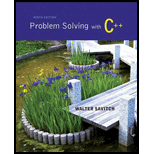
Problem Solving with C++ (9th Edition)
9th Edition
ISBN: 9780133591743
Author: Walter Savitch
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 17.1, Problem 2STE
Program Plan Intro
Function template:
In C++, a function template is referred as a “generic” function, which can work with different data types.
- While writing a function template, a programmer should use a “type parameter” to denote a “generic” data type instead of using the actual parameter.
- The compiler generates the code, when it encounters a function call to a function template. This code will handle the particular data type which is used in the function call.
- The compiler identifies the argument type and generates the code to work with those types.
- The generated code is referred as “template function”.
Example:
For example consider the following function template for finding a cube of value:
template <class T>
T cube(T x)
{
return x * x * x ;
}
- A function template must begin with the keyword “template” and it is followed by a pair of angle brackets, which contains one or more “generic” data types.
- A “generic” type must start with the keyword “class”, followed by an argument name which stands for the data type.
- The statement “T cube(T x)” is referred as function header, where “T” is a “type parameter”, “cube” is the function name and the variable “x” is declared for the type “T”.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Write a Racket function "combine" that takes two functions, f and g, as parameters and evaluates to a new function. Both f and g will be functions that take one parameter and evaluate to some result. The returned function should be the composition of the two functions with f applied first and g applied to f's result.
For example (combine add1 sub1) should evaluate to a function equivalent to (define (h x) (sub1 (add1 x))). You will need to use a lambda function to achieve your result. Essentially you want (combine f g) to evaluate to a lambda function with f and g embedded inside.
You can test your combine with invocations such as ((combine add1 add1) 1), which should evaluate to 3 since the anonymous function returned by combine is being applied to 1.
Note: This is an example of a "function closure". f and g are defined in the scope of combine, but the lambda embeds a copy of them in the lambda function that can be used outside of combine's scope. When creating a lambda function in…
Template functions are considered to be an efficient alternative of function overloading. Justify this statement by comparing the pros and cons of both with real-world examples.
Given the code segment below, what should be the data type of the formal parameter in the function prototype of func(), given the call from main()?
Note that function prototypes need not include the identifier (name of the parameter), so do NOT put any variable name in your answer. Remove any space in your answer. If the accessing is wrong, answer INVALID (in all capital letters).
void func( ______ );int main(){ double aData[6][4];func(&aData[4][1]);return 0;}
Chapter 17 Solutions
Problem Solving with C++ (9th Edition)
Ch. 17.1 - Write a function template named maximum. The...Ch. 17.1 - Prob. 2STECh. 17.1 - Define or characterize the template facility for...Ch. 17.1 - Prob. 4STECh. 17.1 - Display 7.10 shows a function called search, which...Ch. 17.1 - Prob. 6STECh. 17.2 - Give the definition for the member function...Ch. 17.2 - Give the definition for the constructor with zero...Ch. 17.2 - Give the definition of a template class called...Ch. 17.2 - Is the following true or false? Friends are used...
Ch. 17 - Write a function template for a function that has...Ch. 17 - Prob. 2PCh. 17 - Prob. 3PCh. 17 - Redo Programming Project 3 in Chapter 7, but this...Ch. 17 - Display 17.3 gives a template function for sorting...Ch. 17 - (This project requires that you know what a stack...Ch. 17 - Prob. 6PPCh. 17 - Prob. 7PPCh. 17 - This project requires that you complete...
Knowledge Booster
Similar questions
- Given the code segment below, what should be the data type of the formal parameter in the function prototype of func(), given the call from main()?Note that function prototypes need not include the identifier (name of the parameter), so do NOT put any variable name in your answer. Remove any space in your answer. If the accessing is wrong, answer INVALID (in all capital letters). void func( ______ );int main(){ double aData[6][4];func(&aData[4]);return 0;}arrow_forwardIn Kotlin, write a higher-order function with an expression body that takes an int n and a function f from int to int and returns the result of calling f(f(n)). For example if you call the function with n = -5 anda function that calculates absolute value, your function will return 5, and if you send the value 2 and a function that calculates the sqaure of an int, the function will return 16. in addition to the function, write the syntax to call it with:a. a function name as the function arguementb. some lambda expression as the function argumentarrow_forwardWrite a function numRolls that simulates the following dice game: a player repeatedly rolls a pair ofdice until the second time that doubles is rolled. The function then returns the total number of rollsmade. For example, suppose the player rolls the following pairs: (3,2), (3,3), (1,5), (5,5). The game thenstops because the player has rolled doubles twice, (3,3) and (5,5). The function returns 4, the totalnumber of rolls made: Use pythonarrow_forward
- Write the definition of a function named isSorted that receives three arguments: an array of int, an int that indicates the number of elements of interest in the array, and a bool.If the bool argument is true then the function returns true if and only if the array is sorted in ascending order. If the bool argument is false then the function returns true if and only if the array is sorted in descending order. In all other cases the function returns false.You may assume that the array has at least two elements.arrow_forwardInteresting, intersecting def squares_intersect(s1, s2): A square on the two-dimensional plane can be defined as a tuple (x, y, r) where (x, y) are the coordinates of its bottom left corner and r is the length of the side of the square. Given two squares as tuples (x1, y1, r1) and (x2, y2, r2), this function should determine whether these two squares intersect, that is, their areas have at least one point in common, even if that one point is merely the shared corner point when these two squares are placed kitty corner. This function should not contain any loops or list comprehensions of any kind, but should compute the result using only integer comparisons and conditional statements. This problem showcases an idea that comes up with some problems of this nature; it is actually far easier to determine that the two squares do not intersect, and negate that answer. Two squares do not intersect if one of them ends in the horizontal direction before the other one begins, or if the same…arrow_forwardGiven the Class Definition for ClockType discussed extensively in class, write what would have to be added to the IMPLEMENTATION FILE for the Class ClockType to overload the “= =”, i.e., the comparison “equal-equal sign,” here: That is, write the FULL FUNCTION DEFINITION for THE FUNCTION associated with Class ClockType to overload the “= =” remembering the private members are: b) int hr; // that contains the hours int min; // that contains the minutes int sec; // that contains the secondsarrow_forward
- Using C++ Using your own creativity, make a set of function templates that have these features: This function must return a value. A function template with 1 template parameter, T. And, any other parameters you want. and then another function template but this time with 2 template parameters, T1 and T2. And, any other parameters you want.arrow_forwardGive an example of how calling an inline function is different from calling a normal function in the background, and explain how the difference came to be. Also, give a specific example of how the change came about.arrow_forwardLet’s define a simple effect-free function that can be used like this (once the function is defined). yell("Yippee") res1: String = Yippee! val result = yell("Muhaha") result: String = Muhaha! val louder = yell(result) louder: String = Muhaha!! Here’s a template for the function definition. ??? ???(phrase: ???) = ??? + ??? Fill in the blanks: replace the question marks to define a yell function that can be used as in the REPL example. Each of the five blanks calls for a different code fragment. You can model your answer on the average function shown above. Enter the yell function’s full definition here. (That is, write the above line with the question marks replaced with proper code.)arrow_forward
- Can you write a C++ program for this: Create a class of function objects called StartsWith that satisfies the following specification: when initialized with character c, an object of this class behaves as a unary predicate that determines if its stringargument starts with c. For example, StartsWith(’a’) is a functionobject that can be used as a unary predicate to determine if a string startswith an a. So StartsWith(’a’)("alice") would return true butStartsWith(’a’)("bob") would return false. The function objectsshould return false when called on an empty string. Test your functionobjects by using one of them together with the STL algorithm count_ifto count the number of strings that start with some letter in some vectorof stringsarrow_forwardUsing C++ Using your own creativity, make a set of function templates that have these features: This function must return a value. A function template with 1 template parameter, T. And, any other parameters you want. A function template with 2 template parameters, T1 and T2. And, any other parameters you want. Using your own creativity, make a set of class templates that have these features: For this class template, put everything in one place--do not declare the member functions and have separate definition of the member functions elsewhere. Keep them in one place. Include a private variable. Include a constructor that loads the private variable when constructed. Include a destructor that clears the private variable to zero. Include set and get functions to set and get the private variable. For this class template, use declarations for variables and functions, like you do in header file (which you may use if you want). Then, separately put the full function definitions for…arrow_forwardGive an example of how the process of calling inline functions varies from the process of calling a standard function so that we may better understand the difference.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
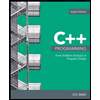
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
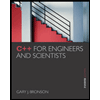
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr