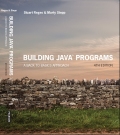
Concept explainers
Write a method called fractionSum that accepts an integer parameter n and returns as a double the sum of the first n terms of the sequence
In other words, the method should generate the following sequence:
You may assume that the parameter n is nonnegative.

Explanation of Solution
Given code snippet:
//define the static function
public static double fractionSum(int n) {
//declare the required variables
int denominator = 1;
double sum = 0.0;
//loop to generate the sequence
for (int i = 1; i <= n; i++) {
//evaluate the value
sum += (double) 1 / denominator;
//increment the value
denominator++;
}
//return statement
return sum;
}
Explanation to the above code snippet:
- In the above code, the static method named “fractionSum” is defined.
- Required variables are declared and initialized.
- For loop is initialized to generate the required sequence.
- Evaluate the value and store it in the variable “sum”.
- The value stored in the variable “sum” is returned.
Want to see more full solutions like this?
Chapter 4 Solutions
EBK BUILDING JAVA PROGRAMS
Additional Engineering Textbook Solutions
Objects First with Java: A Practical Introduction Using BlueJ (6th Edition)
Java: An Introduction to Problem Solving and Programming (7th Edition)
Big Java Late Objects
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
Survey of Operating Systems, 5e
- Write a method that will receive 3 integers and return the largest integer. public static int findMax(int a, int b, int c) {arrow_forwardwrite a method that calculate the average of all numbers from 1 to n, return a double valuearrow_forwardWrite a Java method that generates and returns a random number between 2 valuesreceived as parameters. You must consider 2 cases when there are or not differencebetween values (please refer to slide 43 from 04 Methods). The function should checkif the difference is strictly positive otherwise replace it with the value 1.Test your method using main as follows (bold values are input by the user):Lowest value: 60Highest value: 100Diff. between values: 5Number of random numbers: 5060 90 70 65 70 60 75 100 100 8580 90 60 95 75 65 80 70 90 9085 65 95 60 85 95 75 85 60 7090 80 65 65 90 90 80 60 95 7560 90 70 70 90 60 65 60 85 60arrow_forward
- Write a program with a method named getTotal that accepts two integers as an argument and return its sum. Call this method from main( ) and print the results.arrow_forwardWrite a method in Java called printPowersOfN that accepts a base and an exponent as arguments and prints each power of the base from base0 (1) up to that maximum power, inclusive. For example, consider the following calls: printPowersOfN(4, 3);printPowersOfN(5, 6);printPowersOfN(-2, 8);These calls should produce the following output: 1 4 16 641 5 25 125 625 3125 156251 -2 4 -8 16 -32 64 -128 256arrow_forwardWrite a java method named findLargest that returns the largest number stored in it's parameter, an array of int value.arrow_forward
- Write a method in Java called largestAbsVal that accepts three integers as parameters and returns the largest of their three absolute values. For example, a call of largestAbsVal(7, -2, -11) would return 11, and a call of largestAbsVal(-4, 5, 2) would return 5.arrow_forwardWrite a java method sumOfAllOddNumbersBetween() that takes as input two integers and returns the sum of all odd numbers that are between the two given numbers (the given numbers should be excluded from the sum). Write a main program to test your method by accepting the two integers from the user.arrow_forwardWrite a Java program that implements static method roundSum() so that given 3 ints: a, b, and c; return the sum of their rounded values. We'll round an int value up to the next multiple of 10 if its rightmost digit is 5 or more, so 15 rounds up to 20. Alternately, round down to the previous multiple of 10 if its rightmost digit is less than 5, so 12 rounds down to 10. roundSum(16, 17, 18) → 50roundSum(12, 13, 14) → 40roundSum(6, 4, 4) → 10 The examples of input and output you can see in the picture. (the code structure should be as in the second picture)arrow_forward
- Write a java class method named countDigits that returns the number of digits in it's parameter, which is of type int.arrow_forwardConsider the below method which computes the value x. Specifically, x = (a*b)/c, based on inputs a, b, and c. This function have several problems. public int computeX (int a, int b, int c){ int x = (a*b)/c; Rewrite the method to fix these errors. return x;}arrow_forwardin java write a method (void rect(int a,int b)), which draws the following figure: a rectangle size a x b , each even row contains line numbers (2,4,6,8,...), written consecutively, each odd row contains numbers that increment from 1 to half of the width and back to 1. Example: input: a= 6, b= 5 Result: 123321 222222 123321 444444 123321arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
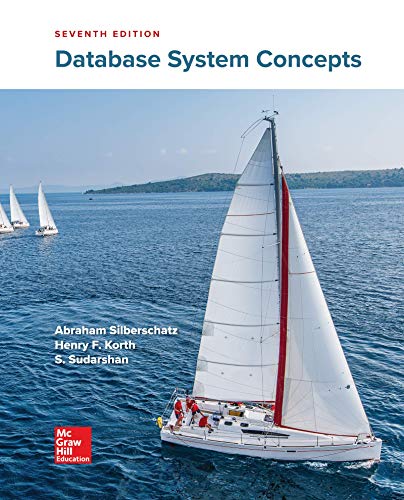
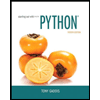
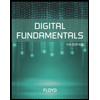
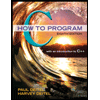
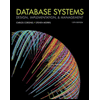
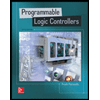