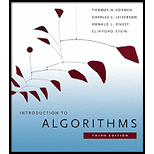
Concept explainers
(a)
To show the difference between the output of the array when it is passed as an array for BUILD-MAX-HEAP and BUILD-MAX-HEAP'.
(a)

Explanation of Solution
Given Information: A heap that has n-elements.
Explanation:
Pseudo code for BUILD-MAX-HEAP is given below:
BUILD-MAX-HEAP( A ) |
A.heapsize = A.length |
for i = A.length/2 downto 1 |
MAX-HEAPIFY( A,i ) |
Consider an array A= {1, 2, 3, 4, 5, 6}. If the above
The for-loop will iterate from 3 to 1.
At i=3 , MAX-HEAPIFY will be called with arguments as array A and i=3 . The following pseudo code for MAX-HEAPIFY is as follows:
MAX-HEAPIFY( A,i ) |
l = LEFT( i ) |
r = RIGHT( i ) |
ifl <= A.heapsize and A[l]> A[i] |
largest = l |
Else |
largest = i |
ifr <= A.heapsize and A[r]> A[largest] |
largest=r |
iflargest != i |
exchange A [ i ] with A [ largest ] |
MAX-HEAPIFY( A,largest ) |
Currently at i=3 , A [3]=3 and have the following tree:
At the end of the iteration:
At i=2 , A [2]=2 and after executing the algorithm, the following tree is evolved:
At i=1 , A [1]=1 and after executing the algorithm, the following tree is evolved:
Resultant array after BUILD-MAX-HEAP is A= {6, 5, 3, 4, 2, 1}
However, according to the question, the algorithm for BUILD-MAX-HEAP ', there is an alteration in A.heapsize, which is by default 1. Moreover, the value of i starts from 2 and goes on till A.length that is 6.
At i=2 , A [2]=2. The tree is given below-
Look at the algorithm of BUILD-MAX-HEAP' . It is using MAX-HEAP-INSERT. So, it becomes-
At i=3 , A [3]=3 and after using MAX-HEAP-INSERT
At i=4 , A [4]=4 and after using MAX-HEAP-INSERT
At i=5 , A [5]=5 and after using MAX-HEAP-INSERT
Finally at i=6,A [6]=6 and after using MAX-HEAP-INSERT
Resultant array after BUILD-MAX-HEAP' is A= {6, 4, 5, 1, 3, 2}
Hence, the resultant array after BUILD-MAX-HEAP and BUILD-MAX-HEAP' is not the same.
(b)
To determine the worst case scenario for BUILD-MAX-HEAP' while entering n-elements.
(b)

Explanation of Solution
The BUILD-MAX-HEAP' has MAX-HEAP-INSERT in it, which has worst case of Θ(log n). The call to this function is done for n-1 times, since it is not considering the parent node here.
The worst case for MAX-HEAP-INSERT happens when an array is sorted in ascending order.
Each insert step takes maximum Θ(log n ) steps, and since it is done for n times, it takes a worst case of Θ(nlog n ). Each iteration in the new block is actually taking more time as the older version as it has more iteration in it. The new block will work in even work in even worse case as it has the usage of other algorithm as well.
Want to see more full solutions like this?
Chapter 6 Solutions
Introduction to Algorithms
- What does the initially empty max-heap h contain after the following sequence of pseudo-code operations? Show at least 5 binary heaps as stated in Figure 1 to show the changes after a sequence of heap operations.arrow_forwardWe can build a heap by top-down (iterated insertion), or by bottom-up. Given the numbers 10, 9, 12, 13, 14, 8 a. run the bottom-up algorithm to create a heap and show the resulting heap and ALLsteps b. run the top-down algorithm to create heap and show the resulting heap and ALLstepsarrow_forwardA d-heap is similar to a binary heap, except that nodes can have up to d children. Suppose d = 3, insert the following items into a max 3-heap: 10, 2, 6, 20, 9, 25, 12, 19. Show all the steps.arrow_forward
- Design and implement a data structure Median − Heap to maintain a collection of numbers S that supports Build(S), Insert(x), Extract(), and Peek() operations, defined as follows: Build(S): Produces, in linear time, a data structure Median − Heap from an unordered input array S. For implementing Build(S), you can assume access to the procedure Find_Median(S), which finds the median of S in linear time. Insert(x): Insert element x into Median − Heap in O(log n) time. Peek(): Returns, in O(1) time, the value of the median of Median − Heap. Extract(): Remove and return, in O(log n) time, the value of the median element in Median − Heap.arrow_forwardAs we already studied different algorithms associated with binary heap. In place of binary heap,now consider an n-ary heap. Re-write following algorithms and their time complexities based on theproperties of n-ary heap. (i) Parent (i)(ii) index of kth child of node i(iii) indices of leaf nodes(iv) Max-Heapify (A, i) (v) Build-Max-Heap (A)arrow_forwardConsider a min heap with 1023 distinct integers. If root is at Oth level then fill the blank given in belowsentence. The 10th minimum of the heap might be at _____ level. A:1 B:3 C:8 D.9arrow_forward
- Suppose that H is a min-heap, and we have a pointer (index of the array representation) to an element in the heap. If we decrease the value of that element, the heap properties may be violated. Give an algorithm to restore the properties of the heap, given the index of the element to change and the new value of the element. You may assume that the new value is smaller than the original value of the element. The algorithm should have complexity Θ(log n).arrow_forwardAn input array B contains 2k-1 distinct elements (k is a positive integer). Allelements in B are arranged in ascending order. The constructHeap algorithm inHeapsort is applied to B to construct a maximizing heap. Analyze how many keycomparisons are performed in this heap construction. Your result must be in termsof k.arrow_forward.1. Draw the binary min-heap tree that results from inserting 65, 12, 73, 36, 30, 55, 24, 92 in that order into an initially empty binary min heap. BOX IN YOUR FINAL BINARY MIN HEAP. .2. Using the final binary min-heap above, show the result of performing a dequeue( ) operation, assuming a priority queue is implemented using a binary min-heap. BOX IN YOUR FINAL BINARY MIN HEAP.arrow_forward
- USING LANGUAGE C Implement a heap by inserting the following nodes in this order: 11, 5, 13, 3, 7, 6, 77, 1, 4, 222 ▪Print the heap after each insertion. ▪Dequeue each node and print each deleted node. ▪Your final output should be similar to the screen shot in the next slidearrow_forwardWhat will be the position of 5, when a max heap is constructed on the input elements 5, 70, 45, 7, 12, 15, 13, 65, 30, 25?a) 5 will be at rootb) 5 will be at last levelc) 5 will be at second leveld) 5 can be anywhere in heaparrow_forwardSuppose we use a 0-based array h[] to implement a heap of 200 elements. If an element of the heap is stored in h[71], the parent of this element can be found at h[]? Suppose we use a 0-based array h[] to implement a heap of 200 elements. If an element of the heap is stored in h[71], the left child of this element can be found at h[]? Suppose we use a 0-based array h[] to implement a heap of 200 elements. If an element of the heap is stored in h[71], the right child of this element can be found at h[]?arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
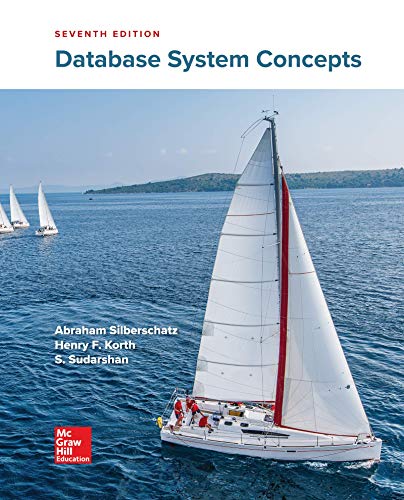
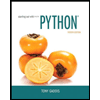
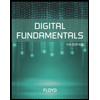
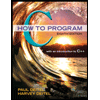
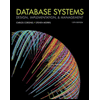
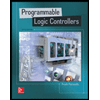