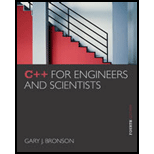
(a)
The following program will determine the value of int(a) where value of a=10.6.
(a)

Explanation of Solution
Program
//included header file #include <iostream> //included namespace usingnamespacestd; //main function intmain() { //declare variable a with value 10.6 floata=10.6; //determine and print the value of int(a) cout<<int(a); //exiting from program return0; }
Explanation:
In the above code, the value of a is 10.6, which is floating-point type value. The int(a) will typecast the value of a to integer type so that it will print only integer part only.
Sample Output:
(b)
The following program will determine the value of int(b) where the value of b=13.9.
(b)

Explanation of Solution
Program
//included header file #include <iostream> //included namespace usingnamespacestd; //main function intmain() { //declare variable b with value 13.9 floatb=13.9; //determine and print the value of int(b) cout<<int(b); //exiting from program return0; }
Explanation:
In the above code, the value of b is 13.9, which is floating-point type value. The int(b) will typecast the value of a to integer type so that it will print only integer part only.
Sample Output:
(c)
The following program will determine the value of int(c) where the value of c=-3.42.
(c)

Explanation of Solution
Program
//included header file #include <iostream> //included namespace usingnamespacestd; //main function intmain() { //declare variable c with value -3.42 floatc=-3.42; //determine and print the value of int(c) cout<<int(c); //exiting from program return0; }
Explanation:
In the above code, the value of c is -3.42, which is floating-point type value. The int(c) will typecast the value of a to integer type so that it will print only integer part only.
Sample Output:
(d)
The following program will determine the value of int(a+b) where the value of a=10.6 and b=13.9.
(d)

Explanation of Solution
Program
//included header file #include <iostream> //included namespace usingnamespacestd; //main function intmain() { //declare variable a with value 10.6 and bwith value 13.9 float a=10.6, b=13.9; //determine and print the value of int(a+b) cout<<int(a+b); //exiting from program return0; }
Explanation:
In the above code, the value of a is 10.6 and b is 13.9, which is floating-point type value. The int(a+b) will first add the value a and b then typecast the value of its result to an integer type and then print the value using cout statement.
Sample Output:
(e)
The following program will determine the value of int(a+b) where the value of a=10.6, b=13.9 and c=-3.42.
(e)

Explanation of Solution
Program
//included header file #include <iostream> //included namespace usingnamespacestd; //main function intmain() { //declare variable a with value 10.6, b with 13.9 and c with -3.42 float a=10.6, b=13.9, c=-3.42; //determine and print the value of int(a)+b+c cout<<int(a)+b+c; //exiting from program return0; }
Explanation:
In the above code, the value of a is 10.6, b is 13.9, and c is -3.42, which is floating-point type value. The int(a)+b+c will typecast the value of a to an integer type and then add and print the integer value of a, b and c.
Sample Output:
(f)
The following program will determine the value of int(a+b)+c where the value of a=10.6, b=13.9 and c=-3.42.
(f)

Explanation of Solution
Program
//included header file #include <iostream> //included namespace usingnamespacestd; //main function intmain() { //declare variable a with value 10.6, b with 13.9 and c with -3.42 float a=10.6, b=13.9, c=-3.42; //determine and print the value of int(a+b)+c cout<<int(a+b)+c; //exiting from program return0; }
Explanation:
In the above code, the value of a is 10.6, b is 13.9, and c is -3.42, which is floating-point type value. The int(a+b) will first add the value a and b then typecast the value of its result to an integer type and then add the value of c to it.
Sample Output:
(g)
The following program will determine the value of int(a+b+c) where the value of a=10.6, b=13.9 and c=-3.42.
(g)

Explanation of Solution
Program
//included header file #include <iostream> //included namespace usingnamespacestd; //main function intmain() { //declare variable a with value 10.6, b with 13.9 and c with -3.42 float a=10.6, b=13.9, c=-3.42; //determine and print the value of int(a+b+c) cout<<int(a+b+c); //exiting from program return0; }
Explanation:
In the above code, the value of a is 10.6, b is 13.9, and c is -3.42, which is floating-point type value. The int(a+b+c) will first add the value of a, b and c then typecast the value of its result to an integer type and print the value using cout statement.
Sample Output:
(h)
The following program will determine the value of float(int(a))+b where the value of a=10.6and b=13.9.
(h)

Explanation of Solution
Program
//included header file #include <iostream> //included namespace usingnamespacestd; //main function intmain() { //declare variable a with value 10.6 and b with 13.9 float a=10.6, b=13.9, c=-3.42; //determine and print the value of float(int(a))+b cout<<float(int(a))+b ; //exiting from program return0; }
Explanation:
In the above code, the value of a is 10.6and b is 13.9, which is floating-point type value. The float(int(a))+bwill first typecast the value of a to an integer type and then typecast this integer value to float and then add value b to it and print the value using cout statement.
Sample Output:
(i)
The following program will determine the value of float(int(a+b)) where the value of a=10.6and b=13.9.
(i)

Explanation of Solution
Program
//included header file #include <iostream> //included namespace usingnamespacestd; //main function intmain() { //declare variable a with value 10.6 and b with 13.9 float a=10.6, b=13.9, c=-3.42; //determine and print the value of float(int(a+b)) cout<<float(int(a+b)) ; //exiting from program return0; }
Explanation:
In the above code, the value of a is 10.6and b is 13.9, which is floating-point type value. The float(int(a+b))will first add the value of a+b and then typecast the value of its result to an integer type and then typecast this integer value to float and print the value using cout statement.
Sample Output:
(j)
The following program will determine the value of abs(a)+abs(b) where the value of a=10.6and b=13.9.
(j)

Explanation of Solution
Program
//included header file #include <iostream> #include <cmath> //included namespace usingnamespacestd; //main function intmain() { //declare variable a with value 10.6 and b with 13.9 float a=10.6, b=13.9, c=-3.42; //determine and print the value of abs(a)+abs(b) cout<<abs(a)+abs(b) ; //exiting from program return0; }
Explanation:
In the above code, the value of a is 10.6and b is 13.9, which is floating-point type value. The abs(a) and abs(b)function are used to get the absolute value of a and b then add this absolute value with each other and print the value using cout statement.
Sample Output:
(j)
The following program will determine the value of sqrt(abs(a-b)) where the value of a=10.6and b=13.9.
(j)

Explanation of Solution
Program
//included header file #include <iostream> #include <cmath> //included namespace usingnamespacestd; //main function intmain() { //declare variable a with value 10.6 and b with 13.9 float a=10.6, b=13.9, c=-3.42; //determine and print the value of sqrt(abs(a-b)) cout<<sqrt(abs(a-b)); //exiting from program return0; }
Explanation:
In the above code, the value of a is 10.6and b is 13.9, which is floating-point type value. The abs(a-b)function will determine the absolute value of a-b then the sqrt()function will determine the square root value of this resultand print the value using cout statement.
Sample Output:
Want to see more full solutions like this?
Chapter 3 Solutions
C++ for Engineers and Scientists
- (Practice) State whether the following variable names are valid. If they are invalid, state the reason. prod_a c1234 abcd _c3 12345 newamp watts $total new$al a1b2c3d4 9ab6 sum.of average volts1 finvoltarrow_forward(Practice) a. To convert inches (in) to feet (ft), the number of inches should be multiplied by which of the following conversion factors? i. 12 in/1 ft ii. 1 ft/12 in b. To convert feet (ft) to meters (m), the number of feet should be multiplied by which of the following conversion factors? i. 1 m/3.28 ft ii. 3.28 ft/1 m c. To convert sq.yd to sq.ft, the number of sq.yd should be multiplied by which of the following conversion factors? i. 1 sq.yd/9 sq.ft ii. 9 sq.ft/1 sq.yd d. To convert meters (m) to kilometers (km), the number of meters should be multiplied by which of the following conversion factors? i. 1000 m/1 km ii. 1 km/1000 m e. To convert sq.in to sq.ft, the number of sq.in should be multiplied by which of the following conversion factors? i. 144 sq.in/1 sq.ft ii. 1 sq.ft/144 sq.in f. To convert minutes (min) to seconds (sec), the number of minutes should be multiplied by which of the following conversion factors? i. 60 sec/1 min ii. 1 min/60 sec g. To convert seconds (sec) to minutes (min), the number of seconds should be multiplied by which of the following conversion factors? i. 60 sec/1 min ii. 1 min/60 secarrow_forwardPLEASE STEP BY STEP NO CODE Solve the following equations using Horner’s Rule: 2x4+4x3+7x2+6x+5 at the point x=2arrow_forward
- Problem 2. Evaluate the expressions in each of the following triples: (a) 8 (mod 3) = 12 (mod 3) = 8 + 12 (mod 3) = arrow_forwardSimplify the following formula. 1. F = XY’Z’ + X’Y’Z’ + X’Y’Z + X’YZ 2. F = X’YZ’ + XYZ’ + XYZ + XY’Zarrow_forwardComputer Science f(x)= Sin(x) – x 3 + C Where C = Max [0.15, (69/100)] x20, (x is in radian). Apply the false position method three iterations to find the root of the function (Decide the starting values of Xl and Xu yourself)arrow_forward
- 1. Simplify the following Boolean functions, using three-variable Kmaps: (a) F(x, y, z) = Σ(0, 1, 5, 7) (b) F(x, y, z) = Σ(1, 2, 3, 6, 7) (c) F(x, y, z) = Σ(2, 3, 4, 5)arrow_forward(Practice) Run Program 7.10 to determine the average and standard deviation of the following list of 15 grades: 68, 72, 78, 69, 85, 98, 95, 75, 77, 82, 84, 91, 89, 65, and 74.arrow_forwardProblem 1. Evaluate the following expressions: (d) 22 (mod 11) = (e) −17 (mod 8) = (f)0(mod9)=arrow_forward
- State whether the following are true or false. If the answer is false, explain why.c) The expression (x > y && a < b) is true if either x > y is true or a < b is true.arrow_forward4. Look up the Pythagorean theorem if you are not already familiar with it. Use the following formula to solve for c in the formula: c = √a2 + b2. Use the proper functions from the cmath header file. Be sure to output the result..arrow_forwardCouldn't fit the whole problem into 1arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
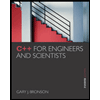