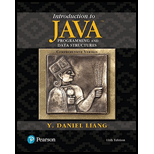
(Financial: credit card number validation) Credit card numbers follow certain patterns. A credit card number must have between 13 and 16 digits. It must start with
- for Visa cards
- for Master cards
- for American Express cards
- for Discover cards
In 1954, Hans Luhn of IBM proposed an
- 1. Double every second digit from right to left. If doubling of a digit results in a two-digit number, add up the two digits to get a single-digit number.
- 2. Now add all single-digit numbers from Step 1.
4 + 4 + 8 + 2 + 3 + 1 + 7 + 8 = 37
- 3. Add all digits in the odd places from right to left in the card number.
6 + 6 + 0 + 8 + 0 + 7 + 8 + 3 = 38
- 4. Sum the results from Step 2 and Step 3.
37+38=75
- 5. If the result from Step 4 is divisible by 10, the card number is valid; otherwise, it is invalid. For example, the number 4388576018402626 is invalid, but the number 4388576018410707 is valid.
Write a
/** Return true if the card number is valid */
public static boolean isValid(long number)
/** Get the result from Step 2 */
public static int sumOfDoubleEvenPlace(long number)
/** Return this number if it is a single digit, otherwise, • return the sum of the two digits */
public static int getDigit(int number)
/** Return sum of odd-place digits in number */
public static int sumOfOddPlace(long number)
/** Return true if the number dis a prefix for number *I
public static boolean prefixMatched(long number, int d)
/** Return the number of digits in d */
public static int getSize(long d)
/ ** Return the first k number of digits from number. If the • number of digits in number is less than k, return number. */
public static long getPrefix(long number , int k)
Here are sample runs of the program: (You may also implement this program by reading the input as a string and processing the string to validate the credit card.)

Trending nowThis is a popular solution!

Chapter 6 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Additional Engineering Textbook Solutions
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
Starting Out with Programming Logic and Design (4th Edition)
Modern Database Management
Starting Out with C++ from Control Structures to Objects (8th Edition)
Starting Out With Visual Basic (7th Edition)
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
- can you please type the codeto the following questionarrow_forward- Write a for loop with index i that iterates over all consecutive integers from 4 to 10 and prints the current loop index to screen for each value of i. As always, be careful with indentation and use proper python syntax. - Same question as above, but add a logical (if) statement inside the loop that prints both the loop index and the message "is greater than 6" or "is less than or equal to 6" for the appropriate indices. Hint, use the syntax print(i, "string") to print both the loop index and the string at the same time.arrow_forward- Write a for loop with index i that iterates over all consecutive integers from 4 to 10 and prints the current loop index to screen for each value of i. As always, be careful with indentation and use proper python syntax. - Same question as above, but add a logical (if) statement inside the loop that prints both the loop index and the message "is greater than 6" or "is less than or equal to 6" for the appropriate indices. Hint, use the syntax print(i, "string") to print both the loop index and the string at the same time. - Write the same for loop as in the first question above, but modify it to only print the loop index when i is not equal to 8.arrow_forward
- Rewrite the following statements using combined assignment operators: length = length / 2;arrow_forwardState whether the following are true or false. If the answer is false, explain why.b) The break statement is required in the default case of a switch selection statement.arrow_forwardProgram - python A nutritionist who works for a fitness club helps members by evaluating their diets. As part of her evaluation, she asks members for the number of fat grams and carbohydrate grams that they consumed in a day. Then, she calculates the number of calories that result from the fat, using the following formula: calories from fat = fat grams x 9. Next, she calculates the number of calories that result from the carbohydrates, using the following formula: calories from carbs = carb grams x 4. Write a program that will make these calculations. Your program should use two separate functions to calculate fat and carb calories. Write a 3rd function that is called from main to capture user input and call the others.arrow_forward
- 4. Odd-Even-inator by CodeChum Admin My friends are geeking out with this new device I invented. It checks if a number is even or odd! ? Do you want to try it out? Instructions: In the code editor, you are provided with a function that checks whether a number is even or odd. Your task is to ask the user for the number of integer, n, they want to input and then the actual n number values. For each of the number, check whether it is even or odd using the function provided for you. Make sure to print the correct, required message. Input 1. Integer n 2. N integer valuesarrow_forwardPlease, solve the following problem and explain how the loop works .arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
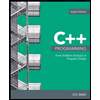