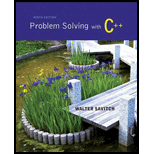
Problem Solving with C++ (9th Edition)
9th Edition
ISBN: 9780133591743
Author: Walter Savitch
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 17, Problem 7PP
Program Plan Intro
Creation of program to construct template class to implement a set of items
Program Plan:
- Define a class “Set” to define properties and methods.
- Define a constructor “Set()” to create instance.
- Define a destructor “~Set()” to destroy set.
- Define a method “operator=()” to define overloaded function.
- Define a method “add()” to add new items.
- Define a method “remove()” to remove item from set.
- Define a method “size()” to get size of set.
- Define a method “contains()” to check whether an element is in set.
- Define a method “to_array()” to copy values.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
in kotlin, Use this Employee class
data class Employee(val name: String, val wage: Double, val hours: Double)
and this list of Employees:
val l: List = listOf(Employee("Bob", 34.33, 42.0), Employee ("Cathy", 27.33, 15.25), Employee("Carlos", 46.99, 38.75), Employee("Tomas", 49.66,28.45), Employee("Xavier", 34.56, 11.5))
Write a function called raise which takes a list of Employees and a Double and returns a list of Employees like the original but with each having received a raise, with the Double representing the percentage raise. For example, if an employee's wage was $20 per hour and the Double is .15 (that is, 15%), her new wage will be $23 per hour.
Don't change the Employees; create and return a list of new Employee objects with the new wages. Use Kotlin's map() function.
Write a template-based class that implements a set of items. The class should allow the user to:
Add a new item to the set(addition of new item if already element then it will completely ignored)
Get the number of items in the set
Test your class by creating sets of different data types (e.g. integers, strings, etc.)
Please use the following class template to finish the program.
MAX 100
template<class T>
class Set
{
public:
Set();
void add(T newItem);// Add new item to the set (definition should be outside of the class)
int getSize();// Return size of set (definition should be outside of the class)
private:
int numOfelements;
T data[MAX];
};
Q# In Java, if you see a class name followed by “<T>”, as in List<T>, what does this mean?Group of answer choices
1.It is what is called a lambda-function.
2.It’s for making collections of objects of many types at the same time. For example, if I make List<T> myList = new List<T> I can put a String as one item, an int as another, and a Random as a third item.
3.You must specify an object type in place of the T; eg. List<Shape> will make a list of Shape objects.
4. It means that the object is of type Time.
Chapter 17 Solutions
Problem Solving with C++ (9th Edition)
Ch. 17.1 - Write a function template named maximum. The...Ch. 17.1 - Prob. 2STECh. 17.1 - Define or characterize the template facility for...Ch. 17.1 - Prob. 4STECh. 17.1 - Display 7.10 shows a function called search, which...Ch. 17.1 - Prob. 6STECh. 17.2 - Give the definition for the member function...Ch. 17.2 - Give the definition for the constructor with zero...Ch. 17.2 - Give the definition of a template class called...Ch. 17.2 - Is the following true or false? Friends are used...
Ch. 17 - Write a function template for a function that has...Ch. 17 - Prob. 2PCh. 17 - Prob. 3PCh. 17 - Redo Programming Project 3 in Chapter 7, but this...Ch. 17 - Display 17.3 gives a template function for sorting...Ch. 17 - (This project requires that you know what a stack...Ch. 17 - Prob. 6PPCh. 17 - Prob. 7PPCh. 17 - This project requires that you complete...
Knowledge Booster
Similar questions
- Add the function min as an abstract function to the class arrayListType to return the smallest element of the list. Also, write the definition of the function min in the class unorderedArrayListType and write a program to test this function. I have 5 tabs: I have tried every solution I can think of with no luck. These are the guides: arrayListType.h arrayListTypeImp.cpp: main.cpp unorderedArraryListType.h unorderedArrayListTypeImp.cpp I am needing these in order to pass the assignment in Cengage Mindtap, please help with codes for each one if possible.arrow_forwardUsing java, answer the following questions about the code below. 1) Create a new function or method that checks to see if an int parameter is present in the list. 2) Create a main() method or JUnit tests to show that your list code functions as intended. (Code) private class Nodeint data;Node next;Node(int data) {this.data = data;this.next = null;}}private Node first;private Node last; public void addToEnd(int data){ Node newNode = new Node(data);if (first == null){first = newNode;last = newNode;} else {last.next = newNode;last = newNode;}}public void printList(){ Node current = first;while (current != null){System.out.print(current.data + " ");current = current.next;}System.out.println();}public static void main(String[] args){node list = new node();list.addToEnd(2);list.addToEnd(4);list.addToEnd(6);list.printList();}}arrow_forwardWrite a JAVA program Write a function inside ProblemSolution class whose return type is void, accepts an array and the length of the array as input parameters. The function should call a static method display of MyArray class by passing an array and length value. Input 5 1 5 8 2 0 Where, First line of input represents the size of an array. Second line represents array elements. Output 1 5 8 2 0 Assume that, N is an integer within the range [0 to 10000]. Array elements are integers within the range [-2147483648 to 2147483647].arrow_forward
- In Kotlin, use this Employee class Use this Employee class data class Employee(val name: String, val wage: Double, val hours: Double) and this list of Employees: val l: List = listOf(Employee("Bob", 34.33, 42.0), Employee ("Cathy", 27.33, 15.25), Employee("Carlos", 46.99, 38.75), Employee("Tomas", 49.66,28.45), Employee("Xavier", 34.56, 11.5)) Write a function called formatPaycheck that takes a Pair and returns a String. formatPaycheck should use the two parts of the Pair to put together and return a String like this, with the paycheck amount shown to two digits past the decimal point: Pay to the order of Cathy $359.47 Use raise() to raise all the Employees' pay by 15%. Then use map with the payroll and formatPaycheck functions to get a list of the paychecks in the format shown above. Print the result. The output should look like this: [Pay to the order of Bob $1077.79, Pay to the order of Cathy $359.47, Pay to the order of Carlos $1361.09, Pay to the order of Tomas $1056.09,…arrow_forwardWrite the definitions of the member functions of the classes arrayListType and unorderedArrayListType that are not given in this chapter. The specific methods that need to be implemented are listed below. Implement the following methods in arrayListType.h: isEmpty isFull listSize maxListSize clearList Copy constructor Implement the following method in unorderedArrayListType.h insertAt Also, write a program (in main.cpp) to test your function.arrow_forwardReuse your Car class . In a main, build an object of that class, and print out the object using System.out.println(). Notice that this simply reports the memory address of the object in question, and we’d like to do something more useful. To replace (or override) the toString (or equals) function. Now, build a toString function that prints out the make, model, and odometer reading for a vehicle object. public class Car { //instance variables private int odometer; private String make; private String model; //overloading //constructors public Car(int odometer,String make, String model) { this.odometer = odometer; this.make = make; this.model = model; } public Car(String make, String model) { this.make = make; this.model = model; } public Car(String make) { this.make = make; } /* *getter & setter methods */ public int getOdometer() { return odometer; } public void…arrow_forward
- for c++ A set is a collection of distinct elements of the same type. Design the class unorderedSetType. Design the class unorderedSetType, derived from the class unorderedArrayListType, to manipulate sets. Note that you need to redefine only the functions insertAt, insertEnd, and replaceAt. If the item to be inserted is already in the list, the functions insertAt and insertEnd output an appropriate message. Similarly, if the item to be replaced is already in the list, the function replaceAt outputs an appropriate message. Write a program to test your class.arrow_forwardConsider the following definition of class Person: 1 public class Person 2 { 3 public final String name; 4 5 public Person(String name) 6 { 7 if (name == null) this.name = "<NoName>"; //No null names... 8 else this.name = name; 9 } 10 } and the definition of class PersonListUtils implemented by a student... 1 public class PersonListUtils 2 { 3 //Replaces the first occurrence of a Person in the list with the given oldPersonName with a new Person having the newPersonName. 4 //Returns true if the substitution is successful, false otherwise 5 public static boolean substitute(ArrayList<Person> list, String oldPersonName, String newPersonName) 6 { 7 for (Person person: list) { 8 if (person.name == oldPersonName) { 9 person = new Person(newPersonName); 10 return true; 11 } 12 } 13 return false; 14 } 15 16 //Removes from the list the first occurrence of a Person with the given nameToRemove. 17 //Returns true if the deletion is successful, false otherwise 18 public static boolean…arrow_forwardin PYTHON, please Given a base Plant class and a derived Flower class, write a program to create a list called my_garden. Store objects that belong to the Plant class or the Flower class in the list. Create a function called print_list(), that uses the print_info() instance methods defined in the respective classes and prints each element in my_garden. The program should read plants or flowers from input (ending with -1), add each Plant or Flower to the my_garden list, and output each element in my_garden using the print_info() function. Note: A list can contain different data type and also different objects. Ex. If the input is: plant Spirea 10 flower Hydrangea 30 false lilac flower Rose 6 false white plant Mint 4 -1 the output is: Plant Information: Plant name: Spirea Cost: 10 Plant Information: Plant name: Hydrangea Cost: 30 Annual: false Color of flowers: lilac Plant Information: Plant name: Rose Cost: 6 Annual: false Color of flowers: white Plant…arrow_forward
- ????????: Implement the design of the Pizza class so that the following output is produced: [Your code should work for any number of parameters added in the set_toppings_info method] # Write your codes here. print("Pizza Count:", Pizza.pizza_count) print("=======================") p1 = Pizza("Chicken") p1.set_toppings_info(25, 1, 4, 0) p1.display() print("------------------------------------") p2 = Pizza("Olives") p2.set_toppings_info(15, 1.5, 0, 0) p2.display() print("------------------------------------") p3 = Pizza("Sausage") p3.set_toppings_info(50, 5, 2, 0) p3.display() print("=======================") print("Pizza Count:", Pizza.pizza_count) Output: Pizza Count: 0 ======================= Toppings: Chicken 25 calories 1 g fat 4 g protein 0 g carbs ------------------------------------ Toppings: Olives 15 calories 1.5 g fat 0 g protein 0 g carbs ------------------------------------ Toppings: Sausage 50 calories 5 g fat 2 g protein 0 g carbs ======================= Pizza…arrow_forwardWrite a generic class that stores two elements of the same type and can tell the user which one is the larger between the two. The type of the two elements should be parameterized and the class should have a function called “maxElement” that returns the larger element. write this java codearrow_forwardImplement the Rectangle class as discussed this week. Use the class declaration below. Your job is to implement the functions. Implement the missing functions yourself. class Rectangle{ private: double width; double length; char *name; void initName(char *); public://constructorsRectangle();Rectangle(double, double, char*);//destructor ~Rectangle(); void setWidth(double); void setLength(double); void setWidth(char *); void setLength(char *); void setName(char *); double getWidth() const; double getLength() const; void printName() const { cout << name; }}; A few notes on the functions: initName(char *): this is a private member function. It should be the only function which dynamically allocates a char array to hold the name. setName(char *): this is a public member function which changes the name of the rectangle to a new name. It does not dynamically allocate memory, it only changes name. Demonstrate your class works…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
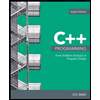
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning