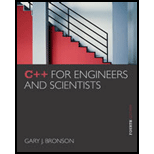
(General math) a. Write, compile, and run a C++
b. Check the result of your program written for Exercise 7a by using the following test data:
After finishing your verification, use your program to complete the following chart
a.

Program plan:
- The variable a, b and c of type float is used to accept the coefficient of second order polynomial.
- The variable x of type float is used to accept the value of x of second order polynomial.
- The variable p of type float calculates the value of second order polynomial.
Program description:
The main purpose of the program is to accept the coefficient of x and value of x and then calculate and display the value of second order polynomial.
Explanation of Solution
Program
#include <iostream> #include <math.h> using namespace std; int main() { // declaration of variables float a, b, c; float x; float p; // Input values cout<<"Input values to calculate value of the second-order polynomial :: ax^2 + bx +c"; cout<<"\nEnter the coefficient of the x-squared term(a) :: "; cin>>a; cout<<"\nEnter the coefficient of the x term (b) :: "; cin>>b; cout<<"\nEnter the coefficient of the term (c) :: "; cin>>c; cout<<"\nEnter the value of x :: "; cin>>x; // Calculate value of the second-order polynomial p = a * x * x + b * x + c ; cout<<"\nThe value of the second-order polynomial is :: "<<p; return 0; }
Explanation:
In the above program a, b and c accepts the coefficient of x for second order polynomial. The variable x accepts the value of x and then second order polynomial is calculated and displayed.
Sample Output:
Enterthecoefficientofthex-squaredterm(a)::0
Enterthecoefficientofthexterm(b)::0
Enterthecoefficientoftheterm(c)::22
Enterthevalueofx::56
Thevalueofthesecond-orderpolynomialis::22
b.

To check the given program for the test values as follows:
Test data set 1: a = 0, b = 0, c = 22, x = 56
Test data set 2: a = 0, b = 22, c = 0, x = 2
Test data set 3: a = 22, b = 0, c = 0, x = 2
Test data set 4: a = 2, b = 4, c = 5, x = 2
To complete the following chart:
a | b | c | x | Polynomial Value |
2.0 | 17.0 | -12.0 | 1.3 | |
3.2 | 2.0 | 15.0 | 2.5 | |
3.2 | 2.0 | 15.0 | -2.5 | |
-2.0 | 10.0 | 0.0 | 2.0 | |
-2.0 | 10.0 | 0.0 | 4.0 | |
-2.0 | 10.0 | 0.0 | 5.0 | |
-2.0 | 10.0 | 0.0 | 6.0 | |
5.0 | 22.0 | 18.0 | 8.3 | |
4.2 | -16.0 | -20.0 | -5.2 |
Explanation of Solution
Given Program
#include <iostream> #include <math.h> using namespace std; int main() { // declaration of variables float a, b, c; float x; float p; // Input values cout<<"Input values to calculate value of the second-order polynomial :: ax^2 + bx +c"; cout<<"\nEnter the coefficient of the x-squared term(a) :: "; cin>>a; cout<<"\nEnter the coefficient of the x term (b) :: "; cin>>b; cout<<"\nEnter the coefficient of the term (c) :: "; cin>>c; cout<<"\nEnter the value of x :: "; cin>>x; // Calculate value of the second-order polynomial p = a * x * x + b * x + c ; cout<<"\nThe value of the second-order polynomial is :: "<<p; return 0; }
Explanation:
Test data set 1:
a = 0, b = 0, c = 22, x = 56
Sample Output:
Input values to calculate value of the second-order polynomial :: ax^2 + bx +c
Enterthecoefficientofthex-squaredterm(a)::0
Enterthecoefficientofthexterm(b)::0
Enterthecoefficientoftheterm(c)::22
Enterthevalueofx::56
Thevalueofthesecond-orderpolynomialis:: 22
Test data set 2:
a = 0, b = 22, c = 0, x = 2
Sample Output:
Input values to calculate value of the second-order polynomial :: ax^2 + bx +c
Enter the coefficient of the x-squared term(a) :: 0
Enter the coefficient of the x term (b) :: 22
Enter the coefficient of the term (c) :: 0
Enter the value of x :: 2
The value of the second-order polynomial is :: 44
Test data set 3:
a = 22, b = 0, c = 0, x = 2
Sample Output:
Input values to calculate value of the second-order polynomial :: ax^2 + bx +c
Enter the coefficient of the x-squared term(a) :: 22
Enter the coefficient of the x term (b) :: 0
Enter the coefficient of the term (c) :: 0
Enter the value of x :: 2
The value of the second-order polynomial is :: 88
Test data set 4:
a = 2, b = 4, c = 5, x = 2
Sample Output
Input values to calculate value of the second-order polynomial :: ax^2 + bx +c
Enter the coefficient of the x-squared term(a) :: 2
Enter the coefficient of the x term (b) :: 4
Enter the coefficient of the term (c) :: 5
Enter the value of x :: 2
The value of the second-order polynomial is :: 21
Chart
Following chart displays the polynomial value for different values of a, b, c and x.
a | b | c | x | Polynomial Value |
2.0 | 17.0 | -12.0 | 1.3 | 13.480 |
3.2 | 2.0 | 15.0 | 2.5 | 40.000 |
3.2 | 2.0 | 15.0 | -2.5 | 30.000 |
-2.0 | 10.0 | 0.0 | 2.0 | 12.000 |
-2.0 | 10.0 | 0.0 | 4.0 | 8.000 |
-2.0 | 10.0 | 0.0 | 5.0 | 0.000 |
-2.0 | 10.0 | 0.0 | 6.0 | -12.000 |
5.0 | 22.0 | 18.0 | 8.3 | 545.050 |
4.2 | -16.0 | -20.0 | -5.2 | 176.768 |
Want to see more full solutions like this?
Chapter 3 Solutions
C++ for Engineers and Scientists
- (General math) The volume of oil stored in an underground 200-foot deep cylindrical tank is determined by measuring the distance from the top of the tank to the surface of the oil. Knowing this distance and the radius of the tank, the volume of oil in the tank can be determined by using this formula: volume=radius2(200distance) Using this information, write, compile, and run a C++ program that accepts the radius and distance measurements, calculates the volume of oil in the tank, and displays the two input values and the calculated volume. Verify the results of your program by doing a hand calculation using the following test data: radius=10feetanddistance=12feet.arrow_forward(Practice) Determine the values of the following integer expressions: a.3+46f.202/( 6+3)b.34/6+6g.( 202)/6+3c.23/128/4h.( 202)/( 6+3)d.10( 1+73)i.5020e.202/6+3j.( 10+3)4arrow_forward(Physics) a. Design, write, compile, and run a C++ program to calculate the elapsed time it takes to make a 183.67-mile trip. This is the formula for computing elapsed time: elapsedtime=totaldistance/averagespeed The average speed during the trip is 58 mph. b. Manually check the values computed by your program. After verifying that your program is working correctly, modify it to determine the elapsed time it takes to make a 372-mile trip at an average speed of 67 mph.arrow_forward
- (General math) a. Design, write, compile, and run a C++ program to calculate the volume of a sphere with a radius, r, of 2 in. The volume is given by this formula: Volume=4r33 b. Manually check the values computed by your program. After verifying that your program is working correctly, modify it to determine the volume of a cube with a radius of 1.67 in.arrow_forward(Physics) a. The weight of an object on Earth is a measurement of the downward force onth e object caused by Earth’s gravity. The formula for this force is determined by using Newton’s Second Law: F=MAeFistheobjectsweight.Mistheobjectsmass.AeistheaccelerationcausedbyEarthsgravity( 32.2ft/se c 2 =9.82m/ s 2 ). Given this information, design, write, compile, and run a C++ program to calculate the weight in lbf of a person having a mass of 4 lbm. Verify the result produced by your program with a hand calculation. b. After verifying that your program is working correctly, use it to determine the weight, on Earth, of a person having a mass of 3.2 lbm.arrow_forward(Practice) Run Program 7.10 to determine the average and standard deviation of the following list of 15 grades: 68, 72, 78, 69, 85, 98, 95, 75, 77, 82, 84, 91, 89, 65, and 74.arrow_forward
- (General math) a. Write a C++ program to calculate and display the value of the slope of the line connecting two points with the coordinates (3,7) and (8,12). Use the fact that the slope between two points with the coordinates (x1,y1)and(x2,y2)is(y2y1)/(x2x1). b. How do you know the result your program produced is correct? c. After verifying the output your program produces, modify it to determine the slope of the line connecting the points (2,10) and (12,6). d. What do you think will happen if you use the points (2,3) and (2,4), which results in a division by zero? How do you think this situation can be handled? e. If your program doesn’t already do so, change its output to this: The value of the slope is xxx.xx The xxx.xx denotes placing the calculated value in a field wide enough for three places to the left of the decimal point and two places to the right of it.arrow_forward(General math) a. Design, write, compile, and run a C++ program that calculates and displays the area of a triangle, such as the one in Figure 2.18, with a base of 1 in and a height of 1.5 in. The area is given by this formula: Area=12(base)(height) b. Manually check the values computed by your program. After verifying that your program is working correctly, modify it to determine the area of a two-dimensional triangle with a base of 3.5 in and a height of 1.45 in.arrow_forwardState whether the following are true or false. If the answer is false, explain why.c) The expression (x > y && a < b) is true if either x > y is true or a < b is true.arrow_forward
- For the following code, match the outcome if x =:arrow_forward4. Look up the Pythagorean theorem if you are not already familiar with it. Use the following formula to solve for c in the formula: c = √a2 + b2. Use the proper functions from the cmath header file. Be sure to output the result..arrow_forwardAnswer problem 14 and 15 pleasearrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
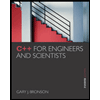