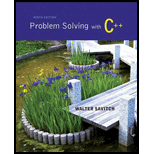
Concept explainers
Explanation of Solution
Given code:
//Header file
#include <iostream>
//For standard input and output
using namespace std;
//Function declaration for "rose" function
int rose(int n);
//Precondition: n >= 0.
//Main function
int main()
{
/* Call function "rose()" with argument "4" and display it */
cout << rose(4);
return 0;
}
/* Function definition for rose() function with parameter "n" */
int rose(int n)
{
/* If "n" is less than or equal to "1", then */
if (n <= 0)
//Returns "1"
return 1;
//Otherwise
else
/* Recursively call the function "rose()" with decrement the value of "n" by "1" and multiply by "n" */
return (rose(n - 1) * n);
}
Explanation:
The given code is used to display the value after calling the function “rose()”.
- First, declare the function for “rose()”.
- Define main function
- Call “rose()” function with argument “4” and display the given value.
- Define “rose()” function with parameter “n”.
- If “n” is less than or equal to “1”, then returns “1”.
- Otherwise, recursively call the function “rose()” with decrement the value of “n” by “1” and then multiply with “n”.
Reasons for displaying given output:
- In main function, call “rose()” function with argument “4”.
- So, in the function “rose()”, first check the value of “n”.
- Here, the value of “n” is not less than or not equal to “1”. So, performs “else” statement.
- In “else” statement, return “(rose(4 - 1) * 4)” implies “(rose(3) * 4)”...
- Here, the value of “n” is not less than or not equal to “1”. So, performs “else” statement.

Want to see the full answer?
Check out a sample textbook solution
Chapter 14 Solutions
Problem Solving with C++ (9th Edition)
- Implement in C Programming 6.10.2: Pass by pointer. Write a function call to ConvertMoney() to store the number of quarters, nickels, and pennies within the integer variables numQuarters, numNickels, and numPennies, respectively. Ex: If the input is 133, then the output is: Quarters: 5 Nickels: 1 Pennies: 3 #include <stdio.h> void ConvertMoney(int totalPennies, int* numQuarters, int* numNickels, int* numPennies) { *numQuarters = totalPennies / 25; totalPennies = totalPennies % 25; *numNickels = totalPennies / 5; totalPennies = totalPennies % 5; *numPennies = totalPennies;} int main(void) { int totalPennies; int numQuarters; int numNickels; int numPennies; scanf("%d", &totalPennies); /* Your code goes here */ printf("Quarters: %d\n", numQuarters); printf("Nickels: %d\n", numNickels); printf("Pennies: %d\n", numPennies); return 0;}arrow_forward12. Consider the recursive function int gcd( int a, int b) int gcd( int a, int b){ if (b > a) return gcd(b,a); if ( b == 0 ) return a; return gcd( b, a% b); } How many invocation (calls) of the gcd() function will be made by the call gcd(72, 30)?arrow_forwardWrite a recursive void function that has one parameter that is a positive integer. When called, the function writes its argument to the screen backward. That is,, if the argument is 1234, it outputs the following to the screen: 4321. I've literally tried to create this program and I have no idea how to make it work. Please help me. Is it possible to explain line by line what the code does?arrow_forward
- 1.The following is the C code that you need to implement for this lab: uint8_ t f(uint8_tn) return(n<2)?(n):(f(n-1)+f(n-2)); The main function can be assumed as follows: int main() uint8_ t x; x=f(???); return 0; Obviously, " ? ? ? " is representing a value used to test the algorithm! Is "f"recursive? a.No answer text provided. b.Yes c.no d.depends on whether it is in for main 2.First, make the code in the previous question an actual C program so that it can be compiled and it runs. Play with it so that you feel comfortable with the logic of the code. Then implement the code in TTPASM. Note that you need to preserve the actual C code structure, this means you cannot it into a non-recursive subroutine. Furthermoreall conventions discussed in class regarding subroutines must be followed. The idea is that I should be able to substitute f with my own code, and main should work. Or, I can substitute main with my own, and f should work. Attach the source code of your assembly…arrow_forwardA java program where the system should use the following methods: .a) Lagrange interpolation for finding missing values of a function .b) A function implementing multiple application of the trapezoidal rule .c) A function implementing the composite Simpson's rule SHOULD ALLOW THE USER TO : Enter the known values of a function to be integrated or load them from a text file Enter a value of the parameter h and n (the number of intervals) .c) Choose a method to be usedarrow_forwardIn C++ Consider the following recursive function (Chapter 17, #9, modified) void recFun(int x) { if (x > 10) { recFun(x / 10); cout<< x % 10; } else cout<< x; } How can the code be modified so that the sum of all digits is printed?arrow_forward
- Write a Haskell program numBulls that takes as input two positive integers as input and returns -1 if either the two integers have different number of digits or either of them have repeating digits. However, if the two integers have the same number of digits and also do not have any repeating digits, the function returns the number of digits that match in the exact same positions in the integers.arrow_forwardGive solution in C ++ Language with secreenshoot of source code. Part 01In this task, you need to do the following:• Write a function named displayMessage() that takes user name as input in character array and then shows greetings• Now take the name input in main() and pass the name as an argument to displayMessage() function• Change the displayMessage() method such that it returns the number of characters after displaying the greetings part 02Write a function power that takes two parameters a and b. And it returns the power as ab.arrow_forwardConsider the following recursive function: def rec1(aList,first,last): if first==last: return aList[first] else: return aList[last]*rec1(aList,first,last-1) Show the output of the following call (show your work and all recursive calls): print(rec1([1,2,3,4,5,6,7],3,5))? describe the task of the rec1 function.arrow_forward
- Write in C++ a polymorphic and recursive function, print(x, n, w),which can print an object, x, the given number of times, n, separated by the user’s choice of white space character, w.Both n and w must be “default arguments”. The default value of “n” must be 1 and the default value of “w” must be the space character.(1) (2) (3)NB: If any part of your solution to a question has been extracted from a book, past tests or exams, then full disclosure must be given with complete references to the source. Non-disclosures will carry heavy penalties.arrow_forwardWrite a definition for a void-function that has two int value parameters and outputs to the screen the product of these arguments. Write a main function that asks the user for these two numbers, reads them in, calls your function, then terminates. c++arrow_forwardGiven two integers x snf y, the following recurrsive definition determined the greatest common divisor of x and y, Write gcd(xy). Write a recursive function, gcd, that takes two integers as parameters and returns the greatest commmon divisorof numbers.Also write a program to test your function. Write a recursive function,reverseDigits, that takes an integer as a parameter snd returns the number with the digits reversed. Also write a program to test your application.arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
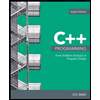