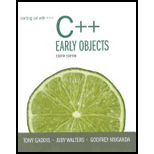
Starting Out With C++, Early Objects - With Access Package
8th Edition
ISBN: 9780133441840
Author: GADDIS
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 19, Problem 1PC
Program Plan Intro
Simple Binary Search Tree Class
Program Plan:
- Include the required header files.
- Define the class BTreeNode.
- Create constructor by passing the three parameters.
- Declare the object for binary tree.
- Declare class BST has friend.
- Define the class BST.
- Define the search() function.
- Call search() function recursively and then return the result.
- Declare insert() function prototype.
- Define inorder() function.
- Call inorder() function recursively and then return the result.
- Declare the private search() function prototype.
- Declare the private inorder() function prototype.
- Define the search() function.
- In the search() function,
- Check whether the tree is empty. If yes, return false, there is no node in tree.
- Check whether the tree is equal to x. If yes, return true, the search value is found
- Check whether the tree is greater than x. If yes, call search() function by passing left node and result is returned.
- Otherwise, call search() function by passing right node and result is returned.
- In the insert() function,
- Check whether the tree is empty. If yes, create an object for binary tree.
- Loop executes until the tree is not empty. If yes,
- Check whether x is less than or equal to tree. If yes, x goes to left node of binary tree.
- Otherwise, x goes to right node of binary tree.
- In the inorder() function,
- Check whether the tree is empty. If yes, exit the statement.
- Call inorder() function by passing left node.
- List the inorder elements from tree.
- Call inorder() function by passing right node.
- Define the “main()” function.
- Read the five inputs from user.
- Call insert() function to insert all elements into binary tree.
- Call inorder() function to inorder the elements present in binary tree.
- Call search() function to search the specified values and then displays it.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Binary Search Tree Implementation(Java)In the binary search tree implementation, which is completely unrelated to the linked list implementation above, you will use an unbalanced binary search tree. Since duplicates are allowed, each node will have to store a singly-linked list of all the entries that are considered identical. Two values are identical if the comparator returns 0, even if the objects are unequal according to the equals method. A sketch of the private representation looks something like this:
private Comparator<? super AnyType> cmp;private Node<AnyType> root = null;
private void toString( Node<AnyType> t, StringBuffer sb ){ ... the recursive routine to be called by toString ... }
private static class Node<AnyType>{private Node<AnyType> left;private Node<AnyType> right;private ListNode<AnyType> items;
private static class ListNode<AnyType>{private AnyType data;private ListNode<AnyType> next;
public ListNode( AnyType d,…
Attached is a C program traveselter.c. This is a solution to the problem of constructing a binary tree from the inorder and preorder listing
of the nodes. It has the recursive function
void list_inorder(struct Node * root)
which lists the nodes of the tree in-order. Rewrite this function so that it is iterative.
Hint: Implement a stack and you may assume it can hold a maximum of 100 items. You have to decide what will be in an item of the stack,
and design a structure for it. I suggest that one member of an item is a pointer to a tree node.
#include <stdlib.h>
#include <stdio.h>
struct Node {
int val;
struct Node * left;
struct Node * right;
};
struct Node * create_node(char val);
void destroy_node(struct Node * root);
void destroy_tree(struct Node * root);
void list_preorder(struct Node * root);
struct Node * create_tree(char pre_order[], int pre_first, int pre_last, char in_order[], int in_first, int in_last);
void list_inorder(struct Node *…
C++ PROGRAMMINGTopic: Binary Search Trees
Explain the c++ code below.: It doesn't have to be long, as long as you explain what the important parts of the code do. (The code is already implemented and correct, only the explanation needed)
node* left(node* p) {
return p->left;
}
node* right(node* p) {
return p->right;
}
node* sibling(node* p){
if(p != root){
node* P = p->parent;
if(left(P) != NULL && right(P) != NULL){
if(left(P) == p){
return right(P);
}
return left(P);
}
}
return NULL;
}
node* addRoot(int e) {
if(size != 0){
cout<<"Error"<<endl;
return NULL;
}
root = create_node(e,NULL);
size++;
return root;
}
node* addLeft(node* p, int e) {
if(p->left == NULL){
node* newLeft =…
Chapter 19 Solutions
Starting Out With C++, Early Objects - With Access Package
Ch. 19.1 - Prob. 19.1CPCh. 19.1 - Prob. 19.2CPCh. 19.1 - Prob. 19.3CPCh. 19.1 - Prob. 19.4CPCh. 19.1 - Prob. 19.5CPCh. 19.1 - Prob. 19.6CPCh. 19.2 - Prob. 19.7CPCh. 19.2 - Prob. 19.8CPCh. 19.2 - Prob. 19.9CPCh. 19.2 - Prob. 19.10CP
Ch. 19.2 - Prob. 19.11CPCh. 19.2 - Prob. 19.12CPCh. 19 - Prob. 1RQECh. 19 - Prob. 2RQECh. 19 - Prob. 3RQECh. 19 - Prob. 4RQECh. 19 - Prob. 5RQECh. 19 - Prob. 6RQECh. 19 - Prob. 7RQECh. 19 - Prob. 8RQECh. 19 - Prob. 9RQECh. 19 - Prob. 10RQECh. 19 - Prob. 11RQECh. 19 - Prob. 12RQECh. 19 - Prob. 13RQECh. 19 - Prob. 14RQECh. 19 - Prob. 15RQECh. 19 - Prob. 16RQECh. 19 - Prob. 17RQECh. 19 - Prob. 18RQECh. 19 - Prob. 19RQECh. 19 - Prob. 20RQECh. 19 - Prob. 1PCCh. 19 - Prob. 2PCCh. 19 - Prob. 3PCCh. 19 - Prob. 4PCCh. 19 - Prob. 5PCCh. 19 - Prob. 6PCCh. 19 - Prob. 7PCCh. 19 - Prob. 8PCCh. 19 - Prob. 9PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Array_based circular queue: Define the class Queue using one dimensional circular array representation with no implementation; i.e. declare the data members, and the function members only (Enqueue, Dequeue, IsEmpty, GetHead etc.). Implement the Ennqueue method of the above classarrow_forwardAdd to the BinarySearchTree class a member function string smallest() const that returns the smallest element of the tree. Provide a test program to test your function. #include <iostream>#include <string>using namespace std;class TreeNode{public:void insert_node(TreeNode* new_node);void print_nodes() const;bool find(string value) const;private:string data;TreeNode* left;TreeNode* right;friend class BinarySearchTree;};class BinarySearchTree{public:BinarySearchTree();void insert(string data);void erase(string data);int count(string data) const;void print() const;private:TreeNode* root;};BinarySearchTree::BinarySearchTree(){root = NULL;}void BinarySearchTree::print() const{if (root != NULL)root->print_nodes();}void BinarySearchTree::insert(string data){TreeNode* new_node = new TreeNode;new_node->data = data;new_node->left = NULL;new_node->right = NULL;if (root == NULL) root = new_node;else root->insert_node(new_node);}void TreeNode::insert_node(TreeNode*…arrow_forward2 ASAP PLEASE.... Define a Haskell instance of the Functor class for the following type of binary tree with data in nodes and leaves: The definition should be used as below.data Tree a = Leaf a | Node a (Tree a) (Tree a) Note: I need code for exactly as above, noooooooooooooo expalanations, just code for above.arrow_forward
- a) The preorder traversal of a Binary Search Tree (BST) is given below.40 20 10 15 25 30 60 50 80 100 Draw the BST and briefly explain the process you followed to build the tree. b) Write a function f1 that takes the root of a binary tree as a parameter and returns the sum of the nodes, which are the left child of another node. The root of the tree is not a child of any node. Consider the following class definitions while writing your code.class Node {public:int key;Node* left;Node* right;};arrow_forwardC++ DATA STRUCTURES Implement the TNode and Tree classes. The TNode class will include a data item name of type string,which will represent a person’s name. Yes, you got it right, we are going to implement a family tree!Please note that this is not a Binary Tree. Write the methods for inserting nodes into the tree,searching for a node in the tree, and performing pre-order and post-order traversals.The insert method should take two strings as input. The second string will be added as a child node tothe parent node represented by the first string. Hint: The TNode class will need to have two TNode pointers in addition to the name data member:TNode *sibling will point to the next sibling of this node, and TNode *child will represent the first child ofthis node. You see two linked lists here??? Yes! You’ll need to use the linked listsarrow_forwardComputer Science QUESTION: Expression tree is an application of binary tree to represent arithmetic expression. Write a program to implement an ExpressionTree class that can represent an arithmetic expression with binary operators and integer operands including floating-point. Limit the operators to addition, subtraction, multiplication and division. Operands can be constants or variables. Your class should be able to build a tree from its signature, and evaluate it on a set of values for the variable operands.arrow_forward
- In c++, Write a Binary Search Tree for strings datatype, using pointers Besure that it has a method that can Rebalance the tree. See below for driver file Testing requirments: int main() { myBTS BTS1; // Declare/Instanitate an instance of the BTS BTS1.inTree("Fred"); BTS1.inTree("Able"); BTS1.inTree("Tuyet"); BTS1.inTree("Mojo"); BTS1.inTree("Linda"); BTS1.inTree("Leena"); BTS1.inTree("Xoe"); BTS1.inTree("Zohe"); BTS1.inTree("Alfred"); BTS1.inTree("Thanos"); BTS1.inTree("koji"); BTS1.inTree("Hally"); BTS1.inTree("Lee"); BTS1.inTree("Mode"); cout << "Number of names in the tree: " << BTS1.count() << endl; if ( BTS1.verify () ) // Function returns a Boolean value { cout << "BST Verified" <arrow_forward1. Do some research about binary search trees. 2. Create a structure in C for the nodes of this tree. Which variables do the structure have? 3. Write a function that builds a balanced binary search tree. The input argument of this function is a sorted array of integers. 4. Write a recursive function that inserts a new element to the tree. The input argument of this function is a pointer to the head node of the tree. 5. What is the time complexity of this insertion function?arrow_forwardIn c++ A company wants to store his Employees data but orderly. Because of that owner wants to use a binary search tree. Each Node must store the employee number, name, and salary double (class). Implement in java/C++ a binary search tree that stores the employees and implements the following methods: a) Include a new Employee (insert values) b) Search an employee given its number. c) Delete an employee. d) Get in-order, pre-order, post-order traversal of tree and display values.arrow_forward
- BST - Binary Search Tree - implement a BSTNode ADT with a data attribute and two pointer attributes, one for the left child and the other for the right child. Implement the usual getters/setters for these attributes -implement a BST as a link-based ADT whose data will be Dollar objects - the data will be inserted based on the actual money value of your Dollar objects as a combination of the whole value and fractional value attributes. - BST, implement the four traversal methods as well as methods for the usual search, insert, delete, print, count, isEmpty, empty operations and any other needed. - BST - Binary Search Tree - implement a BSTNode ADT with a data attribute and two-pointer attributes, one for the left child and the other for the right child. Implement the usual getters/setters for these attributes -implement a BST as a link-based ADT whose data will be Dollar objects - the data will be inserted based on the actual money value of your Dollar objects as a combination of the…arrow_forwardDefine the function (doubleBubbleLst lst). This function should resolve to a list of sublists, where each sublist holds a single element from lst and all sublists from lst are also bubbled, so that every list and sublist has no atoms. This is the deep recursion version of bubbleLst. For example: (doubleBubbleLst '(1 2 (3 4)) ) resolves to '((1) (2) (( (3) (4) ))).arrow_forwardComplete the Binary Search Tree class: 1. Implement member function for deleteNode 2. Implement member functions for Pre-order and Post-order traversal 3. Develop the menu for different tasks as shown in sample output. Sample Output: -arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
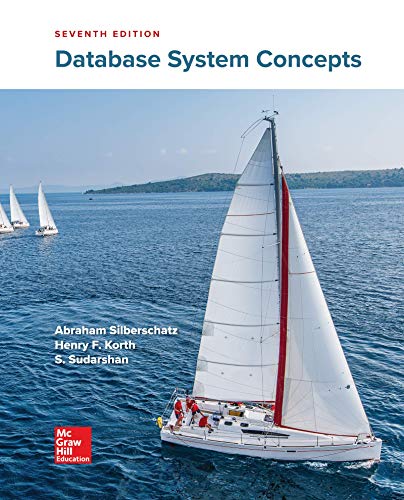
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
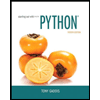
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
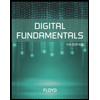
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
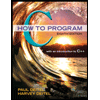
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
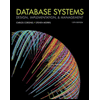
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
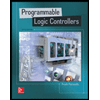
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education