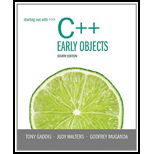
Starting Out with C++: Early Objects
8th Edition
ISBN: 9780133360929
Author: Tony Gaddis, Judy Walters, Godfrey Muganda
Publisher: Addison-Wesley
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 15, Problem 18RQE
Program Plan Intro
- Start a program.
- Declare a class named “Sorter”.
- Declare a required member variables and pure virtual function.
- In public, define the member function “set_Array()”.
- Inside the function set the array and size of an array.
- Define the “sort” function.
- Inside the function, call the “sort()” function with an argument size.
- Define the “sort” function.
- If the size is less than 1, the condition will ended.
- Find the position of largest value in array and put it at the end of the array.
- Use the “compare ()” function for sorting the given array.
- Swap a pair of array elements.
- Decrement the size by 1.
- Define the derived class “Incr_Sorter” from the class “Sorter”.
- In private, define the pure virtual function “compare()”.
- If the “x” value is greater than “y” value return true.
- In private, define the pure virtual function “compare()”.
- Define the derived class “Decr_Sorter” from the class “Sorter”.
- In private, define the pure virtual function “compare()”.
- If the “x” value is less than “y” value return true.
- In private, define the pure virtual function “compare()”.
- Inside the “main” function,
- Create the objects for the classes.
- Declare and initialize an array of 5 values.
- Call the “Incr_Sorter” function.
- Call the “print_Array()” function for displaying the output.
- Call the “Decr_Sorter” function.
- Call the “print_Array()” function for displaying the output.
- Define the “print_Array()” function.
- Display the array.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Write the definition of the function template reverseVector to reverse the elements of a vector object.
template<class elemType>
void reverseVector(vector<elemType> &list);
//Reverses the elements of the vector list.
//Example: Suppose list = {4, 8, 2, 5}.
// After a call to this function, list = {5, 2, 8, 4}.
Also, write the (C++) program to test the function reverseVector. When declaring the vector object, do not specify its size. Use the function push_back to insert elements in the vector object.
Binary Search
Write a C++ Program for searching an element on a given list of integers using the Binary Search method.Strictly adhere to the Object-Oriented specifications given in the problem statement. All class names, member variable names, and function names should be the same as specified in the problem statement.Initialize the array size in the constructor of the Search class.
Function
Description
int search(int n, int k)
The function defines the logic for searching an element from the given array.If the element is found return '1'. Otherwise, return '0'.
Input Format:The first line is an integer 'n' which corresponds to the number of elements in the array.The next 'n' lines correspond to the elements of the array.The last line is an integer 'k' which corresponds to the key element to be searched.Output Format:Print "Element Found" if the element is present in the array otherwise print "Element not Found".[All text in bold corresponds to the input and the rest…
In C++, define a “Conflict” function that accepts an array of Course object pointers. It will return two numbers:- the count of courses with conflicting days. If there is more than one course scheduled in the same day, it is considered a conflict. It will return if there is no conflict.- which day of the week has the most conflict. It will return 0 if there is none.
Show how this function is being called and returning proper values.
you may want to define a local integer array containing the count for each day of the week with the initial value of 0.Whenever you have a Course object with a specific day, you can increment that count for that corresponding index in the schedule array.
Chapter 15 Solutions
Starting Out with C++: Early Objects
Ch. 15.3 - Prob. 15.1CPCh. 15.3 - Prob. 15.2CPCh. 15.3 - What will the following program display? #include...Ch. 15.3 - What will the following program display? #include...Ch. 15.3 - What will the following program display? #include...Ch. 15.3 - What will the following program display? #include...Ch. 15.3 - How can you tell from looking at a class...Ch. 15.3 - What makes an abstract class different from other...Ch. 15.3 - Examine the following classes. The table lists the...Ch. 15 - A class that cannot be instantiated is a(n) _____...
Ch. 15 - A member function of a class that is not...Ch. 15 - A class with at least one pure virtual member...Ch. 15 - In order to use dynamic binding, a member function...Ch. 15 - Static binding takes place at _____ time.Ch. 15 - Prob. 6RQECh. 15 - Prob. 7RQECh. 15 - Prob. 8RQECh. 15 - The is-a relation between classes is best...Ch. 15 - The has-a relation between classes is best...Ch. 15 - If every C1 class object can be used as a C2 class...Ch. 15 - A collection of abstract classes defining an...Ch. 15 - C++ Language Elements Suppose that the classes Dog...Ch. 15 - Will the statement pAnimal = new Cat; compile?Ch. 15 - Will the statement pCreature = new Dog ; compile?Ch. 15 - Will the statement pCat = new Animal; compile?Ch. 15 - Rewrite the following two statements to get them...Ch. 15 - Prob. 18RQECh. 15 - Find all errors in the following fragment of code,...Ch. 15 - Soft Skills 22. Suppose that you need to have a...Ch. 15 - Prob. 1PCCh. 15 - Prob. 2PCCh. 15 - Sequence Sum A sequence of integers such as 1, 3,...Ch. 15 - Prob. 4PCCh. 15 - File Filter A file filter reads an input file,...Ch. 15 - Bumper Shapes Write a program that creates two...Ch. 15 - Bow Tie In Tying It All Together, we defined a...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Write a function in C++ which (normally) allocates new space for a double array. It should alter its pointer argument to point to the newly allocated space. The size of the array should be a second argument. If this argument is not a valid array size, allocate a single double instead of an array. An example call might be: double * p; allocate(p, 25); // p now points to an array of 25 doubles -- if successfularrow_forwardin c++ . Create a class IntegerSet for which each object can hold integers in the range 0 through 100. A set is represented internally as an array of ones and zeros. Array element a[ i ] is 1 if integer i is in the set. Array element a[ j ] is 0 if integer j is not in the set. • The default constructor initializes a set to the so-called “empty set,” i.e., a set whose array representation contains all zeros. • Provide member functions for the common set operations. For example, provide a unionOfSets member function that creates a third set that is the settheoretic union of two existing sets (i.e., an element of the third set’s array is set to 1 if that element is 1 in either or both of the existing sets, and an element of the third set’s array is set to 0 if that element is 0 in each of the existingsets). Provide an intersectionOfSets member function which creates a third set which is the set theoretic intersection of two existing sets (i.e., an element of the third set’s array is set…arrow_forwardin c++ Declare and implement 5 classes: FloatArray, SortedArray, FrontArray, PositiveArray & NegativeArray. 1- The FloatArray class stores a dynamic array of floats and its size. It has: - A parameterized constructor that takes the array size. - An add method that adds a float at the end of the array. - Overloading for the insertion operator << to write the array to a file (ofstream) - Overloading for the extraction operator >> to read the array elements from the file (ifstream) and add them to the array. - A destructor to deallocate the array 2- The SortedArray inherits from FloatArray. It has: - A parameterized constructor that takes the array size. - An add method that adds a float at the right place in the array such that the array remains sorted with every add. Don’t add to the array then sort but rather add in the right place. 3- The FrontArray inherits from FloatArray. It has: - A parameterized constructor that takes the array size. - An add method that adds a…arrow_forward
- ( In c++and oop ) without using vectors or data structure . Create a class IntegerSet for which each object can hold integers in the range 0 through 100. A set is represented internally as an array of ones and zeros. Array element a[ i ] is 1 if integer i is in the set. Array element a[ j ] is 0 if integer j is not in the set. • The default constructor initializes a set to the so-called “empty set,” i.e., a set whose array representation contains all zeros. • Provide member functions for the common set operations. For example, provide a unionOfSets member function that creates a third set that is the settheoretic union of two existing sets (i.e., an element of the third set’s array is set to 1 if that element is 1 in either or both of the existing sets, and an element of the third set’s array is set to 0 if that element is 0 in each of the existingsets). Provide an intersectionOfSets member function which creates a third set which is the set theoretic intersection of two existing sets…arrow_forwardWrite a program in C++ with class template to represent a generic vector. Include member functions to perform the following tasks: (a)to create a vector (b)to modify the value of a given element (c)to multiply by a scalar value (d)to display the vector in the form (10,20,30,...)arrow_forwardC++ programming Recall that in C++, there is no check on an array index out of bounds. However, during program execution, an array index out of bounds can cause serious problems. Also, in C++, the array index starts at 0. Design and implement the class myArray that solves the array index out of bounds problem and also allows the user to begin the array index starting at any integer, positive or negative. Every object of type myArray is an array of type int. During execution, when accessing an array component, if the index is out of bounds, the program must terminate with an appropriate error message. Consider the following statements: myArray list(5); //Line1 myArray myList(2, 13); //Line 2 myArray yourList(-5, 9); //Line 3 The statement in Line 1 declares list to be an array of five components, the component type is int, and the components are: list[0], list[1], ..., list[4]; The statement in Line 2 declares myList to be an array of 11 components, the component…arrow_forward
- Write c++ code Implement a class (template) SortedSet which is actually a singly linked list with head and tail pointers. It will store data in ascending order while disallowing duplicate data. Implement the following member functions for this class.1. Default Constructor. SortedSet();2. An insert function that will insert the data such that resultant set is in ascending order. Duplicate data will not be allowed. void insert(T const data);3. A delete function that will delete the element at the given index. void delete(int const index);4. A print function that will print the contents of the sorted set. void print() const;5. A union function that will be passed another sorted set. This function will take union of two setsand store the result in the first set. void union(SortedSet<T>const &otherSet);For example:SortedSet a; (suppose it has 1, 2, 3, 4, 10, 50)SortedSet b; (suppose it has 6, 10, 11)a.union(b); (a will now contain 1, 2, 3, 4, 6, 10, 11, 50)6. An intersection…arrow_forwardPlease write a full C++ code and provide code and output Two stacks of the same type are the same if they have the same number of elements and their elements at the corresponding positions are the same. Overload the relational operator == for the class linkedStackTypethat returns true if two stacks of the same type are the same; it returns false otherwise. Also, write the definition of the function template to overload this operator.arrow_forwardWrite a Menu Driven C++ program that creates one-dimensional array arr[] and initialize it with user. Theprogram should do following Tasks using Menu, the menu operations are implemented using functions:a) Write a function Count(), that counts the occurrences of x (a number) in arr[].b) Write a function Partition(), that take the first element of the array x and put x in a position suchthat all smaller elements (smaller than x) are before x, and put all greater elements (greaterthan x) after x.c) Write a function next_XOR(),the count of elements which are equal to the XOR of the next twoelements.d) Write a function Duplicates(),which calculated the frequencies of all the elements and displaythem.e) Write a function Circular(),which replace every element of the array by the sum of next twoconsecutive elements in a circular manner i.e. arr[0] = arr[1] + arr[2], arr[1] = arr[2] + arr[3],... arr[n – 1] = arr[0] + arr[1].f) Write a function Search(), takes an array and element to search in…arrow_forward
- I have written a class that has the characteristics of the data structure Set and within it house an array that stores 10 integers. I would like a function written in C++ that will perform a set union comparison between two class set instances and only print out one of each element from both of them, (no duplicates). Thanks!arrow_forwardWrite a C++ program to search an element in an array using a function template. Note: Define an array size using Preprocessor directivesExample: #define array_size 5 Strictly adhere to the Object-Oriented specifications given in the problem statement. All class names, member variable names, and function names should be the same as specified in the problem statement. Include following member function using Template Function Name Description T SearchInArray(T x[], T element) This function is used to search an element in an array. If the element is found it returns true otherwise false. include in function:- If an element is found in an array then print "Element # is found" otherwise "Element # is not found. (Eg. Element 4 is found) In the main method, read the array elements and search for an element in the array. Input and Output Format: Refer sample Input and output for formatting specifications. Sample Input and…arrow_forwardWrite a C++ program to search an element in an array using a function template. Note: Define an array size using Preprocessor directivesExample: #define array_size 5 Strictly adhere to the Object-Oriented specifications given in the problem statement. All class names, member variable names, and function names should be the same as specified in the problem statement. Include following member function using Template Function Name Description T SearchInArray(T x[], T element) This function is used to search an element in an array. If the element is found it returns true otherwise false. In the main method, read the array elements and search for an element in the array. Input and Output Format:If an element is found in an array then print "Element # is found" otherwise "Element # is not found. (Eg. Element 4 is found) Refer sample Input and output for formatting specifications. Sample Input and Output: [ All text in bold…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
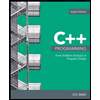
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning