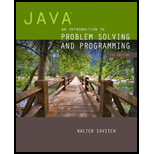
Java: Introduction to Problem Solving and Programming
7th Edition
ISBN: 9780133834604
Author: SAVITCH
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 8, Problem 4PP
Program Plan Intro
Displaying right triangle, rectangle, and square along with their areas and perimeters
Program Plan:
Filename: Test.java
- • Define main function.
- ○ Create the objects for “RightTriangle”, “Rectangle”, and “Square” classes and pass the input value to the respective methods.
- ○ Call the “drawHere” method with “RightTriangle” object “rt”.
- ○ Call the “findArea” and “findCircumference” methods with “RightTriangle” object “rt”.
- ○ Call the “drawHere”, and “drawAt” methods with “Rectangle” object “r”.
- ○ Call the “findArea” and “findCircumference” methods with “Rectangle” object “r”.
- ○ Call the “drawHere”, and “drawAt” methods with “Square” object “s”.
- ○ Call the “findArea” and “findCircumference” methods with “Square” object “s”.
- ○ Display the all the outputs.
Filename: RightTriangle.java
- • Define the “RightTriangle.java” class extends from “ShapeBase” class that implements “ShapeInterface”.
- • Declare the required variables.
- • Define the default constructor, and constructor for the class.
- • Define the “set” method.
- ○ Set the value.
- • Define “drawHere” method.
- ○ Call the “drawTop” method.
- • Define the “drawTop” method.
- ○ Declare the variable “startOfLine” and assign the value of offset plus base divided by 2.
- ○ Call the “skipSpaces()” method with “startOfLine” value.
- ○ Display the “*” character.
- ○ Declare the variable “lineCount” and assign the value of base divided by 2 minus 1.
- ○ Declare the “insideWidth” with value 1.
- ○ The “for” condition is used to display the “*” character in triangle shape.
- ■ Decrement the “startOfLine” value.
- ■ Call the “skipSpaces()” method with “startOfLine” value.
- ■ Display the “*” character.
- ■ Call the “skipSpaces()” method with “insideWidth” value.
- ■ Display the “*” character.
- ■ Increment the “insideWidth” value by 1.
- ○ Call the “drawBase” method with an argument.
- • Define “drawBase” method.
- ○ Call the “skipSpaces()” method.
- ○ Display the “*” character in base of the triangle.
- • Define “skipSpaces()” method.
- ○ Display the space.
- • Define the “findArea” method.
- ○ Return the area of right triangle.
- • Define the “findCircumference” method.
- ○ Return perimeter of the right triangle.
Filename: Rectangle.java
- • Define the “Rectangle.java” class extends from “ShapeBase” class that implements “ShapeInterface”.
- • Include all the methods in the Listing 8.13 in chapter 8.
- • Define another constructor with two parameters.
- ○ Set the values.
- • Define the “findArea” method.
- ○ Return the area of rectangle.
- • Define the “findCircumference” method.
- ○ Return perimeter of the rectangle.
Filename: Square.java
- • Define the “Sqaure.java” class which extends from the “Rectangle” class that implements “ShapeInterface”.
- • Declare the required variables.
- • Define the constructor for the class.
- • Define the accessor and mutator methods.
- • Define the “findArea” method.
- ○ Return the area of square.
- • Define the “findCircumference” method.
- ○ Return perimeter of the square.
Filename: ShapeInterface.java
Define the interface “SpaceInterface”.
- • Declare the “setOffset()” method.
- • Declare the “getOffset()” method.
- • Declare the “drawAt()” method.
- • Declare the “drawHere()” method.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Implement the Shape hierarchy -- create an abstract class called Shape, which will be the parent class to TwoDimensionalShape and ThreeDimensionalShape. The classes Circle, Square, and Triangle should inherit from TwoDimensionalShape, while Sphere, Cube, and Tetrahedron should inherit from ThreeDimensionalShape.Each TwoDimensionalShape should have the methods getArea() and getPerimeter(), which calculate the area and perimeter of the shape, respectively. Every ThreeDimensionalShape should have the methods getArea() and getVolume(), which respectively calculate the surface area and volume of the shape. Every class should have a member variable containing its dimensions -- for example, the Circle class should have a member variable describing its radius, while the Triangle class should have three member variables describing the length of each side. Note that the Tetrahedron cass should describe a regular tetrahedron, and as such, should only have one member variable.Create a Driver class…
Implement a class Season that represents a season during a specified year. For purposes of thisproblem, assume that the seasons in each year are, in order, 'Winter', 'Spring', 'Summer', 'Autumn' .__init__, __repr__A Season object is created by calling the constructor and supplying two optional arguments:1. season, defaults to 'Winter'2. calendar year, an int , defaults to 2023For the __repr__ , see the usage below:
The next and prev methods modify a Season object by changing it to the next season or previousseason in sequence, respectively. Note that the seasons can "roll over" or "roll back" and that this maychange the year by +/-1. 1. During each year, the seasons occur in this sequence: 'Winter', 'Spring', 'Summer', 'Autumn' 2. next - change to next season, if the current season is Autumn then year will increase by 1 3. prev - change to previous season, if Winter then year will decrease by 1
Implement the == operator ( __eq__ method) to compare two Season…
In Java. Screenshot explains.
Implement the Shape hierarchy -- create an abstract class called Shape, which will be the parent class to TwoDimensionalShape and ThreeDimensionalShape. The classes Circle, Square, and Triangle should inherit from TwoDimensionalShape, while Sphere, Cube, and Tetrahedron should inherit from ThreeDimensionalShape.Each TwoDimensionalShape should have the methods getArea() and getPerimeter(), which calculate the area and perimeter of the shape, respectively. Every ThreeDimensionalShape should have the methods getArea() and getVolume(), which respectively calculate the surface area and volume of the shape. Every class should have a member variable containing its dimensions -- for example, the Circle class should have a member variable describing its radius, while the Triangle class should have three member variables describing the length of each side. Note that the Tetrahedron cass should describe a regular tetrahedron, and as such, should only have one member…
Chapter 8 Solutions
Java: Introduction to Problem Solving and Programming
Ch. 8.1 - Prob. 1STQCh. 8.1 - Suppose the class SportsCar is a derived class of...Ch. 8.1 - Suppose the class SportsCar is a derived class of...Ch. 8.1 - Can a derived class directly access by name a...Ch. 8.1 - Can a derived class directly invoke a private...Ch. 8.1 - Prob. 6STQCh. 8.1 - Suppose s is an object of the class Student. Base...Ch. 8.2 - Give a complete definition of a class called...Ch. 8.2 - Add a constructor to the class Student that sets...Ch. 8.2 - Rewrite the definition of the method writeoutput...
Ch. 8.2 - Rewrite the definition of the method reset for the...Ch. 8.2 - Can an object be referenced by variables of...Ch. 8.2 - What is the type or types of the variable(s) that...Ch. 8.2 - Prob. 14STQCh. 8.2 - Prob. 15STQCh. 8.2 - Consider the code below, which was discussed in...Ch. 8.2 - Prob. 17STQCh. 8.3 - Prob. 18STQCh. 8.3 - Prob. 19STQCh. 8.3 - Is overloading a method name an example of...Ch. 8.3 - In the following code, will the two invocations of...Ch. 8.3 - In the following code, which definition of...Ch. 8.4 - Prob. 23STQCh. 8.4 - Prob. 24STQCh. 8.4 - Prob. 25STQCh. 8.4 - Prob. 26STQCh. 8.4 - Prob. 27STQCh. 8.4 - Prob. 28STQCh. 8.4 - Are the two definitions of the constructors given...Ch. 8.4 - The private method skipSpaces appears in the...Ch. 8.4 - Describe the implementation of the method drawHere...Ch. 8.4 - Is the following valid if ShapeBaSe is defined as...Ch. 8.4 - Prob. 33STQCh. 8.5 - Prob. 34STQCh. 8.5 - What is the difference between what you can do in...Ch. 8.5 - Prob. 36STQCh. 8 - Consider a program that will keep track of the...Ch. 8 - Implement your base class for the hierarchy from...Ch. 8 - Draw a hierarchy for the components you might find...Ch. 8 - Suppose we want to implement a drawing program...Ch. 8 - Create a class Square derived from DrawableShape,...Ch. 8 - Create a class SchoolKid that is the base class...Ch. 8 - Derive a class ExaggeratingKid from SchoolKid, as...Ch. 8 - Create an abstract class PayCalculator that has an...Ch. 8 - Derive a class RegularPay from PayCalculator, as...Ch. 8 - Create an abstract class DiscountPolicy. It should...Ch. 8 - Derive a class BulkDiscount from DiscountPolicy,...Ch. 8 - Derive a class BuyNItemsGetOneFree from...Ch. 8 - Prob. 13ECh. 8 - Prob. 14ECh. 8 - Create an interface MessageEncoder that has a...Ch. 8 - Create a class SubstitutionCipher that implements...Ch. 8 - Create a class ShuffleCipher that implements the...Ch. 8 - Define a class named Employee whose objects are...Ch. 8 - Define a class named Doctor whose objects are...Ch. 8 - Create a base class called Vehicle that has the...Ch. 8 - Create a new class called Dog that is derived from...Ch. 8 - Define a class called Diamond that is derived from...Ch. 8 - Prob. 2PPCh. 8 - Prob. 3PPCh. 8 - Prob. 4PPCh. 8 - Create an interface MessageDecoder that has a...Ch. 8 - For this Programming Project, start with...Ch. 8 - Modify the Student class in Listing 8.2 so that it...Ch. 8 - Prob. 8PPCh. 8 - Prob. 9PPCh. 8 - Prob. 10PP
Knowledge Booster
Similar questions
- We have to calculate the area of a rectangle, a square and a circle.Create an abstract class 'Shape' with 2 dimension fields and an abstractmethod namely 'area'. Now, write 3 classes ‘Rectangle', 'Circle' and‘Triangle’ derived from the ‘Shape’ class. Implement the area() methodas necessary in each class.Now create another class 'Geometry' containing the main method andcreate objects of 'Rectangle', 'Square' and 'Circle' and printing theirarea.arrow_forwardConsider a class BankAccount that has • Two attributes i.e. accountID and balance and• A function named balanceInquiry() to get information about the current amount in the account Derive two classes from the BankAccount class i.e. CurrentAccount and the SavingsAccount. Both classes (CurrentAccount and SavingsAccount) inherit all attributes/behaviors from the BankAccount class. In addition, followings are required to be the part of both classes• Appropriate constructors to initialize data fields of base class• A function named amountWithdrawn(amount) to withdraw certain amount while taken into account the following conditionso While withdrawing from current account, the minimum balance should not decrease Rs. 5000o While withdrawing from savings account, the minimum balance should not decrease Rs. 10,000• amountDeposit(amount) to deposit amount in the accountIn the main() function, create instances of derived classes (i.e. CurrentAccount and SavingsAccount) and invoke their respective…arrow_forwardConsider the case of a shop that sells CDs in cash. The CDs are of three types: Movie CDs, Software CDs and Music CDs. For each of the following CD types, the required attributes are:Movie CD: Movie Title, Year of Release, Lead Actor Name, CD price and CD quantitySoftware CD: Software Name, Edition, Year of Release, CD price and CD quantityMusic CD: Album Title, Year of Release, Number of Songs, Format, CD price and CD quantity All objects of the classes cannot change their state once they are instantiated. How will you implement this?arrow_forward
- Write a complete program for the description given below. Consider a Billing class that implements an interface Payable having a method getTotalPayment Amount(). Besides this, you have a Doctor class with private instance variables (docID, docName, and docFee) and a public getDoc() method, Patient class with private instance variables (pName, pID. pDisease), Medicine class with private instance variables (medID, medName, medQty, medPrice), and Medical Test class with private instance variables (testID, testName, testPrice). Each of these classes has the toString() method to display the information of its object. The Billing class is having "Has A" relationship with the other four classes (Doctor, Patient, Medicine, and MedicalTest) mentioned above. The getPayment Amount() method of Billing class returns the total billing amount that includes doc fee, medicine cost, and medical test fee that a patient has to pay. After implementing these classes, you are required to do the following in…arrow_forwardFor this exercise, you are given a Team superclass with a BaseballTeam and FootballTeam subclass. Take a moment to examine these classes and the instance variables and methods in each. In the TeamTester class, you will see three objects declared and instantiated using the Team, FootballTeam, or BaseballTeam classes. For each object, use a print statement to print out any public method that returns information that is available for that particular object, including the toString. For example, the dolphin object is a FootballTeam object. One of the pieces of information that can be printed is the getTies() method, so you will call that method in your TeamTester file: System.out.println(dolphins.getTies()); Do this for all the methods that each of the objects can call. Hint: Remember, for the program to compile and run, Java looks for methods to exist in certain classes. ================================= public class Team { private String name;private String location;private int…arrow_forwardCreate a parent class Person with one attribute age and one method greet() that prints out "Hello, world!". By default, the age is 20. Then create a subclass CollegeStudent that inherits from Person and has its own attributes School_Name and Major. Then create an object (a college student, his name is Harry, age is 21, School_Name is "Felician University", and Major is "Computer Science"), then let Harry say"Hello World!".arrow_forward
- Take the tax program from the last homework assignment and implement it using classes and objects instead. To do so, create a class definition for a class called Customer with attributes income and tax. It should also have a set method for income, and a calcTax() method to assign tax and return it. As a reminder, the tax is calculated as follows: Income Tax Due $0 - $50,000 5% $50,000-$100,000 $2,500 + 10% of (income > $50,000) > $100,000 $7,500 + 15% of (income > $100,000) Create a Customer object in main, have the user enter the income and assign this to the income variable of the Customer object, and then call the calcTax() method for the Customer object and print the tax due.arrow_forwardSolve this in java oop. In order to handle the “Carbon Footprint”, your job is to create an interface called CarbonFootPrintProducer that shall contain a method declaration called getCarbonFootPrint(), that shall return a double value indicating the amount of CO2 generated by an instance of the implementing class. Your next job is to create two classes i.e. House and Car, both shall implement CarbonFootPrintProducer interface. House must have an attribute coveredArea, while Car must have an attribute milesDriven, both public. Create fully parametrized constructor in each subclass. Both subclasses must contain their own getCarbonFootPrint() implementation that shall calculate the CO2. For the calculation of CO2, use the following formulas. CO2 produced by House = coveredArea * 0.005 CO2 produced by a Car = milesDriven * 0.0000292 Create a class CarbonFootPrintTest. Define static method calculateTotalFootPrint(ArrayList entities) method that shall calculate and return total CO2 of…arrow_forwardComplete the class LeprechaunGold to model a stash of gold coins at the end of the rainbow. There is a maximum capacity to the number of gold coins a leprechaun can store at any given time (the pot they store them in can only hold so many). The pot also holds a current amount. What does the LeprechaunGold object need to remember? Those are the instance variables. Provide a constructor that takes the maximum capacity as a parameter. The constructor initializes the instance variables (two of them). Provide methods public int getCapacity() - gets the maximum capacity public int getAmount() - gets the number of coins in the pot public void donate(int coins) - simulates making a donation public void save(int coins) - simulates adding more coins to the potarrow_forward
- Imagine you have two classes: Employee (which represents being an employee) and Ninja (which represents being a Ninja). An Employee has both state and behaviour; a Ninja has only behaviour. You need to represent an employee who is also a ninja (a common problem in the real world). By creating only one interface and only one class (NinjaEmployee), show how you can do this without having to copy method implementation code from either of the original classes.arrow_forwardHello, is this getName function requiring a getter in the Poodle.java subclass? If so, is there another way of calling the name as theres a restriction of not creating a getter. A hint I've gotten was "Does the Catfish class have access to the protected name field in Fish?" And a comment regarding "as a subclass of Fish it does, so an instance of Catfish should have access to it's parent class variables, even within another class." but I'm still kinda confused.arrow_forwardNow we are going to use the design pattern for collecting objects. We are going to create two classes, a class AmazonOrder that models Amazon orders and a class Item that models items in Amazon orders. An item has a name and a price, and the name is unique. The Item class has a constructor that takes name and price, in that order. The class also has getters and setters for the instance variables. This is the design pattern for managing properties of objects. The setName() method should do nothing if the parameter is the empty string, and the setPrice() method should do nothing if the parameter is not positive. The class also has a toString() method that returns a string representation for the item in the format “Item[Name:iPad,Price:399.99]”. For simplicity, we assume an Amazon order can have at most 5 items, and class AmazonOrder has two instance variables, an array of Item with a length of 5 and an integer numOfItems to keep track of the number of items in the…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
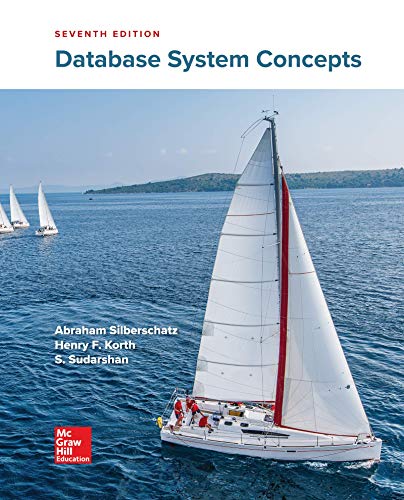
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
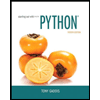
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
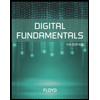
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
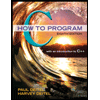
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
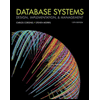
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
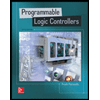
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education