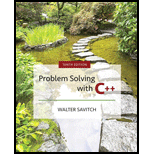
Problem Solving with C++ Plus MyLab Programming with Pearson eText -- Access Card Package (10th Edition)
10th Edition
ISBN: 9780134710747
Author: Walter Savitch
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 17, Problem 6PP
Program Plan Intro
Priority queue
Program plan:
- Define a file “Priority.h” to declare operation and functions.
- Include the directives for header file.
- Declare a “PriorityQueue” template class.
- Define a template class named “Node”.
- Inside the access specifier “public”, define a constructor of class “Node”.
- Declare a friend class “PriorityQueue”.
- Inside the access specifier “private”, create an object for template.
- Declare an integer variable “priority” and create a pointer variable “*next”.
- Define a template class named “PriorityQueue”.
- Inside the access specifier “public”, Declare a constructor of class “PriorityQueue”.
- Declare the “add()” function to add items in the queue.
- Declare the “remove()” function to remove items from the queue.
- Declare the “isEmpty()” Boolean function to return the status of queue.
- Inside the access specifier “public”, Declare a constructor of class “PriorityQueue”.
- Define a file “main().cpp” to call functions from “Priority.h” and perform all computations.
- Define a constructor of class “PriorityQueue()” to assign “null” to “head” of the queue.
- Define “add()” function to add new node onto the front of the queue.
- Define “remove()” function to remove the smallest priority from the queue.
- Define “isEmpty()” function to return “true” or “false” if the queue is empty.
- Define a “main()” function.
- Create an object for class “PriorityQueue”.
- Add and remove some items in the queue and print the result.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
The implementation for the priority queue in this chapter starts by modifying theHeap class so that the order of the elements can be determined by the programmer.Earlier the heap kept the highest value on the top of the container, but now it willbe altered to allow for either the highest or the lowest. The changes made to thisclass involve specifying another template parameter, which will be used for thecomparison operator. Altering the push() and pop() functions to make use of thecomparison operator instead of assuming that the largest value element will be ontop.
use c++ to code.
Given the linked list data structure, implement a sub-class TSortedList that makes sure that elements are inserted and maintained in an ascending order in the list. So, given the input sequence {1,7,3,11,5}, when printing the list after inserting the last element it should print like 1, 3, 5, 7, 11. Note that with inheritance, you have to only care about the insertion situation as deletion should still be handled by the parent class.
Implements clone which duplicates a list. Pay attention, because if there are sublists, they must be duplicated as well. Understand the implementation of the following function, which recursively displays the ids of each node in a list
def show_ids(M, level=0): k = M.first_node while k is not None: print(" "*2*level, id(k)) if (str(k.value.__class__.__name__) == str(M.__class__.__name__)): show_ids(k.value, level+1) k = k.next W=L2(Node(10, Node(L2(Node(14, Node(15, Node(L2(Node(16, Node(17))))))), Node(20, Node(30)))) )show_ids(W)
Develop your solution as follows:
- First copy the nodes of the current list (self)
- Create a new list with the copied nodes
- Loop through the nodes of the new list checking the value field
- If this field is also a list (use isinstance as in the show_ids function) then it calls clone on that list and substitutes the value.
Complete the code:
def L4(*args,**kwargs): class L4_class(L):…
Chapter 17 Solutions
Problem Solving with C++ Plus MyLab Programming with Pearson eText -- Access Card Package (10th Edition)
Ch. 17.1 - Write a function template named maximum. The...Ch. 17.1 - Prob. 2STECh. 17.1 - Define or characterize the template facility for...Ch. 17.1 - Prob. 4STECh. 17.1 - Display 7.10 shows a function called search, which...Ch. 17.1 - Prob. 6STECh. 17.2 - Give the definition for the member function...Ch. 17.2 - Give the definition for the constructor with zero...Ch. 17.2 - Give the definition of a template class called...Ch. 17.2 - Is the following true or false? Friends are used...
Ch. 17 - Write a function template for a function that has...Ch. 17 - Prob. 2PCh. 17 - Prob. 3PCh. 17 - Redo Programming Project 3 in Chapter 7, but this...Ch. 17 - Display 17.3 gives a template function for sorting...Ch. 17 - (This project requires that you know what a stack...Ch. 17 - Prob. 6PPCh. 17 - Prob. 7PPCh. 17 - This project requires that you complete...
Knowledge Booster
Similar questions
- Create a new Java class in a file named "ListPQ.java" that implements the Queue interface and uses the LinkedList provided by the JCF to implement a priority queue. Note that LinkedList does not automatically order values based on priority, so it will be up to you to make sure that values are removed in priority order. You should try to implement your priority queue as efficiently as possible - basic operations should run in constant or linear time. Your priority queue need only work with integers. Do not modify any of the provided code. //This is ArrayHeap (provided code) import java.util.Arrays; public class ArrayHeap implements Heap { private int[ ] array; private int size; public ArrayHeap() { array = new int[3]; size = 0; } @Override public void add(int value) { // add pt 1 if(size == array.length) { array = Arrays.copyOf(array, size*2); } array[size] = value; // sifting up int child = size; int parent = (child - 1) / 2; while(array[parent] > array[child]) { swap(parent,…arrow_forwardConsider the Person class in Problem 1. Implement the interface PersonPriorityQueueInterface provided in the assignment. In your implementation, you must use an instance of AList (which you used in Problem 1) to store the list of persons. We consider that a person whose age is higher than a second person also has a higher priority. Thus, the method peek(), for example, should return the person who is the oldest in the list. Your implementation should be O(n) for add, and O(1) for the remaining methods. Consider the Person class in Problem 1. Implement the interface PersonPriorityQueueInterface provided in the assignment. In your implementation, you must use an instance of AList (which you used in Problem 1) to store the list of persons. We consider that a person whose age is higher than a second person also has a higher priority. Thus, the method peek(), for example, should return the person who is the oldest in the list. Your implementation should be O(n) for add, and O(1)…arrow_forwardWrite a Java public static general method that doesn't belong to the Queue class(assuming the Queue type is Book and Book has a clone method, Book also has a method called getGenre() which returns the genre of the book): Queue findOneGenreBooks(Queue Q, String genreType) that searches in the first parameter Q for all books that has the same genre type as defined by the second parameter genreType, return all of the books that have the specified genre type as a Queue. (For example, you may want to find all cartoon books and return them as a Queue). Please note: You are only allowed to use the Queue class which has constructors, enqueue, dequeue, front, isEmpty, size, toString, and clone methods.arrow_forward
- You may find a doubly-linked list implementation below. Our first class is Node which we can make a new node with a given element. Its constructor also includes previous node reference prev and next node reference next. We have another class called DoublyLinkedList which has start_node attribute in its constructor as well as the methods such as: 1. insert_to_empty_list() 2. insert_to_end() 3. insert_at_index() Hints: Make a node object for the new element. Check if the index >= 0.If index is 0, make new node as head; else, make a temp node and iterate to the node previous to the index.If the previous node is not null, adjust the prev and next references. Print a message when the previous node is null. 4. delete_at_start() 5. delete_at_end() . 6. display() the task is to implement these 3 methods: insert_at_index(), delete_at_end(), display(). hint on how to start thee code # Initialize the Node class Node: def __init__(self, data): self.item = data…arrow_forwardJava: Write a method/programme that will take a generic linked list and make a palindrome of the list. In a seperate test programme the user can add any elements to the generic list and then this list must be made a palindrome. The output should be the original list with the palindrome list added to this list.arrow_forwardIn this project you will implement a Set class which represents a general collection of values. For this assignment, a set is generally defined as a list of values that is sorted and does not contain any duplicate values. More specifically, a set shall contain no pair of elements e1 and e2 such that e1.equals(e2) and no null elements. Requirements To ensure consistency among all implementations there are some requirements that all implementations must maintain. • Your implementation should reflect the definition of a set at all times. • For simplicity, your set will be used to store Integer objects. • An ArrayList object must be used to represent the set. • All methods that have an object parameter must be able to handle an input of null. • Methods such as Collections.sort that automatically sort a list may not be used. • The Set class shall reside in the default package. Recommendations There are deviations in program implementation that are acceptable and will not impact the overall…arrow_forward
- In this project you will implement a Set class which represents a general collection of values. For this assignment, a set is generally defined as a list of values that is sorted and does not contain any duplicate values. More specifically, a set shall contain no pair of elements e1 and e2 such that e1.equals(e2) and no null elements. Requirements To ensure consistency among all implementations there are some requirements that all implementations must maintain. • Your implementation should reflect the definition of a set at all times. • For simplicity, your set will be used to store Integer objects. • An ArrayList<Integer> object must be used to represent the set. • All methods that have an object parameter must be able to handle an input of null. • Methods such as Collections.sort that automatically sort a list may not be used. • The Set class shall reside in the default package. Recommendations There are deviations in program implementation that are acceptable and will not…arrow_forwardIn this project you will implement a Set class which represents a general collection of values. For this assignment, a set is generally defined as a list of values that is sorted and does not contain any duplicate values. More specifically, a set shall contain no pair of elements e1 and e2 such that e1.equals(e2) and no null elements. Requirements To ensure consistency among all implementations there are some requirements that all implementations must maintain. • Your implementation should reflect the definition of a set at all times. • For simplicity, your set will be used to store Integer objects. • An ArrayList<Integer> object must be used to represent the set. • All methods that have an object parameter must be able to handle an input of null. • Methods such as Collections.sort that automatically sort a list may not be used. • The Set class shall reside in the default package. Recommendations There are deviations in program implementation that are acceptable and will not…arrow_forwardWrite the definition of the function moveNthFront that takes as a parameter a positive integer, n. The function moves the nth element of the queue to the front. The order of the remaining elements remains unchanged. For example, suppose:queue = {5, 11, 34, 67, 43, 55} and n = 3.After a call to the function moveNthFront:queue = {34, 5, 11, 67, 43, 55}Add this function to the class queueType. Also, write a program to test your method.arrow_forward
- Create a class called ResizingArrayQueueOfStrings that implements the queue abstraction using a fixed-size array. Then, as an extension, utilise array resizing in your implementation to get rid of the size constraint.arrow_forwardWrite the definition of the function moveNthFront that takes as a parameter a positive integer, n. The function moves the nth clement of the queue to the front. The order of the remaining elements remains unchanged. For example, suppose queue = (5, 11, 34, 67, 43, 55) and n = 3. After a call to the function moveNthFront. queue = (34, 5, 11, 67, 43, 55). Add this function to the class queueType Also write a program to test your methodarrow_forwardAssume you have a class called Deck which represents a deck of cards using a singly linked lists. Each node is an object of type Card with a field called next where a reference to the next Card is stored. A field from the Deck class called head stores a reference to the card on the top of the deck, and a field called tail stores a reference to the last card, at the bottom of the deck. We can cut a deck of cards by performing what is called a triple cut: the deck is partitioned into three parts and the bottom part is positioned between the first two. You would like to write a method that implements such a cut on your deck data structure. The method will take as input two cards, the first one being the last card of the first part of the deck, and the second being the first one of the third part of the deck. For example, assume a deck contains cards in the following order AC 2C 3C 4C 5C AD 2D 3D 4D 5D After calling tripleCut() on the deck the card, with input a reference to 3C and a…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
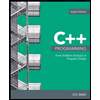
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning