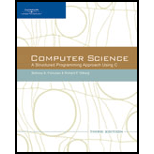
Explanation of Solution
Given: The user passes 3, 5, 4, 6 as inputs in the following
#include
//Function Declarations
int strange(int x, int y);
int main(void)
{
//Local Declarations
int a;
int b;
int c;
int d;
int r;
int s;
int t;
int u;
int v;
//Statements
scanf("%d %d %d %d", &a, &b, &c, &d);
r=strange(a,b);
s=strange(r,c);
t=strange(strange(s,d), strange(4,2));
u=strange(t+3, s+2);
v=strange(strange(strange(u, a), b),c);
printf("%d %d %d %d %d", r, s, t, u, v);
return 0;
}//main
// = = = = = = = = = = strange = = = = = = = = = = =
int strange(int x, int y)
{
//Local Declarations
int t;
int z;
//Statements
t=x+y;
z=x*y;
return (t+z);
} //strange
To find:Â The output of the aforementioned program for the given inputs, 3, 5, 4, and 6.
Solution:
The aforementioned program will display the following output:
23 119 12599 1537565 184507919
The following are the descriptions for determining the output:
In the above program, firstly, the header file is included, and then the function, strange, is declared, which has two integer parameters...

Want to see the full answer?
Check out a sample textbook solution
Chapter 4 Solutions
Computer Science: A Structured Programming Approach Using C, Third Edition
- Consider the function definition: void GetNums(int howMany, float& alpha, float& beta) { int i; beta = 0; for (i = 0; i < howMany; i++) { beta = alpha + beta; } } Describe what happens in MEMORY when the GetNums function is called.arrow_forwardIn C programming, if the return type of a function is Void how can you implement assert statements? For example, Void ceasar(int n, char*x); //if char = c and n = 3 output == f how do i use assert statements as this does not work assert (caesar(3,c)==f);arrow_forwardExercise 9-6: Pass by Reference and Pass by Address In this exercise, you use what you have learned about passing arguments by reference and by address to functions to answer Questions 1-2. 1. Given the following variable and function declarations, write the function call and the function's header a. double price 22.95, increase .10; void changePrice(doubl e&, double); b. double price 22.95, increase .10; void changePrice (double*, double); c. int age = 23; void changeAge(int&); d. int age 23; void changeAge (int*); 2. Given the following function headers and variable declarations, write a function call: a. custNames [] = {"Perez", "Smith", "Patel", "Shaw"}; balances [] = {34.00, 21.00, 45.50, 67.00); void cust (string name [], double bal []) b. int values [] = {1, 77, 89, 321, -2, 34}: void printSum (int nums [])arrow_forward
- Please help me explain the following void function c++ code, which I did not understand. Especially for the first void function and int main(), I do not understand why the first void function enter the number and the int main function print out the final answer. #include <iostream>using namespace std; void getNumber(int *input); void doubleValue(int *val); int main(){ int number; getNumber(&number); doubleValue(&number); cout<<"That value doubled is"<<number<<endl; return 0;}void getNumber(int *input){ cout<<"Enter an integer number:"; cin>>*input; }void doubleValue(int *val){ *val *=2;}arrow_forwardAssume that the declarations for two functions look as follows: int Function1(double x); 2.void Function2(double x); Give some sample code that would show how each function could be used.arrow_forwardWrite a C++ program that: Define a void function named Max() with two parameters. The function accepts two arguments: two double-type numbers, and the function will compare those two numbers and displays the larger one. In the main function of the program, prompt user to enter two double numbers, and then the Max() function will be called to print the result.arrow_forward
- Write a statement that declares a prototype for a function divide that takes four arguments and returns no value. The first two arguments are of type int. The last two arguments arguments are pointers to int that are set by the function to the quotient and remainder of dividing the first argument by the second argument. The function does not return a value.arrow_forwardQUESTION 15 Python supports the creation of anonymous functions at runtime, using a construct called __________ A. def B. pi C. anonymous D. lambdaarrow_forwardin C Given the code below, what would be the proper way to pass the pointers ptr_1, and ptr_2, to the function mix () defined below? #include <stdio.h> void mix( float *, float * ); int main (void) { float *ptr_1, *ptr_2; mix( ?, ? ) <--- What is the correct way to call the function mix (), and pass the pointer variables ptr_1, ptr_2? return 0; }arrow_forward
- Consider the following program in C-like syntax:void f1();void f2();int main(){int x, y;...}int f1()int a, b, x;...}int f2(){int b, c, x, y;...} If the programming language uses dynamic scoping, what are the variables that arevisible in the last function in the following call sequences? Give not only the names ofthe variables, but also where they are declared.(a) main → f1 → f2(b) main → f2 → f1arrow_forward21) What kind of function is shown in the codes below? def printMsg(): print("Midterm Exam") printMsg() a. fruitful function without parameters b. fruitful function with parameters c. void function with parameters d. void function without parameters Python 3arrow_forwardWrite a void function "summax" that asks the user to enter positive number until a negative number is entered. The function will use two referance parameters called "sum" and "max" to return the sum of all the positive numbers and the biggest number entered.arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
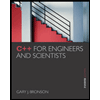
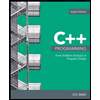