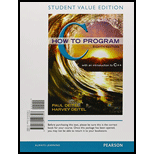
Concept explainers
(Character Testing) Write a

Program Plan:
- mainfunction used to test all the functions.
- Declare character type of variable character.
- printf function used to print the formatted output on console.
- scanf function used to takea formatted inputfrom console.
Program Description:
The following program will input character and test all the character-handling library functions.
Explanation of Solution
Program:
//header file included #include <stdio.h> #include <string.h> #include <stdlib.h> //included main function intmain() { //declare character type variable char character; //display message to user printf("Enter the character:\n"); //input character scanf("%c", &character); //testing each function of character-handling library //isdigit function printf("'%c'%sdigit\n",character, isdigit(character)?"is a ":"is not a "); //isalpha function printf("'%c'%salphabet\n",character, isalpha(character)?"is a ":"is not a "); //isalnum function printf("'%c'%salpha-numeric\n",character, isalnum(character)?"is a ":"is not a "); //isxdigit function printf("'%c'%shexadecimal digit\n",character, isxdigit(character)?"is a ":"is not a "); //islower function printf("'%c'%slowercase letter\n",character, islower(character)?"is a ":"is not a "); //isupper function printf("'%c'%suppercase letter\n",character, isupper(character)?"is a ":"is not a "); //toupper function printf("The uppercase letter of '%c' is '%c'\n",character, toupper(character)); //tolower function printf("The lowercase letter of '%c' is '%c'\n",character, tolower(character)); //isspace function printf("'%c'%sspace\n",character, isspace(character)?"is a ":"is not a "); //iscntrl function printf("'%c'%scontrol character\n",character, iscntrl(character)?"is a ":"is not a "); //ispunct function printf("'%c'%sprinting character of ispunct\n",character, ispunct(character)?"is a ":"is not a "); //isprint function printf("'%c'%sprinting character of isprint\n",character, isprint(character)?"is a ":"is not a "); //isgraph function printf("'%c'%sprinting character of isgraph\n",character, isgraph(character)?"is a ":"is not a "); //exiting from program return 0; }
Explanation:
In the above program, one character is inputted through scanf function. The %c is the format specifier used to input character type value. All the character-handling library function is used to test and print the output. The ternary operator is used inside the printf function to determine if the entered character is a digit, alphanumeric character, hexadecimal digit, lowercase letter or uppercase letter or not.
Sample Output:
Want to see more full solutions like this?
Chapter 8 Solutions
C How To Program, Student Value Edition Plus Myprogramminglab With Pearson Etext -- Access Card Package (8th Edition)
Additional Engineering Textbook Solutions
Starting Out with Programming Logic and Design (4th Edition)
Starting Out With Visual Basic (7th Edition)
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Objects First with Java: A Practical Introduction Using BlueJ (6th Edition)
Introduction To Programming Using Visual Basic (11th Edition)
Computer Science: An Overview (12th Edition)
- What’s the output of the following programs?arrow_forwardWhat is the output of the following c++ codes?arrow_forward-Convert SES variable to a factor and assign the value labels “low”, ”middle” and “high” to the 3 levels of thevariable. Then -Define the variables complic, comorb, depressi, diabetes as factors. For all these factors, a zero means “no” and a 1 means “yes”. Assign these value labels through the function factor() and check the change using str()arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
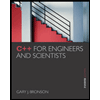
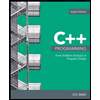