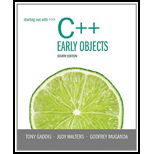
Starting Out with C++: Early Objects
8th Edition
ISBN: 9780133360929
Author: Tony Gaddis, Judy Walters, Godfrey Muganda
Publisher: Addison-Wesley
expand_more
expand_more
format_list_bulleted
Question
Chapter 15, Problem 2PC
Program Plan Intro
Analysis of Quicksort
Program Plan:
- Include the required header files to the program.
- Define “AbstractSort” class.
- In public, declare the pure virtual function.
- In the “get_comparison” function return the total number of comparison.
- In protected, declare the “compare” function.
- In the “reset_comparison” function reset the comparison value to “0”.
- In private, declare the required variable.
- In public, declare the pure virtual function.
- Define the “compare” function outside the class definition.
- Inside the function, increment the comparison count and return the number of comparison.
- Define the derived class “Quicksort”.
- In public, declare the “sort” function.
- In private, declare the “quick” function and “partition” function.
- Define the “quick” function.
- If the least number is greater than the highest number, return the value to the function.
- Call the “partition”, quick” functions.
- Define the “partition” function.
- Set the pivot value.
- Declare and set the “front” variable.
- If the “front” value is less than “u”, swap the values and increment the pivot variable.
- Increment the “front” variable.
- Define the “main()” function.
- Declare and initialize the required variables.
- Get the array value from the user.
- Check the array value with array index.
- If the array value is greater than index value exits the program.
- Initialize the random number generator and generate the random numbers.
- Create the object for the class “Quicksort”.
- Call the “sort” function.
- Display the result.
Expert Solution & Answer

Trending nowThis is a popular solution!

Students have asked these similar questions
A dequeue is a list from which elements can be inserted or deleted at either end
a. Develop an array based implementation for dequeue.
b. Develop a pointer based implementation dequeue.
This chapter describes the array implementation of queues that use a special array slot, called the reserved slot, to distinguish between an empty and a full queue.
Write the definition of the class and the definitions of the function members of this queue design.
Also, write a program (in main.cpp) to test various operations on a queue.
//Header file QueueAsArray
#ifndef H_QueueAsArray
#define H_QueueAsArray
#include <iostream>
#include <cassert>
using namespace std;
template<class Type>
class queueType
{
public:
const queueType<Type>& operator=(const queueType<Type>&);
// overload the assignment operator
void initializeQueue();
int isEmptyQueue() const;
int isFullQueue() const;
Type front() const;
Type back() const;
void addQueue(Type queueElement);
void deleteQueue();
queueType(int queueSize = 100);
queueType(const queueType<Type>& otherQueue); // copy constructor…
It is not desirable to use a static array like aux[] in library software since numerous clients may be using the class at once. Give a Merge implementation that doesn't depend on a static array. Do not localise aux[] to combine()Hint: Pass the auxiliary array to the recursive sort() function as an argument.
Chapter 15 Solutions
Starting Out with C++: Early Objects
Ch. 15.3 - Prob. 15.1CPCh. 15.3 - Prob. 15.2CPCh. 15.3 - What will the following program display? #include...Ch. 15.3 - What will the following program display? #include...Ch. 15.3 - What will the following program display? #include...Ch. 15.3 - What will the following program display? #include...Ch. 15.3 - How can you tell from looking at a class...Ch. 15.3 - What makes an abstract class different from other...Ch. 15.3 - Examine the following classes. The table lists the...Ch. 15 - A class that cannot be instantiated is a(n) _____...
Ch. 15 - A member function of a class that is not...Ch. 15 - A class with at least one pure virtual member...Ch. 15 - In order to use dynamic binding, a member function...Ch. 15 - Static binding takes place at _____ time.Ch. 15 - Prob. 6RQECh. 15 - Prob. 7RQECh. 15 - Prob. 8RQECh. 15 - The is-a relation between classes is best...Ch. 15 - The has-a relation between classes is best...Ch. 15 - If every C1 class object can be used as a C2 class...Ch. 15 - A collection of abstract classes defining an...Ch. 15 - C++ Language Elements Suppose that the classes Dog...Ch. 15 - Will the statement pAnimal = new Cat; compile?Ch. 15 - Will the statement pCreature = new Dog ; compile?Ch. 15 - Will the statement pCat = new Animal; compile?Ch. 15 - Rewrite the following two statements to get them...Ch. 15 - Prob. 18RQECh. 15 - Find all errors in the following fragment of code,...Ch. 15 - Soft Skills 22. Suppose that you need to have a...Ch. 15 - Prob. 1PCCh. 15 - Prob. 2PCCh. 15 - Sequence Sum A sequence of integers such as 1, 3,...Ch. 15 - Prob. 4PCCh. 15 - File Filter A file filter reads an input file,...Ch. 15 - Bumper Shapes Write a program that creates two...Ch. 15 - Bow Tie In Tying It All Together, we defined a...
Knowledge Booster
Similar questions
- Need help with SML/ML/MPL I need to write binarySearch function that recursively implements the binary search algorithm to search a sorted integer list for a specified integer and returns true if it is found, false otherwise. For example, binarySearch ([100,200,300,400,500], 200) returns true, whereas binarySearch([100,200,300,400,500], 299) returns false. Hint: Write a helper function mid that returns a tuple (index, value) representing the middle value in a list. For example, mid [10, 2, 40, 8, 22] returns (2,40) because the value 40 at index 2 is the middle value in the list. Similarly, mid [10, 20] would return (1, 20). Use mid in conjunction with slice to implement binarySearch.arrow_forwardThis chapter described the array implementation of queues that use a special array slot, called the reserved slot, to distinguish between an empty and a full queue. Write the definition of the class and the definitions of the function members of this queue design. Also, write a test program to test various operations on a queue.arrow_forward8.12 LAB: Binary search Binary search can be implemented as a recursive algorithm. Each call makes a recursive call on one-half of the list the call received as an argument. Complete the recursive method binarySearch() with the following specifications: Parameters: a target integer an ArrayList of integers lower and upper bounds within which the recursive call will search Return value: the index within the ArrayList where the target is located -1 if target is not found The template provides main() and a helper function that reads an ArrayList from input. The algorithm begins by choosing an index midway between the lower and upper bounds. If target == integers.get(index) return index If lower == upper, return -1 to indicate not found Otherwise call the function recursively on half the ArrayList parameter: If integers.get(index) < target, search the ArrayList from index + 1 to upper If integers.get(index) > target, search the ArrayList from lower to index - 1…arrow_forward
- Code Restructuring: Methods for Linked List operations are given. Restructure the codes to improve performance of codes. Linked List is a part of the Collection framework present in java.util package, however, to be able to check the complexity of Linked List operations, we can recode the data structure based on Java Documentation https://docs.oracle.com/javase/8/docs/api/java/util/LinkedList.html Instruction 1: Two (2) Java classes are provided, first, the Linked List class and second, the Linked List interface. On the Linked List class, check the comments, analyze then try to recode the said method/s. Post the changes in your code, if any. package com.linkedlist; public class LinkedList<E> implements ListI<E>{ //The Node Object signatures and constructor class Node<E>{ E data; Node<E> next; public Node(E obj) { data=obj; next=null; } }…arrow_forwardStackQueuePostfix A. Pointer_based queuea. Define the class PoiQueue with no implementation; i.e. declare the datamembers, and the function members only (Enqueue, Dequeue, IsEmpty,GetHead etc.).b. Implement the Enqueue method of the above classB. Array_based non-circular queue:a. Define the class Queue using one dimensional array representation with noimplementation; i.e. declare the data members, and the function membersonly (Enqueue, Dequeue, IsEmpty, GetHead etc.).b. Implement the Denqueue method of the above classarrow_forwardList ADT Implementation (via dynamic array) • Implement the following operations of List ADT by using array class (as discussed in the lecture) • Constructors (default, parameterize, copy) & destructor • void printList ( ), int searchElement (int X), void insertElement (int X), void insertElementAt (int X, int pos), bool deleteElement (int X), bool isFull ( ), bool isEmpty ( ), int length ( ), void reverseList ( ), void emptyList ( ), void copyList (...) • Also write a driver (main) program to test your code (provide menu for all operations)arrow_forward
- Wap java code to ArrayList represents an array implementation of a list. The front of the list is kept at array index 0. This class will be extended to create a specific kind of list.arrow_forwardComplete the implementation of the class LinkedSortedList, and write a driver program to fully test it. (Ch. 12, Programming Problems 1, pg. 392 of Data Abstraction and Problem Solving with C++ (7th Edition) ) Name the program sortedlist.cpp. Make sure the following requirements are met. Program must compile and run. Must use the Sorted List ADT SortedListInterface.h For LinkSortedList pg. 376 has the code for insertSorted. You must complete the implementation. Image shown below: Therefore the LinkedSortedList is not based on a LinkedList but uses its own link-based implementation. Do not use throw on function declarations as it is obsolete. Driver program should: create a sorted list insert 21 random numbers (1-100) using the STL random library Display the numbers as they are inserted. Then remove the first number inserted. Last of all display the sorted list of 20 numbers. No user input for driver program. SortedListInterface.h: //Â Created by Frank M. Carrano and Timothy…arrow_forwardNeed help with sml languege I need to write a binarySearch function that recursively implements the binary search algorithm to search a sorted integer list for a specified integer and returns true if it is found, false otherwise. For example, binarySearch ([100,200,300,400,500], 200) returns true, whereas binarySearch([100,200,300,400,500], 299) returns false. Hint: Write a helper function mid that returns a tuple (index, value) representing the middle value in a list. For example, mid [10, 2, 40, 8, 22] returns (2,40) because the value 40 at index 2 is the middle value in the list. Similarly, mid [10, 20] would return (1, 20). Use mid in conjunction with slice to implement binarySearch. Here is my slice function: fun count(index,hd::tl,start,stop) =if index+1 >= stop then hd::nilelse if index >= start then hd::count(index+1,tl,start,stop)else count(index+1,tl,start,stop);fun slice(x::y, start, stop) = count(0,x::y,start,stop); And here is the dummy implementation of binary…arrow_forward
- Merge and quick sort Implementation – Directed Lab Work1. Complete the recursive algorithm for merge sort part in the given data file, ArraySortRecursive.java. 2. Complete the implementation of quick sort by implementing the method partition in thegiven data file, ArraySortRecursive.java.3. Save the file as ArraySortRecursiveYourlastname.java. 4. Save the test driver program as DriverYourlastname.java and run the program and verify.arrow_forwardArray_based circular queue: Define the class Queue using one dimensional circular array representation with no implementation; i.e. declare the data members, and the function members only (Enqueue, Dequeue, IsEmpty, GetHead etc.). Implement the Ennqueue method of the above classarrow_forwardCreate a sort implementation that counts the variety of key values before sorting the array using key-indexed counting using a symbol table. (This approach should not be used if there are many distinct key values.)arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
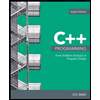
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning