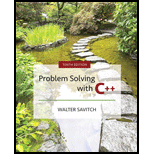
Explanation of Solution
The function “unitPrice()” with “static_cast<double>(2)” is executed in the C++ language which gives the output as follows,
// Include required header files
#include <iostream>
using namespace std;
// Declaration of unitPrice() function
double unitPrice(int diameter, double price);
// Definition of unitPrice() function
double unitPrice(int diameter, double price){
// Define a constant value for PI
const double PI = 3.14159;
// Declare the variables
double radius, area;
// Compute radius
radius = diameter/static_cast<double>(2);
// Compute area
area = PI * radius * radius;
// Compute the unit price and return the result
return (price/area);
}
// Definition of main() function
int main() {
// Call the unitPrice() function and display the result
cout << "Price: " << unitPrice(13, 14) <<"\n";
// Return 0
return 0;
}
Output for the above function is as follows,
Price: 0.105476
The function “unitPrice()” with the constant “2...

Want to see the full answer?
Check out a sample textbook solution
Chapter 4 Solutions
Problem Solving with C++ (10th Edition)
- Consider the following JavaScript skeletal program: //The main program var x; function sub1() { var x; function sub2() { . . . } } function sub3() { . . . } Assuming Dynamic scoping, which declaration of x will be used inthe following functions: a) sub1() b) sub2() c) sub3() You can consider the first declaration of x as x1 and the second as x2arrow_forwardGiven the Class Definition for ClockType discussed extensively in class, write what would have to be added to the IMPLEMENTATION FILE for the Class ClockType to overload the “= =”, i.e., the comparison “equal-equal sign,” here: That is, write the FULL FUNCTION DEFINITION for THE FUNCTION associated with Class ClockType to overload the “= =” remembering the private members are: b) int hr; // that contains the hours int min; // that contains the minutes int sec; // that contains the secondsarrow_forwardImplement the following in a C project structure: - A function called Grader that takes a numeric score and returns a letter grade. Grader has one argument of type integer and returns a value of type char. Use the rule that 90 to 100 is an A, 80 to 90 is a B, 70 to 79 is a C, and less than 70 is an F. - The greatest common divisor of two positive integers is the largest integer that divides them both. For example, the greatest common divisor of 9 and 6 is 3. Write a function declaration for a function with two integer arguments that returns their greatest common divisor. - Write a function that accepts a character argument and returns 1 if the argument is a valid letter(a-z/A-Z), otherwise returns 0. - Write a function declaration for a function that computes interest on a credit card account balance. The function has arguments for the initial balance, the monthly interest rate, and the number of months for which interest must be paid. The value returned is the interest due. Do…arrow_forward
- For 8 bits floating point representation using: seeeffff, what is the bias for normalized numbers? For 8 bits floating point representation using: seeeffff, what is the bias for normalized numbers? Question 69 options: A. 7 B. 6 C. 4 D. 3 E. 2 Which statement is true about static binding? A. the binding of a function call and the function definition occurs at compile time B. the binding of a function call and the function definition occurs at run time C. the binding of the type of a variable and the variable name occurs at run time D. the binding of the type of a variable and the variable name occurs at compile timearrow_forwarda.)Write a C++ program that consists of a function declaration, a function definition and a main. The function declaration is as follows: void endTime (int ch, int cm, char cap, int dh, int dm, int eh, int em, char eap)// given any current hour, minutes and 'A' or 'P' for am/pm in parameters ch, cm and cap respectively,// and also given the duration (hours and minutes) of an activity in parameters dh and dm,// the function stores the end time in parameters eh, em and eap. Where eh will hold the hour of the end time, em holds the minute and eap holds eithe 'A' or 'P' for the end time.// All values are using a 12 hour clock// Function assumes all activities end in the same day Also include a main program that feeds the following arguments to the functions:11, 25, 'A', 3, 45, hour, minute, ampm causing the function to calculate the end time as 3 10 PUsing the calculated results, the main then outputs:If the current time is 11:25AM and we have 3 hours and 45 minutes to go, we have to sit…arrow_forwardConsider the following JavaScript skeletal program: //The main program var x; function sub1() { var x; function sub2() { . . . } } function sub3() { . . . } Assuming Static scoping, which declaration of x will be used inthe following functions: a) sub1() b) sub2() c) sub3() You can consider the first declaration of x as x1 and the second as x2arrow_forward
- 2. David is used to doing programming in Python and since the print() function in Python by default prints a newline after printing the string passed to the print() function. But in his current project, he is using PHP programming language and the echo function does not print a newline by default after the end of the string. So, he often misses giving a newline after printing a message. Help David by writing a function myecho() that takes a string as a parameter and also prints a newline after printing the string.arrow_forwardWrite a function in (java based) Processing called calcDistance, that returns the distance between two points (x1, y1) and (x2, y2) on a 2-dimensional plane. The distance is calculated according to the formula: The coordinates and the distances are realvalues (your parameters and return types should reflect this).arrow_forwardGiven the code segment below, what should be the data type of the formal parameter in the function prototype of func(), given the call from main()? Note that function prototypes need not include the identifier (name of the parameter), so do NOT put any variable name in your answer. Remove any space in your answer. If the accessing is wrong, answer INVALID (in all capital letters). void func( ______ );int main(){ double aData[6][4];func(&aData[4][1]);return 0;}arrow_forward
- Question 2. Consider the following skeletal C program. void fun1(void) {int a, b, c;// call another function} void fun2(void) {int d, e, f;// call another function} void fun3(void) {int c, d, e;// call another function} void main () {int a, f, e;// call another function} Given the following calling sequences and assuming that dynamic scoping is used, whatvariables are visible during execution of the last function called? Include with each visiblevariable the name of the function in which it was defined. a. main calls fun1; fun1 calls fun3.b. main calls fun3; fun3 calls fun1.c. main calls fun1; fun1 calls fun3; fun3 calls fun2.d. main calls fun3; fun3 calls fun2; fun2 calls fun1arrow_forwardGiven the code segment below, what should be the data type of the formal parameter in the function prototype of func(), given the call from main()?Note that function prototypes need not include the identifier (name of the parameter), so do NOT put any variable name in your answer. Remove any space in your answer. If the accessing is wrong, answer INVALID (in all capital letters). void func( ______ );int main(){ double aData[6][4];func(&aData[4]);return 0;}arrow_forwardWrite a function with the interface doRandomWalk(nstep,startPosition), that takes the number of steps nstep for a random walk and the startPosition of the random walk on a straight line, and returns the location of the final step of the random walker. Problem Part B Now, write another function with the interface simulateRandomWalk(nsim,nstep,startPosition) that simulates nsim number of random-walks, each of which contains nstep steps and starts at startPosition. Then, this function calls doRandomWalk() repeatedly for nsim times and finally returns a vector of size nsimcontaining final locations of all of the nsim simulated random-walks. Problem Part C Now write a script that plots the output of simulateRandomWalk() for nsimnstepstartPosition===1000010−10 The resulting plot should look like the following, How do you interpret this result? How can uniformly-distributed random final steps end up having a Gaussian bell-shape distribution.arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
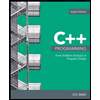