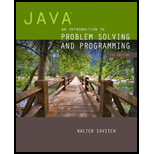
For this
Modify the program in Listing 8.6 to include at least one Faculty object and at least one Staff object in addition to the Undergraduate and Student objects. Without modification to the for loop, the report should output the name, employee ID, department, and title for the Faculty objects, and the name, employee ID, department, and pay grade for the Staff objects.

Want to see the full answer?
Check out a sample textbook solution
Chapter 8 Solutions
Java: Introduction to Problem Solving and Programming
Additional Engineering Textbook Solutions
Experiencing MIS
C++ How to Program (10th Edition)
Starting Out with C++ from Control Structures to Objects (9th Edition)
Objects First with Java: A Practical Introduction Using BlueJ (6th Edition)
Starting Out with Python (3rd Edition)
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
- Consider the following code snippet. 1. /* 2. * The Student class models a student with a name, age 3. * and a faculty in which is currently enrolled in. 4. */ 5. public class Student 6. { 7. // private instance variable, not accessible from outside this class 8. private String firstName; 9. private String lastName; 10. private int birthYear; 11. private String faculty; 12. 13. // The constructor with the. correspondent parameters. 14. public Student(String first, String last, int birthYear, String faculty) 15. { 16. this.firstName= first; 17. this.lastName = last; 18. this.birthYear = birthYear; 19. this.faculty = faculty; 20. } 21. } 1. Implement an accessor method that returns the age of the student given as parameter the current year. 2. Implement a new method called printDetails, taking the currentYear as a parameter, that prints the full name, age and faculty of the student in the following way: Full name: Donald Duck Age: 24 years Faculty:…arrow_forwardClass Student needs to create different constructors, but only have the initialization of instances done in a single place. Analyse and the process used, then give an example for class Student (choose random instances). USING JAVA PLZ, "OBJECT ORIENTED PROGRAMMING".arrow_forwardWrite a complete program for the description given below. Consider a Billing class that implements an interface Payable having a method getTotalPayment Amount(). Besides this, you have a Doctor class with private instance variables (docID, docName, and docFee) and a public getDoc() method, Patient class with private instance variables (pName, pID. pDisease), Medicine class with private instance variables (medID, medName, medQty, medPrice), and Medical Test class with private instance variables (testID, testName, testPrice). Each of these classes has the toString() method to display the information of its object. The Billing class is having "Has A" relationship with the other four classes (Doctor, Patient, Medicine, and MedicalTest) mentioned above. The getPayment Amount() method of Billing class returns the total billing amount that includes doc fee, medicine cost, and medical test fee that a patient has to pay. After implementing these classes, you are required to do the following in…arrow_forward
- Below we have class diagrams with three classes for a simple java based parking management class. Use the classes below to write java code for classes :a parking observer class (that implements an observer pattern) , a transaction manager class and a parking lot class(subject to the observer). Use the class diagram and java class to implement it. You should use an observer pattern to implement your code.The main goal is to implement the following - Once a car enters (in an entry-scan only lot) or leaves (in an entry-scan and exit-scan lot), then the ParkingObserver will be updated, and then can register the charge with the parking system via the TransactionManager’s park() methodarrow_forwardWrite a class named Person with data attributes for a person’s name, address, and telephonenumber. Next, write a class named Customer that is a subclass of the Person class. TheCustomer class should have a data attribute for a customer number, and a Boolean data attributeindicating whether the customer wishes to be on a mailing list. Demonstrate an instance of theCustomer class in a simple program.arrow_forwardConsider the above scenario, where Billing class has composition relationship with Doctor having private instance variables (docName, docID and docFee) and a public getDocID() method, Patient having private instance variables (pName, pID, pDisease), Medicine having private instance variables (medID, medName, medQty, medPrice), and MedicalTest having private instance variables (testID, testName, testPrice). In addition, each class shall have toString method to display its object state. Also define getDocInfo method in Billing class that shall return the doctor. Implement getPaymentAmount() method in Billing class that shall return the total billing amount that includes doctor fee, medicine cost and medical tests fee. Considering the above scenario, write code that shall perform the following in the driver/test class: Get an amount from user. Traverse the ArrayList<Billing> using enhanced for loop. Print the billing details of those bills having total billing amount greater or…arrow_forward
- In an Institute, faculties are planning to conduct activities for students [like workshop, seminar, codeathon …] as well as for Faculties [Training Programs, Faculty Development Programs, …] Define a class for the same with appropriate data members and also inherit to Student and Faculty Subclasses. In main method, if two faculties are planning any event on the same day, display warning message.arrow_forwardwrite a c++ program dont use high end programming symbol such as this-> A single Employee can not belong to multiple Companies (legally!! ), but if we delete the Company, Employee object will not destroy. Identify the relationship between the classes and Implement the given scenerio in the photo using C++arrow_forwardUsing Python, create a program that has the following classes: Point - Just like in the lecture, with attributes x and y set to 0 by default. Circle - Contains two attributes, center and radius. center is of type Point defined above (initialize a Point instance for this), while radius is set to 0 by default. The program must have the function point_in_circle(c, p) where c is a Circle and p is a Point. The function returns True if the point is within the circle, and False otherwise. You may use the math module (and nothing else) if necessary. You may also create other functions that can help you code the main function much easier. Example usage # Initialize a circle with center at (0, 0) and radius 1 (unit circle). c = Circle() c.center.x, c.center.y, c.radius = 0, 0, 1 # Initialize a point located at (1, 1) p = Point() p.x, p.y = 1, 1 # This returns False since (1, 1) is outside the circle point_in_circle(c, p) hint: apply "classes" conceptarrow_forward
- Using JAVA Language Consider a Billing class that implements an interface Payable having a method getTotalPaymentAmount(). Besides this, you have a Doctor class with private instance variables (docID, docName, and docFee) and a public getDoc() method, Patient class with private instance variables (pName, pID, pDisease), Medicine class with private instance variables (medID, medName, medQty, medPrice), and MedicalTest class with private instance variables (testID, testName, testPrice). Each of these classes has the toString() method to display the information of its object. The Billing class is having "Has A" relationship with the other four classes (Doctor, Patient, Medicine, and MedicalTest) mentioned above. The getPaymentAmount() method of Billing class returns the total billing amount that includes doc fee, medicine cost, and medical test fee that a patient has to pay. After implementing these classes, you are required to do the following in the driver class: Create an ArrayList of…arrow_forwardConsider a class BankAccount that has • Two attributes i.e. accountID and balance and• A function named balanceInquiry() to get information about the current amount in the account Derive two classes from the BankAccount class i.e. CurrentAccount and the SavingsAccount. Both classes (CurrentAccount and SavingsAccount) inherit all attributes/behaviors from the BankAccount class. In addition, followings are required to be the part of both classes• Appropriate constructors to initialize data fields of base class• A function named amountWithdrawn(amount) to withdraw certain amount while taken into account the following conditionso While withdrawing from current account, the minimum balance should not decrease Rs. 5000o While withdrawing from savings account, the minimum balance should not decrease Rs. 10,000• amountDeposit(amount) to deposit amount in the accountIn the main() function, create instances of derived classes (i.e. CurrentAccount and SavingsAccount) and invoke their respective…arrow_forwardWrite an abstract class, it can be anything well defined from the very basics.arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
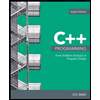
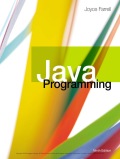