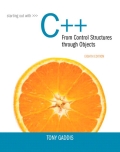
Starting Out with C++
8th Edition
ISBN: 9780133888201
Author: GADDIS
Publisher: PEARSON CUSTOM PUB.(CONSIGNMENT)
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 20, Problem 8PC
Program Plan Intro
Employee Tree
Program Plan:
BinaryTree.h:
- Create a template prefix and define the template class BinaryTree to perform the following functions:
- Declare the required variables.
- Declare the function prototypes.
- Define the no-argument generic constructor BinaryTree() to initialize the root value as null.
- Call the functions insertNode(),displayInOrder(), and searchNode().
- Define the generic function insert () to insert the node in pointed by the tree node pointer in a tree.
- Define the generic function insertNode() to create a new node and it is passed inside the insert() function to insert a new node into the tree.
- Define the generic function searchNode() to search the value in the tree. If it is present, then the function returns a pointer to the value member of the node otherwise it returns false.
- Define the generic function displayInOrder()to display the values in the subtree pointed by the node pointer.
Employee.h:
- Create the header file for employee class.
- Include the required header files.
- Create the Employee class.
- Declare the variables.
- Declare the friend function BinaryTree to pass the instance of employee class nodes.
- Create the constructor for Employee() class and assign the corresponding variables for employee identification number and name.
- Define the method setID() to assign the employee ID.
- Define the method setName() to assign the string to employee name.
- Define the method getID() to return the value of employee ID.
- Return the Boolean value using equal operator to check if the returned employee matches with the value.
- Using friend class return the employee id number and name using stream object.
Main.cpp:
- Include the required header files.
- In main() function,
- Declare the variable.
- Create the object for BinaryTree.
- Create the data for employee workers from 1 to 8 individually using the object.
- Call the function insertNode() to put the employee information into tree.
- Call the function displayInOrder() to display the tree in order.
- Prompt the user to enter the employee number.
- Then call the searchNode() function to search the employee in the tree and assign the information to the pointer.
- If the pointer is found then print the corresponding message otherwise print the error message.
- Return the values.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Text concordance using BST
1 Project description
Write a cross-reference program that constructs a binary search tree (any type, including splay tree) with all words included from a text file and records the positions (word or line numbers) on which these words were used. These line numbers should be stored on linked lists associated with the nodes of the tree. After the input file has been processed, print in alphabetical order all words of the text file along with the corresponding list of numbers of the lines in which the words occur.
2 What to turn in
You will turn in
a short written report containing: A description of the significant choices/issues in the design of your code.
The source-code of your program.
3 Coding standards
A percentage of your grade will be based on the quality of your code, so pay attention to it. Discuss changes (if any) you made to programs presented in class. Take extra care in documenting the code you are implementing on your…
C++ DATA STRUCTURES
Implement the TNode and Tree classes. The TNode class will include a data item name of type string,which will represent a person’s name. Yes, you got it right, we are going to implement a family tree!Please note that this is not a Binary Tree.
Write the methods for inserting nodes into the tree,searching for a node in the tree, and performing pre-order and post-order traversals.The insert method should take two strings as input. The second string will be added as a child node tothe parent node represented by the first string.
Hint:
The TNode class will need to have two TNode pointers in addition to the name data member:TNode *sibling will point to the next sibling of this node, and TNode *child will represent the first child ofthis node. You see two linked lists here??? Yes! You’ll need to use the linked lists
Book{ AvlNode{ AvlTree{String title Book insert()int ID int height printTree()String author AvlNode left height()double price AvlNode right max()} } }From your understanding of AVL trees, implement the four different types of rotations and node swoping inside AvlTree.java; each question must be completed with inline Javadoc and/or comments:1. rotRight(AvlNode someNode) and returns AvlNode.2. rotLeft(AvlNode someNode) and returns AvlNode.3. doubleRotLeft(AvlNode someNode) and returns AvlNode. 4. doubleRotRight(AvlNode someNode) and returns AvlNode. 5. Complete a driver code under the main method
Chapter 20 Solutions
Starting Out with C++
Ch. 20.1 - Prob. 21.1CPCh. 20.1 - Prob. 21.2CPCh. 20.1 - Prob. 21.3CPCh. 20.1 - Prob. 21.4CPCh. 20.1 - Prob. 21.5CPCh. 20.1 - Prob. 21.6CPCh. 20.2 - Prob. 21.7CPCh. 20.2 - Prob. 21.8CPCh. 20.2 - Prob. 21.9CPCh. 20.2 - Prob. 21.10CP
Ch. 20.2 - Prob. 21.11CPCh. 20.2 - Prob. 21.12CPCh. 20 - Prob. 1RQECh. 20 - Prob. 2RQECh. 20 - Prob. 3RQECh. 20 - Prob. 4RQECh. 20 - Prob. 5RQECh. 20 - Prob. 6RQECh. 20 - Prob. 7RQECh. 20 - Prob. 8RQECh. 20 - Prob. 9RQECh. 20 - Prob. 10RQECh. 20 - Prob. 11RQECh. 20 - Prob. 12RQECh. 20 - Prob. 13RQECh. 20 - Prob. 14RQECh. 20 - Prob. 15RQECh. 20 - Prob. 16RQECh. 20 - Prob. 17RQECh. 20 - Prob. 18RQECh. 20 - Prob. 19RQECh. 20 - Prob. 20RQECh. 20 - Prob. 21RQECh. 20 - Prob. 22RQECh. 20 - Prob. 23RQECh. 20 - Prob. 24RQECh. 20 - Prob. 25RQECh. 20 - Prob. 1PCCh. 20 - Prob. 2PCCh. 20 - Prob. 3PCCh. 20 - Prob. 4PCCh. 20 - Prob. 5PCCh. 20 - Prob. 6PCCh. 20 - Prob. 7PCCh. 20 - Prob. 8PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Add to the BinarySearchTree class a member function string smallest() const that returns the smallest element of the tree. Provide a test program to test your function. #include <iostream>#include <string>using namespace std;class TreeNode{public:void insert_node(TreeNode* new_node);void print_nodes() const;bool find(string value) const;private:string data;TreeNode* left;TreeNode* right;friend class BinarySearchTree;};class BinarySearchTree{public:BinarySearchTree();void insert(string data);void erase(string data);int count(string data) const;void print() const;private:TreeNode* root;};BinarySearchTree::BinarySearchTree(){root = NULL;}void BinarySearchTree::print() const{if (root != NULL)root->print_nodes();}void BinarySearchTree::insert(string data){TreeNode* new_node = new TreeNode;new_node->data = data;new_node->left = NULL;new_node->right = NULL;if (root == NULL) root = new_node;else root->insert_node(new_node);}void TreeNode::insert_node(TreeNode*…arrow_forward(binary search tree c++)i am having issues with my code i am able to insert names into into the tree but they do not save(it says tree is empty). i will show you a picture of my output(black background) and a picture of the desired output(white background), i will also include my source main and .h class. //bt.cpp - file contains member functions#include "bt.h"#include <iostream>using namespace std;//ConstructorBT::BT(){ root = NULL;} //Insert new item in BSTvoid BT::insert(string d){ node* t = new node; node* parent; t->data = d; t->left = NULL; t->right = NULL; parent = NULL; if (isEmpty()) root = t; else { //Note: ALL insertions are as leaf nodes node* curr; curr = root; // Find the Node's parent while (curr) { parent = curr; if (t->data > curr->data) curr = curr->right; else curr = curr->left; }…arrow_forwardProgramming questions:typedef struct node { int data; struct node *left, *right;}BT;The node structure of the binary tree (BT) is shown above. There is a binary tree T, please complete the function: int degreeone(BT *T) to compute how many degree 1 node in the BT. The T is the root pointer, and the function shoule return the total number of degree 1 node.arrow_forward
- Cs questions. A binary search tree can be used to store arithmetic expressions. Change the BinarySearchTree class to implement the required operator precedence rules for the evaluation of expressions like 2 + 3 4 / 5.with C++arrow_forwardvoid F(node<int>&*root){if(root!=0){F(root->left); F(root->right); root->data=0; delete root;}root=0;} this code Select one: a. all of them b. Set all data items in the binary tree to 0 c. free the binary tree and returns it empty d. remove all items in the binary tree without changing its sizearrow_forwardvoid F(node<int>&*root){if(root!=0){F(root->left); F(root->right); root->data=0; delete root;}root=0;} this code Select one: a.all of them b.Set all data items in the binary tree to 0 c.free the binary tree and returns it empty d.remove all items in the binary tree without changing its sizearrow_forward
- void F(node<int>&*root){if(root!=0){F(root->left); F(root->right); root->data=0; delete root;}root=0;} this code Select one: a. free the binary tree and returns it empty b. Set all data items in the binary tree to 0 c. remove all items in the binary tree without changing its size d. all of themarrow_forwardJava Programming Language: 1. Make a class that accepts id numbers ranging from 1 to 29. 2. The id numbers are nodes of a binary tree. 3. Traverse the tree in inorder, preorder, and postorder and display the traversed values.arrow_forwardC PROGRAMMING •Write a program that counts how many lotto tickets each person has bought .•Create a sorted binary tree .•In the tree node you have to store first name, last name, number of tickets and references to two nodes. •Read the file lotto300k.txt into a binary tree. •Traverse the completed tree and print those customers that bought more than one ticket.arrow_forward
- JAVA PROGRAMMING LANGUAGE: 1. Create a class that accepts id numbers ranging from 1 to 29. 2. The id numbers are nodes of a binary tree. 3. Traverse the tree in inorder, preorder, and postorder and display the traversed values.arrow_forwardC++ Build a templated version a binary search tree using a linked implementation along with functions for insertion and deletion. You must use the textbook's BinarySearchTree ADT as the base for your code. (See attached file.) Of course, you will need to modify the code a bit. Build a function that searches for a given item in the BST in the tree. It must return the number of compares that it used before returning as well as an indicator telling whether the sought item was or was not in the tree. Build a driver than inserts 10K unique random ints into the BST. Then, use the search function to seek the 1st, 500th, 5,000th, and 10,000th integers that were inserted in the tree, reporting the number of compares for each item. Also seek at least 2 integers that are not in the tree, one integer must be larger than the largest value in the tree and one integer must be within the range of values that are stored within the tree. Report the number of compares for these searches as well.arrow_forward(Java) How can I implement a binary search tree in a program to store data, and use the delete method to trim the tree? The program should have a 'populate' button that obtains a string from the user, creates a sorted binary search tree of characters, and prints the tree using the DisplayTree class. It should also print the characters on one line in sorted order by traversing the tree in inorder fashion. The program should also have a 'Trim Tree' button that obtains a second line of input to delete characters from the tree, trimming the tree accordingly. It should ignore characters that are not in the tree, and only delete one character for each occurrence in the second line of input. When all characters from the second line have been deleted from the tree, the program should print the remaining characters in the tree using the DisplayTree class. The output should be labeled appropriately, and no spaces or commas should be used between tree elements in the inorder traversal.Here is the…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
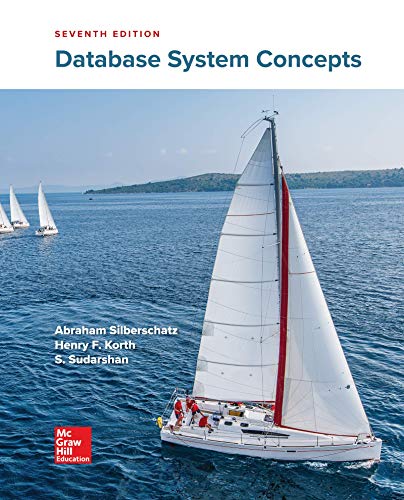
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
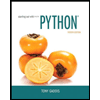
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
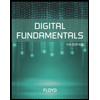
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
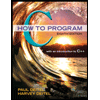
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
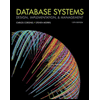
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
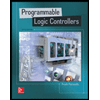
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education