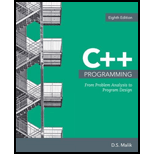
a.
Every node in a linked list has two components: one to store the relevant information and one to store the address. Hence, the given statement is “True”.
A linked list is a collection of components called nodes. Every node except the last node contains the address of the next node and it has two components: one to store the relevant information or data and one to store the address, called the link, of the next node in the list. The address of the first node in the list is stored in a separate location called the head or first.
a.
Every node in a linked list has two components: one to store the relevant information and one to store the address. Hence, the given statement is “True”.

Every node in a linked list has two components: one to store the relevant information and one to store the address. Hence, the given statement is “True”.
Explanation of Solution
Every node in a linked list has two components: one to store the relevant information and one to store the address.
b.
In a linked list, the order of the elements is not determined by the order in which the nodes were created to store the elements. Hence, the given statement is “False”.
A linked list is a collection of components called nodes. Every node except the last node contains the address of the next node and it has two components: one to store the relevant information or data and one to store the address, called the link, of the next node in the list. The address of the first node in the list is stored in a separate location called the head or first.
b.
In a linked list, the order of the elements is not determined by the order in which the nodes were created to store the elements. Hence, the given statement is “False”.

In a linked list, the order of the elements is not determined by the order in which the nodes were created to store the elements. Hence, the given statement is “False”.
Explanation of Solution
In a linked list, the order of the elements is not determined by the order in which the nodes were created to store the elements but by the order of the pointers or links in the list.
c.
In a linked list, memory allocated for the nodes may not necessarily be sequential. Hence, the given statement is “False”.
A linked list is a collection of components called nodes. Every node except the last node contains the address of the next node and it has two components: one to store the relevant information or data and one to store the address, called the link, of the next node in the list. The address of the first node in the list is stored in a separate location called the head or first.
c.
In a linked list, memory allocated for the nodes may not necessarily be sequential. Hence, the given statement is “False”.

In a linked list, memory allocated for the nodes may not necessarily be sequential. Hence, the given statement is “False”.
Explanation of Solution
In a linked list, memory allocated for the nodes may not necessarily be sequential, as memory is dynamically allocated during the node creation using pointer
d.
In a linked list, the link field of the last node does not point to itself. Hence, the given statement is “False”.
A linked list is a collection of components called nodes. Every node except the last node contains the address of the next node and it has two components: one to store the relevant information or data and one to store the address, called the link, of the next node in the list. The address of the first node in the list is stored in a separate location called the head or first.
d.
In a linked list, the link field of the last node does not point to itself. Hence, the given statement is “False”.

In a linked list, the link field of the last node does not point to itself. Hence, the given statement is “False”.
Explanation of Solution
In a linked list, the link field of the last node does not point to itself but to a null pointer (nullptr).
e.
Suppose the nodes of a linked list are in the usual info-link form and current points to a node of the linked list. Then current = current.link; does not advance current to the next node in the linked list. Hence, the given statement is “False”.
A linked list is a collection of components called nodes. Every node except the last node contains the address of the next node and it has two components: one to store the relevant information or data and one to store the address, called the link, of the next node in the list. The address of the first node in the list is stored in a separate location called the head or first.
e.
Suppose the nodes of a linked list are in the usual info-link form and current points to a node of the linked list. Then current = current.link; does not advance current to the next node in the linked list. Hence, the given statement is “False”.

Suppose the nodes of a linked list are in the usual info-link form and current points to a node of the linked list. Then current = current.link; does not advance current to the next node in the linked list. Hence, the given statement is “False”.
Explanation of Solution
Suppose the nodes of a linked list are in the usual info-link form and current points to a node of the linked list. Then current = current->link; advances current to the next node in the linked list. Since link and current both are pointers the member of the structure pointed to by the pointer variable current is accessed using the arrow notation -> and not the dot operator.
f.
To insert a new item in a linked list, create a node, store the new item in the node, and insert the node in the linked list..Hence, the given statement is “True”.
While building a linked list initially the list is empty, and the pointerfirst must be initialized to nullptr. The steps of insertion are -firstly, initialize first to nullptr. Then for each item in the list - create the new node, newNode, store the item in newNode, insert newNode before first and update the value of the pointer first.
f.
To insert a new item in a linked list, create a node, store the new item in the node, and insert the node in the linked list..Hence, the given statement is “True”.

To insert a new item in a linked list, create a node, store the new item in the node, and insert the node in the linked list..Hence, the given statement is “True”.
Explanation of Solution
To insert a new item in a linked list, create a node, store the new item in the node, and insert the node in the linked list.
g.
To build a linked list forward, the new node is inserted at the end of the list. Hence, the given statement is “True”.
When building a linked list forward, a new node is always inserted at the end of the linked list, while building backwards, new node is always inserted at the beginning of the list.
g.
To build a linked list forward, the new node is inserted at the end of the list. Hence, the given statement is “True”.

To build a linked list forward, the new node is inserted at the end of the list. Hence, the given statement is “True”.
Explanation of Solution
When building a linked list forward, a new node is always inserted at the end of the linked list, while building backwards, new node is always inserted at the beginning of the list.
h.
A single linked list cannot be traversed in either direction. Hence, the given statement is “False”.
A single linked list cannot be traversed in either direction but only in forward direction.
h.
A single linked list cannot be traversed in either direction. Hence, the given statement is “False”.

A single linked list cannot be traversed in either direction. Hence, the given statement is “False”.
Explanation of Solution
A single linked list cannot be traversed in either direction but only in forward direction. A doubly linked list can be traversed in either direction as it has links pointing in either direction stored at each node.
i.
In a linked list, nodes are not always inserted either at the beginning or at the end because a linked list is a random-access data structure. Hence, the given statement is “False”.
A linked list is a collection of components called nodes. Every node except the last node contains the address of the next node and it has two components: one to store the relevant information or data and one to store the address, called the link, of the next node in the list. The address of the first node in the list is stored in a separate location called the head or first.
i.
In a linked list, nodes are not always inserted either at the beginning or at the end because a linked list is a random-access data structure. Hence, the given statement is “False”.

In a linked list, nodes are not always inserted either at the beginning or at the end because a linked list is a random-access data structure. Hence, the given statement is “False”.
Explanation of Solution
In a linked list, nodes are not always inserted either at the beginning or at the end because a linked list is a random-access data structure. Hence nodes can be inserted anywhere in the linked list.
j.
The head pointer of a linked list should not be used to traverse the list. Hence, the given statement is “True”.
A linked list is a collection of components called nodes. Every node except the last node contains the address of the next node and it has two components: one to store the relevant information or data and one to store the address, called the link, of the next node in the list. The address of the first node in the list is stored in a separate location called the head or first.
j.
The head pointer of a linked list should not be used to traverse the list. Hence, the given statement is “True”.

The head pointer of a linked list should not be used to traverse the list. Hence, the given statement is “True”.
Explanation of Solution
The head pointer of a linked list should not be used to traverse the list. Because if that is done we will lose the rest of the linked list which has already been traversed. Linked list is a dynamic data structure and the nodes are not stored in contiguous memory locations.
k.
The two most common operations on iterators are ++ and * (the dereferencing operator). Hence, the given statement is “True”.
The two most common operations on iterators are ++ (the increment operator) and * (the dereferencing operator). The increment operator advances the iterator to the next node in the list, and the dereferencing operator returns the info of the current node.
k.
The two most common operations on iterators are ++ and * (the dereferencing operator). Hence, the given statement is “True”.

The two most common operations on iterators are ++ and * (the dereferencing operator). Hence, the given statement is “True”.
Explanation of Solution
The two most common operations on iterators are ++ (the increment operator) and * (the dereferencing operator). The increment operator advances the iterator to the next node in the list, and the dereferencing operator returns the info of the current node.
l.
The function initializeList of the class linkedListType initializes the list not only by setting the pointers first and last to nullptr, but also the count variable to 0. Hence, the given statement is “False”.
The function initializeList of the class linkedListType initializes the list not only by setting the pointers first and last to nullptr, but also the count variable to 0.
l.
The function initializeList of the class linkedListType initializes the list not only by setting the pointers first and last to nullptr, but also the count variable to 0. Hence, the given statement is “False”.

The function initializeList of the class linkedListType initializes the list not only by setting the pointers first and last to nullptr, but also the count variable to 0. Hence, the given statement is “False”.
Explanation of Solution
The function initializeList of the class linkedListType initializes the list not only by setting the pointers first and last to nullptr, but also the count variable to 0.
m.
The function search of the class unorderedLinkedList searches the entire linked list sequentially until the item is found, while the function search of the class ordered-LinkedList searches the list using a mechanism which is not exactly binary search algorithm. Hence, the given statement is“False”.
In case of ordered-LinkedList because the list is sorted, we start the search at the first node in the list and stop the search as soon as we find a node in the list with info greater than or equal to the search item or when we have searched the entire list.
m.
The function search of the class unorderedLinkedList searches the entire linked list sequentially until the item is found, while the function search of the class ordered-LinkedList searches the list using a mechanism which is not exactly binary search algorithm. Hence, the given statement is“False”.

The function search of the class unorderedLinkedList searches the entire linked list sequentially until the item is found, while the function search of the class ordered-LinkedList searches the list using a mechanism which is not exactly binary search
Explanation of Solution
In case of ordered-LinkedList because the list is sorted, we start the search at the first node in the list and stop the search as soon as we find a node in the list with info greater than or equal to the search item or when we have searched the entire list. This is not the binary search algorithm.
n.
A doubly linked list can be traversed in either direction. Hence, the given statement is “True”.
A single linked list cannot be traversed in either direction but only in forward direction. A doubly linked list can be traversed in either direction as it has links pointing in either direction stored at each node.
n.
A doubly linked list can be traversed in either direction. Hence, the given statement is “True”.

A doubly linked list can be traversed in either direction. Hence, the given statement is “True”.
Explanation of Solution
A single linked list cannot be traversed in either direction but only in forward direction. A doubly linked list can be traversed in either direction as it has links pointing in either direction stored at each node.
Want to see more full solutions like this?
Chapter 17 Solutions
C++ Programming: From Problem Analysis to Program Design
- How is an array stored in main memory? How is a linked list stored in main memory? What are their comparative advantages and disadvantages? Give examples of data that would be best stored as an array and as a linked list.arrow_forwardConsider the following linked list: Give the correct sequence to steps to the list be like below: I Move current pointer to the position to delete i.e. node D, II Move the previous pointer behind the current pointer i.e. node C III Make the node C to point to next of node D. IV Delete the node D Select one: A.II, I, IV, III B.I, II, III, IV C.IV, I, II, III D.IV, III, II, Iarrow_forwardConsider the following linked list: Give the correct sequence to steps to the list be like below: I Move pointer to position to insert i.e. node K II Point the new node to next of node K III Point the node K to the new node. IV Create new node S Select one: A.IV, I, II, III B.IV, I, III, II C.IV, III, II, I D.I, II, III, IVarrow_forward
- Answer the following in your own words: Your friend says they implemented a stack as a linked list with reference pointers to both a head and the tail node (i.e. they implemented a stack as a double ended linked list). They said this significantly improved the performance for all functions (push, pop, search, and update). Are they correct? Why or why not? Another friend implemented a queue as a linked list with only a head pointer (no tail node). She said this made performance of all functions (insert, remove, search, and update) categorically equal to a queue as a linked list with a head and a tail node. Is she correct? Why or why not?arrow_forwardCourse: Data Structures Topic: Linked List Question: Suppose that you implement both queue and stack data structures using a linked list.considering both the memory and computation efficieny,What type of linked list(e.g. single-ended singly linked list,double-ended singly linked list,double-ended doubly linked list etc). will be the best, that can be used to implement both data structures sfficiently?.justify your answer.arrow_forwardwrite a c program to remove node after current node in a single link list. travesing is not allowed in single link list. assume you have access only current node. first you design structure of link listarrow_forward
- Write a program in C to insert or delete characters in a buffer. You need to use stack operations to maintain operations for a buffer in a text editor. The program implements the following operations using two stacks that are represented using linked list: Create an empty buffer createBuffer() Insert a character at the cursor position insert() Get the character at the cursor position char get() Delete and return the character at the cursor char delete() Move the cursor k position to the left void left (int k) Move the cursor k positions to the right void right(int k) Requirements: You need to implement the Stack ADT using either Array or Linked list implementation for the following operations in a file named “stack.h”: Stack initialize() boolean IsEmpty (Stack) character pop (Stack) Stack push(Stack and character) character top (Stack) size(Stack) The driver program interface can be either menu-based driven application that displays…arrow_forwardIN C LANGUAGE When inserting a node into a linked list, where (in memory) will that node be placed if there is no overflow? A) The location of the AVAIL pointer B) The next contiguous spot of memory from the last node C) The very end of the contiguous memory block D) A randomized memory locationarrow_forwardThe nodes of a linked list must have continguous memory addresses, like an array.A. True B. Falsearrow_forward
- Consider the following example of a programme that builds and manipulates a linked list:What are the two specific nodes that the programme would typically monitor? Describe two common uses of the null reference in the linked list node.arrow_forwardjava program: A linked queue is a single linked list in which: The first node of the linked list is both the front of the queue and the rear of the queue. The first node of the linked list is the rear of the queue and the last node of the linked list is the front of the queue. The first node of the linked list is the front of the queue and the last node of the linked list is the rear of the queue. The last node of the linked list is both the front of the queue and the rear of the queue.arrow_forwardComplete the following code. The goal is to implement the producer-consumer problem. You are expected to extend the provided C code to synchronize the thread operations consumer() and producer() such that an underflow and overflow of the queue is prevented. You are not allowed to change the code for implementing the queue operations, that is the code between lines 25 and 126 as shown in the Figure below. You must complete the missing parts between lines 226-261 as shown in the screenshot.arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
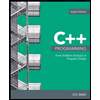
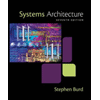