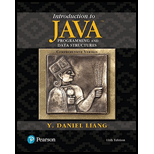
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
11th Edition
ISBN: 9780134670942
Author: Y. Daniel Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 28, Problem 28.10PE
Program Plan Intro
Find the shortest path
Program Plan:
Exercise.java:
- Import the required packages.
- Create a class “Exercise”:
- Define the main method
- Prompt user to enter the file.
- Validate whether the file exists or not.
- Prompt user to enter the vertices.
- Loop that read the contents of the file.
- The token that are present in the file are removed.
- Add the edges and vertices of the graph into the list.
- New graph gets created
- Perform the breadth first search for the graph.
- Display the path of the graph.
- Define the main method
UnweightedGraph.java:
- Import the required packages.
- Create a class “UnweightedGraph”:
- New list for the vertices gets created.
- New list for the neighbor node gets created.
- Create an empty constructor.
- Method to create new graph gets created and adjacency list gets created.
- Method to create an adjacency list gets created.
- Method to return the size of the vertices.
- Method to return the index of the vertices gets defined.
- Method to gets the neighbor node gets defined.
- Method to return the degree of the vertices gets created.
- Method to print the Edges gets created.
- New to clear the graph gets created.
- Method to add vertex gets created.
- Method to add edge gets created.
- Method to perform the depth first search gets defined.
- Method to perform breadth first search gets defined.
- Search tree gets returned.
- Create a class “SearchTree”
- Define the method to return the root.
- Method to return the parent of the vertices
- Method to return the search order gets defined.
- Method to return the number of vertices found gets defined.
- Method to get the path of the vertices gets defined.
- Loop to validate the path gets defined.
- Path gets returned.
- Method to print the path gets defined.
- Method to print the tree gets defined.
- Display the edge.
- Display the root.
- Condition to validate the parent node to display the vertices gets created.
Graph.java:
- A graph interface gets created.
- Method to return the size gets defined.
- Method to return the vertices gets defined.
- Method to return the index gets created.
- Method to get the neighbor node gets created.
- Method to get the degree gets created.
- Method to print the edges.
- Method to clear the node gets created.
- Method to add the edges, add vertex gets created.
- Method to remove the vertices gets defined.
- Method for the depth first search gets defined.
- Method for the breadth first search gets defined.
Edge.java
- Create a class “Edge”
- Define and declare the required variables.
- Constructor gets defined.
- Method that defines Boolean objects gets defined.
- Return the value after validating the vertices.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
What is the output of following code segment?
- Write a for loop with index i that iterates over all consecutive integers from 4 to 10 and prints the current loop index to screen for each value of i. As always, be careful with indentation and use proper python syntax.
- Same question as above, but add a logical (if) statement inside the loop that prints both the loop index and the message "is greater than 6" or "is less than or equal to 6" for the appropriate indices. Hint, use the syntax print(i, "string") to print both the loop index and the string at the same time.
- Write a for loop with index i that iterates over all consecutive integers from 4 to 10 and prints the current loop index to screen for each value of i. As always, be careful with indentation and use proper python syntax.
- Same question as above, but add a logical (if) statement inside the loop that prints both the loop index and the message "is greater than 6" or "is less than or equal to 6" for the appropriate indices. Hint, use the syntax print(i, "string") to print both the loop index and the string at the same time.
- Write the same for loop as in the first question above, but modify it to only print the loop index when i is not equal to 8.
Chapter 28 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Ch. 28.2 - What is the famous Seven Bridges of Knigsberg...Ch. 28.2 - Prob. 28.2.2CPCh. 28.2 - Prob. 28.2.3CPCh. 28.2 - Prob. 28.2.4CPCh. 28.3 - Prob. 28.3.1CPCh. 28.3 - Prob. 28.3.2CPCh. 28.4 - Prob. 28.4.1CPCh. 28.4 - Prob. 28.4.2CPCh. 28.4 - Show the output of the following code: public...Ch. 28.4 - Prob. 28.4.4CP
Ch. 28.5 - Prob. 28.5.2CPCh. 28.6 - Prob. 28.6.1CPCh. 28.6 - Prob. 28.6.2CPCh. 28.7 - Prob. 28.7.1CPCh. 28.7 - Prob. 28.7.2CPCh. 28.7 - Prob. 28.7.3CPCh. 28.7 - Prob. 28.7.4CPCh. 28.7 - Prob. 28.7.5CPCh. 28.8 - Prob. 28.8.1CPCh. 28.8 - When you click the mouse inside a circle, does the...Ch. 28.8 - Prob. 28.8.3CPCh. 28.9 - Prob. 28.9.1CPCh. 28.9 - Prob. 28.9.2CPCh. 28.9 - Prob. 28.9.3CPCh. 28.9 - Prob. 28.9.4CPCh. 28.10 - Prob. 28.10.1CPCh. 28.10 - Prob. 28.10.2CPCh. 28.10 - Prob. 28.10.3CPCh. 28.10 - If lines 26 and 27 are swapped in Listing 28.13,...Ch. 28 - Prob. 28.1PECh. 28 - (Create a file for a graph) Modify Listing 28.2,...Ch. 28 - Prob. 28.3PECh. 28 - Prob. 28.4PECh. 28 - (Detect cycles) Define a new class named...Ch. 28 - Prob. 28.7PECh. 28 - Prob. 28.8PECh. 28 - Prob. 28.9PECh. 28 - Prob. 28.10PECh. 28 - (Revise Listing 28.14, NineTail.java) The program...Ch. 28 - (Variation of the nine tails problem) In the nine...Ch. 28 - (4 4 16 tails problem) Listing 28.14,...Ch. 28 - (4 4 16 tails analysis) The nine tails problem in...Ch. 28 - (4 4 16 tails GUI) Rewrite Programming Exercise...Ch. 28 - Prob. 28.16PECh. 28 - Prob. 28.17PECh. 28 - Prob. 28.19PECh. 28 - (Display a graph) Write a program that reads a...Ch. 28 - Prob. 28.21PECh. 28 - Prob. 28.22PECh. 28 - (Connected rectangles) Listing 28.10,...Ch. 28 - Prob. 28.24PECh. 28 - (Implement remove(V v)) Modify Listing 28.4,...Ch. 28 - (Implement remove(int u, int v)) Modify Listing...
Knowledge Booster
Similar questions
- Please, solve the following problem and explain how the loop works .arrow_forwardUsing the following instruction below, write a header class for orderedLinkedList with a function of search,insert, insertFirst, insertLast and deleteNode. Use the library to write a c++ program to show an operation on an ordered linked list. - include the orderedLinkedList.h library in the codes - Create main function and include your information details - initialize a list1 and list2 as orderedLinkedList type - Declare a variable num as integer data type - Print out an instruction for user to input a numbers ending with -1 - Get a numbers from user input - Use a while loop to insert the numbers into list1 ending with -1 - Assign the list2 to list1 - Print out the current elements in List 1 and 2 - Get user input for the number to be deleted - Delete the number requested by user in list2 - Print out the elements in the list1 and list2 after delete operationarrow_forwardAnswer the given question with a proper explanation and step-by-step solution. 1- Write code that does the following: opens an output file with the filename number_list.txt, uses a loop to write the numbers 1 through 100 to the file, then closes the file.arrow_forward
- Write in C++. Description: wzip and wunzip class overview: The next tools you will build come in a pair, because one (wzip) is a file compression tool, and the other (wunzip) is a file decompression tool. The type of compression used here is a simple form of compression called run-length encoding (RLE). RLE is quite simple: when you encounter n characters of the same type in a row, the compression tool (wzip) will turn that into the number n and a single instance of the character. Thus, if we had a file with the following contents: aaaaaaaaaabbbb the tool would turn it (logically) into: 10a4b The exact format of the compressed file is quite important; here, you will write out a 4-byte integer in binary format followed by the single character in ASCII. Thus, a compressed file will consist of some number of 5-byte entries, each of which is comprised of a 4-byte integer (the run length) and the single character. To write out an integer in binary format (not ASCII), you…arrow_forwardIn C++, my program reads from a file, each line of the text file ends in a 1 or 0. How do you make a function that updates the value in the text file to change to from 1 to 0 or 0 to 1?arrow_forwardWrite the generated code sequence for the following basic block:arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
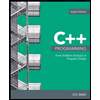
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning