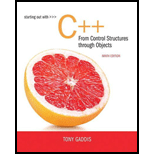
Starting Out with C++ from Control Structures to Objects (9th Edition)
9th Edition
ISBN: 9780134498379
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 20, Problem 10PC
Program Plan Intro
MaxNode function
Program Plan:
“Main.cpp”:
- Include the required header files.
- Declare the necessary function prototype and constants.
- Define the main () function.
- Declare the necessary variables.
- Insert the values into the list.
- Displays to user that the values are inserted.
- Call the method “maximumnode()” to find the largest element of the list.
- The largest valued node gets displayed.
“NumberList.h”:
- Define the template function necessary.
- Define the class “NumberList”.
- Declare the necessary structure variables.
- Define the constructor and destructor.
- Declare the necessary function prototype.
“NumberList.cpp”:
- Include the required header files.
- Declare the necessary function prototype and constants.
- Define the method named “nodeappend()”,
- Declare the necessary variables
- Validate the list using if statement to find the status of the list.
- Use loop to iterate for the contents present in the list and add node to the last of the list.
- Define the method named “viewlist()”,
- Declare the necessary variables.
- Use loop that iterates to display the elements present in the list.
- Define the method named “insertval()”,
- Declare the necessary variables.
- Validate the content of the list using if statement.
- After evaluation of the list the contents the values are inserted at the desired location.
- Loop is used to iterate for the values that are present, to validate whether the particular element is greater or lesser than the elements present in the list.
- After evaluation the value is inserted at the desired location.
- Define the method named “removenode()”,
- Declare the necessary variables.
- Validate the list using if statement in order to position the list based on the elements that is deleted from the list.
- Define the method named “~NumberList()”,
- Declare the necessary variables.
- Loop that iterates to delete the memory allocates.
- Define the method named “sortlist()”,
- Declare the necessary variables.
- Use loop to iterate for the value of the list.
- A condition statement to compare the elements of the list and return the sorted list.
- Define the method named “mergeval()”,
- Loop that is used to iterate to merge the values of the list based on the list values.
- Call the function “sortlist()” to sort the value after being merged.
- Define the method named “maximumnode()”,
- Return the head node to be the maximum node.
- Defined the method named “maxNode()”,
- Use a condition statement to validate the elements of the list to identify the maximum value that is present in the list.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Q13.
Python function that takes two lists and returns True if they have at least one common member.
C++ function Linked list
Write a function, to be included in an unsorted linked list class, called replaceItem, that will receive two parameters, one called olditem, the other called new item. The function will replace all occurrences of old item with new item (if old item exists !!) and it will return the number of replacements done.
def removeMultiples(x, arr) - directly remove the multiples of prime numbers (instead of just marking them) by creating a helper function. This recursive function takes in a number, n, and a list and returns a list that doesn’t contain the multiples of n.def createList(n) - a recursive function, createList(), that takes in the user input n and returns an array of integers from 2 through n (i.e. [2, 3, 4, …, n]). def Sieve_of_Eratosthenes(list) - a recursive function that takes in a list and returns a list of prime numbers from the input list.Template below:
def createList(n): #Base Case/s #ToDo: Add conditions here for base case/s #if <condition> : #return <value> #Recursive Case/s #ToDo: Add conditions here for your recursive case/s #else: #return <operation and recursive call>
#remove the line after this once all ToDo is completed return []
def removeMultiples(x, arr): #Base Case/s #TODO: Add conditions here for your…
Chapter 20 Solutions
Starting Out with C++ from Control Structures to Objects (9th Edition)
Ch. 20.2 - What happens if a recursive function never...Ch. 20.2 - What is a recursive functions base case?Ch. 20.2 - Prob. 20.3CPCh. 20.2 - What is the difference between direct and indirect...Ch. 20 - What is the base case of each of the recursive...Ch. 20 - What type of recursive function do you think would...Ch. 20 - Which repetition approach is less efficient, a...Ch. 20 - When should you choose a recursive algorithm over...Ch. 20 - Explain what is likely to happen when a recursive...Ch. 20 - The _____________ of recursion is the number of...
Ch. 20 - Prob. 7RQECh. 20 - Prob. 8RQECh. 20 - Prob. 9RQECh. 20 - Write a recursive function to return the number of...Ch. 20 - Write a recursive function to return the largest...Ch. 20 - #include iostream using namespace std; int...Ch. 20 - Prob. 13RQECh. 20 - #include iostream #include string using namespace...Ch. 20 - Iterative Factorial Write an iterative version...Ch. 20 - Prob. 2PCCh. 20 - Prob. 3PCCh. 20 - Recursive Array Sum Write a function that accepts...Ch. 20 - Prob. 5PCCh. 20 - Prob. 6PCCh. 20 - Prob. 7PCCh. 20 - Prob. 8PCCh. 20 - Prob. 9PCCh. 20 - Prob. 10PCCh. 20 - Prob. 11PCCh. 20 - Ackermanns Function Ackermanns Function is a...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Exercise, maxCylinderVolume F# system function such as min or methods in the list module such as List.map are not allowed Write a function maxCylinderVolume that takes a list of floating-point tuples that represent dimensions of a cylinder and returns the volume of the cylinder that has the largest volume. Each tuple has two floating point values that are both greater than zero. The first value is the radius r and the second value is the height h. The volume of the cylinder is computed using ??2h. The value π is represented in F# with System.Math.PI. If the list is empty, return 0.0. Examples: > maxCylinderVolume [(2.1, 3.4); (4.7, 2.8); (0.9, 6.1); (3.2, 5.4)];;val it : float = 194.3137888> maxCylinderVolume [(0.33, 0.66)];;val it : float = 0.2257988304arrow_forwardWrite the function splitList that takes the first argument list and splits it into two: a list of all the elements that are less than the head (the second argument), and a list of all the elements that are greater than or equal to the head (the third argument): listElementT splitList(listADT list, listADT* ItList, listADT* geList); Note that the head is returned by the function. a) Write this function as a recursive function. b) Write this function as a nonrecursive function.arrow_forward(Circular linked lists) This chapter defined and identified various operations on a circular linked list.a. Write the definitions of the class circularLinkedList and its member functions. (You may assume that the elements of the circular linked list are in ascending order.)b. Write a program to test various operations of the class defined in (a).arrow_forward
- determine if the following staements are True or False in java A list allows retrieval of information based on when the information was stored in the list. The iterator operation is required by the Iterable interface. A list allows retrieval of information based on the contents of the information.arrow_forwardY3 Using C++ To test your understanding of recursion, you are charged with creating a recursive, singly linked list. You will need to make the class yourself for this data structure. This repository already contains the List ADT and a driver that will create a TUI (terminal user interface) program that uses your data structure to generate a recursive art animation. Submissions that do not compile will receive a zero. You can work on this by yourself or with one other person. LinkedList This class will represent the data structure. Since we want this data structure to be generic, you need to make it a class template. Create two files for this class: a header file (LinkedList.hpp) and an implementation file (LinkedList.tpp). Place these two files in the src directory. It needs to inherit from the List abstract class using the public access specifier. The following are implementation notes for the class. The majority of methods need to be recursive and optimized via tail recursion…arrow_forwarddetemine if the following statement are true or false The iterator operation is required by the Iterable interface. A list allows retrieval of information based on the contents of the information.arrow_forward
- Define the function (doubleBubbleLst lst). This function should resolve to a list of sublists, where each sublist holds a single element from lst and all sublists from lst are also bubbled, so that every list and sublist has no atoms. This is the deep recursion version of bubbleLst. For example: (doubleBubbleLst '(1 2 (3 4)) ) resolves to '((1) (2) (( (3) (4) ))).arrow_forwardFill up the blanks with the appropriate information. The property is the only one that is needed for the basic Linked List class to function.arrow_forwardWrite a recursive function named Multiply2 that multiples by 2 and prints each element in a given list. For example: mylist=[1,2,3,4] Multiply2(mylist) prints: 2 4 6 8 Note: the function takes only one parameter, which is the list on which the operation will be performed.arrow_forward
- HELP Write C code that implements a soccer team as a linked list. 1. Each node in the linkedlist should be a member of the team and should contain the following information: What position they play whether they are the captain or not Their pay 2. Write a function that adds a new members to this linkedlist at the end of the list.arrow_forwardImplement a recursive function void deleteMax() on the IntList class (provided). The function will delete from the IntList the IntNode containing the largest value. If there are multiple nodes containing this largest value, only delete the 1st one. Be careful not to cause any memory leaks or dangling pointers. You may NOT use any kind of loop (must use recursion). You may NOT use global or static variables. You may NOT use any standard library functions. Ex: list: 5->7->1->16->4->16->3 list.deleteMax(); list: 5->7->1->4->16->3 IntList.h #ifndef __INTLIST_H__#define __INTLIST_H__ #include <ostream> using namespace std; struct IntNode {int value;IntNode *next;IntNode(int value) : value(value), next(nullptr) {}}; class IntList { private:IntNode *head; public: /* Initializes an empty list.*/IntList() : head(nullptr) {} /* Inserts a data value to the front of the list.*/void push_front(int val) {if (!head) {head = new IntNode(val);} else {IntNode…arrow_forwardWrite a struct Student that has member variables: (string) first name, (int) age and (double) fee. Write the functions as described in the class for the following purposes.1. Write a c++ function to create a dynamic sorted (in ascending order according to the age)doubly linked list, where the data component of each node is an instance of the structStudent.2. Write a function to insert the instances in the linked list. You also need to write a function to find the spot for insertion of the nodes.3. Write a function to remove the node from the linked list.4. Write a function to count the elements of the linked list.5. Write a function to determine check whether an element belongs to the linked list.6. Write a function to print the linked list (from head node) on the console.7. Write a function to print the linked list (from tail node) on the console. Implement the above functions as follows.Initially, the list must have five nodes that are the instances of the struct whose member…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
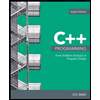
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
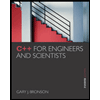
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr