STARTING OUT WITH C++FROM CONTROL STRU
18th Edition
ISBN: 9781323815458
Author: GADDIS
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 17, Problem 5PC
Program Plan Intro
Text File Analysis
Program plan
source.cpp
- Include the required header files to the program.
- Define the main() function,
- Include the required function prototype to the program
- Declare the required variables.
- Create sets to hold each files words.
- Read the first file name from the user.
- Read the second file name from the user.
- Build the sets for two files.
- Display the unique words in both files using “showUnion()” function.
- Make a call to the function “showUnion()”.
- Display only the words that appear in both files using “ShowIntersection()” function.
- Make a call to the function “ShowIntersection ()”.
- Display the words that appear in file #1 but not in file #2 using “showDifference()” function.
- Make a call to the function “showDifference ()”.
- Display the words that appear in only one file, but not in both the files using “showSymDiff()”.
- Function definition for the “SetFile ()”:
- Declare a variable “in” to hold the input.
- Create an object for “ifstream”.
- Open the file using “open()”.
- Loop till “infile” reaches end of the file
- Insert the word to the “setWord”.
- Close the file using “close()”.
- Function definition for the “showUnion()” to display the union of words from two sets:
- Create a
vector to hold the union. - Get the union of the sets using the “begin()” and “end()”.
- Remove the unused words using “resize()” function.
- Display the result.
- Create a
- Function definition for the “showIntersection ()” to display the intersection of words from two sets:
- Create a vector to hold the intersection.
- Get the intersection of the sets using the “begin()” and “end()”.
- Remove the unused words using “resize()” function.
- Display the result.
- Function definition for the “showDifference ()” to show the difference of two sets:
- Create a vector to hold the difference of two sets.
- Get the union of the sets using the “begin()” and “end()”.
- Remove the unused words using “resize()” function.
- Display the result.
- Function definition for the “showSymDiff ()” to show the symmetric difference of two sets:
- Create a vector to hold the symmetric difference of two sets.
- Get the union of the sets using the “begin()” and “end()”.
- Remove the unused words using “resize()” function.
- Display the result.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Phyton:
Program 6:
Write a program that allows the user to add data from files or by hand. The user may add more data whenever they want. The print also allows the user to display the data and to print the statistics. The data is store in a list. It’s easier if you make the list global so that other functions can access it.
You should have the following functions:
· add_from_file() – prompts the user for a file and add the data from it.
· add_by_hand() – prompts the user to enter data until user type a negative number.
· print_stats() – print the stats (min, max, mean, sum). You may use the built-in functions.
Handling errors such as whether the input file exist is extra credit.
To print a 10-column table, you can use the code below:
for i in range(len(data)):
print(f"{data[i]:3}", end="")
if (i+1)%10==0:
print()
print()
Sample run:
Choose an action
1) add data from a file
2) add data by hand
3) print stats
4)…
C++:
You will create a header file with implementation that performs the task.
Together with the header and implementation, you create a test program whose main function demonstrates that your functions work as they should.
Two files, A and B which they are sorted. The files contain Numbers.
Based on the files, create a third file let calles it C . that file C is going to contain all the elements in sorted order from both files A and B.
This algorithm is called a merge..
Read Data
Write a function that reads in data from a file using the prototype below. The function can define and open the file, read the names and marks into the arrays and keep track of how many names there are in numElts, and then closes the file. The list of names and marks can be found at the end of the lab and copied into a text file.
void getNames(string names[], int marks[], int& numElts);
Display Data
Write a function to display the contents of names and marks using the prototype given below.
void displayData(const string names[], const int marks[], int numElts);
Linear Search
Write the searchList function given the prototype below so that it searches for a given name. The functions returns and int which is the index of the name found.
The main program will decide if -1 is returned then it will say name is not found otherwise it will write out the name and the mark for that name.
int linearSearch(const string list[], int numElts, string value);…
Chapter 17 Solutions
STARTING OUT WITH C++FROM CONTROL STRU
Ch. 17.2 - Prob. 17.1CPCh. 17.2 - Prob. 17.2CPCh. 17.2 - Prob. 17.3CPCh. 17.2 - Suppose you are writing a program that uses the...Ch. 17.2 - Prob. 17.5CPCh. 17.2 - Prob. 17.6CPCh. 17.2 - What does a containers begin() and end() member...Ch. 17.2 - Prob. 17.8CPCh. 17.2 - Prob. 17.9CPCh. 17.2 - Prob. 17.10CP
Ch. 17.3 - Write a statement that defines an empty vector...Ch. 17.3 - Prob. 17.12CPCh. 17.3 - Prob. 17.13CPCh. 17.3 - Write a statement that defines a vector object...Ch. 17.3 - What happens when you use an invalid index with...Ch. 17.3 - Prob. 17.16CPCh. 17.3 - If your program will be added a lot of objects to...Ch. 17.3 - Prob. 17.18CPCh. 17.3 - Prob. 17.19CPCh. 17.4 - Prob. 17.20CPCh. 17.4 - Write a statement that defines a nap named myMap....Ch. 17.4 - Prob. 17.22CPCh. 17.4 - Prob. 17.23CPCh. 17.4 - Prob. 17.24CPCh. 17.4 - Prob. 17.25CPCh. 17.4 - Prob. 17.26CPCh. 17.4 - Prob. 17.27CPCh. 17.5 - What are two differences between a set and a...Ch. 17.5 - Write a statement that defines an empty set object...Ch. 17.5 - Prob. 17.30CPCh. 17.5 - Prob. 17.31CPCh. 17.5 - Prob. 17.32CPCh. 17.5 - If you store objects of a class that you have...Ch. 17.5 - Prob. 17.34CPCh. 17.5 - Prob. 17.35CPCh. 17.6 - Prob. 17.36CPCh. 17.6 - What value will be stored in v[0] after the...Ch. 17.6 - Prob. 17.38CPCh. 17.6 - Prob. 17.39CPCh. 17.6 - Prob. 17.40CPCh. 17.6 - Prob. 17.41CPCh. 17.6 - Prob. 17.42CPCh. 17.7 - Prob. 17.43CPCh. 17.7 - Which operator must be overloaded in a class...Ch. 17.7 - Prob. 17.45CPCh. 17.7 - What is a predicate?Ch. 17.7 - Prob. 17.47CPCh. 17.7 - Prob. 17.48CPCh. 17.7 - Prob. 17.49CPCh. 17 - Prob. 1RQECh. 17 - Prob. 2RQECh. 17 - If you want to store objects of a class that you...Ch. 17 - If you want to store objects of a class that you...Ch. 17 - Prob. 5RQECh. 17 - Prob. 6RQECh. 17 - Prob. 7RQECh. 17 - If you want to store objects of a class that you...Ch. 17 - Prob. 9RQECh. 17 - Prob. 10RQECh. 17 - How does the behavior of the equal_range() member...Ch. 17 - Prob. 12RQECh. 17 - When using one of the STL algorithm function...Ch. 17 - You have written a class, and you plan to store...Ch. 17 - Prob. 15RQECh. 17 - Prob. 16RQECh. 17 - Prob. 17RQECh. 17 - Prob. 18RQECh. 17 - Prob. 19RQECh. 17 - Prob. 20RQECh. 17 - Prob. 21RQECh. 17 - A(n) ___________ container stores its data in a...Ch. 17 - _____________ are pointer-like objects used to...Ch. 17 - Prob. 24RQECh. 17 - Prob. 25RQECh. 17 - The _____ class is an associative container that...Ch. 17 - Prob. 27RQECh. 17 - Prob. 28RQECh. 17 - A _______ object is an object that can be called,...Ch. 17 - A _________ is a function or function object that...Ch. 17 - A ____________ is a predicate that takes one...Ch. 17 - A __________ is a predicate that takes two...Ch. 17 - A __________ is a compact way of creating a...Ch. 17 - T F The array class is a fixed-size container.Ch. 17 - T F The vector class is a fixed-size container.Ch. 17 - T F You use the operator to dereference an...Ch. 17 - T F You can use the ++ operator to increment an...Ch. 17 - Prob. 38RQECh. 17 - Prob. 39RQECh. 17 - T F You do not have to declare the size of a...Ch. 17 - T F A vector uses an array internally to store its...Ch. 17 - Prob. 42RQECh. 17 - T F You can store duplicate keys in a map...Ch. 17 - T F The multimap classs erase() member function...Ch. 17 - Prob. 45RQECh. 17 - Prob. 46RQECh. 17 - Prob. 47RQECh. 17 - Prob. 48RQECh. 17 - T F If two iterators denote a range of elements...Ch. 17 - T F You must sort a range of elements before...Ch. 17 - T F Any class that will be used to create function...Ch. 17 - T F Writing a lambda expression usually requires...Ch. 17 - T F You can assign a lambda expression to a...Ch. 17 - Prob. 54RQECh. 17 - Write a statement that defines an iterator that...Ch. 17 - Prob. 56RQECh. 17 - The following statement defines a vector of ints...Ch. 17 - Prob. 58RQECh. 17 - Prob. 59RQECh. 17 - The following code defines a vector and an...Ch. 17 - The following statement defines a vector of ints...Ch. 17 - Prob. 62RQECh. 17 - Prob. 63RQECh. 17 - Prob. 64RQECh. 17 - Look at the following vector definition: vectorint...Ch. 17 - Write a declaration for a class named Display. The...Ch. 17 - Write code that docs the following: Uses a lambda...Ch. 17 - // This code has an error. arrayint, 5 a; a[5] =...Ch. 17 - // This code has an error. vectorstring strv =...Ch. 17 - // This code has an error. vectorint numbers(10);...Ch. 17 - // This code has an error. vectorint numbers ={1,...Ch. 17 - Prob. 72RQECh. 17 - Prob. 73RQECh. 17 - // This code has an error. vectorint v = {6, 5, 4,...Ch. 17 - // This code has an error. auto sum = ()[int a,...Ch. 17 - Unique Words Write a program that opens a...Ch. 17 - Course Information Write a program that creates a...Ch. 17 - Prob. 3PCCh. 17 - File Encryption and Decryption Write a program...Ch. 17 - Prob. 5PCCh. 17 - Prob. 6PCCh. 17 - Prob. 7PCCh. 17 - Prob. 8PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Word IndexWrite a python program that reads the contents of a text file. The program should create a dictionary inwhich the key-value pairs are described as follows:Key. The keys are the individual words found in the file.576Values. Each value is a list that contains the line numbers in the file where the word (thekey) is found.For example, suppose the word “robot” is found in lines 7, 18, 94, and 138. The dictionarywould contain an element in which the key was the string “robot”, and the value was a listcontaining the numbers 7, 18, 94, and 138.Once the dictionary is built, the program should create another text file, known as a word index,listing the contents of the dictionary. The word index file should contain an alphabetical listingof the words that are stored as keys in the dictionary, along with the line numbers where thewords appear in the original file. Figure 9-1 shows an example of an original text file(Kennedy.txt) and its index file (index.txtarrow_forward9.12 LAB: File name change PYTHON A photographer is organizing a photo collection about the national parks in the US and would like to annotate the information about each of the photos into a separate set of files. Write a program that reads the name of a text file containing a list of photo file names. The program then reads the photo file names from the text file, replaces the "_photo.jpg" portion of the file names with "_info.txt", and outputs the modified file names. Assume the unchanged portion of the photo file names contains only letters and numbers, and the text file stores one photo file name per line. If the text file is empty, the program produces no output. Ex: If the input of the program is: ParkPhotos.txt and the contents of ParkPhotos.txt are: Acadia2003_photo.jpg AmericanSamoa1989_photo.jpg BlackCanyonoftheGunnison1983_photo.jpg CarlsbadCaverns2010_photo.jpg CraterLake1996_photo.jpg GrandCanyon1996_photo.jpg IndianaDunes1987_photo.jpg LakeClark2009_photo.jpg…arrow_forward4. WarningWrite a program that will read in a file of student academic credit data and create a list of students on academic warning. The list of students on warning will be written to a file. Each line of the input file will contain the student name (a single String with no spaces), the number of semester hours earned (an integer), the total quality points earned (a double). The following shows part of a typical data file:Smith 27 83.7Jones 21 28.35Walker 96 182.4Doe 60 150The program should compute the GPA (grade point or quality point average) for each student (the total quality points divided by the number of semester hours) then write the student information to the output file if that student should be put on academic warning. A student will be on warning if he/she has a GPA less than 1.5 for students with fewer than 30 semester hours credit, 1.75 for students with fewer than 60 semester hours credit, and 2.0 for all other students. The file Warning.java contains a skeleton…arrow_forward
- 12.9 LAB: Word frequencies (lists) Write a program that first reads in the name of an input file and then reads the file using the csv.reader() method. The file contains a list of words separated by commas. The program must output the words and their frequencies (the number of times each word appears in the file) without any duplicates. Ex: If the input is: input1.csv and the contents of input1.csv are: hello,cat,man,hey,dog,boy,Hello,man,cat,woman,dog,Cat,hey,boy the output is: hello - 1 cat - 2 man - 2 hey - 2 dog - 2 boy - 2 Hello - 1 woman - 1 Cat - 1 Notes: Output words in order of first occurrence in input and end output with a newline. File input1.csv is available to download.arrow_forward7.8 LAB: Word frequencies (lists) Write a program that first reads in the name of an input file and then reads the file using the csv.reader() method. The file contains a list of words separated by commas. Your program should output the words and their frequencies (the number of times each word appears in the file) without any duplicates. Ex: If the input is: input1.csv and the contents of input1.csv are: hello,cat,man,hey,dog,boy,Hello,man,cat,woman,dog,Cat,hey,boy the output is: hello 1 cat 2 man 2 hey 2 dog 2 boy 2 Hello 1 woman 1 Cat 1 Note: There is a newline at the end of the output, and input1.csv is available to download.arrow_forwardURGENT: Python Expense Management: This system will add, deduct, update, sort, and export expenses to a file. The program will initially load a collection of expenses from a .txt file and store them in a dictionary. All of the necessary functions and unit testing are provided below in expenses.py, expenses_test.py, and expenses.txt: expnses.py: def import_expenses(file): '''Reads data from a given file and stores the expenses in a dictionary, where the expense type is the key and the total expense amount for that expense is the value. The same expense type may appear multiple times in the file. Ignores expenses with missing or invalid amounts.''' # create empty dict expenses = {} #TODO insert your code return expenses def get_expense(expenses, expense_type): '''Returns the value for the given expense type in the given expenses dictionary. Prints a friendly message and returns None if the expense type doesn't exist.''' # TODO insert your code def add_expense(expenses,…arrow_forward
- C++: You will create a header file with implementation that performs the task. Together with the header and implementation, you create a test program whose main function demonstrates that your functions work as they should. Two files, A and which they are sorted. The files contain Numbers. Based on the files, create a third file let calles it C . that file C is going to contain all the elements in sorted order from both files A and B. This operation is called a merge..arrow_forwardExercise 6. Create a dictionary from a Text File: When a program incorporates a large dictionary, the dictionary is usually created from a file, such as a text file by using dict function to convert a list into a dictionary. Whenever a word in the original sentence is not a key in the dictionary, the get method places the word itself into the translated sentence. The file “Textese.txt” contains a word and its translation into textese. Write a program to create a dictionary with the file, ask the user to enter a simple sentence then translate it into textese. Define two function createDictionary (filename) and translate (sentence, dictionary). Then define a main function to test your functionsarrow_forward(C Language) A photographer is organizing a photo collection about the national parks in the US and would like to annotate the information about each of the photos into a separate set of files. Write a program that reads the name of a text file containing a list of photo file names. The program then reads the photo file names from the text file, replaces the "_photo.jpg" portion of the file names with "_info.txt", and outputs the modified file names. Assume the unchanged portion of the photo file names contains only letters and numbers, and the text file stores one photo file name per line. If the text file is empty, the program produces no output. Assume also the maximum number of characters of all file names is 100.arrow_forward
- void Pokedex::wakeupProfessorJimi(std::string) − populates the dynamic array of Entries with data from a valid file path, the function should open the file and use the add (Pokemon*, int) function to insert a Pok ́emon into the correct position in the dynamic array of Entries. Once the position is determined, the Pok ́emon should be inserted into the corresponding position in the list of Pok ́emon belonging to the Entries object. i aready have a pokemon class with enum for types, move class and move class setup and working it's just this one part. here is the text file 1,Bulbasaur,45,49,[Grass,Poison],Fire,Water,[[tackle,40,40,100],[vine whip,45,45,100],[growl,10,10,100]] 2,Ivysaur,60,63,[Grass,Poison],Fire,Water,[[leech seed,45,45,90],[razor leaf,55,55,95],[growl,10,10,100]] 3,Venusaur,80,83,[Grass,Poison],Fire,Water,[[tackle,40,40,100],[leech seed,45,45,90],[razor leaf,55,55,95],[growl,10,10,100]] 4,Charmander,39,43,[Fire],Water,Fire,[[growl,10,10,100],[fire fang,65,65,95]]…arrow_forwardUnique WordsWrite a python program that opens a specified text file then displays a list of all the unique words foundin the file.Hint: Store each word as an element of a set.arrow_forwardwrite two functions: load_treasure_map(filename): Takes a string as input corresponding to a filename. Opens the treasure map at that filename and loads the treasure map into a list of lists, then returns said list of lists. You can assume that the file will exist. Note that the treasure map could have any number of rows and columns write_treasure_map(treasure_map, filename): Takes as inputs a list of lists corresponding to a treasure map and a string corresponding to a filename. Writes the treasure map to a file at the given filename, with a newline after each row of the map. Does not return anything.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
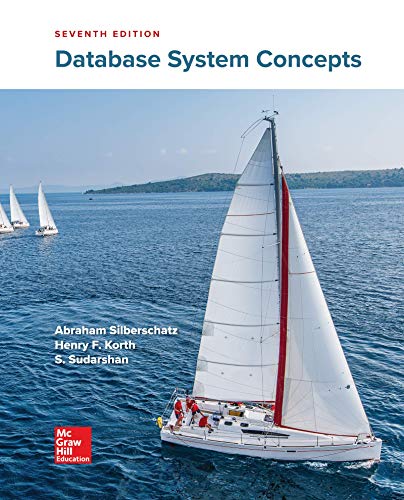
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
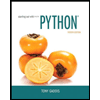
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
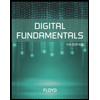
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
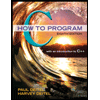
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
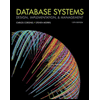
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
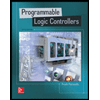
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education