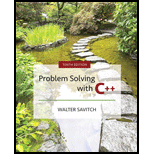
Write a
bool isLeapYear(int year);
This function should return true if year is a leap year and false if it is not. Here is pseudocode to determine a leap year:
leapYear = (year divisible by 400) or (year divisible by 4 and year not divisible by 100))
int getCenturyValue(int year);
This function should take the first two digits of the year (that is. the century), divide by 4. and save the remainder. Subtract the remainder from 3 and return this value multiplied by 2. For example, the year 2008 becomes: (20/4) = 5 with a remainder of 0. 3 - 0 = 3. Return 3*2 = 6.
int getYearValue(int year);
This function computes a value based on the years since the beginning of the century. First, extract the last two digits of the year. For example, 08 is extracted for 2008. Next, factor in leap years. Divide the value from the previous Step by 4 and discard the remainder. Add the two results together and return this value. For example, from 2008 we extract 08. Then (8/4) = 2 with a remainder of 0. Return 2 + 8=10.
int getMonthVa0lue(int month, int year);
This function should return a value based on the table below and will require invoking the isLeapYear function.
Month | Return Value |
January | 0 (6 if year is a leap year) |
February | 3 (2 if year is a leap year) |
March | 3 |
April | 6 |
May | 1 |
June | 4 |
July | 6 |
August | 2 |
September | 5 |
October | 0 |
November | 3 |
December | 5 |
Finally, to compute the day of the week, compute the sum of the date’s day plus the values returned by getMonthValue, getYearValue, and getCenturyValue. Divide the sum by 7 and compute the remainder. A remainder of 0 corresponds to Sunday, 1 corresponds to Monday, etc., up to 6, which corresponds to Saturday. For example, the date July 4, 2008 should be computed as (day of month) 1 (getMonthValue) 1 (getYearValue) 1 (getCenturyValue) = 4 + 6 + 10 + 6 = 26. 26/7 = 3 with a remainder of 5.
The fifth day of the week corresponds to Friday.
Your program should allow the user to enter any date and output the corresponding day of the week in English.
This program should include a void function named getInput that prompts the user for the date and returns the month, day, and year using pass-by-reference Parameters. You may choose to have the user enter the date’s month as either a number (1–12) or a month name.

Want to see the full answer?
Check out a sample textbook solution
Chapter 5 Solutions
Problem Solving with C++ (10th Edition)
Additional Engineering Textbook Solutions
Starting Out with C++: Early Objects
Starting Out with Java: From Control Structures through Objects (6th Edition)
Software Engineering (10th Edition)
Experiencing MIS
Introduction To Programming Using Visual Basic (11th Edition)
Concepts of Programming Languages (11th Edition)
- Write a program that takes in a positive integer as input, and outputs a string of 1's and 0's representing the integer in binary. For an integer x, the algorithm is: As long as x is greater than 0 Output x % 2 (remainder is either 0 or 1) x = x / 2 Note: The above algorithm outputs the 0's and 1's in reverse order. You will need to write a second function to reverse the string. Ex: If the input is: 6 the output is: 110 Your program must define and call the following two functions. The IntegerToReverseBinary() function should return a string of 1's and 0's representing the integer in binary (in reverse). The ReverseString() function should return a string representing the input string in reverse. string IntegerToReverseBinary(int integerValue)string ReverseString(string userString) #include <iostream>using namespace std; /* Define your functions here */ int main() {/* Type your code here. Your code must call the functions. */ return 0;} Please help me with this problem using…arrow_forwardWrite a program that takes in a positive integer as input, and outputs a string of 1's and 0's representing the integer in binary. For an integer x, the algorithm is: As long as x is greater than 0 Output x % 2 (remainder is either 0 or 1) x = x // 2 Note: The above algorithm outputs the 0's and 1's in reverse order. You will need to write a second function to reverse the string. Ex: If the input is: 6 the output is: 110 Your program must define and call the following two functions. The function integer_to_reverse_binary() should return a string of 1's and 0's representing the integer in binary (in reverse). The function reverse_string() should return a string representing the input string in reverse.def integer_to_reverse_binary(integer_value)def reverse_string(input_string) Note: This is a lab from a previous chapter that now requires the use of a function. def integer_to_reverse_binary(integer_value): if number > 0: number % 2 number = number // 2 return number def…arrow_forwardWrite a program, which takes a positive integer as input, and prints which powers of 2 does the numberlie between. For example, the number 269 lies between 28(256) and 29(512). If the input is 269, theoutput should be 8 9. Borderline cases which are powers of 2, such as 256, should be aligned to the lowerlimit of the desired range output, i.e. 28(256). You should not use math functions such as pow.arrow_forward
- Write a program that takes in a positive integer as input, and outputs a string of 1's and 0's representing the integer in binary. For an integer x, the algorithm is: As long as x is greater than 0 Output x % 2 (remainder is either 0 or 1) x = x / 2 Note: The above algorithm outputs the 0's and 1's in reverse order. You will need to write a second function to reverse the string. Ex: If the input is: 6 the output is: 110arrow_forwardWrite a program that reads an integer age of kids, finds the maximum of them, and counts its occurances, finds the minimum of them, and counts its occurances. And Find the average ages. Assum that the input ends with number -1.arrow_forwardCode in Python Write a function, print_integers_less_than(n), which takes an integer parameter n and prints each integer k which is at least 0 and is less than n, in ascending order. Hint: use a simple for loop. For example: Test Result print_integers_less_than(2) 0 1 print_integers_less_than(5) 0 1 2 3 4 print_integers_less_than(-3)arrow_forward
- Write a program in python with a function repeatPhrase(phrase, n), which prints the given phrase n times, alternating between lowercase and uppercase. For example, repeat('The sky is yellow', 5) would print: the sky is yellowTHE SKY IS YELLOW the sky is yellowTHE SKY IS YELLOW the sky is yellowarrow_forwardWrite a program that takes in a positive integer as input, and outputs a string of 1's and 0's representing the integer in binary. For an integer x, the algorithm is: As long as x is greater than 0 Output x % 2 (remainder is either 0 or 1) x = x // 2 Note: The above algorithm outputs the 0's and 1's in reverse order. You will need to write a second function to reverse the string. Ex: If the input is: 6 the output is: 110 The program must define and call the following two functions. Define a function named int_to_reverse_binary() that takes an integer as a parameter and returns a string of 1's and 0's representing the integer in binary (in reverse). Define a function named string_reverse() that takes an input string as a parameter and returns a string representing the input string in reverse.def int_to_reverse_binary(integer_value)def string_reverse(input_string) starting with # Define your functions here. if __name__ == '__main__': # Type your code here. # Your code must call…arrow_forwardWrite a program that takes in a positive integer as input, and outputs a string of 1's and 0's representing the integer in binary. For an integer x, the algorithm is: As long as x is greater than 0 Output x % 2 (remainder is either 0 or 1) x = x // 2 Note: The above algorithm outputs the 0's and 1's in reverse order. You will need to write a second function to reverse the string. Ex: If the input is: 6 the output is: 110 The program must define and call the following two functions. Define a function named int_to_reverse_binary() that takes an integer as a parameter and returns a string of 1's and 0's representing the integer in binary (in reverse). Define a function named string_reverse() that takes an input string as a parameter and returns a string representing the input string in reverse.def int_to_reverse_binary(integer_value)def string_reverse(input_string)arrow_forward
- Write a program in Python where we ask the reader to enter five positive numbers and then print thelargest one. (Hint: first write a input variable for the largest number, then write a range function.The range function should have another input variable for number input. Then do mathematicalcomparisons.)arrow_forwardWrite a program whose input is two integers, and whose output is the first integer and subsequent increments of 10 as long as the value is less than or equal to the second integer. Ex: If the input is -15 30, the output is: -15 -5 5 15 25 Ex: If the second integer is less than the first as in 20 5, the output is: Second integer can't be less than the first. For coding simplicity, output a space after every integer, including the last.arrow_forwardWrite a program that reads an integer and displays all its smallest factors in an increasing order. For example, if the input integer is 120, the output should be as follows: 2, 2, 2, 3, 5.arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
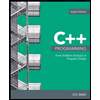