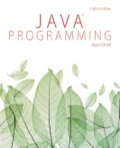
Concept explainers
Explanation of Solution
Program:
File name: “JCarlysCaterring.java”
//Import necessary header files
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
//Define a class named JCarlysCatering
public class JCarlysCatering extends JFrame implements ItemListener, ActionListener
{
//Construct a new FlowLayout object
FlowLayout flow = new FlowLayout();
//Create a JTextField component for capturing number of //guests
JTextField guestsField = new JTextField(8);
//Create a label with the text "Please enter number of //guests"
JLabel guestsLabel = new JLabel("Please enter number of guests");
//Define CheckBoxes to capture user input for main course,
//side, items, and desert.
JCheckBox beefBox = new JCheckBox("Beef", false);
JCheckBox chickenBox = new JCheckBox("Chicken", false);
JCheckBox fishBox = new JCheckBox("Fish", false);
JCheckBox pastaBox = new JCheckBox("Pasta", false);
JCheckBox saladBox = new JCheckBox("Salad", false);
JCheckBox vegBox = new JCheckBox("Mixed vegetables", false);
JCheckBox potBox = new JCheckBox("Baked potato", false);
JCheckBox breadBox = new JCheckBox("Garlic bread", false);
JCheckBox cakeBox = new JCheckBox("Chocolate cake", false);
JCheckBox pieBox = new JCheckBox("Apple pie", false);
JCheckBox pudBox = new JCheckBox("Butterscotch pudding", false);
//Create a label with the text "Carly's Catering"
JLabel mainLabel = new JLabel("Carly's Catering");
//Set the font style of mainLabel contents
Font font = new Font("Ariel",Font.ITALIC, 30);
//Create a label with the text "Total"
JLabel label2 = new JLabel("Total");
//Create a label with the text "Select options"
JLabel label1 = new JLabel("Select options");
JLabel totPrice = new JLabel();
//Initialize the required variables
double price = 0;
String entreeString = "";
String sideString = "";
String dessertString = "";
String output;
int numSelected = 0;
//Define a default constructor
public JCarlysCatering()
{
//Set the title of the JFrame container
super("Menu options");
//Set the close and layout of the JFrame container
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLayout(flow);
//Define a ButtonGroup object entreeGroup
ButtonGroup entreeGroup = new ButtonGroup();
//Add the check boxes for the main menu items
//to the entreeGroup
entreeGroup.add(beefBox);
entreeGroup.add(chickenBox);
entreeGroup.add(fishBox);
entreeGroup.add(pastaBox);
//Define a ButtonGroup object dessertGroup
ButtonGroup dessertGroup = new ButtonGroup();
//Add the check boxes for the dessert menu items
//to the dessertGroup
dessertGroup.add(cakeBox);
dessertGroup.add(pieBox);
dessertGroup.add(pudBox);
//Add respective components in an
//order to the container
add(mainLabel);
add(guestsLabel);
add(guestsField);
add(label1);
add(beefBox);
add(chickenBox);
add(fishBox);
add(pastaBox);
add(saladBox);
add(vegBox);
add(potBox);
add(breadBox);
add(cakeBox);
add(pieBox);
add(pudBox);
mainLabel.setFont(font);
add(label2);
add(totPrice);
totPrice.setText("$0");
//Add the item listener to the buttons
guestsField.addActionListener(this);
beefBox.addItemListener(this);
chickenBox.addItemListener(this);
fishBox.addItemListener(this);
pastaBox.addItemListener(this);
saladBox.addItemListener(this);
vegBox.addItemListener(this);
potBox.addItemListener(this);
breadBox.addItemListener(this);
cakeBox.addItemListener(this);
pieBox.addItemListener(this);
pudBox.addItemListener(this);
}
//Override method
@Override
public void actionPerformed(ActionEvent e)
{
//Get the source
Object source = e.getSource();
final int PRICE_PER_GUEST = 35;
//Check whether the text filed
if(source == guestsField)
{
//Try block
try
{
//Calculate the price
price = Integer.parseInt(guestsField.getText()) * PRICE_PER_GUEST;
}
//Catch exception
catch(Exception exc)
{
//Set the number of quests to zero
//when non-numeric value is entered
price = 0;
}
//Set the output string
output = "$" + price + " Menu includes " + entreeString +
sideString + dessertString;
totPrice...

Want to see the full answer?
Check out a sample textbook solution
Chapter 14 Solutions
EBK JAVA PROGRAMMING
- Create an enumeration named Month that holds values for the months of the year, starting with JANUARY equal to 1. (Recall that an enumeration must be placed within a class but outside of any method.) Write a GUI program named MonthNamesGUl that prompts the user for a month integer. Convert the users entry to a Month value, and display it.arrow_forwardWrite a GUI program named ProjectedRaisesGUI that allows a user to enter an salary. Then display, with explanatory text, next years salary, which reflects a 4 percent increase.arrow_forwardWrite a GUI program named InchesToCentimetersGUl that allows the user to input a measurement in inches, click a Button, and output the value in centimeters. There are 2.54 centimeters in an inch.arrow_forward
- Write a GUI program named Eggs InteractiveGUl that allows a user to input the number of eggs produced in a month by each of five chickens. Sum the eggs, then display the total in dozens and eggs. For example, a total of 127 eggs is 10 dozen and 7 eggs.arrow_forwardIn Chapter 2, you created a program for the Greenville Idol competition that prompts a user for the number of contestants entered in last years competition and in this years competition. The program displays the revenue expected for this years competition if each contestant pays a $25 entrance fee. The application also displays a statement that indicates whether this years competition has more contestants than last years. Now, create an interactive GUI program named GreenvilleRevenueGUl that performs all the same tasks.arrow_forwardI want to create a program for the user to enter the height and weight, perform simple mathematical operations, and tell them whether the weight is healthy or not simple GUI please with buttons and evreythingarrow_forward
- OBAFGKM are the seven categories that are used to describe the absorption lines of a spectrum. Access the Spectral Types Color Slider by opening the Eclipsing Binary Stars section of the NAAP Labs Application and then the Spectral Types of Stars subsection (it is the last simulation on the page) and use it to complete the data table below. Spectral Type: include a letter and a number (no space). Temperature: Kelvin (K) is assumed for Temperature. Color: choose from Blue, White, Orange, or Red (spelling counts!).arrow_forwardCreate a GUI that pops up ONLY if the truck is selected. This new window should contain JLabels with JTextFields that allow the user to enter in all the truck's relevant information - e.g. Make, Model, Year, Number of Doors, Fuel Tank Capacity, Driving Range, Towing Capacity, Payload Capacity, and Extra Load. Use the same inputs from Main.java in the Appendix. The new window should also include two calculate MPG buttons – one for MPG without the extra load and one for MPG with the extra load. When pressed, the buttons use the truck constructor to compute the MPG and output the miles per gallon for each scenario. In this case, the MPG without the extra load is 25.0 and the MPG with the extra load is 24.25. Note: assume that the extra load is 100arrow_forwardThis is in Python. Write a GUI program in which you ask the user to enter the number of miles driven in hour 1, the number of miles driven in hour 2, and the number of miles driven in hour 3. When you click the total button the output in the same GUI should say: the number of miles driven in the three hours is 190 miles (if hour 1 entry is 70, hour 2 entry is 72 and hour 3 entry is 48). There should be three labels, three entry boxes, and two buttons(total and quit)arrow_forward
- A county collects property taxes on the assessment value of property, which is 60 percent of the property’s actual value. If an acre of land is valued at $10,000, its assessment value is $6,000. The property tax is then $0.64 for each $100 of the assessment value. The tax for the acre assessed at $6,000 will be $38.40. Create a GUI application that displays the assess- ment value and property tax when a user enters the actual value of a property.arrow_forwardWrite a JFrame that uses two text boxes to accept two integer numbers. When the user clicks the button "Calculate", arithmetic operations can be performed and the results of the operations can be output. the results of the operations are placed in the multi-line text area JTtextArea component. The GUI interface reference is as follows. When doing division, if the remainder is 0, the integer quotient will be displayed, otherwise, a floating point number with two decimal places will be displayed. The output of the programarrow_forwardUsing Java , please provide a CourseInterFace Class in which the user can click multiple interactive buttons with courses. Dashboard Button one will be "Math101.01". Dashboard Button 2 will be named "MATH211.08". Dashboard Button 3 will be named "MATH 267"arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- Programming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
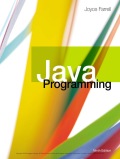
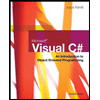
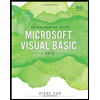
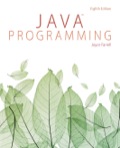