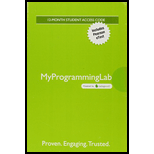
Concept explainers
(Quicksort) The recursive sorting technique called quicksort uses the following basic
- Partitioning Step: Take the first element of the unsorted array and determine its final location in the sorted array (i.e., all values to the left of the element in the array are less than the element’s value, and all values to the right of the element in the array are greater than the element’s value—we show how to do this below). We now have one value in its proper location and two unsorted sub-arrays.
- Recursion Step: Perform the Partitioning Step on each unsorted sub-array.
Each tune Step 1 is performed on a sub-array, another element is placed in its final location of the sorted array, and two unsorted sub-arrays are created. When a sub-array consists of one element, that sub-array must be sorted; therefore, that element is in its final location.
The basic algorithm seems simple enough, but how do we determine the final position of the first element of each sub-array? As an example, consider the following set of values (the element in bold is the partitioning element—it will be placed in its final location in the sorted array):
37 2 6 4 89 8 10 12 68 45
Starting from the rightmost element of the array, compare each element with 37 until an element less than 37 is found. Then swap 37 and that element. The first clement less than 37 is 12, so 37 and 12 are swapped. The values now reside in the array as follows:
12 2 6 4 89 8 10 37 68 45
Element 12 is in italics to indicate that it was just swapped with 37.
Starting from the left of the array, but beginning with the element after 12, compare each element with 37 until an clement greater than 37 is found. Then swap 37 and that element. The first element greater than 37 is 89, so 37 and 89 are swapped. The values now reside in the array as follows:
12 2 6 4 10 8 37 89 68 45
.Starting from the right, but beginning with the element before 89, compare each element with 37 until an element less than 37 is found. Then swap 37 and that element. The first element less than 37 is 10, so 37 and 10 are swapped. The values now reside in the array as follows:
12 2 6 4 10 8 37 89 68 45
Starting from the left, but beginning with the element after 10, compare each element with 37 until an element greater than 37 is found. Then swap 37 and that element. There are no more elements greater than 37, so when we compare 37 with itself, we know that 37 has been placed in its final location of the sorted array.
Once the partition has been applied to the array, there are two unsorted sub-arrays. The subarray with values less than 37 contains 12, 2, 6, 4, 10 and 8. The sub-array with values greater than 3 ' contains 89, 68 and 45. The sort continues with both sub-arrays being partitioned in the same manner as the original array.
Based on the preceding discussion, write recursive function quicksort to sort a single-sub- scripted integer array. The function should receive as arguments an integer array, a starting subscript and an ending subscript. Function partition should be called by quicksort to perform the partitioning step.

Want to see the full answer?
Check out a sample textbook solution
Chapter 20 Solutions
Mylab Programming With Pearson Etext -- Access Code Card -- For C++ How To Program (early Objects Version)
- Instructions Write a recursive function to implement the recursive algorithm of Exercise 16 (reversing the elements of an array between two indices). Also, write a program to test your function. Your program should prompt the user for the integers in the array, as well as the low index and then the high index. The instructions from Exercise 16 have been included below for your convenience: Suppose that intArray is an array of integers, and length specifies the number of elements in intArray. Also, suppose that low and high are two integers such that 0 <= low < length, 0 <= high < length, and low < high. That is, low and high are two indices in intArray. Write a recursive definition that reverses the elements in intArray between low and high. Textbook: C++ Programming: From Problem Analysis to Program Design 8th Edition Publisher: Cengage Learning Edition ISBN: 9781337102087arrow_forwardThe following recursive function returns the index of the minimum value of an array. Assume variable n is the size of the array. Modify the code so only a single recursive call is made when the second "if" statement is true.arrow_forwardCompilation Techniques a. Translate the three-address statement, the following expression: n = f((x+2), y) – 5; b. If A is an array of integers with width = 4; Translate with a three-address statement, the following expression: x = a[i] + 2arrow_forward
- Pointer arithmetic. Implement the following:a. Implement a print function with array and size parameters. This function should print the array using pointer arithmetic.b. Overload the print function to accept array, size and index parameters. If the index is between 1 and size-2 inclusive: Use pointer arithmetic to print: 1. the value at index 2. the value previous to the index 3. the value after the index c. Implement a sum function with array and size parameters. This function should return sum of the array, and use pointer arithmetic.d. Implement a average function with array and size parameters. This function shouldreturn average of the array, and use pointer arithmetic.e. Use provided main function to test your functions. ( Do not change the int main the question has provided. Included a snip of the int main function. Output has to be the same as shown in the snips)arrow_forwardFinish the swap function below using pointers and write the call to swap in the reverse function using the address of the array positions array[i] and array[size-i-1].using pointers. DON'T FORGET TO DECLARE THE PARAMETERS in the function. (Hint: if you can't figure it out with pointers, do it with the references #include <iostream>using namespace std; // Function to swap two ints using pointers// @param pointer to an int// @param pointer to an intvoid swap( ) {// TODO: Add code that swaps two integers using pointers } // Function to reverse arrayvoid reverse(int a[], int size) {for (int i = 0; i < size/2; i++) {// TODO: write the call to swap in the reverse function using the // address of the array positions array[i] and array[size-i-1].arrow_forward1-Write a C++ program to input elements in array and sort array elements in descending order. How to sort array in descending order in using pointers.Use function to print out the original and sorted arrays, Example Input Input size of array: 10 Input array elements: 20, 2, 10, 6, 52, 31, 0, 45, 79, 40 Output Array sorted in descending order: 79 ,52,45,40,31,20,10,6,2,0arrow_forward
- Hello, I am having trouble with my c++ homework. Implement and grow a dynamic array using pointer arithmetic. a) Use the provided main function (see below). b) Implement a populate function which stores values from 0 to size into the array p using pointer arithmetic to access array locations. c) Implement a print function which prints the values of the array p using pointer arithmetic. d) Implement a printMemory function which prints the memory addresses of all elements in array p using pointer arithmetic. e) Implement a grow function which resizes the existing array from the initial size to a new size using pointer arithmetic. f) Verify via the output that the new array is a distinct memory space from the original array. Main: Output Example:Use the following main function to test your program. (cannot change the int main provided) int main(…arrow_forward4. Write a program to find kth smallest element in unsorted array?You are given an unsorted array of numbers and k, you need to find the kth smallest number in the array. Forexample if given array is {1, 2, 3, 9, 4} and k=2 then you need to find the 2nd smallest number in the array,which is 2.arrow_forward5. Using c++, Write a function that determines the index of the second to the last occurrence of a target b in an integer array a. Return -1 if b is not in a or there is only 1 occurrence of b in a.arrow_forward
- 1-Write a JAVA program to delete duplicate elements from array. How to removeduplicate elements from array in JAVA programming. After performing delete operationthe array should only contain unique integer value. Logic to delete duplicate elementsfrom array.ExampleInputInput array elements: 10, 20, 10, 1, 100, 10, 2, 1, 5, 10OutputAfter removing all duplicate elementsElements of array are: 10, 20, 1, 100, 2, 5arrow_forward1. Rewrite the Bubble sort to use recursion. 2. Use the time(0) function to determine how many seconds it takes to sort a vector using the recursive method. 3. Use the time(0) function to determine how many seconds it takes to sort a vector using the non-recursive method described in the videos. Run the sort test on vectors containing random integers. You should sort vectors of the following sizes: 100 elements, 1000 elements, 5,000 elements, 10,000 elements and 50,000 elements. Was the recursive method able to work on vectors of each of those sizes? If not, explain why it errored.arrow_forward1-Write a C++ program to delete duplicate elements from array. How to remove duplicateelements from array in C++ programming. After performing delete operation the arrayshould only contain unique integer value. Logic to delete duplicate elements from array.ExampleInputInput array elements: 10, 20, 10, 1, 100, 10, 2, 1, 5, 10OutputAfter removing all duplicate elementsElements of array are: 10, 20, 1, 100, 2, 5arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
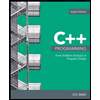
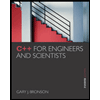