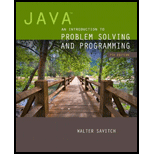
Write a class LapTimer that can be used to time the laps in a race. The class should have the following private attributes:
- running — a boolean indication of whether the timer is running
- startTime — the time when the timer started
- lapStart — the timer’s value when the current lap started
- lapTime — the elapsed time for the last lap
- totalTime — the total time from the start of the race through the last completed lap
- lapsCompleted — the number of laps completed so far
- lapsInRace — the number of laps in the race
The class should have the following methods:
- LapTimer (n) — a constructor for a race having n laps.
- start — starts the timer. Throws an exception if the race has already started.
- markLap — marks the end of the current lap and the start of a new lap. Throws an exception if the race is finished.
- getLapTime — returns the time of the last lap. Throws an exception if the first lap has not yet been completed.
- getTotalTime — returns the total time from the start of the race through the last completed lap. Throws an exception if the first lap has not yet been completed.
- getLapsRemaining — returns the number of laps yet to be completed, including the current one.
Express all times in seconds.
To get the current time in milliseconds from some baseline date, invoke
Calendar.getInstance ().getTimeInMillis ()
This invocation returns a primitive value of type long. By taking the difference between the returned values of two invocations at two different times, you will know the elapsed time in milliseconds between the invocations. Note that the class Calendar is in the package java.util.

Want to see the full answer?
Check out a sample textbook solution
Chapter 9 Solutions
Java: An Introduction to Problem Solving and Programming (7th Edition)
Additional Engineering Textbook Solutions
Database Concepts (8th Edition)
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Problem Solving with C++ (10th Edition)
Programming in C
Starting Out With Visual Basic (7th Edition)
Modern Database Management (12th Edition)
- We have two classes, Person and Employee. Both have a field, firstName. We have instances of these objectsPerson p and Employee e. Both p and e have the same value for firstName, specifically, p.firstName = “joe”and e.firstName = “joe” Which of the following statements is necessarily true? Group of answer choices 1. p.equals(e) will return true because they contain the same firstName. 2. We don’t know if either class will have an equals method. 3. There is not enough information to tell what p.equals(e) will return. 4. p.equals(e) will return false but p == e will be true.arrow_forwardWrite a program which controls a metro train and that keeps track of stations and who gets on and off. Design and implement a class called train, which has at least the following: At least the following attributes (you can include others): metroID stationNum which keeps track of the station that the train is at. iii. direction which keeps track of the direction the train is travelling in. passTotal which keeps track of the number of passengers currently on the train. A default constructor which sets the metroID to a random number between 1 and 1000, the station number to 0, the direction (int), and the passTotal to 0 (train is empty). A constructor with one parameter; the parameter is the metro id. The constructor assigns the passed integer to the metroID attribute; the rest of the attributes are set as described in the default constructor. Accessor methods for each attributes. Mutator methods for each attributes. nextStation(int lastStation) which determines the next…arrow_forwardMake a python class called CellPhone. The init() method for CellPhone needs to store two attributes: brand, and model. Make two attribute called call_time (representing total calls duration) and txt_count (representing total text message count)which both start at 0 for each new CellPhone instance.Define a method named call() that gets a call duration and updates the call_time attribute, then prints out the total calls duration so far.Define another method named txt() that each time we call it just updates the txt_count attribute by adding 1 message to that, then prints the total message count so far.In the end, create a new instance of CellPhone and run each method once (use any number for call duration argument).arrow_forward
- Create a class Rectangle with attributes length and width with are variables, each ofwhich defaults to 1. Provide methods that calculate the rectangle’s perimeter and area.It has set and get methods for both length and width. The set methods should verify thatlength and width are each floating-point numbers (double datatype) larger than 0.0 andless than 20.0. Write a Test class to test all the methods from class Rectanglearrow_forwardWrite a java code for online food delivery system to manage the data of customers who order the food using online application. User have different option for the payment i.e. Credit Card, Debit Card and Cash on Delivery. Credit card user get 10% discount on total bill, debit card customers get 7% discount while cash on delivery customers get 5% discount. Other than food bill each user have to pay delivery charges RS. 100/- Carefully think about number of classes and attribute for each class. All attributes of classes must be private and exposed via getter and setter. For all classes, create fully parameterized constructor. Only Java Write a test class named TerminalTest. In test class, show a menu to user to input data of customers, as well as food items. To input data you have to create static methods. Create another static method to order food items and calculate bill. Input data will be stored in database. Your program should handle all checked exceptions. Once data is saved in…arrow_forwardCreate a class Rectangle with attributes length and width with are variables, each ofwhich defaults to 1. Provide methods that calculate the rectangle’s perimeter and area.It has set and get methods for both length and width. The set methods should verify thatlength and width are each floating-point numbers (double datatype) larger than 0.0 andless than 20.0. Write a Test class to test all the methods from class Rectangle.RectangleTest.java source file should looks like this:class Rectangle{//data fileds//methods}//end Rectangle classpublic class RectangleTest{ //main method}//end RectangleTest classarrow_forward
- Write in java an abstract class Student that includes the following hidden attributes: id(int), name(String), major(String) and grade(double), then create setter and getter for each of them. Write two classes that inherited from Student: ItStudent and ArtStudent. The grade for ItStudent is calculated as: grade = mid*0.30 + project*0.30 + final*40 and the grade for ArtStudent is calculated as: grade = mid*0.40 + report*0.10 + final*50. Crate main class that achieve the following:a. Create an array of some Student objects from both ItStudent and ArtStudent classes.b. Sort the Student objects by grade in descending order using functions.c. Save the sorted objects into file.arrow_forwardDesign and implement a class dayType that implements the day of the week in a program. The class dayType should store the day, such as Sun for Sunday. The program should be able to perform the following operations on an object of type dayType:a. Set the day.b. Print the day.c. Return the day.d. Return the next day.e. Return the previous day.f. Calculate and return the day by adding certain days to the current day. For example, if the current day is Monday and we add 4 days, the day to be returned is Friday. Similarly, if today is Tuesday and we add 13 days, the day to be returned is Monday.g. Add the appropriate constructors.arrow_forwardMake a subclass Food of the Product class. Besides the description and price inherited from Product, Food also has an instance variable carbs (a double). Do not redefine price and description variables. Call the super class to initialize them. Provide Javadoc. Food has methods: public double getCarbs() public boolean isZeroCarb() which tells if this Food can be considered zero carb. A Food is considered zero carb if it has less than 1 gram of carbohydrates per serving. Return true if the Food is zero carb. Otherwise, return false. public String getDescription() overrides the getDescription method in Product to also include the number of carbs. Call the getDescription method in the super class and add the new information on the end. The return string will look like this: spinach carbs=0.2arrow_forward
- Complete the class LeprechaunGold to model a stash of gold coins at the end of the rainbow. There is a maximum capacity to the number of gold coins a leprechaun can store at any given time (the pot they store them in can only hold so many). The pot also holds a current amount. What does the LeprechaunGold object need to remember? Those are the instance variables. Provide a constructor that takes the maximum capacity as a parameter. The constructor initializes the instance variables (two of them). Provide methods public int getCapacity() - gets the maximum capacity public int getAmount() - gets the number of coins in the pot public void donate(int coins) - simulates making a donation public void save(int coins) - simulates adding more coins to the potarrow_forwardConsider an online holiday booking system. The users can register and log in to book a holiday package. The software development team is planning to apply the Gang of Four (GoF) Singleton pattern to the Security_Check class (as the Singleton class) which will monitor customers’ sign in activities. Which of the following is FALSE? Select one: a. The Security_Check class should provide a private method for the access of its object by external objects. b. The get method for the Security_Check object should be public. c. The Security_Check class should be responsible for creating one object to deal with different requests. d. The Security_Check class should have a private attribute for its object.arrow_forwardConsider an online holiday booking system. The users can register and log in to book a holiday package. The software development team is planning to apply the Gang of Four (GoF) Singleton pattern to the Security_Check class (as the Singleton class) which will monitor customers’ sign in activities. Which of the following is FALSE? Select one: a. The Security_Check class should provide a private method for the access of its object by external objects. b. The get method for the Security_Check object should be public. c. The Security_Check class should be responsible for creating one object to deal with different requests. d. The Security_Check class should have a private attribute for its object. I just need only the answerarrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
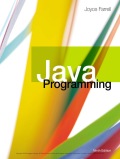