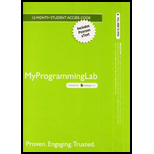
MyProgrammingLab - For Gaddis: Starting Out with C++ From Control Structures through Objects
15th Edition
ISBN: 9780133780611
Author: Pearson
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 17, Problem 13PC
Program Plan Intro
Rainfall Statistics Modification #2
Program Plan:
“IntList.h”:
- Include the required specifications into the program.
- Define a class template named “IntList”.
- Declare the member variables “value” and “*next” in structure named “ListNode”.
- Declare the constructor, copy constructor, destructor, and member functions in the class.
- Declare a class template and define a function named “appendNode()” to insert the node at end of the list.
- Declare the structure pointer variables “newNode” and “dataPtr” for the structure named “ListNode”.
- Assign the value “num” to the variable “newNode” and assign null to the variable “newNode”.
- Using “if…else” condition check whether the list to be empty or not, if the “head” is empty and make a new node into “head” pointer. Otherwise, make a loop find last node in the loop.
- Assign the value of “dataPtr” into the variable “newNode”.
- Declare a class template and define a function named “print()” to print the values in the list.
- Declare the structure pointer “dataPtr” for the structure named “ListNode”.
- Initialize the variable “dataPtr” with the “head” pointer.
- Make a loop “while” to display the values of the list.
- Declare a class template and define a function named “insertNode()” used to insert a value into the list.
- Declare the structure pointer variables “newNode”, “dataPtr”, and “prev” for the structure named “ListNode”.
- Make a “newNode” value into the received variable value “num”.
- Using “if…else” condition to check whether the list is empty or not.
- If the list is empty then initialize “head” pointer with the value of “newNode” variable.
- Otherwise, make a “while” loop to test whether the “num” value is less than the list values or not.
- Use “if…else” condition to initialize the value into list.
- Declare a class template and define a function named “deleteNode()”to delete a value from the list.
- Declare the pointer variables “dataPtr”, and “prev” for the structure named “ListNode”.
- Using “if…else” condition to check whether the “head” value is equal to “num” or not.
- Initialize the variable “dataPtr” with the value of the variable “head”.
- Remove the value using “delete” operator and reassign the “head” value into the “dataPtr”.
- If the “num” value not equal to the “head” value, then define the “while” loop to assign the “dataPtr” into “prev”.
- Use “if” condition to delete the “prev” pointer.
- Declare a class template and define a function named “getTotal()” to calculate total value in a list.
- Define a variable named “total” and initialize it to “0” in type of template.
- Define a pointer variable “nodePtr” for the structure “ListNode” and initialize it to be “NULL”.
- Assign the value of “head” pointer into “nodePtr”.
- Define a “while” loop to calculate “total” value of the list.
- Return a value of “total” to the called function.
- Declare a class template and define a function named “numNodes()” to find the number of values that are presented in the list.
- Declare a variable named “count” in type of “integer”.
- Define a pointer variable “nodePtr” and initialize it to be “NULL”.
- Assign a pointer variable “head” to the “nodePtr”.
- Define a “while” loop to traverse and count the number of elements in the list.
- Declare a class template and define a function named “getAverage()”to find an average value of elements that are presented in list.
- Declare a class template and define a function named “getLargest()”to find largest element in the list.
- Declare a template variable “largest” and pointer variable “nodePtr” for the structure.
- Using “if” condition, assign the value of “head” into “largest” variable.
- Using “while” loop, traverse the list until list will be empty.
- Using “if” condition, check whether the value of “nodePtr” is greater than the value of “largest” or not.
- Assign address of “nodePtr” into “nodePtr”.
- Return a value of “largest” variable to the called function.
- Declare a class template and define a function named “getSmallest()” to find smallest element in the list.
- Declare a template variable “smallest” and pointer variable “nodePtr” for the structure.
- Using “if” condition, assign the value of “head” into “smallest” variable.
- Using “while” loop, traverse the list until list will be empty.
- Using “if” condition, check the value of “nodePtr” is smaller than the value of “smallest”.
- Assign address of “nodePtr” into “nodePtr”.
- Return a value of “smallest” variable to the called function.
- Declare a class template and define a function named “getSmallestPosition()” to find the position of smallest value in the list.
- Declare a template variable “smallest” and pointer variable “nodePtr” for the structure.
- Using “while” loop traverses the list until the list will be empty.
- Using “if” condition, find the position of “smallest” value in the list.
- Return the value of “position” to the called function.
- Declare a class template and define a function named “getLargestPosition()” to find the position of largest value in the list.
- Declare a template variable “largest” and pointer variable “nodePtr” for the structure.
- Using “while” loop traverses the list until the list will be empty.
- Using “if” condition, find the position of “largest” value in the list.
- Return the value of “position” to the called function.
- Define the destructor to destroy the values in the list.
- Declare the structure pointer variables “dataPtr”, and “nextNode” for the structure named “ListNode”.
- Initialize the “head” value into the “dataPtr”.
- Define a “while” loop to make the links of node into “nextNode” and remove the node using “delete” operator.
- Declare a class template and define a function named “storeToFile()” to save the list values into file.
- Declare a pointer variable “nodePtr” for a structure “ListNode” and assign it’s to be null.
- Make a call to create a file object “outFile()” and initialize the value of variable “head” pointer into “nodePtr”.
- Using “while” loop save the values of list into file and assign the next node into “nodePtr”.
- Close the file using “close()” method.
- Declare a class template and define a function named “getFromFile()” to get the data from file into list.
- Declare a pointer variable “nodePtr” for a structure “ListNode” and assign it’s to be null.
- Declare a template variable “newValue” and create an object for file.
- Using “while” loop, get the values from file into list.
- Close the file using “close()” method.
Program #1 “Main.cpp”:
- Include the required header files into the program.
- Declare a variable “n” in type of integer.
- Read the value of “n” from user and using “while” loop to validate the data entered by user.
- Declare an object named “rainfall” for the class “IntList”.
- Using “for” loop, read an input for every month from user.
- Append the value entered from user into the list.
- Make a call to the function “storeToFile()” to write the list values into file.
Program #2 “Main.cpp”:
- Include the required header files into the program.
- Declare an object named “rainfall” for the class “IntList”.
- Make a call to the function “getFromFile()” to read a file into list using “rainfall” object.
- Make a call to “getTotal()”, “getAverage()”, “getLargest()”, “getSmallest()”, “getLargestPosition()”, and “getSmallestPosition()” function and display the values on the screen.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Computer Science
In c++ (There should be three different files, "main.cpp", "sourcefile.cpp" and "header.cpp" your solution must have those three different files):
Write a function that checks if the nodes in a linked list is sorted in ascending order. The function should return true if the values are sorted, otherwise false. Take user input. Call the funciton in the main function to show it is working.
Topic: Singly Linked ListImplement the following functions in C++ program. Read the question carefully. (See attached photo for reference)
void isEmpty()
This method will return true if the linked list is empty, otherwise return false.
void clear()
This method will empty your linked list. Effectively, this should and already has been called in your destructor (i.e., the ~LinkedList() method) so that it will deallocate the nodes created first before deallocating the linked list itself.
Functions that look at a linked list but do not modify it are referred to as______________
Chapter 17 Solutions
MyProgrammingLab - For Gaddis: Starting Out with C++ From Control Structures through Objects
Ch. 17.1 - Prob. 17.1CPCh. 17.1 - Prob. 17.2CPCh. 17.1 - Prob. 17.3CPCh. 17.1 - Prob. 17.4CPCh. 17.2 - Prob. 17.5CPCh. 17.2 - Prob. 17.6CPCh. 17.2 - Prob. 17.7CPCh. 17.2 - Prob. 17.8CPCh. 17.2 - Prob. 17.9CPCh. 17.2 - Prob. 17.10CP
Ch. 17 - Prob. 1RQECh. 17 - Prob. 2RQECh. 17 - Prob. 3RQECh. 17 - Prob. 4RQECh. 17 - Prob. 5RQECh. 17 - Prob. 6RQECh. 17 - Prob. 7RQECh. 17 - Prob. 8RQECh. 17 - Prob. 9RQECh. 17 - Prob. 10RQECh. 17 - Prob. 11RQECh. 17 - Prob. 13RQECh. 17 - Prob. 14RQECh. 17 - Prob. 15RQECh. 17 - Prob. 16RQECh. 17 - Prob. 17RQECh. 17 - Prob. 18RQECh. 17 - Prob. 19RQECh. 17 - Prob. 20RQECh. 17 - Prob. 21RQECh. 17 - Prob. 22RQECh. 17 - Prob. 23RQECh. 17 - Prob. 24RQECh. 17 - Prob. 25RQECh. 17 - T F The programmer must know in advance how many...Ch. 17 - T F It is not necessary for each node in a linked...Ch. 17 - Prob. 28RQECh. 17 - Prob. 29RQECh. 17 - Prob. 30RQECh. 17 - Prob. 31RQECh. 17 - Prob. 32RQECh. 17 - Prob. 33RQECh. 17 - Prob. 34RQECh. 17 - Prob. 35RQECh. 17 - Prob. 1PCCh. 17 - Prob. 2PCCh. 17 - Prob. 3PCCh. 17 - Prob. 4PCCh. 17 - Prob. 5PCCh. 17 - Prob. 6PCCh. 17 - Prob. 7PCCh. 17 - List Template Create a list class template based...Ch. 17 - Prob. 9PCCh. 17 - Prob. 10PCCh. 17 - Prob. 11PCCh. 17 - Prob. 12PCCh. 17 - Prob. 13PCCh. 17 - Prob. 14PCCh. 17 - Prob. 15PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Question: struct node { char *name; int marks; node *next; }; By using the above declaration for the Linked List, write a program in C++ to display the summary report regarding the pass/fail ratio of the subject Data Structure for the class BSI-3 that have only 15 students. The required Report Summary for the exam will be like wise: The No. of A grades........??? The No. of B grades........??? And so on by applying the grading criteria of COMSATS-University Islamabad. Question: struct node { char *name; int marks; node *next; }; By using the above declaration for the Linked List, write a program in C++ to display the summary report regarding the pass/fail ratio of the subject Data Structure for the class BSI-3 that have only 15 students. The required Report Summary for the exam will be like wise: The No. of A grades........??? The No. of B grades........??? And so on by applying the grading criteria of COMSATS-University Islamabad.arrow_forwardCreate two linked lists and then make a concatList(NODE **list1, NODE **list2) function that concatenates the two lists. The concatenated list must be a linked list. Fill in the "Write code here" sections appropriately.arrow_forwardComplete the method “readdata”. In this method you are to read in at least 10 sets of student data into a linked list. The data to read in is: student id number, name, major (CIS or Math) and student GPA. You will enter data of your choice. You will need a loop to continue entering data until the user wishes to stop. Complete the method “printdata”. In this method, you are to print all of the data that was entered into the linked list in the method readdata. Complete the method “printstats”. In this method, you are to search the linked list and print the following: List of student’s id and names who are CIS majors List of student’s id and names who are Math majors List of student’s names along with their gpa who are honor students (gpa 3.5 or greater) All information for the CIS student with the highest gpa (you may assume that different gpa values have been entered for all students) CODE (student_list.java) You MUST use this code: package student_list;import…arrow_forward
- Create class Test in a file named Test.java. This class contains a main program that performs the following actions: Instantiate a doubly linked list. Insert strings “a”, “b”, and “c” at the head of the list using three Insert() operations. The state of the list is now [“c”, “b”, “a”]. Set the current element to the second-to-last element with a call to Tail() followed by a call to Previous()Then insert string “d”. The state of the list is now [“c”, “d”, “b”, “a”]. Set the current element to past-the-end with a call to Tail() followed by a call to Next(). Then insert string “e”. The state of the list is now [“c”, “d”, “b”, “a”, “e”] . Print the list with a call to Print() and verify that the state of the list is correct.arrow_forwardCell Phones Records In this part, you are required to write a program, using linked lists, that manipulates a set of records of cell phones and performs some operations on these records. I) The CellPhone class has the following attributes: a serialNum (long type), a brand (String type), a year (int type, which indicates manufacturing year) and a price (double type). It is assumed that brand name is always recorded as a single word (i.e. Motorola, SonyEricsson, Panasonic, etc.). It is also assumed that all cellular phones follow one system of assigning serial numbers, regardless of their different brands, so no two cell phones may have the same serial number. You are required to write the implementation of the CellPhone class. Beside the usual mutator and accessor methods (i.e. getPrice(), setYear()) the class must have the following: (a) Parameterized constructor that accepts four values and initializes serialNum, brand, year and price to these passed values; (b) Copy constructor,…arrow_forwardGiven the declarations (C++)struct ListNode{ float volume;ListNode* link;};ListNode* headPtr;ListNode* currPtr;assume that headPtr and currPtr point to a linked list of many nodes. Write the statements that remove the second node.arrow_forward
- detemine if the following statement are true or false The iterator operation is required by the Iterable interface. A list allows retrieval of information based on the contents of the information.arrow_forwardGrocery shopping list (linked list: inserting at the end of a list) Given main() in the ShoppingList class, define an insertAtEnd() method in the ItemNode class that adds an element to the end of a linked list. DO NOT print the dummy head node. Ex. if the input is: 4 Kale Lettuce Carrots Peanuts where 4 is the number of items to be inserted; Kale, Lettuce, Carrots, Peanuts are the names of the items to be added at the end of the list. The output is: Kale Lettuce Carrots Peanutsarrow_forwardLinked list is more suitable data structure than array for a program that requires a lot of insert/erase operations.True or False.arrow_forward
- struct node{ int a; struct node * nextptr; }; Write two functions. One for inserting new values to a link list that uses the given node structure. void insert(struct node **head, int value); Second function is called to count the number of even numbers in the link list. It returns an integer that represents the number of even numbers. int countEvenNumbers(struct node *head); Write a C program that reads a number of integers from the user and insert those integers into a link list (use insert function). Later pass the head pointer of this link list to a function called countEvenNumbers. This function counts and returns the number of even numbers in the list. The returned value will be printed on the screen. Note 1: Do not modify the function prototypes. Sample Input1: Sample Output1: 45 23 44 12 37 98 33 35 -1 3 Sample Input2: Sample Output2: 11 33 44 21 22 99 123 122 124 77 -1 4arrow_forwardUsing the following struct: typedef char DATA; struct node{ DATA d; struct node *next; }; Write a program in C to build a single linked list using a loop. Your list should be built in such a way that the new item is added at the beginning of the list. A good solution with comments on the code is appreciatedarrow_forwardCapital QuizWrite a program that creates a dictionary containing the U.S. states as keys, and their capitals as values. (Use the Internet to get a list of the states and their capitals.) The programshould then randomly quiz the user by displaying the name of a state and asking the userto enter that state’s capital. The program should keep a count of the number of correct andincorrect responses. (As an alternative to the U.S. states, the program can use the names ofcountries and their capitals.) in phytonarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
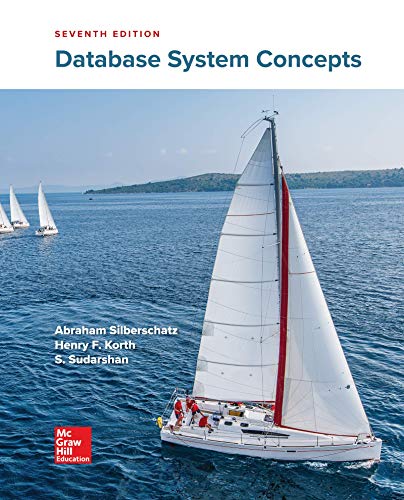
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
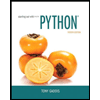
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
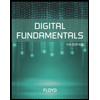
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
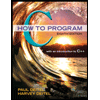
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
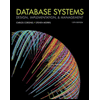
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
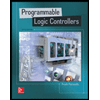
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education