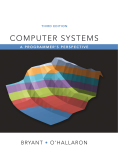
Given code:
//Include necessary header files
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <wait.h>
#include <signal.h>
#include "csapp.h"
//Initialize the global counter variable as 2
volatile long counter = 2;
//Define a function named handler1()
void handler1(int sig)
{
//Variable declaration
sigset_t mask, prev_mask;
//Call the function sigfillset()
sigfillset(&mask);
//Call the function sigprocmask() and block sigs
sigprocmask(SIG_BLOCK, &mask, &prev_mask);
//Call the function sio_putl()
sio_putl(--counter);
//Call the function sigprocmask() and restore sigs
sigprocmask(SIG_SETMASK, &prev_mask, NULL);
//Exit
_exit(0);
}
//Define a function named main()
int main()
{
//Variable declaration
pid_t pid;
sigset_t mask, prev_mask;
//Print the counter value
printf("%ld ", counter);
//Flush the output buffer using fflush()
fflush(stdout);
//Call the function signal()
signal(SIGUSR1, handler1);
//Check the condition
if ((pid = Fork()) == 0)
{
//True, execute the statement
while(1) {};
}
//Call the function kill()
Kill(pid, SIGUSR1);
//Call the function waitpid()
Waitpid(-1, NULL, 0);
//Call the function Sigfillset()
Sigfillset(&mask);
//Call the function sigprocmask() and block sigs
Sigprocmask(SIG_BLOCK, &mask, &prev_mask);
//Print the counter value
printf("%ld", ++counter);
//Call the function sigprocmask() and restore sigs
Sigprocmask(SIG_SETMASK, &prev_mask, NULL);
//Exit
exit(0);
}

Want to see the full answer?
Check out a sample textbook solution
Chapter 8 Solutions
Computer Systems: A Programmer's Perspective (3rd Edition)
- 3. The diagram below shows the main land routes for vehicular traffic between points A and G in a city. The figures in the arcs represent the cost of traveling between each pair of nodes. a) Manually apply Dijkstra's algorithm to find the cheapest route between A and G (visited nodes and total distance). b) Formulate a linear programming problem in extended form, to determine the shortest route to travel from A to G. Do not use subscripts, name 14 variables, for example XFE would be the variable that indicates that the arc from F to E is used. c) If there is a fixed cost for visiting each node, modify the formulation of the problem to include said fixed cost in the objective function, and the variables and restrictions that are required. NODE A B C D E F G FIXED COST 25 18 32 20 28 18 34arrow_forwardI need help with problem 40. What do I need to do?arrow_forwardPROBLEM 13. [TIME ALLOWED = 5 MINUTES] Give the contrapositive, converse, and inverse of the following proposition: “If the number is positive, then its square is positive.”arrow_forward
- Show complete solution please. I am at a loss of how to even begin this problem and would like to learn how to work through each step.arrow_forwardProblem 4 Discrete Mathematics.Combinations and Permutations. (5,10,10): Soccer A local high school soccer team has 20 players. However, only 11 players play at any given time during a game. In how many ways can the coach choose 11 players To be more realistic, the 11 players playing a game normally consist of 4 midfielders, 3 defend ers, 3 attackers and 1 goalkeeper. Assume that there are 7 midfielders, 6 defenders, 5 attackers and 2 goalkeepers on the team 2. In how many ways can the coach choose a group of 4 midfielders, 3 defenders, 3 attackers and 1 goalkeeper? 3. Assume that one of the defenders can also play attacker. Now in how many ways can the coach choose a group of 4 midfielders, 3 defenders, 3 attackers and 1 goalkeeper?arrow_forwardThis solution seems confusing. Please give the correct solution for this problem!!!!arrow_forward
- PROVIDE PYTHON CODE Determine the drag coefficient needed so that an 80-kg parachutist has a velocity of 36 m/safter 4s of free fall.Note: The acceleration of gravity is 9.8m/s2. Start with initial guesses of xl = 0.1 and xu = 0.2 and iterate until the approximationrelative error falls below 2 % in pythonarrow_forwardproblem 7 wasnt solvedarrow_forwardPlease answer Parts D and E of this problemarrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
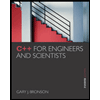