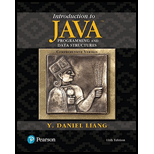
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
11th Edition
ISBN: 9780134670942
Author: Y. Daniel Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 25, Problem 25.15PE
Program Plan Intro
Program Plan:
- Include the required import statement.
- Define the main class.
- Define the main method using public static main.
- Allocate memory to the class “Test”.
- Define the “Test” class.
- Declare the object for the BST.
- Get the 10 integer numbers from the user and insert into the tree.
- Delete the first number in the tree.
- Display the paths from all the nodes.
- Define the “BST” class.
- Declare the required variables.
- Create a default BST class.
- Create a binary tree from an array of objects.
- Define the “search” method.
- Start the traverse from the root of the tree.
- If the search element is in the left subtree set that value in “current” variable otherwise set the “current” variable as right subtree value.
- Define the “insert” method.
- If the root is null create the tree otherwise insert the value into left or right subtree.
- Define the “createNewNode”
- Return the result of new node creations.
- Define the “inorder”
- Inorder traverse from the root.
- Define the protected “inorder” method
- Traverse the tree according to the inorder traversal concept.
- Define the “postorder”
- Postorder traverse from the root.
- Define the protected “postorder” method
- Traverse the tree according to the postorder traversal concept.
- Define the “preorder”
- Preorder traverse from the root.
- Define the protected “preorder” method
- Traverse the tree according to the preorder traversal concept.
- Define the “TreeNode” class
- Declare the required variables.
- Define the constructor.
- Define the “getSize” method.
- Return the size.
- Define the “getRoot” method
- Return the root.
- Define the “java.util.ArrayList” method.
- Create an object for the array list.
- If the “current” is not equal to null, add the value to the list.
- If the “current” is less than 0, set the “current” as left subtree element otherwise set the “current” as right subtree element.
- Return the list.
- Define the “delete” method.
- If the “current” is not equal to null, add the value to the list.
- If the “current” is less than 0, delete the “current” as left subtree element otherwise delete the “current” as right subtree element.
- Return the list.
- Define the “iterator” method.
- Call the “inorderIterator” and return the value.
- Define the “inorderIterator”
- Create an object for that method and return the value
- Define the “inorderIterator” class.
- Declare the variables.
- Define the constructor.
- Call the “inorder” method.
- Define the “inorder” method.
- Call the inner “inorder” method with the argument.
- Define the TreeNode “inorder” method.
- If the root value is null return the value, otherwise add the value into the list.
- Define the “hasNext” method
- If the “current” value is less than size of the list return true otherwise return false.
- Define the “next” method
- Return the list.
- Define the “remove” method.
- Call the delete method.
- Clear the list then call the “inorder” method.
- Define the “clear” method
- Set the values to the variables.
- Define the “getNode” method.
- Start the traversal from the root.
- If the “current” value is not null and the element is less than 0, set the “current” value as left subtree element.
- Otherwise the element is greater than 0, set the “current” value as right subtree element or return the element.
- Define the “isLeaf” method
- Start the traversal from the root.
- Return the value if “node” is not equal to null and left and right subtree value is equal to null.
- Define “getPath” method.
- Start the traversal from the root.
- Create an object for the array list.
- If the “temp” value is not null add the element into the list.
- Assign the parent value to the “temp” variable.
- Return the list.
- Define the interface.
- Declare the required methods.
- Define the required methods.
- Define the main method using public static main.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
BST - Binary Search Tree
- implement a BSTNode ADT with a data attribute and two pointer attributes, one for the left child and the other for the right child. Implement the usual getters/setters for these attributes
-implement a BST as a link-based ADT whose data will be Dollar objects - the data will be inserted based on the actual money value of your Dollar objects as a combination of the whole value and fractional value attributes.
- BST, implement the four traversal methods as well as methods for the usual search, insert, delete, print, count, isEmpty, empty operations and any other needed.
-
BST - Binary Search Tree
- implement a BSTNode ADT with a data attribute and two-pointer attributes, one for the left child and the other for the right child. Implement the usual getters/setters for these attributes
-implement a BST as a link-based ADT whose data will be Dollar objects - the data will be inserted based on the actual money value of your Dollar objects as a combination of the…
Notes:-Give priority to the left child over the right.
-Use a space to separate the node values!
1. List the first 5 nodes, in order, visited by a preorder traversal.
2. List the first 5 nodes, in order, visited by an inorder traversal.
3. List the first 5 nodes, in order, visited by a postorder traversal.
4. List the first 5 nodes, in order, visited by a level order traversal.
Answer the given question with a proper explanation and step-by-step solution.
Write a recursive method(Code ) static void mirrorTree(node root) This will take a tree as input and then change the tree such that it becomes a mirror of itself. We have the following methods implemented(you can use them and assume we coded somewhere else): getLeft(), getRight(), setLeft(), setRight(), getValue(). All the values in a node are integers. [Hint: Use them, and think how you would write when the tree only has 2 children ] Example:
Chapter 25 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Ch. 25.2 - Prob. 25.2.1CPCh. 25.2 - Prob. 25.2.2CPCh. 25.2 - Prob. 25.2.3CPCh. 25.2 - Prob. 25.2.4CPCh. 25.2 - Prob. 25.2.5CPCh. 25.3 - Prob. 25.3.1CPCh. 25.3 - Prob. 25.3.2CPCh. 25.3 - Prob. 25.3.3CPCh. 25.3 - Prob. 25.3.4CPCh. 25.4 - Prob. 25.4.1CP
Ch. 25.4 - Prob. 25.4.2CPCh. 25.4 - Prob. 25.4.3CPCh. 25.4 - Prob. 25.4.4CPCh. 25.4 - Prob. 25.4.5CPCh. 25.5 - Prob. 25.5.1CPCh. 25.5 - Prob. 25.5.2CPCh. 25.5 - Prob. 25.5.3CPCh. 25.5 - Prob. 25.5.4CPCh. 25.5 - Prob. 25.5.5CPCh. 25.6 - Prob. 25.6.1CPCh. 25.6 - Prob. 25.6.2CPCh. 25.6 - Prob. 25.6.3CPCh. 25.6 - How do you replace lines 9499 in Listing 25.11...Ch. 25 - Prob. 25.1PECh. 25 - (Implement inorder traversal without using...Ch. 25 - (Implement preorder traversal without using...Ch. 25 - (Implement postorder traversal without using...Ch. 25 - Prob. 25.6PECh. 25 - Prob. 25.7PECh. 25 - (Implement bidirectional iterator) The...Ch. 25 - Prob. 25.9PECh. 25 - Prob. 25.10PECh. 25 - Prob. 25.11PECh. 25 - (Test BST) Design and write a complete test...Ch. 25 - (Modify BST using Comparator) Revise BST in...Ch. 25 - Prob. 25.15PECh. 25 - (Data compression: Huffman coding) Write a program...Ch. 25 - Prob. 25.17PECh. 25 - (Compress a file) Write a program that compresses...Ch. 25 - (Decompress a file) The preceding exercise...
Knowledge Booster
Similar questions
- Can help in Java? Qustion : Using Binary search tree write a Java program to Insert and print the element in (in-Order traversal ), then search the elements (a and z) in the tree… provided that user enters the values.arrow_forward* void addSpouse(string m, string A method that takes the names of the member and spouse, searches for the member by the name, and update the node with the spouse name.arrow_forwardCan help in Java?? Using Binary search tree write a Java program to Insert the elements from user and print the element in (in-Order traversal ), then search the elements (a and z) in the treearrow_forward
- - Give Java Code that finds the number of children using the sequential representation, Linked Implementations Using Array of Child Pointers implementation, linked implementation left-child/right-sibling.arrow_forwardCan help in Java? Question: Using Binary search tree write a Java program to Insert and print the element in (in-Order traversal ), then search the elements (a and z) in the tree. using the following tree:arrow_forwardWrite a program to create tree of the following elements and display the tree by using Inorder, Preorder, and Postorder techniques. 0, 2, 4, 6, 8, 10, 12, 14, 16, 18 - For Inorder, Preorder, and Postorder, you have to write separate methods.arrow_forward
- Do the complete version of the user-defined Tree interface for the code given below (meaning you need to add the code for all methods that were not coded and have a "Left as an exercise" comment). import java.util.Collection; public interface Tree<E> extends Collection<E> { /** Return true if the element is in the tree */ public boolean search(E e); /** Insert element e into the binary tree * Return true if the element is inserted successfully */ public boolean insert(E e); /** Delete the specified element from the tree * Return true if the element is deleted successfully */ public boolean delete(E e); /** Get the number of elements in the tree */ public int getSize(); /** Inorder traversal from the root*/ public default void inorder() { } /** Postorder traversal from the root */ public default void postorder() { } /** Preorder traversal from the root */ public default void preorder() { } @Override /** Return true if the tree is empty */ public default…arrow_forwardBinary Search Tree Implementation(Java)In the binary search tree implementation, which is completely unrelated to the linked list implementation above, you will use an unbalanced binary search tree. Since duplicates are allowed, each node will have to store a singly-linked list of all the entries that are considered identical. Two values are identical if the comparator returns 0, even if the objects are unequal according to the equals method. A sketch of the private representation looks something like this: private Comparator<? super AnyType> cmp;private Node<AnyType> root = null; private void toString( Node<AnyType> t, StringBuffer sb ){ ... the recursive routine to be called by toString ... } private static class Node<AnyType>{private Node<AnyType> left;private Node<AnyType> right;private ListNode<AnyType> items; private static class ListNode<AnyType>{private AnyType data;private ListNode<AnyType> next; public ListNode( AnyType d,…arrow_forwardCode in Java Code with comments and output screenshot Q. Write a method to count the number of leaf nodes in binary treearrow_forward
- Create a lazy elimination deletion function for the AVLTree class.There are several approaches you can take, but one of the simplest is to add a Boolean property to the Node class that indicates whether or not the node is designated for deletion. This variable must then be considered by your other techniques.arrow_forwardonly c here is "BinaryNode.java" // BinaryNode class; stores a node in a tree. // // CONSTRUCTION: with (a) no parameters, or (b) an Object, // or (c) an Object, left child, and right child. // // *******************PUBLIC OPERATIONS********************** // int size( ) --> Return size of subtree at node // int height( ) --> Return height of subtree at node // void printPostOrder( ) --> Print a postorder tree traversal // void printInOrder( ) --> Print an inorder tree traversal // void printPreOrder( ) --> Print a preorder tree traversal // BinaryNode duplicate( )--> Return a duplicate tree /** * Binary node class with recursive routines to * compute size and height. */ class BinaryNode { public BinaryNode( ) { this( 0, null, null ); } public BinaryNode( int theElement, BinaryNode lt, BinaryNode rt ) { element = theElement; left = lt; right = rt; } /** * Return the size of the binary tree rooted at t. */ public static int size( BinaryNode t ) { if( t == null ) return…arrow_forwardUse java or python to solve Note: 1) Assume that the Node class and Linked Lists constructors are already written. You ONLY need to write the revinsert0 method/function 2) No need to write the Tester Class. 3) Use of Built in Method is not allowed except count(). You must take an inplace approach and modify the given list. 5) You cannot use arrays to solve this problemarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
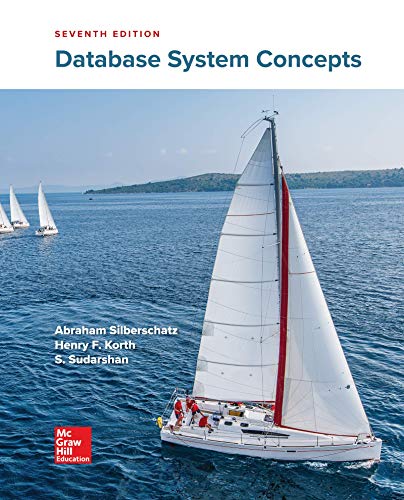
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
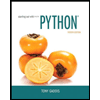
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
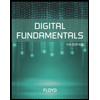
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
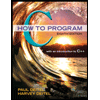
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
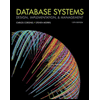
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
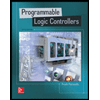
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education