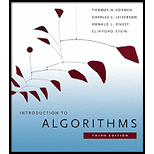
(a)
To discuss the greedy
(a)

Explanation of Solution
To prove that the changing problem of coin has an optimal solution, take ' n ' cent and suppose, for these ' n ' cent changes, there is an optimal solution. The solution takes a coin with value ' c ' and uses ' k ' coins. If the solution of ' n-c ' cent changes problem lies within the solution of ' n ' cent problem solution, that means ' k-1 ' coins are available for ' n-c ' cents. So, take the solution for the ' n-c ' cent changing problem and make sure this should be lesser then ' k- 1 ' coins then figure out the solution for ' n ' cent problem.
While dealing with ' n ' cent problem and try to get an optimal solution by taking minimum no. of coins. Always provide the biggest denomination coin until the rest amount became zero. So, if there is the biggest denomination present at first then by applying greedy methodology changes in quarter, dime, nickel and pennies are:
Q = ⌊ n /25 ⌋ quarter that gives nQ = n mod 25 cent for change.
D = ⌊ nQ /10 ⌋ dimes that gives nD = nQ mod 10 cent for change.
K = ⌊ nD /10 ⌋ dimes that gives nK = nD mod 5 cent for change.
P = nK pennies
By seeing the greedy solution, there are few possibilities like:
- If n = 0; then zero coins in optimal solution.
- If n > 0; then get the biggest denomination with value = ' n ' , suppose it’s ' c ' .
- Now, take a coin and apply process in recursive manner for ' n-c ' cent.
To get the optimal solution through greedy algorithm, one must hold the coin ' c ' with value greater than ' n ' .
There are four cases needs to be considered here:
- If (1= n < 5) then c = 1, this must hold the greedy choice and contains pennies.
- If (5 = n = 10) then c = 5, by supposition, in this it must hold pennies and change 5 pennies to one nickel because this solution is not holding any nickel.
- If (10 = n = 25) then c = 10, by supposition, it must hold a nickel and pennies but not a dime. So, add a dime in the solution.
- If (25 = n ) then c = 25, by supposition, it holds a dime, pennies, and nickel but not quarter. If it has three dimes change it with a quarter and nickel and if has at least two dime then few subset of nickel, dime and pennies add nearly 25 cents and change these coins with a quarter to get a solution.
Here, for showing that it must contains an optimal solution with greedy choices and these choices will further helpful to get the solution of sub problem. Therefore, this greedy approach provides an optimal outcome for the given problem.
For an algorithm that selected a coin and recurses sub problem one by one, the running complexity will be θ ( k ), where ' k ' is the coins used for optimal solution. Now ( k = n), so, running time will be O ( n ) and there is only four type of coins. So, it requires O (1) constant time.
(b)
To show that for given denomination ( c0, c1, c2, ........., ck ), greedy algorithm always gives an optimal solution where ( c > 1) and (1 = k ).
(b)

Explanation of Solution
Take denomination c0, c1, c2, ........., ck and changes amount ' n ' . By taking this, it is clear that
Only few coins are required in changing coin to give an optimal solution. Apply greedy algorithm, take biggest denomination coin first means take a denomination ci (not ck ), where ( k>i ).
- To make sure that solution should be optimal take any denomination ( ci ) no. of coin that must be lesser then ' c ' .
- Take coins of ' ci ' denomination having ( x0, x1, ...., xk ) optimal solution and prove that for every ( k >i ), denomination ( c >xi ).
- And for few j , ( c = xi ) and change denomination coins cj with cj+1coin .
- Therefore, xj reduced by c, xj +1 increased by 1 and total no. of coins reduced by c -1.
This is contradicting about ( x0, x1,....., xk ) being a solution. So, that optimal solution should have denomination ' c ' greater than xi for any ' ci ' denomination.
Thus the only solution of the given problem is xk = n/ck ; for ( k>i ), xi = n mod ci +1/ ci . Therefore this is proved that greedy algorithm with ( c0, c1, c2, ........., ck ) denomination always gives an optimal solution.
(c)
To prove that greedy algorithm does not provides the optimal solution all the time.
(c)

Explanation of Solution
Take the denomination set as {1, 3, 4} and the value of ' n =6’.
Then greedy solution will give two one cent coin {1, 1} and a four-cent coin {4} like {1, 1, 4} but two coins as {3, 3} is optimal solution.
So, for the set {1, 3, 4}, optimal solution will not present by using greedy algorithm.
In the other example where denomination set contains value {1, 10, 25}, for ' n = 30’; greedy algorithm provide a quarter and five pennies {25, 1, 1, 1, 1, 1} but the optimal solution should be three dime like {10, 10, 10}.
Thus, greedy algorithm will not provide an optimal solution always.
(d)
To show that the time complexity of the algorithm is O ( nk ), where ' k ' is the total no. of coins.
(d)

Explanation of Solution
While applying the dynamic programming, take the demonstration ' d ' define as d1 , d2 , d3 ,...., dk and to make changes for ' i ' cent, no. of coins required min( c [ i ]). So, the minimum coins required can be calculated as:
c [ i ] = 1 + min{ c [ i-dj ]}, if ( i > 0) and (1 = j = k ),
c [ i ] = 0, if ( i = 0)
This formula should be implemented as,
MakeChange ( d,n )
- For ( i ← 1) to n
- Do c [ i ] ← 8
- For ( j ← 1) to k
- Do if ( dj = i )
- Then if c [ i ] > c [ i-dj ] + 1
- Then c [ i ] ← c [ i - dj ] + 1
- denom[ i ] ← dj
- Return demon and ' c '
By seeing the algorithmic procedure of MakeChange( d,n ), it is showing that it requires two loops up to ' n ' times as outer loop and ' k ' times as inner loop.
Thus, the complexity of the implementation will be O ( nk ).
Want to see more full solutions like this?
Chapter 16 Solutions
Introduction to Algorithms
- PRINT-OPTIMAL-PARENS(s, i, j){ if (i=j) then print “A”i else{ print “(” PRINT-OPTIMAL-PARENS(s,i,s[i, j]) PRINT-OPTIMAL-PARENS(s, s[i, j] + 1, j) print “)”} } b- Find the complexity of your program? c- Show that the parenthesization algorithm is loop invariant?arrow_forwardGiven the locations of the gas stations in some arbitrary order, describe an algorithm that finds an optimal placement of coffee shops (i.e., a placement of coffee shops that minimizes the number of coffee shops you’d need to open). Hint Try to come up with a greedy algorithm that moves along the turnpike for as long as possible before it’s forced to place a coffee shop.arrow_forwardConsider the problem of providing change for an amount of n-bahts while using the smallest possible quantity of coins. You may assume that each coin’s value is integer. Describe a greedy algorithm to make change consisting of 1-baht coins, 5-baht coins, and 10-baht coins and prove that your algorithm yields an optimal solution.arrow_forward
- Does a greedy algorithm always find an optimal solution? Justify your answer.arrow_forwardConsider the problem of providing change for an amount of n-bahts while using the smallest possible quantity of coins. You may assume that each coin’s value is integer. Assume that the coins available for use have values that can be expressed as powers of c. To clarify, the denominations of the coins can be written as c0,c1,..., ck , where c is an integer greater than 1, and k is a positive integer. Prove that the greedy algorithm always yields an optimal solution.arrow_forwardFind the minimum number of platforms in a train station to avoid delays in the arrival of a train. In other words, given a list of train arrival and departure times, determine in principle what would be the number of operating platforms to avoid delays in the arrival of a train. What would be the greedy strategy if we used a greedy algorithm to solve the problem? To exemplify, use the following information:Arrival = {2.00,2.10,3.00,3.20,3.50,5.00}Output = {2.30,3.40,3.20,4.30,4.00,5.20}arrow_forward
- Algorithm A search using the heuristic h(n) = α for some fixed constant α > 0 is guaranteed to find an optimal solution Select one: True Falsearrow_forwardIs it true that a greedy algorithm always finds the best solution? Justify your response.arrow_forwardGiven a set {y1 ≤ y2 ≤ . . . ≤ yn} of points located on a line, determine the smallest set of unit-length closed intervals that contains all the points (the interval [3, 5] includes all yi such that 3 ≤ yi ≤ 5). Use greedy algorithm to provide an efficient algorithm.arrow_forward
- Consider the following problem. The input consists of n skiers with heights p1, ··· , pn , and n skies with heights s1, ··· , sn. The problem is to assign each skier a ski to to minimize the average difference between the height of a skier and his/her assigned ski. That is, if the ith skier is given the Alpha(i)th ski, then you want to minimize: 1/ffln ∑i=1 to n |pi- s↵(i)| (a) Consider the following greedy algorithm. Find the skier and ski whose height di↵erence is minimized. Assign this skier this ski. Repeat the process until every skier has a ski. Prove of disprove that this algorithm is correct. (b) Consider the following greedy algorithm. Give the shortest skier the shortest ski, give the second shortest skier the second shortest ski, give the third shortest skier the third shortest ski, etc.Prove of disprove that this algorithm is correct. HINT: One of the above greedy algorithms is correct and one is incorrect for the other. The proof of correctness must be done…arrow_forwardFor the problem below, two ”high level” greedy strategy is given. One of the strategies givesa correct solutions, and the other sometimes gives an incorrect solutions. Decide which greedy strategyproduces optimal solutions, and give a proof that it is correct and a counter-example showing the otherstrategy is incorrect.( show proof and counter example) You are given a list of classes C and a list of classrooms R. Each class c has a positive enrollment E(c)and each room r has a positive integer size S(r) which denotes its capacity. You want to assign eachclass a room in a way that minimizes the total sizes of rooms used. However, the capacity of the roomassigned to a class must be at least the enrollment of the class. You cannot assign two classes to thesame room. Greedy strategy A: Repeat until all classes are assigned, or a class cannot be included in any unassignedroom. Assign the largest unassigned class to the smallest unassigned room larger than or equal to itsenrollment. Greedy…arrow_forwardFind the path from S to G using A* algorithm. Is it optimal ?arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
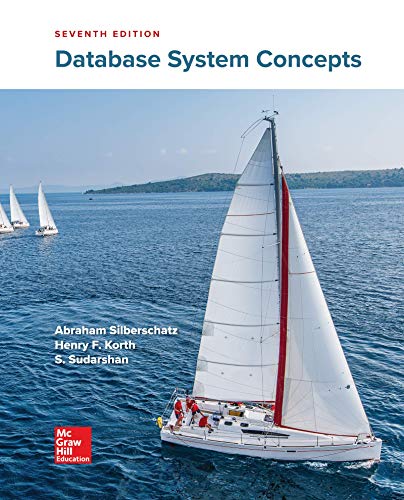
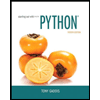
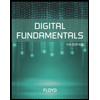
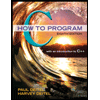
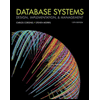
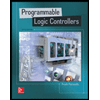