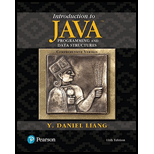
Concept explainers
(Geometry: Graham’s
/** Return the points that form a convex hull */
public static ArrayList<MyPoint> getConvexHull (double[][] s)
MyPoint is a static inner class defined as follows:
private static class MyPoint implements Comparable<MyPoint> {
double x, y;
MyPoint rightMostLowestPoint;
MyPoint (double x, double y) {
this.x = x; this.y = y;
}
public void setRightMostLowestPoint(MyPoint p) {
rightMostLowestPoint = p;
}
@Override
public int compareTo(MyPoint o) {
// Implement it to compare this point with point o
// angularly along the x-axis with rightMostLowestPoint
// as the center, as shown in Figure 22.10b. By implementing
// the Comparable interface, you can use the Array.sort
// method to sort the points to simplify coding.
}
}
Write a test program that prompts the user to enter the set size and the points, and displays the points that form a convex hull, Here is a sample run:
How many points are in the set? 6
Enter six points: 1 2.4 2.5 2 1.5 34.5 5.5 6 6 2.4 5.5 9
The convex hull is
(1.5, 34.5) (5.5, 9.0) (6.0, 2.4) (2.5, 2.0) (1.0, 2.4)

Want to see the full answer?
Check out a sample textbook solution
Chapter 22 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Additional Engineering Textbook Solutions
Web Development and Design Foundations with HTML5 (8th Edition)
Starting Out with Python (4th Edition)
Problem Solving with C++ (9th Edition)
Software Engineering (10th Edition)
Starting Out with C++: Early Objects (9th Edition)
Starting Out with Java: Early Objects (6th Edition)
- 12.10 LAB: All permutations of names- Java Write a program that lists all ways people can line up for a photo (all permutations of a list of Strings). The program will read a list of one word names (until -1), and use a recursive method to create and output all possible orderings of those names, one ordering per line. When the input is: Julia Lucas Mia -1 then the output is (must match the below ordering): Julia Lucas Mia Julia Mia Lucas Lucas Julia Mia Lucas Mia Julia Mia Julia Lucas Mia Lucas Juliaarrow_forwardPrint the following List in the reversed order using lambda / arrow operator. var array = new List <int>{ 8,2,2,24,5,7,5,3,2,10};arrow_forwardProblem (class MergeSalary)First, write a Java method merge that takes as parameter a non-empty double array representingemployees’ salaries. The method merges the values of every 2 adjacent elements. put themerged values at the beginning of the array, and put 0 in the remaining positions. Suppose thatthe original array will contain an even number of elements.Second, write two java methods readArray(int[]a) and printArray(int[]a) thatreads/writes the elements of an array passed in parameter from/to the standard input/output.Finally, Use the following main method to test your different methods:public class MergeSalary{ private static Scanner input = new Scanner(System.in); public static void main(String[] args) { double[] salaries = new double[10]; System.out.print("Enter 10 salaries: "); readArray(salaries); merge(salaries); System.out.print("Salaries after merge: "); printArray(salaries); }}arrow_forward
- 4. Write a program to find kth smallest element in unsorted array?You are given an unsorted array of numbers and k, you need to find the kth smallest number in the array. Forexample if given array is {1, 2, 3, 9, 4} and k=2 then you need to find the 2nd smallest number in the array,which is 2.arrow_forwardIn java Develop a function that accepts an array and returns true if the array contains any duplicate values or false if none of the values are repeated. Develop a function that returns true if the elements are in decreasing order and false otherwise. A “peak” is a value in an array that is preceded and followed by a strictly lower value. For example, in the array {2, 12, 9, 8, 5, 7, 3, 9} the values 12 and 7 are peaks. Develop a function that returns the number of peaks in an array of integers. Note that the first element does not have a preceding element and the last element is not followed by anything, so neither the first nor last elements can be peaks. Develop a function that finds the starting index of the longest subsequence of values that is strictly increasing. For example, given the array {12, 3, 7, 5, 9, 8, 1, 4, 6}, the function would return 6, since the subsequence {1, 4, 6} is the longest that is strictly increasing. Develop a function that takes a string…arrow_forwardQ1 / Write a program in C++ that reads a binary array of dimensions (383) containing integers, and then prints the elements of the third column horizontally on the screen after showing the necessary alert messagesarrow_forward
- **11.18** Java Solve (ArrayList of Character) Using the following header, create a method that produces an array list of characters from a string: toCharacterArray public static ArrayListCharacter> (String s) ToCharacterArray("abc"), for example, returns an array list containing the characters 'a', 'b', and 'c'.arrow_forwardQuestion1. Use recursion [ a. Write a Java method to find the largest element in the array using recursion. public static int largestelement(int[] arr,int n){ } b. Write a Java method to find a target in an array using binary search. (Note the array is sorted descendingly) public static int binarysearch(int[] arr, int target,int l,int r){ } c. Call the methods largestelement() and binarysearch() using the following array a=[13,10,9,8,4,3] and target=10 ANSWER: Output screen capture:arrow_forwardQ1: Write a program in Java that reads 10 integers and store them in AN ArrayList. Then, do the following : a) Print the ArrayList in the reverse order b) Sort the ArrayList, and display the data after sorting c) Print the largest and the smallest element in the ArrayListarrow_forward
- Do not use static variables to implement recursive methods. USING JAVA USING: // P4 public static int min(int [] a, int begin, int end) { } Implement a recursive method min that accepts an array and returns the minimum element in the array. The recursive step should divide the array into two halves and find the minimum in each half. Demonstrate the output of min on the array int [] a = { 2, 3, 5, 7, 11, 13, 17, 19, 47, 53, 59, 61, 67, 71, 73, 79, 83, 89, 97, 23, 29, 31, 37, 41, 43 }arrow_forwardJSK89 Write a java program to Move all zeroes to end of array?arrow_forward3. Implement a function called findLargestIndex which returns the index of the rowwith the largest sum.ex. int array[3][6] = {{3, 6, 8, 2, 4, 1}, // sum = 24{2, 4, 5, 1, 3, 4}, // sum = 19{1, 0, 9, 0, 1, 0}}; // sum = 11If the findLargestIndex function was called using this array, it would return 0, asit is returning the index of the row, not the sum.Note that the number of columns is required when passing a 2d array to a function.Assume that 2D arrays passed to this function will have 6 columns.Title line: int findLargestIndex(int array[][6], int rows, int columns);arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
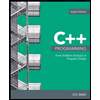