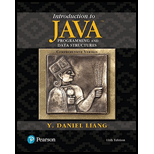
(Revising Listing 19.1) Revise the GenericStack class in Listing 19.1 to implement it using an array rather than an ArrayList. You should check the array size before adding a new element to the stack. If the array is full, create a new array that doubles the current array size and copy the elements front the current array to the new array.

Revising Listing 19.1
Program Plan:
- Define the class named “GenericStack<E>”.
- Declare appropriate variables to program.
- Define constructors “GenericStack()” which assigns initial size of stack and “GenericStack(initialCapaciy)” which assigns size of the stack.
- Define a method “push()” which pass “E o” as parameter.
- Using “if” condition, check the value of “N” greater than “elements.length”.
- Assign size of new array.
- Copy old array into new array.
- Return array elements.
- Using “if” condition, check the value of “N” greater than “elements.length”.
- Define a method “pop()” which pop out stack elements.
- Return array elements.
- Define a method “peek()” which returns top of the stack.
- Define a method “isEmpty()” which returns “0”.
- Define a method “getSize()” which returns size of the stack.
- Define the main method.
- Declare the GenericStack object “obj”, using the object insert elements into stack.
- Using “while” loop, print the elements on screen.
The following JAVA code is to revise the “GenericStack” class which implements array rather than an “ArrayList”.
Explanation of Solution
Program:
//Class definition
class GenericStack<E>
{
/*Declaration of variables*/
public final static int INITIAL_SIZE = 16;
private E[] elements;
private int N;
/*Construct a stack with the default initial capacity */
public GenericStack()
{
//Assign initial size
this(INITIAL_SIZE);
}
/*Construct a stack with the specified initial capacity */
public GenericStack(int initialCapacity)
{
//Assign size
elements = (E[])new Object[initialCapacity];
}
/*Push a new element into the top of the stack */
public E push(E o)
{
//Condition
if (N >= elements.length)
{
//Assign array size into variable "t"
E[] t = (E[])new Object[elements.length * 2];
//Copy array elements
System.arraycopy(elements, 0, t, 0, elements.length);
//Assign "t" into "elements"
elements = t;
}
//Return statement
return elements[N++] = o;
}
/*Return and remove the top element from the stack*/
public E pop()
{
//Return statement
return elements[--N];
}
/*Return the top element from the stack */
public E peek()
{
//Return statement
return elements[N - 1];
}
/*Function definition to check empty*/
public boolean isEmpty()
{
//Return statement
return N == 0;
}
/*Return the number of elements in the stack */
public int getSize()
{
//Return statement
return N;
}
//Main method
public static void main(String[] args)
{
//Assign object to "GenericStack"
GenericStack<String> obj = new GenericStack<>();
//Push elements into stack
obj.push("London");
obj.push("Paris");
obj.push("Berlin");
//Print elements
System.out.print("Stack 1: ");
//Loop
while (!obj.isEmpty())
{
//Print statement
System.out.print(obj.pop() + " ");
}
//Print statement
System.out.println("\n");
}
}
Stack 1: Berlin Paris London
Want to see more full solutions like this?
Chapter 19 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Additional Engineering Textbook Solutions
INTERNATIONAL EDITION---Engineering Mechanics: Statics, 14th edition (SI unit)
Introduction To Programming Using Visual Basic (11th Edition)
Mechanics of Materials (10th Edition)
Java: An Introduction to Problem Solving and Programming (8th Edition)
Web Development and Design Foundations with HTML5 (8th Edition)
Starting Out with Python (4th Edition)
- Ensure you answer the question asked at the end of the document. Do not just paste things without the GNS3 console outputsarrow_forward"Do not use AI tools. Solve the problem by hand on paper only and upload a photo of your handwritten solution."arrow_forward"Do not use AI tools. Solve the problem by hand on paper only and upload a photo of your handwritten solution."arrow_forward
- "Do not use AI tools. Solve the problem by hand on paper only and upload a photo of your handwritten solution."arrow_forward"Do not use AI tools. Solve the problem by hand on paper only and upload a photo of your handwritten solution."arrow_forwardSolve this "Do not use AI tools. Solve the problem by hand on paper only and upload a photo of your handwritten solution."arrow_forward
- "Do not use AI tools. Solve the problem by hand on paper only and upload a photo of your handwritten solution."arrow_forward"Do not use AI tools. Solve the problem by hand on paper only and upload a photo of your handwritten solution."arrow_forwardSpecifications: Part-1Part-1: DescriptionIn this part of the lab you will build a single operation ALU. This ALU will implement a bitwise left rotation. Forthis lab assignment you are not allowed to use Digital's Arithmetic components.IF YOU ARE FOUND USING THEM, YOU WILL RECEIVE A ZERO FOR LAB2!The ALU you will be implementing consists of two 4-bit inputs (named inA and inB) and one 4-bit output (named out). Your ALU must rotate the bits in inA by the amount given by inB (i.e. 0-15).Part-1: User InterfaceYou are provided an interface file lab2_part1.dig; start Part-1 from this file.NOTE: You are not permitted to edit the content inside the dotted lines rectangle. Part-1: ExampleIn the figure above, the input values that we have selected to test are inA = {inA_3, inA_2, inA_1, inA_0} = {0, 1, 0,0} and inB = {inB_3, inB_2, inB_1, inB_0} = {0, 0, 1, 0}. Therefore, we must rotate the bus 0100 bitwise left by00102, or 2 in base 10, to get {0, 0, 0, 1}. Please note that a rotation left is…arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- New Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage LearningProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
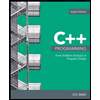
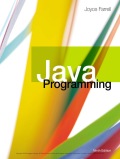
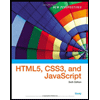
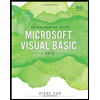
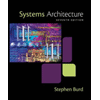