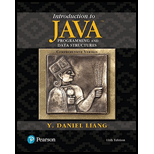
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
11th Edition
ISBN: 9780134670942
Author: Y. Daniel Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 28, Problem 28.9PE
Program Plan Intro
Get bipartite sets
Program Plan:
Exercise.java:
- Import the required packages.
- Create a class “Exercise”:
- Define the main method
- Define and declare the required vertices.
- Define and declare the required edges.
- New graph gets created for the defined edges and vertices.
- Display the cycle that is found.
- Define the main method
- Define and create the class “UnweightedGraphGetBipartiteSets”
- Create a new constructor.
- New graph gets using the constructor.
- Method to “cloneEdges” gets created.
- New list gets created.
- Loop that iterates to add the edges into the list.
- A neighbor copy of the edges is added.
- Returns the result of the neighbor copy.
- Method “getBipartite” gets created.
- New list is created.
- Loop that iterates to add the elements into the list.
- New list that contains the neighbor node, clone edges are created.
- Loop that iterates to assign the value to the parent node and depth is defined.
- New queue gets defined.
- Mark visited node.
- Loop that iterates to validate the size of the vertices to add the vertices are defined.
- Loop that validate the whether the queue is empty.
- Add the neighbor edge into the queue.
- Validate the visited node using if condition.
- Add values to the queue
- Assign the depth value and parent value.
- Return null if the does not contain bipartite.
- New list for storing the bipartite value gets created.
- Loop that iterates to validate the depth.
- Return the bipartite list after validating using if condition.
UnweightedGraph.java:
- Import the required packages.
- Create a class “UnweightedGraph”:
- New list for the vertices gets created.
- New list for the neighbor node gets created.
- Create an empty constructor.
- Method to create new graph gets created and adjacency list gets created.
- Method to create an adjacency list gets created.
- Method to return the size of the vertices.
- Method to return the index of the vertices gets defined.
- Method to gets the neighbor node gets defined.
- Method to return the degree of the vertices gets created.
- Method to print the Edges gets created.
- New to clear the graph gets created.
- Method to add vertex gets created.
- Method to add edge gets created.
- Method to perform the depth first search gets defined.
- Method to perform breadth first search gets defined.
- Search tree gets returned.
- Create a class “SearchTree”
- Define the method to return the root.
- Method to return the parent of the vertices
- Method to return the search order gets defined.
- Method to return the number of vertices found gets defined.
- Method to get the path of the vertices gets defined.
- Loop to validate the path gets defined.
- Path gets returned.
- Method to print the path gets defined.
- Method to print the tree gets defined.
- Display the edge.
- Display the root.
- Condition to validate the parent node to display the vertices gets created.
Graph.java:
- A graph interface gets created.
- Method to return the size gets defined.
- Method to return the vertices gets defined.
- Method to return the index gets created.
- Method to get the neighbor node gets created.
- Method to get the degree gets created.
- Method to print the edges.
- Method to clear the node gets created.
- Method to add the edges, add vertex gets created.
- Method to remove the vertices gets defined.
- Method for the depth first search gets defined.
- Method for the breadth first search gets defined.
Edge.java
- Create a class “Edge”
- Define and declare the required variables.
- Constructor gets defined.
- Method that defines Boolean objects gets defined.
- Return the value after validating the vertices.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Try to do the same question with Map Array
please complete the following in JAVA
Implement the graph ADT using the adjacency list structure.
thanks! also posting a similar question for adjacency matrix. have a good day!
TASK 7. Generic solution (Methods with Variable-Length Arguments). Review varargs, Implement the following code, test it with different input, make sure it runs without errors.
Chapter 28 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Ch. 28.2 - What is the famous Seven Bridges of Knigsberg...Ch. 28.2 - Prob. 28.2.2CPCh. 28.2 - Prob. 28.2.3CPCh. 28.2 - Prob. 28.2.4CPCh. 28.3 - Prob. 28.3.1CPCh. 28.3 - Prob. 28.3.2CPCh. 28.4 - Prob. 28.4.1CPCh. 28.4 - Prob. 28.4.2CPCh. 28.4 - Show the output of the following code: public...Ch. 28.4 - Prob. 28.4.4CP
Ch. 28.5 - Prob. 28.5.2CPCh. 28.6 - Prob. 28.6.1CPCh. 28.6 - Prob. 28.6.2CPCh. 28.7 - Prob. 28.7.1CPCh. 28.7 - Prob. 28.7.2CPCh. 28.7 - Prob. 28.7.3CPCh. 28.7 - Prob. 28.7.4CPCh. 28.7 - Prob. 28.7.5CPCh. 28.8 - Prob. 28.8.1CPCh. 28.8 - When you click the mouse inside a circle, does the...Ch. 28.8 - Prob. 28.8.3CPCh. 28.9 - Prob. 28.9.1CPCh. 28.9 - Prob. 28.9.2CPCh. 28.9 - Prob. 28.9.3CPCh. 28.9 - Prob. 28.9.4CPCh. 28.10 - Prob. 28.10.1CPCh. 28.10 - Prob. 28.10.2CPCh. 28.10 - Prob. 28.10.3CPCh. 28.10 - If lines 26 and 27 are swapped in Listing 28.13,...Ch. 28 - Prob. 28.1PECh. 28 - (Create a file for a graph) Modify Listing 28.2,...Ch. 28 - Prob. 28.3PECh. 28 - Prob. 28.4PECh. 28 - (Detect cycles) Define a new class named...Ch. 28 - Prob. 28.7PECh. 28 - Prob. 28.8PECh. 28 - Prob. 28.9PECh. 28 - Prob. 28.10PECh. 28 - (Revise Listing 28.14, NineTail.java) The program...Ch. 28 - (Variation of the nine tails problem) In the nine...Ch. 28 - (4 4 16 tails problem) Listing 28.14,...Ch. 28 - (4 4 16 tails analysis) The nine tails problem in...Ch. 28 - (4 4 16 tails GUI) Rewrite Programming Exercise...Ch. 28 - Prob. 28.16PECh. 28 - Prob. 28.17PECh. 28 - Prob. 28.19PECh. 28 - (Display a graph) Write a program that reads a...Ch. 28 - Prob. 28.21PECh. 28 - Prob. 28.22PECh. 28 - (Connected rectangles) Listing 28.10,...Ch. 28 - Prob. 28.24PECh. 28 - (Implement remove(V v)) Modify Listing 28.4,...Ch. 28 - (Implement remove(int u, int v)) Modify Listing...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- java program java method: Write a method replace to be included in the class KWLinkedList (for doubly linked list) that accepts two parameters, searchItem and repItem of type E. The method searches for searchItem in the doubly linked list, if found then replace it with repItem and return true. If the searchItem is not found in the doubly linked list, then insert repItem at the end of the linked list and return false. Assume that the list is not empty. You can use ListIterator and its methods to search the searchItem in the list and replace it with repItem if found. Do not call any method of class KWLinkedList to add a new node at the end of the list. Method Heading: public boolean replace(E searchItem, E repItem) Example: searchItem: 15 repItem: 17 List (before method call): 9 10 15 20 4 5 6 List (after method call) : 9 10 17 20 4 5 6arrow_forwardModify the generic Pair.java class in two ways: Make it so that both values have the same type Add a method swap that swaps the first and second elements of the pair Examples:Pair<Integer> intPair = new Pair<>(17, 19);intPair.getFirst(); // returns 17intPair.getSecond(); // returns 19intPair.swap();intPair.getFirst(); // returns 19intPair.getSecond(); // returns 17Pair<String> strPair = new Pair<>("Erick", "Valdez");strPair.getFirst(); // returns "Erick"strPair.getSecond(); // returns "Valdez"strPair.swap();strPair.getFirst(); // returns "Valdez"strPair.getSecond(); // returns "Erick" Pair.java /*** This class collects a pair of elements of different types.*/public class Pair<T, S> {private T first;private S second;/*** Constructs a pair containing two given elements.* * @param firstElement the first element* @param secondElement the second element*/public Pair(T firstElement, S secondElement) {first = firstElement;second = secondElement;}/*** Gets…arrow_forwardprogram e an arraylist from user , d iterate over this using iterator and printarrow_forward
- Write the recursive method for adding a node in a linked list.arrow_forwardInterpreter.java is missing these methods in the code so make sure to add them: -print, printf: Exist, marked as variadic, call Java functions -getline and next: Exist and call SplitAndAssign -gsub, match, sub, index, length, split, substr, tolower, toupper: Exist, call Java functions, correct return Below is interpreter.java import java.util.ArrayList; import java.util.HashMap; import java.util.List; public class Interpreter { private HashMap<String, InterpreterDataType> globalVariables; private HashMap<String, FunctionDefinitionNode> functions; private class LineManager { private List<String> lines; private int currentLineIndex; public LineManager(List<String> inputLines) { this.lines = inputLines; this.currentLineIndex = 0; } public boolean splitAndAssign() { if (currentLineIndex < lines.size()) { String currentLine = lines.get(currentLineIndex);…arrow_forward- Write a Polynomial class that has methods for creating a polynomial, reading and writing a polynomial, and adding a pair of polymomials - In order to add 2 polynomials, traverse both lists, If a particular exponent value is present in either one, it should also be present In the resulting polynomial unless its coefficient is zero. java submit as a text dont use others answers please attach screenshot of output Thank you!!!arrow_forward
- Q1 b) Briefly explain what happens when insertion is performed in Arraylist and Linkedlistarrow_forward* void addSpouse(string m, string A method that takes the names of the member and spouse, searches for the member by the name, and update the node with the spouse name.arrow_forwardConsider Bag, SinglyLinkedList, and DoublyLinkedLists classes for integers. Implement and test the following methods: 1. Write a method in Bag class for integers that finds the number of odd and even numbers in the list. 5. Write a method in DoublyLinkedList class that swaps the first and last nodes in the list. 6. Write a Main class to test all these methods.arrow_forward
- How can you tell whether the performance of an ArrayList is satisfactory?arrow_forwardpackage circularlinkedlist;import java.util.Iterator; public class CircularLinkedList<E> implements Iterable<E> { // Your variablesNode<E> head;Node<E> tail;int size; // BE SURE TO KEEP TRACK OF THE SIZE // implement this constructorpublic CircularLinkedList() {} // I highly recommend using this helper method// Return Node<E> found at the specified index// be sure to handle out of bounds casesprivate Node<E> getNode(int index ) { return null;} // attach a node to the end of the listpublic boolean add(E item) {this.add(size,item);return false; } // Cases to handle// out of bounds// adding to empty list// adding to front// adding to "end"// adding anywhere else// REMEMBER TO INCREMENT THE SIZEpublic void add(int index, E item){ } // remove must handle the following cases// out of bounds// removing the only thing in the list// removing the first thing in the list (need to adjust the last thing in the list to point to the beginning)// removing the last…arrow_forward14.12 NVCC Lab: Count number of subsets of a graph A graph is made up of a set of nodes called vertices and a set of lines called edges that connect the nodes. Informally, a connected component of a graph is a subset in which each pair of nodes is connected with each other via a path. For example, the following graph consists of two connected components (subsets). For this assignment, you are required to find out the number of connected components in a graph as specified below: implement the following method: public static int countConnectedComponents(String fileName): where fileName is the input data file representing a graph: each line in the data file contains two numbers representing a pair of vertices that are connected (an edge between these two nodes exists). For example, given the following data file: 1 2 1 3 2 3 4 5 6 8 6 7 *Code must be written in JAVAarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
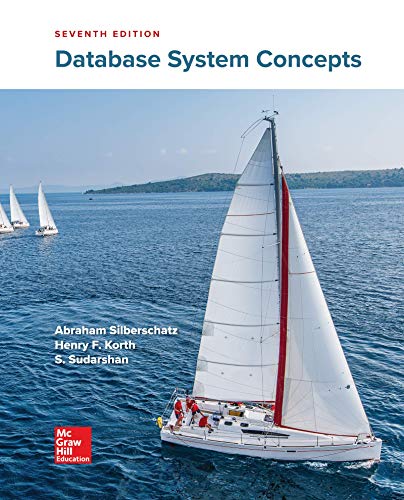
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
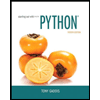
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
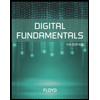
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
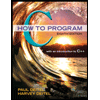
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
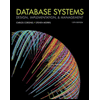
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
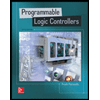
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education