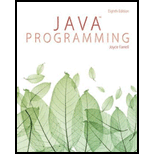
Explanation of Solution
a.
CarlysEventPriceWithMethods.java
//import the required packages
import java.util.Scanner;
//define a method CarlysEventPriceWithMethods
public class CarlysEventPriceWithMethods
{
//Scanner object
private static Scanner sc = new Scanner(System.in);
//define a method getEventNum()
public static String getEventNum()
{
//print the statement
System.out.println("Enter event number: ");
//scan the values
return sc.next();
}
//define the method getNoGuests
public static int getNoOfGuests()
{
//print the statement
System.out.println("Enter no of guests: ");
//return the value scanned
return sc.nextInt();
}
//define the method displayMotto()
public static void displayMotto(Event e)
{
//prin the values
System.out.println(String.format("%26s", " ").replace(" ", "*"));
System.out.printf("* %22s *", " ");
System.out.println("\n* Carly’s Catering *");
System.out.printf("* %22s *\n", " ");
System.out.println(String.format("%26s", " ").replace(" ", "*"));
System.out.println("Event No: " + e.getEventNum());
System.out.println("No. of guests:" + e.getNoOfGuest());
System.out.println("Price per guest: $" + Event.PRICE_PER_GUEST);
//call the method computePrice
computePrice(e);
}
//define the method computePrice
public static void computePrice(Event e)
{
//print the values
System.out.println("Price: $" + e.getPrice());
System.out.println("Large event: " + ((e.getNoOfGuest() > Event.CUT_OFF) ? "Yes" : "No"));
}
//define the main method
public static void main(String[] args)
{
//Close scanner
sc.close();
}
}
Explanation:
The above snippet of code is used to create a class “CarlysEventPriceWithMethods”. In the code,
- Import the required header files.
- Define a class “CarlysEventPriceWithMethods”
- Declare the object of “Scanner” class.
- Define the “getEventNum()” method.
- Prompt the user to enter the event number.
- Return the value of “name”.
- Define the “getNoGuests()” method.
- Return the scanned value.
- Define the “displayMotto()” method.
- Print the values.
- Call the method “computePrice()”.
- Define the “computePrice()” method.
- Print the values.
- Define the “main()” method.
- Close the scanner.
b.
Event.java
//define a class Event
public class Event
{
//declare the class members
public final static int PRICE_PER_GUEST = 35;
public final static int CUT_OFF = 50;
private String eventNum;
private int noOfGuest;
private int price;
//define a method setEventNum()
public void setEventNum(String eventNum)
{
//set the value of eventNum
this.eventNum = eventNum;
}
//define a method setNoOfGuest()
public void setNoOfGuest(int noOfGuest)
{
//set the values of noOfGuest
this.noOfGuest = noOfGuest;
//set the values of price
this...

Trending nowThis is a popular solution!

Chapter 3 Solutions
Java Programming (MindTap Course List)
- 2. Case Problems 1 (three parts) - a - Carly’s Catering provides meals for parties and special events. In Chapter 2, you wrote an application that prompts the user for the number of guests attending an event, displays the company motto with a border, and then displays the price of the event and whether the event is a large one. Now modify the program so that the main() method contains only three executable statements that each call a method as follows: The first executable statement calls a public static int method that prompts the user for the number of guests and returns the value to the main() method. The second executable statement calls a public static void method that displays the company motto with the border. The last executable statement passes the number of guests to a public static void method that computes the price of the event, displays the price, and displays whether the event is a large event. Save the file as CarlysEventPriceWithMethods.java. b. Create a class to…arrow_forward// Question 4: // Declare an integer variable named "variableQ4" with an initial value of 10. // Write a method named "SetToOne" that takes one out parameter. // The method should set the out parameter to 1. // Call the method SetToOne passing variableQ4 in the btnQ4 click method. // Display the variable variableQ4 in the lblQ4. 1 reference private void btnQ4_Click(object sender, EventArgs e) { }arrow_forwardThe program must include two classesclGradesGraph and GradesGrapghDemo. GradesGraphDemo (one method)- Main method only GradesGraph- Include at least 4 methods (No credits without at least 4 methods!!!) Create a class that represents the grade distribution for a given course. In this class you should write methods to perform the following tasks: Read the number of each of the letter grades A, B, C D and F Set the number of letter grades A, B, C, D and F Return the total number of grades Return the percentage of each letter grade as a whole number between 0 and 100 inclusive Draw a bar graph of the grade distributionThe graph should have five bars, one per grade. Each bar can be a horizontal row of asterisks, such that the number of asterisks in a row is proportionate to the percentage of grades in each category. For example, let on asterisk represent 2%, so 50 asterisks correspond to 100%. Mark the horizontal axis at 10% increments from 0 to 100% and label each line with a letter…arrow_forward
- You invoke a method by its name followed by a pair of brackets and the usual semi-colon, PLEASE USE ONLY C# PROGRAMMING. Write a method called DisplayPersonalInfo(). This method will display your name, school, program and your favorite course. Call the DisplayPersonalInfo() method from your program Main() method Write a method called CalculateTuition(). This method will prompt the user for the number of courses that she is currently taking and then calculate and display the tuition cost. (cost = number of course * 569.99). Call the CalculateTuition() method two times from the same Main() method as in question 1. Write a method call CalculateAreaOfCircle(). This method will prompt the user for the radius of a circle and then calculate and display the area.[A = πr2].Call the CalculateAreaOfCircle() method twice from the same Main() method as in question 1. Use Math.Pi for the value of π Write a method call CalculateAreaOfTriangle(), that prompts the user for the base and height…arrow_forward// Question 4: // 20 Points // Declare an integer variable named "variableQ4" with an initial value of 10. // Write a method named "SetToOne" that takes one out parameter. // The method should set the out parameter to 1. // Call the method SetToOne passing variableQ4 in the btnQ4 click method. // Display the variable variableQ4 in the lblQ4. 1 reference private void btnQ4_Click(object sender, EventArgs e) { }arrow_forwardTrue or False: When passing multiple arguments to a method, the order in which the arguments are passed is not important.arrow_forward
- Don't use AI.arrow_forwardTravel Tickets Company sells tickets for airlines, tours, and other travel-related services. Because ticket agents frequently mistype long ticket numbers, Travel Tickets has asked you to write an application that indicates invalid ticket number entries. The class prompts a ticket agent to enter a six-digit ticket number. Ticket numbers are designed so that if you drop the last digit of the number, then divide the number by 7, the remainder of the division will be identical to the last dropped digit. Accept the ticket number from the agent and verify whether it is a valid number. Test the application with the following ticket numbers: . 123454; the comparison should evaluate to true . 147103; the comparison should evaluate to true . 154123; the comparison should evaluate to false Save the program as TicketNumber.java.arrow_forwardc# uppose there is a method called Current Deposit is your project class. The method header is as below: public static decimal Current Deposit( in years , decimal future, double rate) This method will return the current amount of money in an account if you pass the future amount, the annual interest rate, and years number. Write one statement to declare a decimal variable myMoney, call the method with the future amount $50,000.00, interest rate 8%, and 15 years, and assign the returned value to myMoney.arrow_forward
- True or False: During method calls, the value of a local variable is preserved.arrow_forwardA(n) __________ is a special variable that receives an argument when a method is called. a. reference variable b. argument variable c. parameter variable d. method variablearrow_forwardFor this interactive assignment, you will create an application that grades the written portion of the driver’s license exam. The exam has 20 multiple-choice questions. Here are the correct answers: B 2. D 3. A 4. A 5. B 6. A 7. B 8. A 9. C 10. D B 12. C 13. D 14. A 15. D 16. C 17. C 18. B 19. D 20. A Your program should store these correct answers in a list. The program should read the student’s answers for each of the 20 questions from a text file and store the answers in another list. (Create your own answer text file to test the application.) After the student’s answers have been read from the text file, the program should display a message indicating whether the student passed or failed the exam. (A student must correctly answer 15 of the 20 questions to pass the exam.) It should then display the total number of correctly answered questions, the total number of incorrectly answered questions, and a list showing the…arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
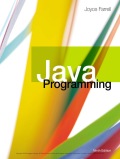
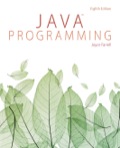
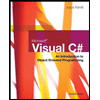