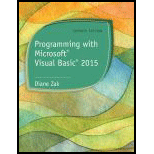
Bakery project
Program plan:
- Create new Windows Forms Application.
- Design the form by placing the labels, textboxes, and buttons and then change their name and properties.
- Inside the “Calculate” button,
- Declare the required variables.
- Assign the name to the variable.
- Get the item price.
- Convert the string type values into a decimal value.
- Get the muffin price.
- Convert the string type values into a decimal value.
- Convert the string type values into the integer values
- Calculate the total item, subtotal of the item, sales tax, and total sales.
- Display the total amount, tax, and salesclerk’s name.
- Inside the “Clear Screen” button,
- Clear all the dates except date.
- Inside the “Exit” button,
- Close the form using “Me.Close” method
- Inside the “Print Receipt” button,
- Print the sales receipt.
- Inside the “ClearLabels”,
- Clear the calculated amounts.

This program is used to allow the user to enter the doughnut price, muffin price and then calculate the total items and total costs.
Explanation of Solution
Program:
'Definition of class frmMain
Public Class frmMain
'Definition of calculate
Private Sub btnCalc_Click(sender As Object, e As EventArgs) Handles btnCalc.Click
' Declare the variables
Const strPROMPT As String = "Salesclerk's name:"
Const strTITLE As String = "Name Entry"
Const decTAX_RATE As Decimal = 0.02D
Dim intDonuts As Integer
Dim intMuffins As Integer
Dim intTotalItems As Integer
Dim decSubtotal As Decimal
Dim decSalesTax As Decimal
Dim decTotalSales As Decimal
Static strClerk As String
Static strDonutPrice As String
Static strMuffinPrice As String
Dim decDonutPrice As Decimal
Dim decMuffinPrice As Decimal
'Assign name to variable
strClerk = InputBox(strPROMPT, strTITLE, strClerk)
'Get item prices
strDonutPrice = InputBox("Doughnut price:", "Doughnut Price Entry", strDonutPrice)
'Convert the string type value into a decimal 'value
Decimal.TryParse(strDonutPrice, decDonutPrice)
'Get muffin price
strMuffinPrice = InputBox("Muffin price:", "Muffin Price Entry", strMuffinPrice)
'Convert the string type value into a decimal 'value
Decimal.TryParse(strMuffinPrice, decMuffinPrice)
'Convert the string type’s value into an 'integer values
Integer.TryParse(txtDonuts.Text, intDonuts)
Integer.TryParse(txtMuffins.Text, intMuffins)
'Calculate the total items
intTotalItems = intDonuts + intMuffins
'Calculate the subtotal
decSubtotal = intDonuts * decDonutPrice + intMuffins * decMuffinPrice
'Calculate the sales tax
decSalesTax = decSubtotal * decTAX_RATE
'Calculate the total sales
decTotalSales = decSubtotal + decSalesTax
'Display total amounts
lblTotalItems.Text = Convert.ToString(intTotalItems)
lblTotalSales.Text = decTotalSales.ToString("C2")
'Display tax and salesclerk's name
lblMsg.Text = "The sales tax was " &
decSalesTax.ToString("C2") & "." &
ControlChars.NewLine & strClerk
End Sub
'Definition of Clear button
Private Sub btnClear_Click(sender As Object, e As EventArgs) Handles btnClear.Click
'Clear calculated amounts
txtDonuts.Text = String.Empty
txtMuffins.Text = String.Empty
lblTotalItems.Text = String.Empty
lblTotalSales.Text = String.Empty
lblMsg.Text = String.Empty
'Send the focus to the Doughnuts box
txtDonuts.Focus()
End Sub
'Definition of button Exit
Private Sub btnExit_Click(sender As Object, e As EventArgs) Handles btnExit.Click
'Close the form
Me.Close()
End Sub
'Definition of button Print
Private Sub btnPrint_Click(sender As Object, e As EventArgs) Handles btnPrint.Click
'Print the sales receipt
btnCalc.Visible = False
btnClear.Visible = False
btnExit.Visible = False
btnPrint.Visible = False
PrintForm1.Print()
btnCalc.Visible = True
btnClear.Visible = True
btnExit.Visible = True
btnPrint.Visible = True
End Sub
'Definition of "ClearLabels"
Private Sub ClearLabels(sender As Object, e As EventArgs) _
Handles txtDonuts.TextChanged, txtMuffins.TextChanged
'Clear the total items, total sales, and 'message
lblTotalItems.Text = String.Empty
lblTotalSales.Text = String.Empty
lblMsg.Text = String.Empty
End Sub
End Class
Output:
Enter the date, doughnuts, and muffins. Then click “Calculate” button.
Screenshot of “Meyer’s Purple Bakery” form
Enter the salesclerk name and then “OK” button.
Screenshot of “Name Entry” form
Enter the Doughnut price and then “OK” button.
Screenshot of “Doughnut Price Entry” form
Enter the muffin price and then “OK” button.
Screenshot of “Muffin Price Entry” form
Screenshot of “Meyer’s Purple Bakery” form
After clicking “Print Receipt” button the following window will appear:
Screenshot of “Print preview” form
After clicking “Clear Screen” button the entire textbox is cleared except date:
Screenshot of “Meyer’s Purple Bakery” form
Want to see more full solutions like this?
Chapter 3 Solutions
Programming with Microsoft Visual Basic 2015 (MindTap Course List)
- Programming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
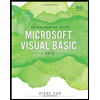