Bakery application
Program plan:
- Create new Windows Forms Application.
- Design the form by placing the labels, textbox, and button and then change their name and properties.
- Inside the “Calculate” button,
- Declare required variables.
- Assign the name to the variables.
- Calculate the items sold.
- Calculate the sub total
- Calculate the sales tax.
- Display total amounts.
- Display tax and sales clerk’s name.
- Inside the “Exit” button,
- Close the form.
- Inside the “btnPrint_Click”
- Print the sales receipt.
- Inside the “txtDate_Enter”
- Select all the values.
- Inside the “txtDate_keyPress”,
- Check the condition.
- Inside the “txtDonuts_Enter”,
- Select all the text.
- Inside the “CancelKeys”,
- Check the condition.
- Inside the “txtMuffins_Enter”,
- Select all the text.

This program is to modify the bakery application.
Explanation of Solution
Program:
'Definition of class frmMain
Public Class frmMain
'Definition of button calculate
Private Sub btnCalc_Click(sender As Object, e As EventArgs) Handles btnCalc.Click
' Calculate number of items sold and total sales
Const PromtMsg As String = "Salesclerk's name:"
Const title As String = "Name Entry"
Const ItemPrice As Decimal = 0.5D
Const tax As Decimal = 0.02D
Dim donuts As Integer
Dim muffins As Integer
Dim totalItems As Integer
Dim subTotal As Decimal
Dim salesTax As Decimal
Dim totalSales As Decimal
Static clerk As String
'Assign the name to variable
clerk = InputBox(PromtMsg, title, clerk)
' Calualte the items sold
Integer.TryParse(txtDonuts.Text, donuts)
Integer.TryParse(txtMuffins.Text, muffins)
totalItems = donuts + muffins
' calculate the subtotal
subTotal = totalItems * ItemPrice
' calculate the sales tax
salesTax = subTotal * tax
' calculate the total sales
totalSales = subTotal + salesTax
' display total amounts
lblTotalItems.Text = Convert.ToString(totalItems)
lblTotalSales.Text = totalSales.ToString("C2")
' display tax and salesclerk's name
lblMsg.Text = "The sales tax was " &
salesTax.ToString("C2") & "." &
ControlChars.NewLine & clerk
End Sub
'Definition of button clear
Private Sub btnClear_Click(sender As Object, e As EventArgs) Handles btnClear.Click
'Assign text box to empty
txtDonuts.Text = String.Empty
txtMuffins.Text = String.Empty
lblTotalItems.Text = String.Empty
lblTotalSales.Text = String.Empty
lblMsg.Text = String.Empty
' send the focus to the Doughnuts box
txtDonuts.Focus()
End Sub
'Definition of button exit
Private Sub btnExit_Click(sender As Object, e As EventArgs) Handles btnExit.Click
'Close the form
Me.Close()
End Sub
'Definition of button print
Private Sub btnPrint_Click(sender As Object, e As EventArgs) Handles btnPrint.Click
'print the sales receipt
btnCalc.Visible = False
btnClear.Visible = False
btnExit.Visible = False
btnPrint.Visible = False
PrintForm1.Print()
btnCalc.Visible = True
btnClear.Visible = True
btnExit.Visible = True
btnPrint.Visible = True
End Sub
'Definition of ClearLabels
Private Sub ClearLabels(sender As Object, e As EventArgs) _
Handles txtDonuts.TextChanged, txtMuffins.TextChanged
' Clear the total items, total sales, and message
lblTotalItems.Text = String.Empty
lblTotalSales.Text = String.Empty
lblMsg.Text = String.Empty
End Sub
'Definition of txtDate_Enter
Private Sub txtDate_Enter(sender As Object, e As EventArgs) Handles txtDate.Enter
'Select all the values
txtDate.SelectAll()
End Sub
'Defintion of txtDate_keyPress
Private Sub txtDate_KeyPress(sender As Object, e As KeyPressEventArgs) Handles txtDate.KeyPress
'Check the conditon
If (e.KeyChar < "0" OrElse e.KeyChar > "9") AndAlso
e.KeyChar <> "/" AndAlso e.KeyChar <> "-" AndAlso
e.KeyChar <> ControlChars.Back Then
e.Handled = True
End If
End Sub
'Definition of txtDonuts_Enter
Private Sub txtDonuts_Enter(sender As Object, e As EventArgs) Handles txtDonuts.Enter
'Select all the text
txtDonuts.SelectAll()
End Sub
'Defintion of CancelKeys
Private Sub CancelKeys(sender As Object, e As KeyPressEventArgs) Handles txtDonuts.KeyPress, txtMuffins.KeyPress
'Check the condition
If (e.KeyChar < "0" OrElse e.KeyChar > "9") AndAlso e.KeyChar <> ControlChars.Back Then
e.Handled = True
End If
End Sub
'Definition of txtMuffins_Enter
Private Sub txtMuffins_Enter(sender As Object, e As EventArgs) Handles txtMuffins.Enter
'Select all the text
txtMuffins.SelectAll()
End Sub
End Class
Run the program, enter the date, number of Doughnuts and Muffins and then click on the “Calculate” button.
Screenshot of form
Want to see more full solutions like this?
Chapter 4 Solutions
PROGRAMMING WITH MICROSOFT VIS PKG
- Which property determines the tab order for the controls in an interface? a. SetOrder b. SetTab c. TabIndex d. TabOrderarrow_forwardWhich menu item allows you to view the interface's TabIndex boxes?arrow_forwardWhich property determines the tab order for the controls in an interface?a. SetIndex b. SetOrder c. TabIndex d. TabOrderarrow_forward
- This is the question I am stuck on - Add a prompt to the ShadyRestRoom application to ask the user to specify a (1) lake view or a (2) park view, but ask only if the bed size entry is valid. Add $15 to the price of any room with a lake view. If the view value is invalid, display a you selected an invalid option and assume that the price is for a room with a lake view. This is the code I have from the previous probelm but I am unsure how to add all that onto it - import java.util.Scanner; public class ShadyRestRoom2 { // Modify the code below public static void main (String args[]) { int selection; int price; String result; final int QUEEN = 1, KING = 2, SUITE = 3; final int QPRICE = 125, KPRICE = 139, SPRICE = 165; final String QSTRING = "Queen bed", KSTRING = "King bed", SSTRING = "Suite with a king bed and pull-out couch", INVALIDSTRING = "an invalid option"; Scanner in = new Scanner(System.in);…arrow_forwardMFC In the second part of your project, you need to develop a mathematical worksheet graphical interface for elementary school students. The worksheet consists of 10 questions of operations between two random numbers. The operations are defined randomly from the set {+, -, *, /}. The GUI uses a text field to enter the maximum value of random numbers. A button with the caption "Check Answers" should be added to the GUI, and when clicked, a correct icon () should appear in front of the correct answer and an incorrect iconarrow_forwardEnhance the Fahrenheit to Celsius applicationIn this assignment, you’ll add data validation to the application you created in Assignment 1. You’ll also let the user do multiple conversions before ending the application. This is the dialog box for an invalid entry: 1. If you didn’t already do Assignment 1, do it now.2. Add data validation to the application so it won’t do the conversion until the user enters aFahrenheit temperature between -100 and 212. If the entry is invalid, a dialog box like theone above should be displayed.3. Add a loop to the code so the user can do a series of calculations without restarting theapplication. To end the application, the user must enter 999 as the temperaturearrow_forward
- When a form has been modifi ed since the last time it was saved, whatappears on its tab in the designer window?arrow_forwardWrite an application that allows the user to choose insurance options inJCheckBoxes. Use a ButtonGroup to allow the user to select only one of twoinsurance types—HMO (health maintenance organization) or PPO (preferredprovider organization). Use regular (single) JCheckBoxes for dental insurance andvision insurance options; the user can select one option, both options, or neitheroption. As the user selects each option, display its name and price in a text field;the HMO costs $200 per month, the PPO costs $600 per month, the dentalcoverage adds $75 per month, and the vision care adds $20 per month. When auser deselects an item, make the text field blank. Be sure to include & test any and all Exception Handling. Save the file as JInsurance.java.arrow_forwardIn Visual Basic, when you drag a field object to an existing control in the interface, Visual Basic replaces the current control with the newly created control. True or false? a. True b. Falsearrow_forward
- Programming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
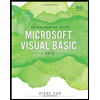
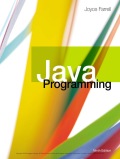
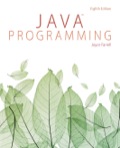