data; Node *temp = node->next;…arrow_forward(IntelliJ) Write an easier version of a linked list with only a couple of the normal linked list functions and the ability to generate and utilize a list of ints. The data type of the connection to the following node can be just Node, and the data element can be just an int. You will need a reference (Java) or either a reference or a pointer (C++) to the first node, as well as one to the last node. * Create a method or function that accepts an integer, constructs a node with that integer as its data value, and then includes the node to the end of the list. If the new node is the first one, this function will also need to update the reference to the first node. This function will need to update the reference to the final node. Consider how to insert the new node following the previous last node, and keep in mind that the next reference for the list's last node should be null. * Create a different method or function that iteratively explores the list, printing the int data values as…arrow_forward
- Suppose a node of a linked list is defined as follows in your program: typedef struct{ char data; struct Node* link; } Node; Write the definitions of two functions (i) Node* pop(Node* top) – which removes the top element of the stack, and (ii) bool compare(Node* topA, Node* topB) - which returns true if the both stacks have identical data. The function returns false if the data in both stacks are not identical. In the function definitions, top, topA, and topB point the top elements of corresponding stacks. You must use the following function definitions in your answer. The peek function has already been defined. You need to write the definition of pop and compare functions. You are not allowed to modify the parameters and/or return types of the functions. Node* pop(Node* top){ //write the function definition } char peek(Node* top){ return top->data; } bool compare(Node* topA, Node* topB){ //write the function definition } Write the time complexity in Big-O notation…arrow_forwardWrite a program which will concatenate two single linked lists. The structure is defined below. Assume that head1 contains the starting address of the first list and head2 contains the starting address of the second list. You need to join them together so that all the students’ roll no and names of both the lists will be displayed together with the help of head1 pointer. struct link { int rollno; char name[20]; struct link *next; }; typedef struct link node; node *head1=NULL, *head2=NULL; //global declarationarrow_forwardProvide full C++ Code: Your code must have the following three files: Playlist.hpp - Class declaration for a linked list of Nodes (very similar to lab 08 but with some changes to methods, as described below) with following private data members: private:Node* first;Node* last;int size; Playlist.cpp - Class definition main.cpp - main() function Checkpoint A Build a playlist (of songs) by reading data members of several songs from a file and printing them. Implement the following methods: PlayList(); //Default constructorPlayList(string filename); //Parameterized constructor that reads data members of song from a file and builds the PlayList~PlayList(); //Deletes all nodes of the linked listbool InsertNodeLast(Node *myNewNode); //Inserts myNewNode at the end of the linked listbool InsertNodeFirst(Node *myNewNode); //Inserts myNewNode at the start of the linked listbool DeleteFirst(); //Deletes the first node of the linked listint Size() const { return size; }friend ostream&…arrow_forward
- No need source code Write a sample function with one parameter that is a head pointer for a linked list of items. The function prints all the values of the items to console.e.g., 1 -> 2 -> 3 -> 4 -> 5arrow_forwardprogram by using C language In the struct structure given below, the data of a student is stored in a singly linear linked list. Write a function named "secondHighest" that takes the starting address of the list as a parameter and returns the student number with the second highest grade? typedef struct node { int OgrNo; int not; struct node *next; };arrow_forwardFor the following problems, you need to submit a python code that performs the required functions. Q2) Write a member functions to the class (CircularQueue) that performs the followings: 1.Sum-even(CQ) : Print the sum of all even numbers stored in CircularQueue. 2. Perform the followings: a. Create two circular linked lists (CQ1, CQ2). b. Merge the two circular linked lists in one →CQ1. //Remember: you need to use the class (CircularQueue) methods.//arrow_forward
- in c++ In a linked list made of integer data type, write only an application file that prints thoseintegers that are divisible by 5. Note that you assume that linked list exists, you onlyprints the value of data items that meet the condition (divisible by 5)arrow_forwardSuppose you have two objects of Doubly Linked List D1 and D2. Each object is representing a different Doubly Linked List. Write a function to concatenate both doubly linked lists. The concatenation function must return the address of head node of concatenated Doubly Linked List, and it must take two Node* parameters. You need to keep in mind all exceptional cases for example what if either one of the doubly linked lists is empty? Perform this task on paper, take a clear picture of solution and paste it in answer section.arrow_forward*IN C++ A handy feature of lists in Python is that you can use negative indices to get at elements starting from the very end. For example, x[-1] returns the last element in x, x[-2] returns the penultimate element, etc. Implement a struct named "PythonicIntVector" in the files "PythonicIntVector.h" and "PythonicIntVector.cpp" that supports the push_back and at methods of a vector of ints. However, be sure to add the functionality of negative indices. Don't forget that the at method still should throw exceptions for invalid indices (just like in Python)!arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
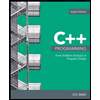
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning