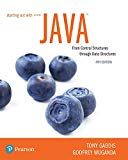
Explanation of Solution
Method definition for “removeMin()”:
The method definition for “removeMin()” is given below:
/* Method definition for "removeMin()" */
String removeMin()
{
/* If the list head is null, then */
if (first == null)
//Returns null
return null;
/* Set minimum string to "first" node */
Node minimumString = first;
/* Set minimum string predecessor to list head*/
Node minimumPred = first;
/* Set reference node to next node of list head */
Node refNode = first.next;
/* Set the reference of predecessor node */
Node refPred = first;
/* If the reference node "refNode" is not "null", then */
while (refNode != null)
{
/* Check condition */
if (refNode.value.compareTo(minimumString.value) < 0)
{
/* Assign minimum string to "refNode" */
minimumString = refNode;
/* Set minimum predecessor to reference of predecessor */
minimumPred = refPred;
}
/* Set "refPred" to "refNode*/
refPred = refNode;
/* Set "refNode" to next reference node */
refNode = refNode.next;
}
// Compute If the first node is the minimum or not
String resultantString = minimumString.value;
/* If the minimum string is list head, then */
if (minimumString == first)
{
//Remove the first string
first = first.next;
/* If the list head is "null", then */
if (first == null)
/* Set "last" to "null" */
last = null;
}
//Otherwise
else
{
//Remove an element with a predecessor
minimumPred.next = minimumString.next;
// If the last item removed, then
if (minimumPred.next == null)
/* Assign "last" to "minimumPred" */
last = minimumPred;
}
/* Finally returns the resultant string elements */
return resultantString;
}
Explanation:
The above method definition is used to remove a minimum element from a list.
- If the list head is null, then returns null.
- Set minimum string and minimum predecessor to “first” node.
- Set reference node to next node of list head and also set the reference of predecessor node.
- Performs “while” loop. This loop will perform up to the “refNode” becomes “null”.
- Check condition using “if” loop.
- If the given condition is true, then assign minimum string to “refNode”.
- Set minimum predecessor to reference of predecessor.
- Set “refPred” to “refNode”.
- Set “refNode” to next reference node.
- Check condition using “if” loop.
- Compute if the first node is minimum or not.
- If the minimum string is list head, then
- Remove the first string.
- If the list head is “null”, then set “last” to “null”.
- Otherwise,
- Remove an element with a predecessor.
- If the last item removed, then assign “last” to “minimumPred”.
- Finally returns the resultant string elements.
Complete code:
The complete executable code for remove a minimum string element from a linked list is given below:
//Define "LinkedList1" class
class LinkedList1
{
/** The code for this part is same as the textbook of "LinkedList1" class */
/* Method definition for "removeMin()" */
String removeMin()
{
/* If the list head is null, then */
if (first == null)
//Returns null
return null;
/* Set minimum string to "first" node */
Node minimumString = first;
/* Set minimum string predecessor to list head*/
Node minimumPred = first;
/* Set reference node to next node of list head */
Node refNode = first...

Want to see the full answer?
Check out a sample textbook solution
Chapter 19 Solutions
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
- Create a method for a linked list that will delete any nodes that have the same key.arrow_forwardPlease use Java Write a method “removeHighestLinkedList” that takes a LinkedList and removes the highest value.arrow_forwardimplement this method: numOccurrencesRec(LNode node, int n, int key) – This method takes as parameters a reference to the head of a linked list, a position specified by n, and a key. It returns the number of occurrences of the key in the linked list beginning at the n-th node. If n = 0, it means you should search in the entire linked list. If n = 1, then you should skip the first node in the list.arrow_forward
- Apart from the main list in the list above, elements with the same value are also linked. According to this; a) Write the Generic Node class. b) Write the method that adds a new element to the end of the list. JAVA CODEarrow_forwardCreate an algorithm that deletes a given piece of information from a linked list, every time it finds it. Specify the methods and classes used (no code, just algorithms)arrow_forwardIn the following code, it errors at / Return the maximum subarray among the left, right, and cross subarrays if (left.getLast() >= right.getLast() && left.getLast() >= cross.getLast()) { returnleft; } else if (right.getLast() >= left.getLast() && right.getLast() >= cross.getLast()) { returnright; } else { returncross; } Code: publicstatic Triple<Node,Node,Integer> getMaxSubList(LinkedListlist) { // If the list is null or empty, return a Triple with all values set to 0 if (list == null || list.isEmpty()) { returnnew Triple(0, 0, 0); } // Call the private helper method to find the maximum subarray returnfindMaxSubList(list, 0, list.size() - 1); } // This is a recursive helper method that divides the list into smaller subarrays // and finds the maximum subarray of each subarray. It then combines these maximum subarrays // to find the maximum subarray of the entire list. privatestaticTriple findMaxSubList(LinkedListlist, intlow, inthigh) { //…arrow_forward
- Develop a plan that will allow you to remove from a linked list all of the nodes that have the same key.arrow_forwardUsing the Collections framework (ArrayList or LinkedList), create and add a stack of 5 names and then, remove 3 elements and display a count of the names left.arrow_forwarddelete the Nth node from the end of the LinkedList in javaarrow_forward
- Java Given main() in the Inventory class, define an insertAtFront() method in the InventoryNode class that inserts items at the front of a linked list (after the dummy head node).arrow_forwardCreate a new Hash class that uses an arraylist instead of an array for the hash table. Test your implementation by rewriting (yet again) the computer terms glossary application.arrow_forwardRemoves the element with key k if it exists. The position of current is// unspecified after calling this method.The first element of the returned pair // indicates whether k was removed, and the second is the number of key// comparisons made.Pair<Boolean, Integer> remove(K key); // Returns all keys of the map as a list sorted in increasing order. List<K> getAll(); } write this method javaarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
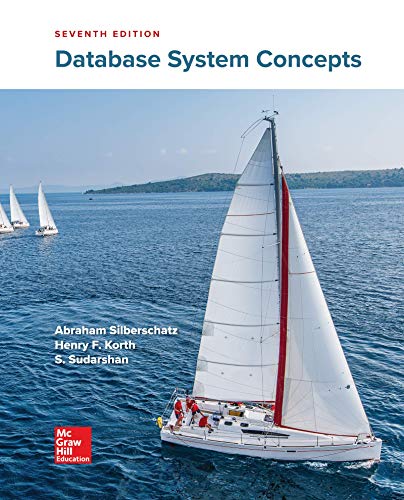
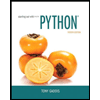
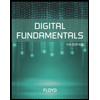
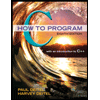
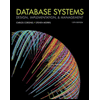
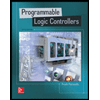