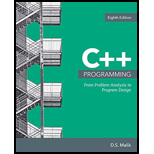
Concept explainers
Mark the following statements as true or false:
To use a predefined function in a program, you need to know only the name of the function and how to use it. (1)
A value-returning function returns only one value. (2, 3)
Parameters allow you to use different values each time the function is called. (2, 7, 9)
When a return statement executes in a user-defined function, the function immediately exits. (3, 4)
A value-returning function returns only integer values. (4)
A variable name cannot be passed to a value parameter. (3, 6)
If a C++ function does not use parameters, parentheses around the empty parameter list are still required. (2, 3, 6)
In C + +, the names of the corresponding formal and actual parameters must be the same. (3, 4, 6)
A function that changes the value of a reference parameter also changes the value of the actual parameter. (7)
Whenever the value of a reference parameter changes, the value of the actual parameter changes. (7)
In C++, function definitions can be nested; that is, the definition of one function can be enclosed in the body of another function. (9)
Using global variables in a program is a better
programming style than using local variables, because extra variables can be avoided. (10)In a program, global constants are as dangerous as global variables. (10)
The memory for a static variable remains allocated between function calls. (11)
(a)

To find whether the given statement is true or false.
False.
Explanation of Solution
Given information:
The name of the function and the definition of the function are given.
Explanation:
To use a predefined function in a program, the complete signature of a function should be known. The signature of a function comprises the return type, the name of the function, and the number and data types of the parameters required by the function if any.
Hence, the given statement is FALSE.
(b)

To find whether the given statement is true or false.
True.
Explanation of Solution
Given information:
The function returns a value hence the return type is not void.
Explanation:
A function can either return a value or not return any value. A function that does not returns a value has the return type of void whereas a function that returns a value has a return type such as int, float, and so on. The return type can be any valid data type supported by the language.
A non-void function can have only one return statement. That means, a function can return only one value.
Hence, the given statement is TRUE.
(c)

To find whether the given statement is true or false.
True.
Explanation of Solution
Given information:
A function that takes parameters.
Explanation:
A function that takes parameters can take any value of the specified data type of the parameter. Hence, such a function can be called many times using a different set of values, and calling the same function multiple times with a different list of parameters is called function overloading.
An example program is as follows:
void display(int n) { cout<<n<<endl; } int main() { display(1); display(2); return 0; }
Output:
The output of the above example program is as follows:
1
2
In the above program, the display() function is called multiple times with different values and generates different outputs.
Conclusion:
Hence, the given statement is TRUE.
(d)

To find whether the given statement is true or false.
True.
Explanation of Solution
Given information:
A function that returns a value.
Explanation:
A function that returns a value ends with a return statement. The return statement is the last in a value-returning function. The return statement returns a value and passes the control outside of the function. Therefore, it can be said that after executing the return statement, the function exits.
Hence, the given statement is TRUE.
(e)

To find whether the given statement is true or false.
False.
Explanation of Solution
Given information:
A function that returns a value.
Explanation:
The nature of the value returned by a function depends upon the return type of the function. The return type of a function can be any valid data type supported by the language. It can be of primitive data type such as int, float, char, and so on and it can also be of a user-defined type such as an object.
Conclusion:
Therefore, a value-returning function will always return a value having type as of return type of function and will not always be an integer. Hence, the given statement is FALSE.
(f)

To find whether the given statement is true or false.
True.
Explanation of Solution
Given information:
A function that takes parameters.
Explanation:
A function that takes value parameters must be passed values and not variable names. A variable name can be passed as a reference variable using the & symbol. This is not acceptable to a function that takes value parameters.
Hence, the given statement is TRUE.
(g)

To find whether the given statement is true or false.
True.
Explanation of Solution
Given information:
A function that does not takes parameters.
Explanation:
A set of parenthesis identifies a function irrespective of whether a function takes parameters or not. A function is always defined and declared using the parenthesis after the function name. If a function is not having any parameters, then the parenthesis will be blank otherwise variables can be declared in the parenthesis. Hence, parentheses in a function are always required.
Thus, the given statement is TRUE.
(h)

To find whether the given statement is true or false.
False.
Explanation of Solution
Given information:
A function that takes parameters.
Explanation:
When a function takes parameters, the formal variables refer to the names of the parameters included in the function definition. While the actual variables are the names of the variables that are passed as parameters while calling the parameterized function.
When a function that takes parameters is called, the parameters are passed either using direct values or variable names that have been initialized. In case, variables are passed when calling the parameterized function, the name of the actual variables doesn't have to be the same as the names of the formal variables.
Hence, the given statement is FALSE.
(i)

To find whether the given statement is true or false.
True.
Explanation of Solution
Given information:
A function that takes parameters.
Explanation:
When a variable is passed by reference to a parameterized function, the address of the actual parameter is passed via the reference variable. Any change made to the value at the given address will reflected in the actual parameter also.
Hence, the given statement is TRUE.
(j)

To find whether the given statement is true or false.
True.
Explanation of Solution
Given information:
A function that takes parameters by reference.
Explanation:
Whenever a function to which parameters are passed by reference, the address of the actual parameters is passed. Any change to the value at the given address will reflect in the actual parameter.
Hence, the given statement is TRUE.
(k)

To find whether the given statement is true or false.
True.
Explanation of Solution
Given information:
Nested functions.
Explanation:
C++ allows functions to be nested. That is, the body of one function can be placed inside the body of another function.
An example program is as follows:
int main() { void outside() { cout << “outside” << endl; void inside() { cout << “inside” << endl; } } inside(); return 0; }
Output:
inside
outside
In the above example, it is seen that the inside() function is defined within the outside() function. While calling the function, the function that is nested, that is inside() function is called.
Conclusion:
Hence, the given statement is TRUE.
(l)

To find whether the given statement is true or false.
False.
Explanation of Solution
Given information:
Global variables.
Explanation:
C++ allows global variables to be used in a program. However, there are some issues associated with the usage of global variables. First, the use of global variables makes the maintenance of the code difficult. Secondly, it is difficult to track global variables throughout the program. To avoid such issues, local variables are used.
Hence, the given statement is FALSE.
(m)

To find whether the given statement is true or false.
False.
Explanation of Solution
Given information:
Global variables.
Explanation:
C++ allows global constants to be used in a program. Such constants are declared at the beginning of the program outside all the functions. Such constants help in the faster execution of the program and make the code compact since the constant is defined only once throughout the program.
Hence, the given statement is FALSE.
(n)

To find whether the given statement is true or false.
True.
Explanation of Solution
Given information:
Static variables.
Explanation:
C++ allows static variables in a program. The value of a static variable remains unchanged in between the function calls. This is possible since the memory remains allocated to the static variables even between the function calls.
Hence, the given statement is TRUE.
Want to see more full solutions like this?
Chapter 6 Solutions
C++ Programming: From Problem Analysis To Program Design, Loose-leaf Version
- (Numerical) Heron’s formula for the area, A, of a triangle with sides of length a, b, and c is A=s(sa)(sb)(sc) where s=(a+b+c)2 Write, test, and execute a function that accepts the values of a, b, and c as parameters from a calling function, and then calculates the values of sand[s(sa)(sb)(sc)]. If this quantity is positive, the function calculates A. If the quantity is negative, a, b, and c do not form a triangle, and the function should set A=1. The value of A should be returned by the function.arrow_forwardWhy do you need to include function prototypes in a program that contains user-defined functions? (5)arrow_forwardPlease circle True or False for each of the following statements. C++ Local variables used in a function are defined in the parameter list of that functions definition. TRUE FALSEarrow_forward
- Examine the following function header, and then write a statement that calls the function, passing 12 as an argument.def show_value(quantity):arrow_forwardPlease help me with my homework, in computer programming as follows: A number, a is a power of b if it is divisible by b and a/b is a power of b. Write a function called is_power that takes parameters a and b and returns True if a is a power of b. Note: you will have to think of the base case.arrow_forwardWhen calling a function with several arguments, parameter order matters.arrow_forward
- Calling a function and defining a function mean the same thing True or Falsearrow_forwardCould you write the function comment. Please explain what the function does step-by-step. Thanks!arrow_forwardTopic: A function with parameters passed by value and passed by reference Do not use anything beyond Chapter 6 materials. Write a C++ Write a single function rectangleAreaPerim (width, height, area, perimeter) that calculates both the area and the perimeter of a rectangle given its width and height. -Input parameters to the functions are the width and height of the rectangle. Function cannot modify the input parameters. -Output parameters of the function are the area, perimeter, and a boolean return (success or failure). -This function returns true if both width and height are not negative, and thus calculation is required. Otherwise the function returns false and skips all calculations. -The function rectangleAreaPerim(width, height, area, perimeter) should Not use any "cin" or "cout". Only the main() function will contain "cin" and "cout". -The main() function calls the function rectangleAreaPerim() in a loop until the user enters 0 0. These are "Sentinel" values that stop the…arrow_forward
- Please fill in the blanks for the following statements: If a function does not return a value its return type is __________________.arrow_forwardIf you are writing a function that accepts an argument and you want to make sure thefunction cannot change the value of the argument, what do you do?arrow_forwardWhen passing many arguments to a function, does it matter in what sequence those arguments are passed?arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
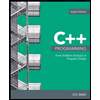
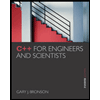