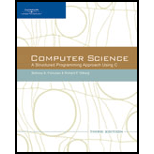
Explanation of Solution
Given: The following code:
// Header files.
#include
// Defining the function.
int main()
{
//Function declaration
int funA (int x);
int funB (int x);
//calling the main Function
int main(void)
{
//Local declarations
int a;
int b;
int c;
//Statements
a=10;
funB(a);
b=5;
c=funA(b);
funB(c);
printf("%3d %3d %3d", a, b, c);
return 0;
}
int funA (int x)
{
//Statements
return x*x;
}
void funB (int x)
{
//Local declarations
int y;
//Statements
y=x%2;
x/=2;
printf("\n%3d %3d\n", x, y);
return;
}
To find:Â The structure chart for the following code.
#include

Want to see the full answer?
Check out a sample textbook solution
Chapter 4 Solutions
Computer Science: A Structured Programming Approach Using C, Third Edition
- Book : Peter Sestof - Programming Language Concept Page: 158 pdf Exercise 8.9 Extend the language and compiler to accept initialized declarations such as int i = j + 32; Doing this for local variables (inside functions) should not be too hard. For global ones it requires more changes.arrow_forwardWrite a program in C++ using OOP, to create a class (student), that contain a private data (name, five grads). then create the following function: 1) (set_info()) to read the private data 2) (Print_info()) to Print student name and grades. 3) (Grade E()) as a friend function that calculate the grades average and print the student evaluation according to the following conditions: FAIL when (av=60 and av=70 and av 80 and av=90)arrow_forwardNote for programmer: 1.Write a separate main() function as a driver for each question. The driver should demonstrate all the required functionalities of a question. 2. Write a c++ code and do not use any String or Math libraries (such as cmath, cstring, string, etc.) and also do not use built-in functions (such as pow, etc.).arrow_forward
- Exercise Write a C++ program to develop a Vehicle Fine Management System for Police. The program will havethe following features.1. A user defined function called “Student Registration”. This function prompts a user to enter astudent’s personal data i.e., Student Number, Student Name, Age and City and stores them.2. A user defined function called “Module Enrolment”. This function takes student number from themain function as an argument and prompts a user to enter student two modules details i.e. Modulename, Module Code and Module Credits Hours (15 or 30) and stores them. The programs shoulddisplay an error message if a user enters credit hour other than 15 or 30.3. A user defined function called “Student Assessment”. This function takes student number from themain function as an argument and prompts a user to enter Module 1 and Module 2 marks and storesthem.4. A user defined function which takes a Student ID and search option (1 for Personal data search, 2 forModule Search and 3 for…arrow_forwardSet-up and implementation code for a void function MaxYou are not required to write a complete C++ program but must write and submit just your responses to the four specific function related questions below: QC1: Write the heading for a void function called Max that has three intparameters: num1, num2 and greatest. The first two parameters receive data from the caller, and greatest is used to return a value as a reference parameter. Document the data flow of the parameters with appropriate comments*. QC2: Write the function prototype for the function in QC1. QC3: Write the function definition of the function in QC1 so that it returns the greatest of the two input parameters via greatest, a reference parameter. QC4: Add comments to the function definition* you wrote in QC3 that also states its precondition and postcondition.arrow_forwardImplement C Programming 7.6.1: LAB: Simple car Given two integers that represent the miles to drive forward and the miles to drive in reverse as user inputs, create a SimpleCar variable that performs the following operations: Drives input number of miles forward Drives input number of miles in reverse Honks the horn Reports car status SimpleCar.h contains the struct definition and related function declarations. SimpleCar.c contains related function definitions. Ex: If the input is: 100 4 the output is: beep beep Car has driven: 96 miles #include <stdio.h> #include "SimpleCar.h" int main() { /* Type your code here. */ return 0;}arrow_forward
- Write a C language function DrivingCost() with input parameters milesPerGallon, dollarsPerGallon, and milesDriven, that returns the dollar cost to drive those miles. All items are of type double. The function called with arguments (20.0, 3.1599, 50.0) returns 7.89975. Define that function in a program whose inputs are the car's miles per gallon and the price of gas in dollars per gallon (both doubles). Output the gas cost for 10 miles, 50 miles, and 400 miles, by calling your DrivingCost() function three times. Output each floating-point value with two digits after the decimal point, which can be achieved as follows:printf("%0.2lf", yourValue); Ex: If the input is: 20.0 3.1599 the output is: 1.58 7.90 63.20 Your program must define and call a function:double DrivingCost(double milesPerGallon, double dollarsPerGallon, double milesDriven)arrow_forwardIn C++, write a simple software that computes the total points based on the Practical Examination Rubric Detail using functions inside a class. Create objects with three input arguments in the main function (SO1.3, SO6.1, and SO6.4, respectively) which values range from 1 to 4 (1 = Beginning, 2 = Developing, 3 = Accomplished, 4 = Exemplary). The sum of the related points will be displayed in the output.arrow_forwardAnswer the question with C - language. Question. In the following code, assume that all function calls are successful. void sigusr1_handler(int sig) { } int main(void) { pid_t child; if ((child = fork()) == 0) { struct sigaction sa; sa.sa_handler = sigusr1_handler; sa.sa_flags = 0; sigemptyset(&sa.sa_mask); sigaction(SIGUSR1, &sa, NULL); while (1) { printf("working ...\n"); sleep(1); } } else { //line 19 sleep(30); } // line 22 return 0; } (a) If you run the above program can it create either an orphan or a zombie process? Explain how. (b) Insert statements in only the part of the code related to the parent process (Lines 19–22) and function siguse1_handler() so that the child process would end after about 30 seconds of running. Clearly indicate where you insert any statements. thank you for your timearrow_forward
- code for this in C: Instructions: Given the function header, write the appropriate function call with variable declarations. 1. void printHello(void) 2. void depositSavings(float currentS, float addS) 3. void checkAgeBracket(int age, char gender)arrow_forwardAssume the following JavaScript program was interpreted using staticscoping rules. What value of x is displayed in function sub1? Underdynamic-scoping rules, what value of x is displayed in function sub1?var x;function sub1() {document.write("x = " + x + "");}function sub2() {var x;x = 10;sub1();}x = 5;sub2();arrow_forwardImplement C Programming 7.7.1: LAB: Vending machine Given two integers as user inputs that represent the number of drinks to buy and the number of bottles to restock, create a VendingMachine variable that performs the following operations: Purchases input number of drinks Restocks input number of bottles Reports inventory VendingMachine.h contains the struct definition and related function declarations. VendingMachine.c contains related function definitions. A VendingMachine's initial inventory is 20 drinks. Ex: If the input is: 5 2 the output is: Inventory: 17 bottles #include <stdio.h> #include "VendingMachine.h" int main() { /* Type your code here. */ return 0;}arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
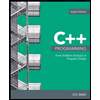
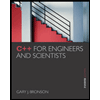