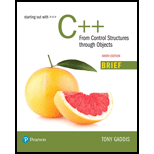
Concept explainers
Explanation of Solution
Output of the Given Program:
//Include the required header files
#include <iostream>
#include <iomanip>
using namespace std;
//Main Function
int main()
{
//Declare the required variables
int unus, duo, tres;
//Initialize the variables
unus = duo = tres = 5;
//Add 4 to 5 and store it in a variable
unus += 4;
//Multiply 2 to 5 and store it in a variable
duo *= 2;
//Subtract 4 from 5 and store it in a variable
tres -= 4;
//Divide unus value by 3 and store it in a variable
unus /= 3;
//Add duo value to tres and store it in a variable
duo += tres;
//Print the value
cout << unus << endl;
//Print the value
cout << duo << endl;
//Print the value
cout << tres << endl;
//Return the statement
return 0;
}
Explanation:
- Include the required header files
- Give the “main ()” function
- Declare the variables “unus”, “duo”, and “tres”.
- Initialize those variables to 5...

Want to see the full answer?
Check out a sample textbook solution
Chapter 3 Solutions
Mylab Programming With Pearson Etext -- Access Card -- For Starting Out With C++: From Control Structures Through Objects, Brief Version
- FOR C++, PLASE SEND THE ANSER IN 30 MINUTES. ACCORDING TO CODE BELOW, #include "Vehicle.hh" class Ship: public Vehicle { private: int passenger; public: Ship(); Ship(int, float, float); int getPassenger(); void setPassenger(int); void print(); }; (DEFINE FUNCTIONS CORRESPONDING TO SHIP HEADER CLASS IN PART 2 BELOW) #include "Vehicle.hh" #include "Ship.hh" #include <iostream> using namespace std; // *** DEFINE --VEHICLE-- FUNCTIONS FOR PART 1 *** // *** DEFINE --SHIP-- FUNCTIONS FOR PART 2 *** void reduceSpeed(Ship*, float); void takePassenger(Ship*, int); int main() { return 0; } // reduce the speed of ship given in percentage (0 < percentage < 1). void reduceSpeed(Ship *s, float percentage) { // *** FILL THIS FUNCTION FOR PART 3 *** } // takes the number of passengers to the ship void takePassenger(Ship *s, int pas) { // *** FILL THIS FUNCTION FOR PART 4 *** }arrow_forward- Create a struct called Complex for performing arithmetic with complex numbers. Write a driver program to test your struct. Complex numbers have the form: realPart + imaginaryPart * iwhere i is the square root of -1Use double variables to represent data of the struct. Provide a function that enables an object of this struct to be initialized when it is declared. The function should contain default values in case no initializers are provided. Also provide functions for each of the following:a) Addition of two Complex numbers: The real parts are added together and the imaginary parts are added together.b) Subtraction of two Complex numbers: The real part of the right operand is subtracted from the real part of the left operand and the imaginary part of the right operand is subtracted from the imaginary part of the left operand.c) Printing Complex numbers in the form (a, b) where a is the real part and b is the imaginary partSubmit one file which contains all code above: the structure…arrow_forwardThis is in c++ The base class Pet has private data members petName, and petAge. The derived class Dog extends the Pet class and includes a private data member for dogBreed. Complete main() to: create a generic pet and print information using PrintInfo(). create a Dog pet, use PrintInfo() to print information, and add a statement to print the dog's breed using the GetBreed() function. main.cpp #include <iostream> #include<string> #include "Dog.h" using namespace std; int main() { string petName, dogName, dogBreed; int petAge, dogAge; Pet myPet; Dog myDog; getline(cin, petName); cin >> petAge; cin.ignore(); getline(cin, dogName); cin >> dogAge; cin.ignore(); getline(cin, dogBreed); // TODO: Create generic pet (using petName, petAge) and then call PrintInfo // TODO: Create dog pet (using dogName, dogAge, dogBreed) and then call PrintInfo // TODO: Use GetBreed(), to output the breed of the dog } Dog.cpp #include "Dog.h" #include…arrow_forward
- FOR C++, ACCORDING THE VEHICLE HEADER, FILL THE PART 1 OF THE CODE #ifndef _Vehicle_hh_ #define _Vehicle_hh_ class Vehicle { private: float speed; float mile; public: Vehicle(); Vehicle(float, float); float getSpeed(); float getMile(); void setSpeed(float); void setMile(float); void print(); }; #endif (DEFINE FUNCTIONS CORRESPONDING TO VEHICLE HEADER CLASS IN PART 1 BELOW) #include "Vehicle.hh" #include "Ship.hh" #include <iostream> using namespace std; // *** DEFINE --VEHICLE-- FUNCTIONS FOR PART 1 *** // *** DEFINE --SHIP-- FUNCTIONS FOR PART 2 *** void reduceSpeed(Ship*, float); void takePassenger(Ship*, int); int main() { return 0; } // reduce the speed of ship given in percentage (0 < percentage < 1). void reduceSpeed(Ship *s, float percentage) { // *** FILL THIS FUNCTION FOR PART 3 *** } // takes the number of passengers to the ship void takePassenger(Ship *s, int pas) { // *** FILL THIS FUNCTION FOR…arrow_forwardThis exercise assesses the skills required to develop user defined functions, and pointers. Task:Develop the program with Graphics properties to give a better look to your program. You need to explore the graphics libraries available in C++ e.g. <Graphic.h> *need to solve this Q with #include <iostream> Not other libary .* Exercise 2 Write a C++ program to develop a Vehicle Fine Management System for Police. The program will have the following features.1. A user defined function called “Student Registration”. This function prompts a user to enter a student’s personal data i.e., Student Number, Student Name, Age and City and stores them. 2. A user defined function called “Module Enrolment”. This function takes student number from themain function as an argument and prompts a user to enter student two modules details i.e. Module name, Module Code and Module Credits Hours (15 or 30) and stores them. The programs should display an error message if a user enters credit hour…arrow_forwardPROGRAMMING LANGUAGE: C++ Write a complete C++ program with the following features.a. Declare a class Time with two fields, hour and minute.b. Provide appropriate constructors to initialize the data members.c. Overload the pre and postfix increment operators (using memberfunctions) to increment the minutes by one. Also make sure if m inutes are59 and you increment the Time it should update hours and minutesaccordingly.d. Provide a function display() to display the hours and minutes.e. In main(), create objects of Time, initialize them using constructors andcall the display functions.f. Test the following in your main:a. T3= ++T1;b. T4=T2++;arrow_forward
- In C++Using the code provided belowDo the Following: Modify the Insert Tool Function to ask the user if they want to expand the tool holder to accommodate additional tools Add the code to the insert tool function to increase the capacity of the toolbox (Dynamic Array) USE THE FOLLOWING CODE and MODIFY IT: #define _SECURE_SCL_DEPRECATE 0 #include <iostream> #include <string> #include <cstdlib> using namespace std; class GChar { public: static const int DEFAULT_CAPACITY = 5; //constructor GChar(string name = "john", int capacity = DEFAULT_CAPACITY); //copy constructor GChar(const GChar& source); //Overload Assignment GChar& operator=(const GChar& source); //Destructor ~GChar(); //Insert a New Tool void insert(const std::string& toolName); private: //data members string name; int capacity; int used; string* toolHolder; }; //constructor GChar::GChar(string n, int cap) { name = n; capacity = cap; used = 0; toolHolder = new…arrow_forwardstruct date{ int day; int month; int year; }; Write a function named void increaseDay(struct date *d) that increases the value of a variable of struct date type with integer year, month, and day members by one day. Write a function named void decreaseDay(struct date *d) that decreases the value of a variable of struct date type with integer year, month, and day members by one day. Write a C program that reads from the user a date in d/m/y format and the amount of increase or decrease as an integer. Display the new date in d/m/y format. You may call related functions as many as given increase or decrease value. Note 1: You do not need to consider leap years. Use always 28 days for month February. Note 2: Do not modify the function prototypes. Sample Input1: Sample Output1: 12/8/1990 -5 7/8/1990 Sample Input2: Sample Output2: 26/2/2005 5 3/3/2005 Sample Input3: Sample Output3: 29/12/1998 7 5/1/1999…arrow_forwardC++ simple friend function.Create a C++ class called cube that calculates the volume of a cube. Use appropriate accessors (getters) and mutators (setters), constructors, etc. Your program must also include a friend function that will sum the volume of two cube class instances.Output the individual volumes of each of the two cube classes and then the total volume from the friend function. Example of friend funtion #include <iostream> using namespace std; class Box { double width; public: friend void printWidth( Box box ); void setWidth( double wid );}; // Member function definitionvoid Box::setWidth( double wid ) { width = wid;} // Note: printWidth() is not a member function of any class.void printWidth( Box box ) { /* Because printWidth() is a friend of Box, it can directly access any member of this class */ cout << "Width of box : " << box.width <<endl;} // Main function for the programint main() { Box box; // set box width…arrow_forward
- In C language. Please don't copy similar programs from, bartleby or chegg Define a structure type auto_t to represent an automobile. Include components for the make and model (strings), the odometer reading, the manufacture and purchase dates (use another user-defined type called date_t), and the gas tank (use a user-defined type tank_t with components for tank capacity and current fuel level, giving both in gallons). Write I/O functions scan_date, scan_tank, scan_auto, print_date, print_tank, and print_auto, and also write a driver function that repeatedly fills and displays an auto structure variable until input is -30. Note: Each record has a number (1, 2, 3 ...),once the program scans record number -30, it should terminate without processing it. SAMPLE RUN #4: ./Structures Interactive Session Show Invisibles Highlight: Enter record number:1 Enter Make:Mercury Enter Model:Sable Enter Odometer Reading:99842 Enter Month:1 Enter Day:18 Enter Year:2001 Enter Month:5 Enter Day:30…arrow_forwardCreate class Employee that has the following pieces of information called first name, last name, day off and salary. Write a C++ program to implement the following functions for three employees: 1- Constructor to initialize data members. 2- Set and get functions for data members 3- Operate decrement operator to decrement the salary when the employee has a day off. 4- Display the results using friend function called Display().arrow_forwardQuestion 1: Write a C function that declares a student structure that contains his name, his first name and his student number. Question 2: Write a C function that initializes the cell by taking the 3 parameters from the keyboard. Question 3: Write a function that displays the contents of the cell passed as a parameter.arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
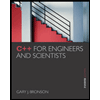