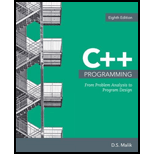
Concept explainers
Implementation of a
Program Plan:
Write a C++ program with a main function and the required set of statements to accomplish the following:
Write C++ statements that include the header files iostream and string
Write a C++ statement that allows you to use cin, cout, and endl without the prefix std::.
Write C++ statements that declare and initialize the following named constants: SECRET of type int initialized to 11 and RATE of type double initialized to 12.50
Write C++ statements that declare the following variables: num1, num2, and newNum of type int; name of type string; and hoursWorked and wages of type double
Write C++ statements that prompt the user to input two integers and store the first number in num1 and the second number in num2
Write a C++ statement(s) that outputs the values of num1 and num2, indicating which is num1 and which is num2. For example, if num1 is 8 and num2 is 5, then the output is,
The value of num1 = 8 and the value of num2 = 5.
Write a C++ statement that multiplies the value of num1 by 2, adds the value of num2 to it, and then stores the result in newNum. Then, write a C++ statement that outputs the value of newNum
Write a C++ statement that updates the value of newNum by adding the value of the named constant SECRET to it. Then, write a C++ statement that outputs the value of newNum with an appropriate message
Write C++ statements that prompt the user to enter a person’s last name and then store the last name into the variable name
Write C++ statements that prompt the user to enter a decimal number between 0 and 70 and then store the number entered into hoursWorked
Write a C++ statement that multiplies the value of the named constant RATE with the value of hoursWorked and then stores the result into the variable wages
Write C++ statements that produce output similar to:
Name: Rainbow
Pay Rate: $12.50
Hours Worked: 45.50
Salary: $568.75
Write a C++ program that tests each of the C++ statements that you wrote in parts A through L. Place the statements at the appropriate place in the C++ program segment given at the beginning of this problem. Test run your program (twice) on the following input data:
num1 = 13, num2 = 28; name = "Jacobson"; hoursWorked = 48.30.
num1 = 32, num2 = 15; name = "Crawford"; hoursWorked = 58.45.

Trending nowThis is a popular solution!

Chapter 2 Solutions
C++ Programming: From Problem Analysis to Program Design
- Consider the following C++ code and fill the blanks: string name, phrase; cout<<“Please enter your full name:” <<endl; cin>>name; //What is your input? ___ ________________________ phrase = “ loves holidays!” phrase = name + phrase; cout<<phrase<<endl; a) What will be the output of this code? b) How can you modify the code so that your full name will be displayed in the output?arrow_forwardThis is a c++ programming question. Write a header file called apple.h It should contain the following items: - a macro which gets one input parameter and return the square of the input value. - an inline function which gets two float type input parameters and returns the greater value. - a symbolic constant called M which has the value 100.arrow_forwardConsider the following C++ code and fill the blanks: string name, phrase; cout<<“Please enter your full name:” <<endl; cin>>name; //What is your input? ___ ________________________ phrase = “ loves holidays!” phrase = name + phrase; cout<<phrase<<endl;arrow_forward
- *Correct all syntax errors so that the C++ program will compile correctly.* Procided C++ code: #include<iostream>#include<conio.h> Standard namespace declarationusing namespace std; Main Functionint main(){ double myMoney = 1000.50; //this should be printed out Standard Ouput Statement cout"Please be sure to correct all syntax errors in this program"<<endl; cout<<"I have corrected all errors for this program. <<endl; cout<<" The total amount of money available is = "<< <<endl; // Wait For Output Screen Main Function return Statement return 0;} Thank you for your helparrow_forwardThis is for Python Programing version 3.8.5 - Need a program that uses a text file to store weekly payroll data for the office. The program should enable the user to enter the name, hourly pay rate, and weekly hours for a number of office employees. The pay rate and hours should be type float, and each datum should be written to its own line in the file. Enter data for at least five employees and then close the file. Please include Pseudocode and def main () function.arrow_forwardWrite a c++ program to display a calendar for a given year. Type of Branches such as switch statements, if-else, and etc. should be used as well. You can't define functions or use Void. Everything should be written inside int main(). Code should be written in this type of format: #include <iostream>using namespace std; int main() { /* Type your code here. */ return 0;} Ask a user to enter the year and the new year's day of the year entered: cout <<"Enter a calendar year to display?"; cout <<" what is the new year's day?"; a leap year is: IF year%4 = 0 AND year%100 != 0 OR year%400 = 0 An example of what the program should display as a result is in the two images attached.arrow_forward
- Find errors in the following lines of c++ code assuming included header files. Write correct form with line number. 1. int main() 2. { 3. Int a=4.5 4. Char ch=’a’ 5. Int a++; 6. Cout<<”the value of a=’; 7. Cout>>a; 8. For( ; a<=5; ) 9. Cout<<a; 10. }arrow_forward(Numerical) a. Write a C++ program that accepts an integer argument and determines whether the passed integer is even or odd. (Hint: Use the % operator.) b. Enter, compile, and run the program written for Exercise 8a.arrow_forwardC++ Please! Also please don't use functions, arrays, or classes. Just basic c++ please. Password Verifier Write a C++ program to verify that a user’s password meets the following criteria: 1. The password should be at least 6 characters long. 2. The password should contain at least one uppercase and one lowercase letter. 3. The password should have at least one digit. Your program should ask the user for a password and verify that it meets the following criteria. If it doesn’t a message should be displayed telling the user why.arrow_forward
- Use Dev C++ The Problem: Topics: Switch statement, Loops, Functions, Creating Program-Defined Value-Returning Functions, Calling a Function, Void Functions Write a program in C++ involving a Switch statement from the 4 program given below : 1. Create a Program that allows the user to have a switch statement menu from your following BOM and Info. (5 Materials per BOM) Use 12% as your tax for Manufacturing and Construction BOMs. (VAT Registered) Use 3% as your tax for Electrical BOMs. (Example as Non-VAT Registered) Program Requirement: 1. The program should compute for the : Total added tax Net total Amount = Amount without tax Gross total amount = Amount with tax 2. Quantity should be asked for every material given. 3. Create an IPO chart and Desk CheckingCompany name Date : LastName, FirstName, M.I. LastName, FirstName, M.I. LastName, FirstName, M.I. 4. The program should use both VR Functions and Void Functions for the 3 BOMs.arrow_forwardScope and Limitation: Your program must include ONLY and ALL of the following C LANGUAGE statements: Basic I/O, Ladderized if -else, switch statements, and any looping statements. Engineering EquationsResearch two COMPLEX ENGINEERING EQUATIONS () related to Chemistry or Biology. Equations should have four variables. (Do not create your own equation. Algebra, trigonometric, or analytic geometry are not considered complex engineering equations) Program Requirements1. Program shows a formula selection screen that allows the user to select a formula.2. Formula selection screen allows the user to quit the program.3. Program asks the user what variable in the equation to compute.4. Variable selection screen allows the user to go back to the formula selection screen5. Program asks for values for input variables and computes the selected variable.6. Program goes back to the variable selection screen. Users can select another variable to compute or select to go back to the formula selection…arrow_forwardScope and Limitation: Your program must include ONLY and ALL of the following C LANGUAGE statements: Basic I/O, Ladderized if -else, switch statements, and any looping statements. Engineering EquationsResearch two COMPLEX ENGINEERING EQUATIONS () related to Chemistry or Biology. Equations should have four variables. (Do not create your own equation. Algebra, trigonometric, or analytic geometry are not considered complex engineering equations) Program Requirements1. Program shows a formula selection screen that allows the user to select a formula.2. Formula selection screen allows the user to quit the program.3. Program asks the user what variable in the equation to compute.4. Variable selection screen allows the user to go back to the formula selection screen5. Program asks for values for input variables and computes the selected variable.6. Program goes back to the variable selection screen. Users can select another variable to compute or select to go back to the formula selection…arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
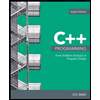
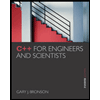